
Simple demo of BMI160 Library
Dependencies: BMI160 max32630fthr mbed
Fork of MAX32630FTHR_IMU_Test by
main.cpp
00001 /********************************************************************** 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 **********************************************************************/ 00032 00033 00034 #include "mbed.h" 00035 //#include "max32630fthr.h" 00036 #include "bmi160.h" 00037 00038 00039 void dumpImuRegisters(BMI160 &imu); 00040 void printRegister(BMI160 &imu, BMI160::Registers reg); 00041 void printBlock(BMI160 &imu, BMI160::Registers startReg, BMI160::Registers stopReg); 00042 00043 00044 int main() 00045 { 00046 //MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00047 00048 DigitalOut rLED(LED1, LED_OFF); 00049 DigitalOut gLED(LED2, LED_OFF); 00050 DigitalOut bLED(LED3, LED_OFF); 00051 00052 I2C i2cBus(P5_7, P6_0); 00053 i2cBus.frequency(400000); 00054 BMI160_I2C imu(i2cBus, BMI160_I2C::I2C_ADRS_SDO_LO); 00055 00056 printf("\033[H"); //home 00057 printf("\033[0J"); //erase from cursor to end of screen 00058 00059 uint32_t failures = 0; 00060 00061 if(imu.setSensorPowerMode(BMI160::GYRO, BMI160::NORMAL) != BMI160::RTN_NO_ERROR) 00062 { 00063 printf("Failed to set gyroscope power mode\n"); 00064 failures++; 00065 } 00066 wait_ms(100); 00067 00068 if(imu.setSensorPowerMode(BMI160::ACC, BMI160::NORMAL) != BMI160::RTN_NO_ERROR) 00069 { 00070 printf("Failed to set accelerometer power mode\n"); 00071 failures++; 00072 } 00073 wait_ms(100); 00074 00075 00076 BMI160::AccConfig accConfig; 00077 //example of using getSensorConfig 00078 if(imu.getSensorConfig(accConfig) == BMI160::RTN_NO_ERROR) 00079 { 00080 printf("ACC Range = %d\n", accConfig.range); 00081 printf("ACC UnderSampling = %d\n", accConfig.us); 00082 printf("ACC BandWidthParam = %d\n", accConfig.bwp); 00083 printf("ACC OutputDataRate = %d\n\n", accConfig.odr); 00084 } 00085 else 00086 { 00087 printf("Failed to get accelerometer configuration\n"); 00088 failures++; 00089 } 00090 00091 //example of setting user defined configuration 00092 accConfig.range = BMI160::SENS_4G; 00093 accConfig.us = BMI160::ACC_US_OFF; 00094 accConfig.bwp = BMI160::ACC_BWP_2; 00095 accConfig.odr = BMI160::ACC_ODR_8; 00096 if(imu.setSensorConfig(accConfig) == BMI160::RTN_NO_ERROR) 00097 { 00098 printf("ACC Range = %d\n", accConfig.range); 00099 printf("ACC UnderSampling = %d\n", accConfig.us); 00100 printf("ACC BandWidthParam = %d\n", accConfig.bwp); 00101 printf("ACC OutputDataRate = %d\n\n", accConfig.odr); 00102 } 00103 else 00104 { 00105 printf("Failed to set accelerometer configuration\n"); 00106 failures++; 00107 } 00108 00109 BMI160::GyroConfig gyroConfig; 00110 if(imu.getSensorConfig(gyroConfig) == BMI160::RTN_NO_ERROR) 00111 { 00112 printf("GYRO Range = %d\n", gyroConfig.range); 00113 printf("GYRO BandWidthParam = %d\n", gyroConfig.bwp); 00114 printf("GYRO OutputDataRate = %d\n\n", gyroConfig.odr); 00115 } 00116 else 00117 { 00118 printf("Failed to get gyroscope configuration\n"); 00119 failures++; 00120 } 00121 00122 wait(1.0); 00123 printf("\033[H"); //home 00124 printf("\033[0J"); //erase from cursor to end of screen 00125 00126 if(failures == 0) 00127 { 00128 float imuTemperature; 00129 BMI160::SensorData accData; 00130 BMI160::SensorData gyroData; 00131 BMI160::SensorTime sensorTime; 00132 00133 while(1) 00134 { 00135 imu.getGyroAccXYZandSensorTime(accData, gyroData, sensorTime, accConfig.range, gyroConfig.range); 00136 imu.getTemperature(&imuTemperature); 00137 00138 printf("ACC xAxis = %s%4.3f\n", "\033[K", accData.xAxis.scaled); 00139 printf("ACC yAxis = %s%4.3f\n", "\033[K", accData.yAxis.scaled); 00140 printf("ACC zAxis = %s%4.3f\n\n", "\033[K", accData.zAxis.scaled); 00141 00142 printf("GYRO xAxis = %s%5.1f\n", "\033[K", gyroData.xAxis.scaled); 00143 printf("GYRO yAxis = %s%5.1f\n", "\033[K", gyroData.yAxis.scaled); 00144 printf("GYRO zAxis = %s%5.1f\n\n", "\033[K", gyroData.zAxis.scaled); 00145 00146 printf("Sensor Time = %s%f\n", "\033[K", sensorTime.seconds); 00147 printf("Sensor Temperature = %s%5.3f\n", "\033[K", imuTemperature); 00148 00149 printf("\033[H"); //home 00150 gLED = !gLED; 00151 } 00152 } 00153 else 00154 { 00155 while(1) 00156 { 00157 rLED = !rLED; 00158 wait(0.25); 00159 } 00160 } 00161 } 00162 00163 00164 //***************************************************************************** 00165 void dumpImuRegisters(BMI160 &imu) 00166 { 00167 printRegister(imu, BMI160::CHIP_ID); 00168 printBlock(imu, BMI160::ERR_REG,BMI160::FIFO_DATA); 00169 printBlock(imu, BMI160::ACC_CONF, BMI160::FIFO_CONFIG_1); 00170 printBlock(imu, BMI160::MAG_IF_0, BMI160::SELF_TEST); 00171 printBlock(imu, BMI160::NV_CONF, BMI160::STEP_CONF_1); 00172 printRegister(imu, BMI160::CMD); 00173 printf("\n"); 00174 } 00175 00176 00177 //***************************************************************************** 00178 void printRegister(BMI160 &imu, BMI160::Registers reg) 00179 { 00180 uint8_t data; 00181 if(imu.readRegister(reg, &data) == BMI160::RTN_NO_ERROR) 00182 { 00183 printf("IMU Register 0x%02x = 0x%02x\n", reg, data); 00184 } 00185 else 00186 { 00187 printf("Failed to read register\n"); 00188 } 00189 } 00190 00191 00192 //***************************************************************************** 00193 void printBlock(BMI160 &imu, BMI160::Registers startReg, BMI160::Registers stopReg) 00194 { 00195 uint8_t numBytes = ((stopReg - startReg) + 1); 00196 uint8_t buff[numBytes]; 00197 uint8_t offset = static_cast<uint8_t>(startReg); 00198 00199 if(imu.readBlock(startReg, stopReg, buff) == BMI160::RTN_NO_ERROR) 00200 { 00201 for(uint8_t idx = offset; idx < (numBytes + offset); idx++) 00202 { 00203 printf("IMU Register 0x%02x = 0x%02x\n", idx, buff[idx - offset]); 00204 } 00205 } 00206 else 00207 { 00208 printf("Failed to read block\n"); 00209 } 00210 }
Generated on Thu Jul 14 2022 02:46:11 by
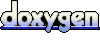