
ME11B Sample Code in Maxim Integrated Team
Dependencies: BMI160 max32630hsp3 MemoryLCD USBDevice
Fork of Host_Software_MAX32664GWEB_HR_EXTENDED by
cmdInterface.cpp
00001 /* 00002 * cmdInterface.cpp 00003 * 00004 * Created on: Jan 30, 2019 00005 * Author: Yagmur.Gok 00006 */ 00007 00008 #include <mbed.h> 00009 #include "cmdInterface.h" 00010 #include "SH_Max8614x_BareMetal.h" 00011 #include "bootldrAPI.h" 00012 #include "SHComm.h" 00013 #include "demoDefinitions.h" 00014 00015 static uint8_t hostOperatingMode = HOSTMODEAPPLICATION; 00016 static uint8_t hostCommEchoMode = HOSTCOMMMODE_ECHO_OFF; 00017 00018 /* @brief function to get the current operating mode of HOST: "APPMODE" or "BOOTLOADERMODE" 00019 * associated with command: "get_host_opmode". 00020 * 00021 * @param[in] arg : NULL string, just to match the form of command table function pointer type 00022 * 00023 * */ 00024 static int get_host_operating_mode(const char* arg){ 00025 00026 int status = 0x00; 00027 SERIALOUT("\r\n%s host_operating_mode=%s\r\n", "get_host_mode", (hostOperatingMode ==HOSTMODEAPPLICATION)? "APPMODE":"BOOTLOADERMODE" ); 00028 return status; 00029 } 00030 00031 /* @brief function to set the current operating mode of HOST: "APPMODE" or "BOOTLOADERMODE" 00032 * associated with command: "set_host_opmode". 00033 * 00034 * @param[in] arg: whole command string with argument included: "set_host_opmode X", X: 1 or 0 00035 * 0 : APPMODE 00036 * 1 : BOOTLOADERMODE 00037 * 00038 * */ 00039 static int set_host_operating_mode(const char* arg){ 00040 00041 int status = -1; 00042 uint32_t val; 00043 if( sscanf(arg, "%*s %x", &val) == 1 ){ 00044 hostOperatingMode = ( val > 0 )? HOSTMODEBOOTLOADER:HOSTMODEAPPLICATION; 00045 00046 status = 0x00; 00047 } 00048 SERIALOUT("\r\n%s err=%d\r\n", "set_host_opmode", status); 00049 return status; 00050 00051 } 00052 00053 00054 /* @brief function to get the current firmware version of Sensor Hub". 00055 * 00056 * @param[in] arg : NULL string, just to match the form of command table function pointer type 00057 * 00058 * */ 00059 static int get_hub_firmware_version(const char* arg){ 00060 00061 int idx; 00062 int status = -1; 00063 static const int MAXFWDESCSIZE = 5; 00064 uint8_t descArray[MAXFWDESCSIZE]; 00065 uint8_t descSz; 00066 00067 status = sh_get_ss_fw_version( &descArray[0] , &descSz); 00068 if(status == 0x00 && descSz > 0 && descSz < MAXFWDESCSIZE){ 00069 SERIALOUT("\r\n Firmware Version of Sensor Hub is = "); 00070 for(idx = 0 ; idx != descSz ; idx++) 00071 SERIALOUT("%d.", descArray[idx]); 00072 SERIALOUT("\r\n"); 00073 } 00074 00075 00076 } 00077 00078 static int set_host_comm_echomode(const char* arg){ 00079 00080 int status = -1; 00081 uint32_t val; 00082 if( sscanf(arg, "%*s %x", &val) == 1 ){ 00083 hostCommEchoMode = ( val > 0 )? HOSTCOMMMODE_ECHO_ON:HOSTCOMMMODE_ECHO_OFF; 00084 00085 status = 0x00; 00086 } 00087 SERIALOUT("\r\n%s err=%d\r\n", "set_host_comm_echomode", status); 00088 return status; 00089 00090 } 00091 00092 /* 00093 static int get_hub_operating_mode(const char* arg){ 00094 00095 uint8_t hubMode; 00096 int status = sh_get_sensorhub_operating_mode(&hubMode); 00097 if( status == 0x00) 00098 SERIALOUT("\r\n hub_operating_mode=%s\r\n", (hubMode == 0x00)? "APPMODE":"BOOTLOADERMODE" ); 00099 else 00100 SERIALOUT("\r\n%s err=%d\r\n", "get_sensorhub_opmode", status); 00101 00102 return status; 00103 }*/ 00104 00105 //__attribute__((__always_inline__)) 00106 uint8_t get_internal_operating_mode(void) { 00107 00108 return hostOperatingMode; 00109 } 00110 00111 00112 /* HOST MCU related mode functions inoredr to keep application&bootloader side modular: 00113 * 00114 * 1. in app mode Host accepts commnands related to algo/ppg applications ( command table in SH_Max8614x_BareMetal.h) 00115 * and does not check/relpy to bootloder related commnads 00116 * 00117 * 2. in bootloader mode Host accepts commands related to bootloader ( command table in bootldrAPI.h) only. 00118 * 00119 * */ 00120 cmd_interface_t setCommEchoModeCMD = {"set_host_echomode" , set_host_comm_echomode , "enables/disables echoing of command console commands"}; 00121 cmd_interface_t setHostModeCMD = {"set_host_opmode" , set_host_operating_mode , "sets mode of host to app or bootloader"}; 00122 cmd_interface_t getHostModeCMD = {"get_host_opmode" , get_host_operating_mode , "gets mode of host app or bootloader"}; 00123 cmd_interface_t getHubFwVersionCMD = {"get_hub_fwversion" , get_hub_firmware_version , "gets mode of host app or bootloader"}; 00124 //cmd_interface_t getHubModeCMD = {"get_sensorhub_opmode", get_hub_operating_mode , "gets mode of host app or bootloader"}; 00125 00126 /* @brief Compares two string to check whether they match. 