MAX7032 Transceiver Mbed Driver
Embed:
(wiki syntax)
Show/hide line numbers
Max7032.cpp
00001 /******************************************************************************* 00002 * Copyright(C) Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files(the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc.shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc.Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc.retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include <iostream> 00035 #include "Max7032.h" 00036 #include "math.h" 00037 00038 using namespace std; 00039 00040 const uint8_t rx_quick_start[Q_START_CONF_LEN] = {0xFA,0x04,0x00,0x02,0x00,0xB0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00}; 00041 const uint8_t tx_quick_start[Q_START_CONF_LEN] = {0xFE,0x04,0x40,0x02,0x00,0xB0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x89,0xB5,0x00,0x00}; 00042 00043 MAX7032::MAX7032(SPI *spi, DigitalOut *cs, DigitalOut *powerPin, PinName dataPin, DigitalOut *dioPin, DigitalOut *trxPin) 00044 { 00045 operation_mode = UNINITIALIZED; 00046 00047 if (spi == NULL || cs == NULL || powerPin == NULL || dataPin == NULL || dioPin == NULL || trxPin == NULL) 00048 return; 00049 00050 max7032_reg_map_t reg; 00051 00052 reg_map = ® 00053 spi_handler = spi; 00054 ssel = cs; 00055 power_pin = powerPin; 00056 data_pin = dataPin; 00057 dio = dioPin; 00058 trx_pin = trxPin; 00059 preset_mode = 0; 00060 00061 encoding = Manchester; 00062 f_xtal = 16.0f; 00063 f_rf = 315.0f; 00064 fsk_dev = 0.05f; 00065 00066 data_rate = 1.0f; 00067 data_read = NULL; 00068 data_send = NULL; 00069 00070 if (initial_programming() < 0) 00071 return; 00072 00073 operation_mode = INITIALIZED; 00074 } 00075 00076 MAX7032::MAX7032(SPI *spi, DigitalOut *powerPin, PinName dataPin, DigitalOut *dioPin, DigitalOut *trxPin) 00077 { 00078 operation_mode = UNINITIALIZED; 00079 00080 if (spi == NULL || powerPin == NULL || dataPin == NULL || dioPin == NULL || trxPin == NULL) 00081 return; 00082 00083 max7032_reg_map_t reg; 00084 00085 reg_map = ® 00086 spi_handler = spi; 00087 ssel = NULL; 00088 power_pin = powerPin; 00089 data_pin = dataPin; 00090 dio = dioPin; 00091 trx_pin = trxPin; 00092 preset_mode = 0; 00093 00094 encoding = Manchester; 00095 f_xtal = 16.0f; 00096 f_rf = 315.0f; 00097 fsk_dev = 0.05f; 00098 00099 data_rate = 1.0f; 00100 data_read = NULL; 00101 data_send = NULL; 00102 00103 if (initial_programming() < 0) 00104 return; 00105 00106 operation_mode = INITIALIZED; 00107 } 00108 00109 MAX7032::~MAX7032() 00110 { 00111 if(data_read != NULL) 00112 delete data_read; 00113 00114 if(data_send != NULL) 00115 delete data_send; 00116 } 00117 00118 int MAX7032::read_register(uint8_t reg, uint8_t *value) 00119 { 00120 00121 int rtn_val = 0; 00122 //Since 3-wire spi is not supported by mbed, the read_register function must be implemented by the user. 00123 //Here you can find an implemented read_register function for the max32630fthr. 00124 #if defined(TARGET_MAX32630FTHR) 00125 if (value == NULL) { 00126 return -1; 00127 } 00128 00129 if (this->reg_map == NULL) { 00130 return -1; 00131 } 00132 00133 spi_handler->format(8,0); 00134 spi_handler->frequency(400000); 00135 00136 if (ssel != NULL) { 00137 *ssel = 0; 00138 } 00139 spi_handler->write((uint8_t)0x80 | reg); // Set R/W bit 1 for reading 00140 spi_handler->write(0x00); // dummy write command for waiting data read 00141 00142 MAX7032_SPI->mstr_cfg |= MXC_F_SPIM_MSTR_CFG_THREE_WIRE_MODE; 00143 00144 // Disable SPI for General Control Configuration 00145 MAX7032_SPI->gen_ctrl = 0; 00146 MAX7032_SPI->gen_ctrl |= (MXC_F_SPIM_GEN_CTRL_TX_FIFO_EN | MXC_F_SPIM_GEN_CTRL_RX_FIFO_EN | MXC_F_SPIM_GEN_CTRL_ENABLE_SCK_FB_MODE | (1 << MXC_F_SPIM_GEN_CTRL_SIMPLE_MODE_POS)); // simple header 00147 00148 MAX7032_SPI->simple_headers &= 0x0000FFFF; 00149 MAX7032_SPI->simple_headers |= 0x2016<<16; 00150 MAX7032_SPI->gen_ctrl |=MXC_F_SPIM_GEN_CTRL_START_RX_ONLY; 00151 00152 if (ssel != NULL) { 00153 *ssel = 1; 00154 wait_us(5); 00155 *ssel = 0; 00156 } 00157 00158 // Enable the SPI 00159 MAX7032_SPI->gen_ctrl |= MXC_F_SPIM_GEN_CTRL_SPI_MSTR_EN; 00160 00161 volatile mxc_spim_fifo_regs_t *fifo; 00162 00163 fifo = MXC_SPIM_GET_SPIM_FIFO(MXC_SPIM_GET_IDX(MAX7032_SPI)); 00164 00165 int avail = ((MAX7032_SPI->fifo_ctrl & MXC_F_SPIM_FIFO_CTRL_RX_FIFO_USED) >> MXC_F_SPIM_FIFO_CTRL_RX_FIFO_USED_POS); 00166 00167 Timer *t = NULL; 00168 t = new Timer(); 00169 00170 t->start(); 00171 00172 while (avail < 1) { 00173 if (t->read_ms() > 1000) { 00174 rtn_val = -1; 00175 break; 00176 } else { 00177 avail = ((MAX7032_SPI->fifo_ctrl & MXC_F_SPIM_FIFO_CTRL_RX_FIFO_USED) >> MXC_F_SPIM_FIFO_CTRL_RX_FIFO_USED_POS); 00178 } 00179 } 00180 00181 t->stop(); 00182 00183 *(value++) = fifo->rslts_8[0]; 00184 00185 while (MAX7032_SPI->fifo_ctrl & MXC_F_SPIM_FIFO_CTRL_RX_FIFO_USED) { 00186 fifo->rslts_8[0]; 00187 } 00188 00189 MAX7032_SPI->gen_ctrl = 0; 00190 MAX7032_SPI->gen_ctrl |= (MXC_F_SPIM_GEN_CTRL_TX_FIFO_EN | MXC_F_SPIM_GEN_CTRL_RX_FIFO_EN | MXC_F_SPIM_GEN_CTRL_ENABLE_SCK_FB_MODE | (0 << MXC_F_SPIM_GEN_CTRL_SIMPLE_MODE_POS)); // simple header 00191 00192 MAX7032_SPI->gen_ctrl |= MXC_F_SPIM_GEN_CTRL_SPI_MSTR_EN; 00193 00194 if (ssel != NULL) { 00195 *ssel = 1; 00196 } 00197 00198 t->~Timer(); 00199 delete t; 00200 #else 00201 if (value == NULL) { 00202 return -1; 00203 } 00204 00205 if (this->reg_map == NULL) { 00206 return -2; 00207 } 00208 00209 spi_handler->format(8,0); 00210 spi_handler->frequency(400000); 00211 00212 if (ssel != NULL) { 00213 *ssel = 0; 00214 } 00215 spi_handler->write((uint8_t)0x80 | reg); 00216 00217 spi_handler->write(0x00); // dummy write command for waiting data read 00218 00219 *(value++) = spi_handler->write(0x00); // read back data bytes 00220 00221 if (ssel != NULL) { 00222 *ssel = 1; 00223 } 00224 #endif 00225 *dio = 0; 00226 return rtn_val; 00227 } 00228 00229 int