
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
BluetoothLE.h
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #ifndef _BLUETOOTHLE_H_ 00034 #define _BLUETOOTHLE_H_ 00035 00036 #include "mbed.h" 00037 #include "BLE.h" 00038 #include "Characteristic.h" 00039 00040 class BluetoothLE { 00041 public: 00042 BluetoothLE(BLE *ble, int numberOfCharacteristics); 00043 ~BluetoothLE(void); 00044 void addCharacteristic(Characteristic *characteristic); 00045 void initService(uint8_t *serialNumber, uint8_t *deviceName, int nameSize, uint8_t *serviceUUID); 00046 void notifyCharacteristic(int index, uint8_t *data); 00047 00048 00049 /** 00050 * @brief Return a characteristic based on incoming index 00051 * @param index index into an array of characteristics 00052 */ 00053 Characteristic *getCharacteristic(int index) { 00054 return characteristics[index]; 00055 } 00056 00057 /** 00058 * @brief Get the payload for this characteristic 00059 */ 00060 uint8_t *getCharacteristicPayload(int index) { 00061 return characteristics[index]->getPayloadPtr(); 00062 } 00063 typedef void (*PtrFunction)(int index); 00064 00065 /** 00066 * @brief Used to connect a connection callback 00067 */ 00068 template<typename T> 00069 void onConnect(T *object, void (T::*member)(void)) { 00070 _onConnect.attach( object, member ); 00071 } 00072 /** 00073 * @brief Used to connect a disconnection callback 00074 */ 00075 template<typename T> 00076 void onDisconnect(T *object, void (T::*member)(void)) { 00077 _onDisconnect.attach( object, member ); 00078 } 00079 /** 00080 * @brief Used to connect a characteristic written callback 00081 */ 00082 void onDataWritten(PtrFunction _onDataWritten) { 00083 this->_onDataWritten = _onDataWritten; 00084 } 00085 /** 00086 * @brief Get the connection state of the BLE 00087 * @return true if connection BLE connection is present, false if no connection 00088 */ 00089 bool isConnected(void); 00090 00091 /** 00092 * @brief Perform a notification test 00093 * @param index Index into an array of characteristic objects 00094 */ 00095 void notifyCharacteristicTest(int index); 00096 00097 /** 00098 * @brief Get the data that was written to the indexed characteristic 00099 * @return true if connection BLE connection is present, false if no connection 00100 */ 00101 uint8_t *getDataWritten(int index, int *length); 00102 00103 private: 00104 /** 00105 * @brief Disconnection callback 00106 */ 00107 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params); 00108 /** 00109 * @brief Connection callback 00110 */ 00111 void connectionCallback(const Gap::ConnectionCallbackParams_t *params); 00112 /** 00113 * @brief Characteristic written callback 00114 */ 00115 void onDataWritten(const GattWriteCallbackParams *params); 00116 00117 /// array of characteristic class pointers 00118 Characteristic **characteristics; 00119 /// array of gatt characteristic pointers 00120 GattCharacteristic **gattCharacteristics; 00121 /// pointer to mbed BLE layer 00122 BLE *ble; 00123 /// total number of characteristics 00124 int numberOfCharacteristics; 00125 /// flag to keep track of BLE connection state 00126 bool _isConnected; 00127 /// running index for building characteristic array 00128 int runningIndex; 00129 /// callback function for when a connection is made 00130 FunctionPointer _onConnect; 00131 /// callback function for when a connection is disconnected 00132 FunctionPointer _onDisconnect; 00133 /// callback function for when characteristic data is written 00134 PtrFunction _onDataWritten; 00135 }; 00136 00137 #endif // _BLUETOOTHLE_H_
Generated on Tue Jul 12 2022 17:59:19 by
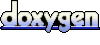