00127 * 00128 * @param[in] str1, str2 : strings to compare 00129 * 00130 * */ 00131 00132 static bool starts_with(const char* str1, const char* str2) 00133 { 00134 while (*str1 && *str2) { 00135 if (*str1 != *str2) 00136 return false; 00137 str1++; 00138 str2++; 00139 } 00140 00141 if (*str2) 00142 return false; 00143 00144 return true; 00145 } 00146 00147 00148 00149 /** 00150 * @brief Command parser and executer for user input commands 00151 * @details Gets the command string from command builder, compares with the commands of defined command tables of 8614x and bootloader 00152 * if found calls the function associated with the command, passsing the whole command string with arguments to the called 00153 * function 00154 * 00155 * @param[in] cmd_str Input commnad from user. 00156 */ 00157 00158 int parse_execute_command( const char *cmd_str) 00159 { 00160 00161 int found = 0; 00162 int tableIdx; 00163 00164 if( starts_with(&cmd_str[0], setCommEchoModeCMD.cmdStr)) { 00165 int status = setCommEchoModeCMD.execute(cmd_str); 00166 if( status != 0x00){ 00167 SERIALOUT("\r\n%s err=%d\r\n", "set_host_mode", COMM_INVALID_PARAM); 00168 hostCommEchoMode = HOSTCOMMMODE_ECHO_OFF; 00169 } 00170 found = 1; 00171 } 00172 00173 if( starts_with(&cmd_str[0], setHostModeCMD.cmdStr)) { 00174 int status = setHostModeCMD.execute(cmd_str); 00175 if( status != 0x00){ 00176 SERIALOUT("\r\n%s err=%d\r\n", "set_host_mode", COMM_INVALID_PARAM); 00177 hostOperatingMode = HOSTMODEAPPLICATION; 00178 } 00179 found = 1; 00180 } 00181 00182 if( starts_with(&cmd_str[0], getHostModeCMD.cmdStr)) { 00183 int status = getHostModeCMD.execute(cmd_str); 00184 found = 1; 00185 } 00186 00187 if( starts_with(&cmd_str[0], getHubFwVersionCMD.cmdStr)) { 00188 int status = getHubFwVersionCMD.execute(cmd_str); 00189 found = 1; 00190 } 00191 00192 /* if( starts_with(&cmd_str[0], getHubModeCMD.cmdStr)) { 00193 int status = getHubModeCMD.execute(cmd_str); 00194 found = 1; 00195 }*/ 00196 00197 if( hostOperatingMode == HOSTMODEAPPLICATION) { 00198 00199 tableIdx = NUMCMDS8614X; 00200 do{ 00201 tableIdx -= 1; 00202 if (starts_with(&cmd_str[0], CMDTABLE8614x[tableIdx].cmdStr)){ 00203 00204 CMDTABLE8614x[tableIdx].execute(cmd_str); 00205 /*MYG DEBUG8*/// SERIALPRINT("___SELECTED COMMAND IDX IS: %d \r\n", tableIdx); 00206 SERIALOUT(" \r\n"); // Here is needed due to a bug on mbed serial! 00207 found = 1; 00208 } 00209 00210 }while(tableIdx && found == 0 ); 00211 00212 } 00213 00214 if( hostOperatingMode == HOSTMODEBOOTLOADER) { 00215 00216 00217 tableIdx = NUMCMDSBOOTLDRAPI; 00218 do{ 00219 tableIdx -= 1; 00220 if (starts_with(&cmd_str[0], CMDTABLEBOOTLDR[tableIdx].cmdStr)){ 00221 00222 CMDTABLEBOOTLDR[tableIdx].execute(cmd_str); 00223 /*MYG DEBUG8*/// SERIALPRINT("___SELECTED COMMAND IDX IS: %d \r\n", tableIdx); 00224 SERIALOUT(" \r\n"); // Here is needed due to a bug on mbed serial! 00225 found = 1; 00226 } 00227 00228 }while(tableIdx && found == 0 ); 00229 } 00230 00231 return found; 00232 } 00233 00234 00235 /** 00236 * @brief Command builder from serial i/o device. 00237 * @details Reads character and builds commands for application. 00238 * 00239 * @param[in] ch Input character from i/o device. 00240 */ 00241 //__attribute__((__always_inline__)) 00242 void cmdIntf_build_command(char ch) 00243 { 00244 static char cmd_str[1024]; 00245 static int cmd_idx = 0; 00246 int status; 00247 00248 if(hostCommEchoMode == HOSTCOMMMODE_ECHO_ON) 00249 SERIALOUT("%c", ch); 00250 00251 if (ch == 0x00) { 00252 return; 00253 } 00254 00255 if ((ch == '\n') || (ch == '\r')) { 00256 if (cmd_idx < 1024) 00257 cmd_str[cmd_idx++] = '\0'; 00258 status = parse_execute_command(cmd_str); 00259 00260 //Clear cmd_str 00261 while (cmd_idx > 0) 00262 cmd_str[--cmd_idx] = '\0'; 00263 00264 } else if ((ch == 0x08 || ch == 0x7F) && cmd_idx > 0) { 00265 //Backspace character 00266 if (cmd_idx > 0) 00267 cmd_str[--cmd_idx] = '\0'; 00268 } else { 00269 00270 if (cmd_idx < 1024) 00271 cmd_str[cmd_idx++] = ch; 00272 } 00273 00274 } 00275 00276 00277
Generated on Fri Jul 15 2022 18:24:31 by
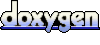