MAX7032::write_register(uint8_t reg, const uint8_t *value, uint8_t len) 00230 { 00231 int rtn_val = -1; 00232 uint8_t local_data[1 + len]; 00233 00234 if (value == NULL) { 00235 return -1; 00236 } 00237 00238 memcpy(&local_data[0], value, len); 00239 00240 if (ssel != NULL) { 00241 *ssel = 0; 00242 } 00243 00244 rtn_val = spi_handler->write(0x40 | reg); // write mode and adress send 00245 for (int i = 0; i < len; i++) { 00246 rtn_val = spi_handler->write(local_data[i]); // write adress 00247 } 00248 00249 if (ssel != NULL) { 00250 *ssel = 1; 00251 } 00252 00253 *dio = 0; 00254 00255 if (rtn_val < 0) { 00256 return rtn_val; 00257 } 00258 00259 return 0; 00260 } 00261 00262 #define SET_BIT_FIELD(address, reg_name, bit_field_name, value) \ 00263 do { \ 00264 int ret; \ 00265 ret = read_register(address, (uint8_t *)&(reg_name)); \ 00266 if (ret) { \ 00267 return ret; \ 00268 } \ 00269 bit_field_name = value; \ 00270 ret = write_register(address, (uint8_t *)&(reg_name), 1); \ 00271 if (ret) { \ 00272 return ret; \ 00273 } \ 00274 } while (0) 00275 00276 int MAX7032::initial_programming(void) 00277 { 00278 uint8_t address; 00279 00280 set_power_on_off(1); 00281 00282 rx_quich_start(); 00283 00284 set_center_freq(f_rf); 00285 adjust_osc_freq(f_xtal); 00286 set_trx_state(MAX7032::RECEIVE_MODE); 00287 00288 return -1; 00289 } 00290 00291 int MAX7032::rx_quich_start(void) 00292 { 00293 uint8_t address; 00294 int ret; 00295 00296 for(address = 0; address < Q_START_CONF_LEN; address++) 00297 { 00298 if(address == 4) 00299 continue; 00300 00301 ret = write_register(address, (uint8_t *)&(rx_quick_start[address]), 1); 00302 if (ret) { 00303 return ret; 00304 } 00305 00306 // ((max7032_dummy_t *)(&this->reg_map->power + address))->raw = rx_quick_start[address]; 00307 } 00308 00309 this->reg_map->power.raw = rx_quick_start[POWER_ADDR]; 00310 this->reg_map->contrl.raw = rx_quick_start[CONTRL_ADDR]; 00311 this->reg_map->conf0.raw = rx_quick_start[CONF0_ADDR]; 00312 this->reg_map->conf1.raw = rx_quick_start[CONF1_ADDR]; 00313 this->reg_map->osc.raw = rx_quick_start[OSC_ADDR]; 00314 this->reg_map->toff_upper.raw = rx_quick_start[TOFFMSB_ADDR]; 00315 this->reg_map->toff_lower.raw = rx_quick_start[TOFFLSB_ADDR]; 00316 this->reg_map->tcpu.raw = rx_quick_start[TCPU_ADDR]; 00317 this->reg_map->trf_upper.raw = rx_quick_start[TRFMSB_ADDR]; 00318 this->reg_map->trf_lower.raw = rx_quick_start[TRFLSB_ADDR]; 00319 this->reg_map->ton_upper.raw = rx_quick_start[TONMSB_ADDR]; 00320 this->reg_map->ton_lower.raw = rx_quick_start[TONLSB_ADDR]; 00321 this->reg_map->txlow_upper.raw = rx_quick_start[TXLOWMSB_ADDR]; 00322 this->reg_map->txlow_lower.raw = rx_quick_start[TXLOWLSB_ADDR]; 00323 this->reg_map->txhigh_upper.raw = rx_quick_start[TXHIGHMSB_ADDR]; 00324 this->reg_map->txhigh_lower.raw = rx_quick_start[TXHIGHLSB_ADDR]; 00325 00326 return 0; 00327 } 00328 00329 int MAX7032::tx_quich_start(void) 00330 { 00331 uint8_t address; 00332 int ret; 00333 00334 for(address = 0; address < Q_START_CONF_LEN; address++) 00335 { 00336 if(address == 4) 00337 continue; 00338 00339 ret = write_register(address, (uint8_t *)&(tx_quick_start[address]), 1); 00340 if (ret) { 00341 return ret; 00342 } 00343 00344 // ((max7032_dummy_t *)(&this->reg_map->power + address))->raw = tx_quick_start[address]; 00345 } 00346 00347 this->reg_map->power.raw = tx_quick_start[POWER_ADDR]; 00348 this->reg_map->contrl.raw = tx_quick_start[CONTRL_ADDR]; 00349 this->reg_map->conf0.raw = tx_quick_start[CONF0_ADDR]; 00350 this->reg_map->conf1.raw = tx_quick_start[CONF1_ADDR]; 00351 this->reg_map->osc.raw = tx_quick_start[OSC_ADDR]; 00352 this->reg_map->toff_upper.raw = tx_quick_start[TOFFMSB_ADDR]; 00353 this->reg_map->toff_lower.raw = tx_quick_start[TOFFLSB_ADDR]; 00354 this->reg_map->tcpu.raw = tx_quick_start[TCPU_ADDR]; 00355 this->reg_map->trf_upper.raw = tx_quick_start[TRFMSB_ADDR]; 00356 this->reg_map->trf_lower.raw = tx_quick_start[TRFLSB_ADDR]; 00357 this->reg_map->ton_upper.raw = tx_quick_start[TONMSB_ADDR]; 00358 this->reg_map->ton_lower.raw = tx_quick_start[TONLSB_ADDR]; 00359 this->reg_map->txlow_upper.raw = tx_quick_start[TXLOWMSB_ADDR]; 00360 this->reg_map->txlow_lower.raw = tx_quick_start[TXLOWLSB_ADDR]; 00361 this->reg_map->txhigh_upper.raw = tx_quick_start[TXHIGHMSB_ADDR]; 00362 this->reg_map->txhigh_lower.raw = tx_quick_start[TXHIGHLSB_ADDR]; 00363 return 0; 00364 } 00365 00366 int MAX7032::set_power_on_off(uint8_t power) 00367 { 00368 00369 if (power == 0) { // Shut down the device 00370 00371 *this->power_pin = 0; 00372 00373 } else { // Turn on the device 00374 00375 *this->power_pin = 0; 00376 wait_us(1500); 00377 *this->power_pin = 1; 00378 } 00379 00380 return 0; 00381 } 00382 00383 int MAX7032::get_bit_field(reg_bits_t bit_field, uint8_t *val) 00384 { 00385 uint8_t reg_addr, bit_pos, reg_val, reg_mask; 00386 00387 reg_addr = bit_field / 8; 00388 bit_pos = bit_field % 8; 00389 00390 int ret; 00391 ret = read_register(reg_addr, (uint8_t *)&(reg_val)); 00392 if (ret) { 00393 return ret; 00394 } 00395 00396 reg_mask = 0x01; 00397 reg_val = reg_val >> bit_pos; 00398 reg_val &= reg_mask; 00399 00400 *val = reg_val; 00401 00402 return 0; 00403 } 00404 00405 int MAX7032::set_bit_field(reg_bits_t bit_field, uint8_t val) 00406 { 00407 uint8_t reg_addr, bit_pos, reg_val, reg_mask; 00408 00409 reg_addr = bit_field / 8; 00410 bit_pos = bit_field % 8; 00411 00412 int ret; 00413 ret = read_register(reg_addr, (uint8_t *)&(reg_val)); 00414 if (ret) { 00415 return ret; 00416 } 00417 00418 reg_mask = 0x01; 00419 reg_mask = reg_mask << bit_pos; 00420 00421 if(val == 0) 00422 reg_val &= ~reg_mask; 00423 else if(val == 1) 00424 reg_val |= reg_mask; 00425 else 00426 return -1; 00427 00428 ret = write_register(reg_addr, (uint8_t *)®_val, 1); 00429 if (ret) { 00430 return ret; 00431 } 00432 00433 if(reg_addr == POWER_ADDR) 00434 this->reg_map->power.raw = reg_val; 00435 else if(reg_addr == CONTRL_ADDR) 00436 this->reg_map->contrl.raw = reg_val; 00437 else if(reg_addr == CONF0_ADDR) 00438 this->reg_map->conf0.raw = reg_val; 00439 else if(reg_addr == CONF1_ADDR) 00440 this->reg_map->conf1.raw = reg_val; 00441 else 00442 return -2; 00443 00444 return 0; 00445 } 00446 00447 int MAX7032::set_lna(uint8_t lna) 00448 { 00449 if(lna > 1) 00450 return -1; 00451 00452 SET_BIT_FIELD(POWER_ADDR, this->reg_map->power, this->reg_map->power.bits.lna, lna); 00453 00454 return 0; 00455 } 00456 00457 int MAX7032::get_lna() 00458 { 00459 int ret; 00460 00461 ret = read_register(POWER_ADDR, (uint8_t *) & (this->reg_map->power)); 00462 if (ret < 0) 00463 return ret; 00464 00465 return this->reg_map->power.bits.lna; 00466 } 00467 00468 int MAX7032::set_agc(uint8_t agc) 00469 { 00470 if(agc > 1) 00471 return -1; 00472 00473 SET_BIT_FIELD(POWER_ADDR, this->reg_map->power, this->reg_map->power.bits.agc, agc); 00474 00475 return 0; 00476 } 00477 00478 int MAX7032::get_agc() 00479 { 00480 int ret; 00481 00482 ret = read_register(POWER_ADDR, (uint8_t *) & (this->reg_map->power)); 00483 if (ret < 0) 00484 return ret; 00485 00486 return this->reg_map->power.bits.agc; 00487 } 00488 00489 int MAX7032::set_mixer(uint8_t mixer) 00490 { 00491 if(mixer > 1) 00492 return -1; 00493 00494 SET_BIT_FIELD(POWER_ADDR, this->reg_map->power, this->reg_map->power.bits.mixer, mixer); 00495 00496 return 0; 00497 } 00498 00499 int MAX7032::get_mixer() 00500 { 00501 int ret; 00502 00503 ret = read_register(POWER_ADDR, (uint8_t *) & (this->reg_map->power)); 00504 if (ret < 0) 00505 return ret; 00506 00507 return this->reg_map->power.bits.mixer; 00508 } 00509 00510 int MAX7032::set_baseb(uint8_t baseb) 00511 { 00512 if(baseb > 1) 00513 return -1; 00514 00515 SET_BIT_FIELD(POWER_ADDR, this->reg_map->power, this->reg_map->power.bits.baseb, baseb); 00516 00517 return 0; 00518 } 00519 00520 int MAX7032::get_baseb() 00521 { 00522 int ret; 00523 00524 ret = read_register(POWER_ADDR, (uint8_t *) & (this->reg_map->power)); 00525 if (ret < 0) 00526 return ret; 00527 00528 return this->reg_map->power.bits.baseb; 00529 } 00530 00531 int MAX7032::set_pkdet(uint8_t pkdet) 00532 { 00533 if(pkdet > 1) 00534 return -1; 00535 00536 SET_BIT_FIELD(POWER_ADDR, this->reg_map->power, this->reg_map->power.bits.pkdet, pkdet); 00537 00538 return 0; 00539 } 00540 00541 int MAX7032::get_pkdet() 00542 { 00543 int ret; 00544 00545 ret = read_register(POWER_ADDR, (uint8_t *) & (this->reg_map->power)); 00546 if (ret < 0) 00547 return ret; 00548 00549 return this->reg_map->power.bits.pkdet; 00550 } 00551 00552 int MAX7032::set_pa(uint8_t pa) 00553 { 00554 if(pa > 1) 00555 return -1; 00556 00557 SET_BIT_FIELD(POWER_ADDR, this->reg_map->power, this->reg_map->power.bits.pa, pa); 00558 00559 return 0; 00560 } 00561 00562 int MAX7032::get_pa() 00563 { 00564 int ret; 00565 00566 ret = read_register(POWER_ADDR, (uint8_t *) & (this->reg_map->power)); 00567 if (ret < 0) 00568 return ret; 00569 00570 return this->reg_map->power.bits.pa; 00571 } 00572 00573 int MAX7032::set_rssio(uint8_t rssio) 00574 { 00575 if(rssio > 1) 00576 return -1; 00577 00578 SET_BIT_FIELD(POWER_ADDR, this->reg_map->power, this->reg_map->power.bits.rssio, rssio); 00579 00580 return 0; 00581 } 00582 00583 int MAX7032::get_rssio() 00584 { 00585 int ret; 00586 00587 ret = read_register(POWER_ADDR, (uint8_t *) & (this->reg_map->power)); 00588 if (ret < 0) 00589 return ret; 00590 00591 return this->reg_map->power.bits.rssio; 00592 } 00593 00594 00595 int MAX7032::set_agclk(uint8_t agclk) 00596 { 00597 if(agclk > 1) 00598 return -1; 00599 00600 SET_BIT_FIELD(CONTRL_ADDR, this->reg_map->contrl, this->reg_map->contrl.bits.agclk, agclk); 00601 00602 if(!get_mgain()) 00603 return -99; 00604 00605 return 0; 00606 } 00607 00608 int MAX7032::get_agclk() 00609 { 00610 int ret; 00611 00612 ret = read_register(CONTRL_ADDR, (uint8_t *) & (this->reg_map->contrl)); 00613 if (ret < 0) 00614 return ret; 00615 00616 return this->reg_map->contrl.bits.agclk; 00617 } 00618 00619 int MAX7032::set_gain(uint8_t gain) 00620 { 00621 if(gain > 1) 00622 return -1; 00623 00624 SET_BIT_FIELD(CONTRL_ADDR, this->reg_map->contrl, this->reg_map->contrl.bits.gain, gain); 00625 00626 if(!get_mgain()) 00627 return -99; 00628 00629 return 0; 00630 } 00631 00632 int MAX7032::get_gain() 00633 { 00634 int ret; 00635 00636 ret = read_register(CONTRL_ADDR, (uint8_t *) & (this->reg_map->contrl)); 00637 if (ret < 0) 00638 return ret; 00639 00640 return this->reg_map->contrl.bits.gain; 00641 } 00642 00643 int MAX7032::set_trk_en(uint8_t trk_en) 00644 { 00645 if(trk_en > 1) 00646 return -1; 00647 00648 SET_BIT_FIELD(CONTRL_ADDR, this->reg_map->contrl, this->reg_map->contrl.bits.trk_en, trk_en); 00649 00650 return 0; 00651 } 00652 00653 int MAX7032::get_trk_en() 00654 { 00655 int ret; 00656 00657 ret = read_register(CONTRL_ADDR, (uint8_t *) & (this->reg_map->contrl)); 00658 if (ret < 0) 00659 return ret; 00660 00661 return this->reg_map->contrl.bits.trk_en; 00662 } 00663 00664 int MAX7032::set_pcal() 00665 { 00666 SET_BIT_FIELD(CONTRL_ADDR, this->reg_map->contrl, this->reg_map->contrl.bits.pcal, 1); 00667 00668 return 0; 00669 } 00670 00671 int MAX7032::get_pcal() 00672 { 00673 int ret; 00674 00675 ret = read_register(CONTRL_ADDR, (uint8_t *) & (this->reg_map->contrl)); 00676 if (ret < 0) 00677 return ret; 00678 00679 return this->reg_map->contrl.bits.pcal; 00680 } 00681 00682 int MAX7032::set_fcal() 00683 { 00684 SET_BIT_FIELD(CONTRL_ADDR, this->reg_map->contrl, this->reg_map->contrl.bits.fcal, 1); 00685 00686 return 0; 00687 } 00688 00689 int MAX7032::get_fcal() 00690 { 00691 int ret; 00692 00693 ret = read_register(CONTRL_ADDR, (uint8_t *) & (this->reg_map->contrl)); 00694 if (ret < 0) 00695 return ret; 00696 00697 return this->reg_map->contrl.bits.fcal; 00698 } 00699 00700 int MAX7032::set_ckout(uint8_t ckout) 00701 { 00702 if(ckout > 1) 00703 return -1; 00704 00705 SET_BIT_FIELD(CONTRL_ADDR, this->reg_map->contrl, this->reg_map->contrl.bits.ckout, ckout); 00706 00707 return 0; 00708 } 00709 00710 int MAX7032::get_ckout() 00711 { 00712 int ret; 00713 00714 ret = read_register(CONTRL_ADDR, (uint8_t *) & (this->reg_map->contrl)); 00715 if (ret < 0) 00716 return ret; 00717 00718 return this->reg_map->contrl.bits.ckout; 00719 } 00720 00721 int MAX7032::set_sleep(uint8_t sleep) 00722 { 00723 if(sleep > 1) 00724 return -1; 00725 00726 SET_BIT_FIELD(CONTRL_ADDR, this->reg_map->contrl, this->reg_map->contrl.bits.sleep, sleep); 00727 00728 return 0; 00729 } 00730 00731 int MAX7032::get_sleep() 00732 { 00733 int ret; 00734 00735 ret = read_register(CONTRL_ADDR, (uint8_t *) & (this->reg_map->contrl)); 00736 if (ret < 0) 00737 return ret; 00738 00739 return this->reg_map->contrl.bits.sleep; 00740 } 00741 00742 int MAX7032::set_mode(ask_fsk_sel_t ask_fsk_sel) 00743 { 00744 SET_BIT_FIELD(CONF0_ADDR, this->reg_map->conf0, this->reg_map->conf0.bits.mode, ask_fsk_sel); 00745 00746 return 0; 00747 } 00748 00749 int MAX7032::get_mode(ask_fsk_sel_t* ask_fsk_sel) 00750 { 00751 int ret; 00752 00753 ret = read_register(CONF0_ADDR, (uint8_t *) & (this->reg_map->conf0)); 00754 if (ret < 0) 00755 return ret; 00756 00757 *ask_fsk_sel = (ask_fsk_sel_t)this->reg_map->conf0.bits.mode; 00758 00759 return 0; 00760 } 00761 00762 int MAX7032::get_mode() 00763 { 00764 int ret; 00765 00766 ret = read_register(CONF0_ADDR, (uint8_t *) & (this->reg_map->conf0)); 00767 if (ret < 0) 00768 return ret; 00769 00770 return this->reg_map->conf0.bits.mode; 00771 } 00772 00773 int MAX7032::set_t_r(uint8_t t_r) 00774 { 00775 if(t_r > 1) 00776 return -1; 00777 00778 SET_BIT_FIELD(CONF0_ADDR, this->reg_map->conf0, this->reg_map->conf0.bits.t_r, t_r); 00779 00780 return 0; 00781 } 00782 00783 int MAX7032::get_t_r() 00784 { 00785 int ret; 00786 00787 ret = read_register(CONF0_ADDR, (uint8_t *) & (this->reg_map->conf0)); 00788 if (ret < 0) 00789 return ret; 00790 00791 return this->reg_map->conf0.bits.t_r; 00792 } 00793 00794 int MAX7032::set_mgain(uint8_t mgain) 00795 { 00796 if(mgain > 1) 00797 return -1; 00798 00799 SET_BIT_FIELD(CONF0_ADDR, this->reg_map->conf0, this->reg_map->conf0.bits.mgain, mgain); 00800 00801 return 0; 00802 } 00803 00804 int MAX7032::get_mgain() 00805 { 00806 int ret; 00807 00808 ret = read_register(CONF0_ADDR, (uint8_t *) & (this->reg_map->conf0)); 00809 if (ret < 0) 00810 return ret; 00811 00812 return this->reg_map->conf0.bits.mgain; 00813 } 00814 00815 int MAX7032::set_drx(uint8_t drx) 00816 { 00817 if(drx > 1) 00818 return -1; 00819 00820 SET_BIT_FIELD(CONF0_ADDR, this->reg_map->conf0, this->reg_map->conf0.bits.drx, drx); 00821 00822 return 0; 00823 } 00824 00825 int MAX7032::get_drx() 00826 { 00827 int ret; 00828 00829 ret = read_register(CONF0_ADDR, (uint8_t *) & (this->reg_map->conf0)); 00830 if (ret < 0) 00831 return ret; 00832 00833 return this->reg_map->conf0.bits.drx; 00834 } 00835 00836 00837 int MAX7032::set_acal(uint8_t acal) 00838 { 00839 if(acal > 1) 00840 return -1; 00841 00842 SET_BIT_FIELD(CONF1_ADDR, this->reg_map->conf1, this->reg_map->conf1.bits.acal, acal); 00843 00844 return 0; 00845 } 00846 00847 int MAX7032::get_acal() 00848 { 00849 int ret; 00850 00851 ret = read_register(CONF1_ADDR, (uint8_t *) & (this->reg_map->conf1)); 00852 if (ret < 0) 00853 return ret; 00854 00855 return this->reg_map->conf1.bits.acal; 00856 } 00857 00858 int MAX7032::set_clkof(uint8_t clkof) 00859 { 00860 if(clkof > 1) 00861 return -1; 00862 00863 SET_BIT_FIELD(CONF1_ADDR, this->reg_map->conf1, this->reg_map->conf1.bits.clkof, clkof); 00864 00865 return 0; 00866 } 00867 00868 int MAX7032::get_clkof() 00869 { 00870 int ret; 00871 00872 ret = read_register(CONF1_ADDR, (uint8_t *) & (this->reg_map->conf1)); 00873 if (ret < 0) 00874 return ret; 00875 00876 return this->reg_map->conf1.bits.clkof; 00877 } 00878 00879 int MAX7032::set_cdiv(uint8_t cdiv) 00880 { 00881 if(cdiv > 3) 00882 return -1; 00883 00884 SET_BIT_FIELD(CONF1_ADDR, this->reg_map->conf1, this->reg_map->conf1.bits.cdiv, cdiv); 00885 00886 return 0; 00887 } 00888 00889 int MAX7032::get_cdiv() 00890 { 00891 int ret; 00892 00893 ret = read_register(CONF1_ADDR, (uint8_t *) & (this->reg_map->conf1)); 00894 if (ret < 0) 00895 return ret; 00896 00897 return this->reg_map->conf1.bits.cdiv; 00898 } 00899 00900 int MAX7032::set_dt(uint8_t dt) 00901 { 00902 if(dt > 7) 00903 return -1; 00904 00905 SET_BIT_FIELD(CONF1_ADDR, this->reg_map->conf1, this->reg_map->conf1.bits.dt, dt); 00906 00907 return 0; 00908 } 00909 00910 int MAX7032::get_dt() 00911 { 00912 int ret; 00913 00914 ret = read_register(CONF1_ADDR, (uint8_t *) & (this->reg_map->conf1)); 00915 if (ret < 0) 00916 return ret; 00917 00918 return this->reg_map->conf1.bits.dt; 00919 } 00920 00921 int MAX7032::set_osc(uint8_t osc) 00922 { 00923 SET_BIT_FIELD(OSC_ADDR, this->reg_map->osc, this->reg_map->osc.bits.osc, osc); 00924 00925 return 0; 00926 } 00927 00928 int MAX7032::get_osc() 00929 { 00930 int ret; 00931 00932 ret = read_register(OSC_ADDR, (uint8_t *) & (this->reg_map->osc)); 00933 if (ret < 0) 00934 return ret; 00935 00936 return this->reg_map->osc.bits.osc; 00937 } 00938 00939 int MAX7032::set_toff(uint16_t toff) 00940 { 00941 uint8_t toff_lsb, toff_msb; 00942 00943 if(toff > 65535) 00944 return -99; 00945 00946 toff_lsb = (uint8_t)(toff & 0x00FF); 00947 toff_msb = (uint8_t)((toff & 0xFF00) >> 8); 00948 00949 SET_BIT_FIELD(TOFFMSB_ADDR, this->reg_map->toff_upper, this->reg_map->toff_upper.bits.toff_upper, toff_msb); 00950 SET_BIT_FIELD(TOFFLSB_ADDR, this->reg_map->toff_lower, this->reg_map->toff_lower.bits.toff_lower, toff_lsb); 00951 00952 return 0; 00953 } 00954 00955 uint16_t MAX7032::get_toff() 00956 { 00957 uint8_t toff_lsb, toff_msb; 00958 uint16_t toff = 0; 00959 00960 if(read_register(TOFFLSB_ADDR, (uint8_t *) & (this->reg_map->toff_lower)) < 0) 00961 return -1; 00962 00963 if(read_register(TOFFMSB_ADDR, (uint8_t *) & (this->reg_map->toff_upper)) < 0) 00964 return -2; 00965 00966 toff = this->reg_map->toff_upper.bits.toff_upper; 00967 toff = toff << 8; 00968 toff |= this->reg_map->toff_lower.bits.toff_lower; 00969 00970 return toff; 00971 } 00972 00973 int MAX7032::set_tcpu(uint8_t tcpu) 00974 { 00975 SET_BIT_FIELD(TCPU_ADDR, this->reg_map->tcpu, this->reg_map->tcpu.bits.tcpu, tcpu); 00976 00977 return 0; 00978 } 00979 00980 uint8_t MAX7032::get_tcpu() 00981 { 00982 int ret; 00983 00984 ret = read_register(TCPU_ADDR, (uint8_t *) & (this->reg_map->tcpu)); 00985 if (ret < 0) 00986 return ret; 00987 00988 return this->reg_map->tcpu.bits.tcpu; 00989 } 00990 00991 int MAX7032::set_trf(uint16_t trf) 00992 { 00993 uint8_t trf_lsb, trf_msb; 00994 00995 if(trf > 65535) 00996 return -99; 00997 00998 trf_lsb = (uint8_t)(trf & 0x00FF); 00999 trf_msb = (uint8_t)((trf & 0xFF00) >> 8); 01000 01001 SET_BIT_FIELD(TRFMSB_ADDR, this->reg_map->trf_upper, this->reg_map->trf_upper.bits.trf_upper, trf_msb); 01002 SET_BIT_FIELD(TRFLSB_ADDR, this->reg_map->trf_lower, this->reg_map->trf_lower.bits.trf_lower, trf_lsb); 01003 01004 return 0; 01005 } 01006 01007 uint16_t MAX7032::get_trf() 01008 { 01009 uint8_t trf_lsb, trf_msb; 01010 uint16_t trf = 0; 01011 01012 if(read_register(TRFLSB_ADDR, (uint8_t *) & (this->reg_map->trf_lower)) < 0) 01013 return -1; 01014 01015 if(read_register(TRFMSB_ADDR, (uint8_t *) & (this->reg_map->trf_upper)) < 0) 01016 return -2; 01017 01018 trf = this->reg_map->trf_upper.bits.trf_upper; 01019 trf = trf << 8; 01020 trf |= this->reg_map->trf_lower.bits.trf_lower; 01021 01022 return trf; 01023 } 01024 01025 int MAX7032::set_ton(uint16_t ton) 01026 { 01027 uint8_t ton_lsb, ton_msb; 01028 01029 if(ton > 65535) 01030 return -99; 01031 01032 ton_lsb = (uint8_t)(ton & 0x00FF); 01033 ton_msb = (uint8_t)((ton & 0xFF00) >> 8); 01034 01035 SET_BIT_FIELD(TONMSB_ADDR, this->reg_map->ton_upper, this->reg_map->ton_upper.bits.ton_upper, ton_msb); 01036 SET_BIT_FIELD(TONLSB_ADDR, this->reg_map->ton_lower, this->reg_map->ton_lower.bits.ton_lower, ton_lsb); 01037 01038 return 0; 01039 } 01040 01041 uint16_t MAX7032::get_ton() 01042 { 01043 uint8_t ton_lsb, ton_msb; 01044 uint16_t ton = 0; 01045 01046 if(read_register(TONLSB_ADDR, (uint8_t *) & (this->reg_map->ton_lower)) < 0) 01047 return -1; 01048 01049 if(read_register(TONMSB_ADDR, (uint8_t *) & (this->reg_map->ton_upper)) < 0) 01050 return -2; 01051 01052 ton = this->reg_map->ton_upper.bits.ton_upper; 01053 ton = ton << 8; 01054 ton |= this->reg_map->ton_lower.bits.ton_lower; 01055 01056 return ton; 01057 } 01058 01059 int MAX7032::set_txlow(int txlow_val) 01060 { 01061 uint8_t tx_low_lsb, tx_low_msb; 01062 01063 if(txlow_val > 65535) 01064 return -99; 01065 01066 tx_low_lsb = (uint8_t)(txlow_val & 0x00FF); 01067 tx_low_msb = (uint8_t)((txlow_val & 0xFF00) >> 8); 01068 01069 01070 SET_BIT_FIELD(TXLOWLSB_ADDR, this->reg_map->txlow_lower, this->reg_map->txlow_lower.bits.txlow_lower, tx_low_lsb); 01071 SET_BIT_FIELD(TXLOWMSB_ADDR, this->reg_map->txlow_upper, this->reg_map->txlow_upper.bits.txlow_upper, tx_low_msb); 01072 01073 return 0; 01074 } 01075 01076 int MAX7032::get_txlow() 01077 { 01078 uint8_t tx_low_lsb, tx_low_msb; 01079 uint32_t tx_low = 0; 01080 01081 if(read_register(TXLOWLSB_ADDR, (uint8_t *)&tx_low_lsb) < 0) 01082 return -1; 01083 01084 if(read_register(TXLOWMSB_ADDR, (uint8_t *)&tx_low_msb) < 0) 01085 return -2; 01086 01087 tx_low = tx_low_msb; 01088 tx_low = tx_low << 8; 01089 tx_low |= tx_low_lsb; 01090 01091 return tx_low; 01092 } 01093 01094 int MAX7032::set_txhigh(int txhigh_val) 01095 { 01096 uint8_t tx_high_lsb, tx_high_msb; 01097 01098 if(txhigh_val > 65535) 01099 return -99; 01100 01101 tx_high_lsb = (uint8_t)(txhigh_val & 0x00FF); 01102 tx_high_msb = (uint8_t)((txhigh_val & 0xFF00) >> 8); 01103 01104 SET_BIT_FIELD(TXHIGHLSB_ADDR, this->reg_map->txhigh_lower, this->reg_map->txhigh_lower.bits.txhigh_lower, tx_high_lsb); 01105 SET_BIT_FIELD(TXHIGHMSB_ADDR, this->reg_map->txhigh_upper, this->reg_map->txhigh_upper.bits.txhigh_upper, tx_high_msb); 01106 01107 return 0; 01108 } 01109 01110 int MAX7032::get_txhigh() 01111 { 01112 uint8_t tx_high_lsb, tx_high_msb; 01113 uint32_t tx_high = 0; 01114 01115 if(read_register(TXHIGHLSB_ADDR, (uint8_t *)&tx_high_lsb) < 0) 01116 return -1; 01117 01118 if(read_register(TXHIGHMSB_ADDR, (uint8_t *)&tx_high_msb) < 0) 01119 return -2; 01120 01121 tx_high = tx_high_msb; 01122 tx_high = tx_high << 8; 01123 tx_high |= tx_high_lsb; 01124 01125 return tx_high; 01126 } 01127 01128 int MAX7032::get_lckd() 01129 { 01130 int ret; 01131 01132 ret = read_register(STATUS_ADDR, (uint8_t *) & (this->reg_map->status)); 01133 if (ret < 0) 01134 return ret; 01135 01136 return this->reg_map->status.bits.lckd; 01137 } 01138 01139 int MAX7032::get_gains() 01140 { 01141 int ret; 01142 01143 ret = read_register(STATUS_ADDR, (uint8_t *) & (this->reg_map->status)); 01144 if (ret < 0) 01145 return ret; 01146 01147 return this->reg_map->status.bits.gains; 01148 } 01149 01150 int MAX7032::get_clkon() 01151 { 01152 int ret; 01153 01154 ret = read_register(STATUS_ADDR, (uint8_t *) & (this->reg_map->status)); 01155 if (ret < 0) 01156 return ret; 01157 01158 return this->reg_map->status.bits.clkon; 01159 } 01160 01161 int MAX7032::get_pcald() 01162 { 01163 int ret; 01164 01165 ret = read_register(STATUS_ADDR, (uint8_t *) & (this->reg_map->status)); 01166 if (ret < 0) 01167 return ret; 01168 01169 return this->reg_map->status.bits.pcald; 01170 } 01171 01172 int MAX7032::get_fcald() 01173 { 01174 int ret; 01175 01176 ret = read_register(STATUS_ADDR, (uint8_t *) & (this->reg_map->status)); 01177 if (ret < 0) 01178 return ret; 01179 01180 return this->reg_map->status.bits.fcald; 01181 } 01182 01183 int MAX7032::adjust_clockout(cdiv_t cdiv) 01184 { 01185 if(cdiv == DISABLE) { 01186 SET_BIT_FIELD(CONTRL_ADDR, this->reg_map->contrl, this->reg_map->contrl.bits.ckout, 0); 01187 return 0; 01188 } 01189 01190 if(cdiv == F_XTAL) { 01191 SET_BIT_FIELD(CONF1_ADDR, this->reg_map->conf1, this->reg_map->conf1.bits.cdiv, 0); 01192 }else if(cdiv == F_XTAL_X0_5) { 01193 SET_BIT_FIELD(CONF1_ADDR, this->reg_map->conf1, this->reg_map->conf1.bits.cdiv, 1); 01194 }else if(cdiv == F_XTAL_X0_25) { 01195 SET_BIT_FIELD(CONF1_ADDR, this->reg_map->conf1, this->reg_map->conf1.bits.cdiv, 2); 01196 }else if(cdiv == F_XTAL_X0_125) { 01197 SET_BIT_FIELD(CONF1_ADDR, this->reg_map->conf1, this->reg_map->conf1.bits.cdiv, 3); 01198 }else{ 01199 return -1; 01200 } 01201 01202 SET_BIT_FIELD(CONTRL_ADDR, this->reg_map->contrl, this->reg_map->contrl.bits.ckout, 1); 01203 return 0; 01204 } 01205 01206 int MAX7032::get_clockout_conf(cdiv_t *cdiv) 01207 { 01208 int ret; 01209 01210 ret = read_register(CONTRL_ADDR, (uint8_t *) & (this->reg_map->contrl)); 01211 if (ret < 0) 01212 return ret; 01213 01214 if(this->reg_map->contrl.bits.ckout == 0){ 01215 *cdiv = DISABLE; 01216 return 0; 01217 } 01218 01219 ret = read_register(CONF1_ADDR, (uint8_t *) & (this->reg_map->conf1)); 01220 if (ret < 0) 01221 return ret; 01222 01223 if(this->reg_map->conf1.bits.cdiv == 0) 01224 *cdiv = F_XTAL; 01225 else if(this->reg_map->conf1.bits.cdiv == 1) 01226 *cdiv = F_XTAL_X0_5; 01227 else if(this->reg_map->conf1.bits.cdiv == 2) 01228 *cdiv = F_XTAL_X0_25; 01229 else if(this->reg_map->conf1.bits.cdiv == 3) 01230 *cdiv = F_XTAL_X0_125; 01231 else 01232 return -1; 01233 01234 return 0; 01235 } 01236 01237 int MAX7032::set_ofps(uint8_t ofps) 01238 { 01239 if(ofps > 3) 01240 return -1; 01241 01242 SET_BIT_FIELD(CONF0_ADDR, this->reg_map->conf0, this->reg_map->conf0.bits.ofps, ofps); 01243 01244 return 0; 01245 } 01246 01247 int MAX7032::get_ofps() 01248 { 01249 int ret; 01250 01251 ret = read_register(CONF0_ADDR, (uint8_t *) & (this->reg_map->conf0)); 01252 if (ret < 0) 01253 return ret; 01254 01255 return (int)this->reg_map->conf0.bits.ofps; 01256 } 01257 01258 int MAX7032::set_onps(uint8_t onps) 01259 { 01260 if(onps > 3) 01261 return -1; 01262 01263 SET_BIT_FIELD(CONF0_ADDR, this->reg_map->conf0, this->reg_map->conf0.bits.onps, onps); 01264 01265 return 0; 01266 } 01267 01268 int MAX7032::get_onps() 01269 { 01270 int ret; 01271 01272 ret = read_register(CONF0_ADDR, (uint8_t *) & (this->reg_map->conf0)); 01273 if (ret < 0) 01274 return ret; 01275 01276 return (int)this->reg_map->conf0.bits.onps; 01277 } 01278 01279 int MAX7032::adjust_osc_freq(float osc_freq) 01280 { 01281 uint8_t osc_reg_val; 01282 01283 if (osc_freq < 12.05 || osc_freq > 18.31) 01284 return -99; 01285 01286 osc_reg_val = (osc_freq*1000) / 100; 01287 01288 if(write_register(OSC_ADDR, &osc_reg_val, 1) >= 0) 01289 { 01290 f_xtal = osc_freq; 01291 return 0; 01292 } 01293 01294 return -1; 01295 } 01296 01297 float MAX7032::get_osc_freq() 01298 { 01299 return f_xtal; 01300 } 01301 01302 int MAX7032::set_center_freq(float center_freq) 01303 { 01304 uint8_t tx_low_msb, tx_low_lsb, tx_high_msb, tx_high_lsb; 01305 uint16_t center_freq_reg_val; 01306 int fsk_high, fsk_low, ask_fsk_sel; 01307 01308 if (center_freq < 300 || center_freq > 450) 01309 return -99; 01310 01311 ask_fsk_sel = this->get_mode(); 01312 01313 if(ask_fsk_sel < 0){ 01314 return -98; 01315 } 01316 01317 if(ask_fsk_sel == ASK_FSK_SEL_ASK){ 01318 center_freq_reg_val = (uint16_t)round(((center_freq / f_xtal) - 16) * 4096); 01319 01320 tx_low_lsb = (uint8_t)(center_freq_reg_val & 0x00FF); 01321 tx_low_msb = (uint8_t)((center_freq_reg_val & 0xFF00) >> 8); 01322 01323 if(write_register(TXLOWLSB_ADDR, &tx_low_lsb, 1) < 0) 01324 return -1; 01325 if(write_register(TXLOWMSB_ADDR, &tx_low_msb, 1) < 0) 01326 return -2; 01327 } 01328 else{ 01329 fsk_high = round(((center_freq + fsk_dev) / f_xtal - 16) * 4096); 01330 fsk_low = round(((center_freq - fsk_dev) / f_xtal - 16) * 4096); 01331 01332 tx_low_lsb = ((uint)(fsk_low) & 0X00FF); 01333 tx_low_msb = ((uint)(fsk_low) & 0XFF00) >> 8; 01334 tx_high_lsb = ((uint)(fsk_high) & 0X00FF); 01335 tx_high_msb = ((uint)(fsk_high) & 0XFF00) >> 8; 01336 01337 if(write_register(TXLOWLSB_ADDR, &tx_low_lsb, 1) < 0) 01338 return -3; 01339 if(write_register(TXLOWMSB_ADDR, &tx_low_msb, 1) < 0) 01340 return -4; 01341 if(write_register(TXHIGHLSB_ADDR, &tx_high_lsb, 1) < 0) 01342 return -5; 01343 if(write_register(TXHIGHMSB_ADDR, &tx_high_msb, 1) < 0) 01344 return -6; 01345 } 01346 01347 f_rf = center_freq; 01348 01349 return 0; 01350 } 01351 01352 float MAX7032::get_center_freq() 01353 { 01354 return f_rf; 01355 } 01356 01357 int MAX7032::set_data_rate(float data_rate_set) 01358 { 01359 if(encoding == Manchester){ 01360 if(data_rate_set < 0 || data_rate_set > 33) 01361 return -99; 01362 } 01363 else{ 01364 if(data_rate_set < 0 || data_rate_set > 66) 01365 return -99; 01366 } 01367 01368 this->data_rate = data_rate_set; 01369 return 0; 01370 } 01371 01372 float MAX7032::get_data_rate() 01373 { 01374 return this->data_rate; 01375 } 01376 01377 int MAX7032::set_fsk_dev(float fsk_dev_set) 01378 { 01379 if(fsk_dev_set <= 0 || fsk_dev_set > fsk_dev_max) 01380 return -99; 01381 01382 this->fsk_dev = fsk_dev_set; 01383 01384 return set_center_freq(f_rf); 01385 } 01386 01387 float MAX7032::get_fsk_dev() 01388 { 01389 return this->fsk_dev; 01390 } 01391 01392 int MAX7032::set_encoding(encoding_t encoding_set) 01393 { 01394 this->encoding = encoding_set; 01395 return 0; 01396 } 01397 01398 int MAX7032::get_encoding() 01399 { 01400 return this->encoding; 01401 } 01402 01403 int MAX7032::adjust_agc_dwell_timer(uint8_t k_val) 01404 { 01405 if(k_val < 9 || k_val > 23) 01406 return -99; 01407 01408 if(!(k_val & 0x01)) 01409 return -98; 01410 01411 k_val = (k_val - 9) / 2; 01412 01413 SET_BIT_FIELD(CONF1_ADDR, this->reg_map->conf1, this->reg_map->conf1.bits.dt, k_val); 01414 return 0; 01415 } 01416 01417 int MAX7032::adjust_agc_dwell_timer(int dwell_time) 01418 { 01419 float k_val; 01420 uint8_t k_val_byte; 01421 01422 if(dwell_time <= 0) 01423 return -99; 01424 01425 k_val = log10(dwell_time * f_xtal) * 3.3; 01426 k_val_byte = (uint8_t)ceil(k_val); 01427 01428 if(!(k_val_byte & 0x01)) 01429 k_val_byte++; 01430 01431 if(k_val_byte < 9) 01432 k_val_byte = 9; 01433 01434 if(k_val_byte > 23) 01435 return -1; 01436 01437 k_val_byte = (k_val_byte - 9) / 2; 01438 01439 SET_BIT_FIELD(CONF1_ADDR, this->reg_map->conf1, this->reg_map->conf1.bits.dt, k_val_byte); 01440 return 0; 01441 } 01442 01443 int MAX7032::get_agc_dwell_timer() 01444 { 01445 int ret; 01446 uint8_t k_val; 01447 01448 ret = read_register(CONF1_ADDR, (uint8_t *) & (this->reg_map->conf1)); 01449 if (ret < 0) 01450 return ret; 01451 01452 k_val = (2 * this->reg_map->conf1.bits.dt) + 9; 01453 01454 return (int)(pow(2, k_val) / f_xtal); 01455 } 01456 01457 int MAX7032::adjust_off_timer(timer_base_t time_base, int timer_val) 01458 { 01459 uint8_t toff_msb, toff_lsb; 01460 01461 if(timer_val < 1 || timer_val > 65535) 01462 return -99; 01463 01464 toff_msb = (uint8_t)((timer_val & 0xFF00) >> 8); 01465 toff_lsb = (uint8_t)(timer_val & 0x00FF); 01466 01467 if(write_register(TOFFMSB_ADDR, &toff_msb, 1) < 0) 01468 return -1; 01469 if(write_register(TOFFLSB_ADDR, &toff_lsb, 1) < 0) 01470 return -2; 01471 01472 SET_BIT_FIELD(CONF0_ADDR, this->reg_map->conf0, this->reg_map->conf0.bits.ofps, time_base); 01473 01474 return 0; 01475 } 01476 01477 int MAX7032::adjust_off_timer(int toff_time) 01478 { 01479 int time_base, ton_reg_val; 01480 timer_base_t timer_base_param; 01481 01482 if(toff_time < 7860000){ //7.86s 01483 time_base = 120; 01484 timer_base_param = US_120; 01485 }else if(toff_time < 31460000){ //31.46s 01486 time_base = 480; 01487 timer_base_param = US_480; 01488 }else if(toff_time < 126000000){ //2min 6s 01489 time_base = 1920; 01490 timer_base_param = US_1920; 01491 }else if(toff_time < 503000000){ //8min 23s 01492 time_base = 7680; 01493 timer_base_param = US_7680; 01494 }else{ 01495 return -99; 01496 } 01497 01498 ton_reg_val = toff_time / time_base; 01499 01500 if((ton_reg_val * time_base) < toff_time) 01501 ton_reg_val++; 01502 01503 adjust_off_timer(timer_base_param, ton_reg_val); 01504 01505 return 0; 01506 } 01507 01508 int MAX7032::get_off_timer() 01509 { 01510 uint8_t toff_msb, toff_lsb; 01511 int timer_val, time_base, ret; 01512 01513 time_base = this->get_ofps(); 01514 01515 if(time_base < 0) 01516 return -1; 01517 01518 ret = read_register(TOFFMSB_ADDR, (uint8_t *)&toff_msb); 01519 if (ret < 0) 01520 return -2; 01521 01522 ret = read_register(TOFFLSB_ADDR, (uint8_t *)&toff_lsb); 01523 if (ret < 0) 01524 return -3; 01525 01526 timer_val = toff_msb; 01527 timer_val = timer_val << 8; 01528 timer_val += toff_lsb; 01529 01530 if(time_base == 0) 01531 return timer_val * 120; 01532 if(time_base == 1) 01533 return timer_val * 480; 01534 if(time_base == 2) 01535 return timer_val * 1920; 01536 if(time_base == 3) 01537 return timer_val * 7680; 01538 01539 return -4; 01540 } 01541 01542 int MAX7032::adjust_on_timer(timer_base_t time_base, int timer_val) 01543 { 01544 uint8_t ton_msb, ton_lsb; 01545 01546 if(timer_val < 1 || timer_val > 65535) 01547 return -99; 01548 01549 ton_msb = (uint8_t)((timer_val & 0xFF00) >> 8); 01550 ton_lsb = (uint8_t)(timer_val & 0x00FF); 01551 01552 if(write_register(TONMSB_ADDR, &ton_msb, 1) < 0) 01553 return -1; 01554 if(write_register(TONLSB_ADDR, &ton_lsb, 1) < 0) 01555 return -2; 01556 01557 SET_BIT_FIELD(CONF0_ADDR, this->reg_map->conf0, this->reg_map->conf0.bits.onps, time_base); 01558 01559 return 0; 01560 } 01561 01562 int MAX7032::adjust_on_timer(int ton_time) 01563 { 01564 int time_base, ton_reg_val; 01565 timer_base_t timer_base_param; 01566 01567 if(ton_time < 7860000){ //7.86s 01568 time_base = 120; 01569 timer_base_param = US_120; 01570 }else if(ton_time < 31460000){ //31.46s 01571 time_base = 480; 01572 timer_base_param = US_480; 01573 }else if(ton_time < 126000000){ //2min 6s 01574 time_base = 1920; 01575 timer_base_param = US_1920; 01576 }else if(ton_time < 503000000){ //8min 23s 01577 time_base = 7680; 01578 timer_base_param = US_7680; 01579 }else{ 01580 return -99; 01581 } 01582 01583 ton_reg_val = ton_time / time_base; 01584 01585 if((ton_reg_val * time_base) < ton_time) 01586 ton_reg_val++; 01587 01588 adjust_on_timer(timer_base_param, ton_reg_val); 01589 01590 return 0; 01591 } 01592 01593 int MAX7032::get_on_timer() 01594 { 01595 uint8_t ton_msb, ton_lsb; 01596 int timer_val, time_base, ret; 01597 01598 time_base = this->get_onps(); 01599 01600 if(time_base < 0) 01601 return -1; 01602 01603 ret = read_register(TONMSB_ADDR, (uint8_t *)&ton_msb); 01604 if (ret < 0) 01605 return -2; 01606 01607 ret = read_register(TONLSB_ADDR, (uint8_t *)&ton_lsb); 01608 if (ret < 0) 01609 return -3; 01610 01611 timer_val = ton_msb; 01612 timer_val = timer_val << 8; 01613 timer_val += ton_lsb; 01614 01615 if(time_base == 0) 01616 return timer_val * 120; 01617 if(time_base == 1) 01618 return timer_val * 480; 01619 if(time_base == 2) 01620 return timer_val * 1920; 01621 if(time_base == 3) 01622 return timer_val * 7680; 01623 01624 return -4; 01625 } 01626 01627 int MAX7032::adjust_cpu_recovery_timer(int tcpu_time) 01628 { 01629 uint8_t tcpu; 01630 01631 if(tcpu_time > 30600) 01632 return -99; 01633 01634 tcpu = tcpu_time / 120; 01635 01636 if((tcpu * 120) < tcpu_time) 01637 tcpu++; 01638 01639 SET_BIT_FIELD(TCPU_ADDR, this->reg_map->tcpu, this->reg_map->tcpu.bits.tcpu, tcpu); 01640 01641 return 0; 01642 } 01643 01644 int MAX7032::get_cpu_recovery_timer() 01645 { 01646 uint8_t tcpu; 01647 int ret; 01648 01649 ret = read_register(TCPU_ADDR, (uint8_t *)&tcpu); 01650 if (ret < 0) 01651 return -1; 01652 01653 return tcpu * 120; 01654 } 01655 01656 int MAX7032::adjust_rf_settling_timer(int trf_time) 01657 { 01658 uint8_t trf_msb, trf_lsb; 01659 uint16_t timer_val; 01660 01661 if(trf_time > 7864200) 01662 return -99; 01663 01664 timer_val = trf_time / 120; 01665 01666 if((timer_val*120) < trf_time) 01667 timer_val++; 01668 01669 trf_msb = (uint8_t)((timer_val & 0xFF00) >> 8); 01670 trf_lsb = (uint8_t)(timer_val & 0x00FF); 01671 01672 SET_BIT_FIELD(TRFMSB_ADDR, this->reg_map->trf_upper, this->reg_map->trf_upper.bits.trf_upper, trf_msb); 01673 SET_BIT_FIELD(TRFLSB_ADDR, this->reg_map->trf_lower, this->reg_map->trf_lower.bits.trf_lower, trf_lsb); 01674 01675 return 0; 01676 } 01677 01678 int MAX7032::get_rf_settling_timer() 01679 { 01680 uint8_t trf_msb, trf_lsb; 01681 int timer_val, ret; 01682 01683 ret = read_register(TRFMSB_ADDR, (uint8_t *)&trf_msb); 01684 if (ret < 0) 01685 return -1; 01686 01687 ret = read_register(TRFLSB_ADDR, (uint8_t *)&trf_lsb); 01688 if (ret < 0) 01689 return -2; 01690 01691 timer_val = trf_msb; 01692 timer_val = timer_val << 8; 01693 timer_val += trf_lsb; 01694 01695 return timer_val * 120; 01696 } 01697 01698 int MAX7032::set_trx_state(trx_state_t trx_state) 01699 { 01700 if(trx_state == RECEIVE_MODE){ 01701 if(data_send != NULL){ 01702 delete this->data_send; 01703 this->data_send = NULL; 01704 } 01705 01706 if(data_read == NULL){ 01707 this->data_read = new DigitalIn(data_pin); 01708 this->data_read->mode(OpenDrain); 01709 } 01710 01711 if( set_t_r(RECEIVE_MODE) < 0) 01712 return -1; 01713 01714 if(trx_pin == NULL) //trx state depends on how the trx pin is connected 01715 return -2; 01716 01717 trx_pin->write(RECEIVE_MODE); 01718 01719 return 0; 01720 01721 }else{ 01722 if(data_read != NULL){ 01723 delete this->data_read; 01724 this->data_read = NULL; 01725 } 01726 01727 if(data_send == NULL){ 01728 this->data_send = new DigitalOut(MAX7032_MBED_DATA_PIN); 01729 *data_send = 0; 01730 } 01731 01732 return set_t_r(TRANSMIT_MODE); 01733 } 01734 } 01735 01736 int MAX7032::get_trx_state() 01737 { 01738 if(get_t_r()) 01739 return TRANSMIT_MODE; 01740 01741 if(trx_pin == NULL) //trx state depends on how the trx pin is connected 01742 return -1; 01743 01744 return trx_pin->read(); 01745 } 01746 01747 int MAX7032::rf_transmit_data(uint8_t *data, uint8_t data_len) 01748 { 01749 uint8_t *coded_data; 01750 uint8_t byte_idx, bit_idx; 01751 uint32_t bit_duration, coded_data_len, coded_data_idx, transmit_time = 0, curr_time; 01752 Timer timer; 01753 01754 if(data == NULL) 01755 return -99; 01756 01757 if(get_trx_state() == RECEIVE_MODE) 01758 return -98; 01759 01760 if(encoding == Manchester){ 01761 coded_data = new uint8_t[data_len * 2]; 01762 01763 if(coded_data == NULL) 01764 return -97; 01765 01766 for(byte_idx = 0; byte_idx < data_len; byte_idx++) 01767 { 01768 for(bit_idx = 0; bit_idx < 8; bit_idx++) 01769 { 01770 if(data[byte_idx] & (0x80 >> bit_idx)){ 01771 coded_data[byte_idx * 2 + (bit_idx / 4)] |= (0x80>>((bit_idx % 4) * 2)); 01772 coded_data[byte_idx * 2 + (bit_idx / 4)] &= ~(0x80>>((bit_idx % 4) * 2 + 1)); 01773 } else{ 01774 coded_data[byte_idx * 2 + (bit_idx / 4)] &= ~(0x80>>((bit_idx % 4) * 2)); 01775 coded_data[byte_idx * 2 + (bit_idx / 4)] |= (0x80>>((bit_idx % 4) * 2 + 1)); 01776 } 01777 } 01778 } 01779 01780 bit_duration = 1000 / (data_rate*2); //us 01781 coded_data_len = data_len * 2; 01782 } 01783 else if(encoding == NRZ){ 01784 coded_data = new uint8_t[data_len]; 01785 01786 if(coded_data == NULL) 01787 return -96; 01788 01789 for(byte_idx = 0; byte_idx < data_len; byte_idx++) 01790 { 01791 coded_data[byte_idx] = data[byte_idx]; 01792 } 01793 01794 bit_duration = 1000 / data_rate; //us 01795 coded_data_len = data_len; 01796 } 01797 01798 timer.start(); 01799 01800 core_util_critical_section_enter(); 01801 01802 for(coded_data_idx = 0; coded_data_idx < coded_data_len; coded_data_idx++) 01803 { 01804 for(bit_idx = 0; bit_idx < 8; bit_idx++) 01805 { 01806 while(1) 01807 { 01808 curr_time = timer.read_us(); 01809 if((curr_time - transmit_time) >= bit_duration){ 01810 transmit_time = curr_time; 01811 01812 if(coded_data[coded_data_idx] & (0x80 >> bit_idx)) 01813 *data_send = 1; 01814 else 01815 *data_send = 0; 01816 break; 01817 } 01818 } 01819 } 01820 } 01821 01822 while(1) 01823 { 01824 curr_time = timer.read_us(); 01825 if((curr_time - transmit_time) >= bit_duration){ 01826 *data_send = 0; 01827 break; 01828 } 01829 } 01830 01831 core_util_critical_section_exit(); 01832 01833 delete coded_data; 01834 01835 return 0; 01836 } 01837 01838 int MAX7032::rf_receive_data(uint8_t *coded_data, uint8_t coded_data_len) 01839 { 01840 uint8_t byte_idx, bit_idx; 01841 uint32_t bit_duration, coded_data_idx, read_time = 0, curr_time; 01842 Timer timer; 01843 01844 if(coded_data == NULL) 01845 return -99; 01846 01847 if(get_trx_state() == TRANSMIT_MODE) 01848 return -98; 01849 01850 if(encoding == Manchester){ 01851 bit_duration = 1000 / (data_rate*4); //us 01852 }else{ 01853 bit_duration = 1000 / (data_rate*2); //us 01854 } 01855 01856 core_util_critical_section_enter(); 01857 01858 timer.start(); 01859 01860 for(coded_data_idx = 0; coded_data_idx < coded_data_len; coded_data_idx++) 01861 { 01862 for(bit_idx = 0; bit_idx < 8; bit_idx++) 01863 { 01864 while(1) 01865 { 01866 curr_time = timer.read_us(); 01867 if((curr_time - read_time) >= bit_duration){ 01868 if(data_read->read()) 01869 coded_data[coded_data_idx] |= (0x80 >> bit_idx); 01870 else 01871 coded_data[coded_data_idx] &= ~(0x80 >> bit_idx); 01872 01873 read_time = curr_time; 01874 break; 01875 } 01876 } 01877 } 01878 core_util_critical_section_exit(); 01879 } 01880 01881 return 0; 01882 } 01883 01884 int MAX7032::set_trx_pin(trx_state_t pin_state) 01885 { 01886 if(trx_pin == NULL) 01887 return -1; 01888 01889 trx_pin->write(pin_state); 01890 return 0; 01891 }
Generated on Thu Jul 14 2022 04:50:06 by
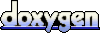