
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
BluetoothLE.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "BluetoothLE.h" 00034 #include "Characteristic.h" 00035 00036 /** 00037 * @brief Constructor for class 00038 */ 00039 BluetoothLE::BluetoothLE(BLE *ble, int numberOfCharacteristics) 00040 : ble(ble), numberOfCharacteristics(numberOfCharacteristics), runningIndex(0) 00041 { 00042 characteristics = new Characteristic *[numberOfCharacteristics]; 00043 gattCharacteristics = new GattCharacteristic *[numberOfCharacteristics]; 00044 } 00045 00046 /** 00047 * @brief Destructor for class 00048 */ 00049 BluetoothLE::~BluetoothLE(void) { 00050 for (int i = 0; i < numberOfCharacteristics; i++) delete characteristics[i]; 00051 delete[] gattCharacteristics; 00052 delete[] characteristics; 00053 } 00054 00055 /** 00056 * @brief Initialize the advertising, characteristics, service for this platform 00057 */ 00058 void BluetoothLE::addCharacteristic(Characteristic *characteristic) { 00059 characteristic->setBLE(ble); 00060 characteristic->setIndex(runningIndex); 00061 characteristics[runningIndex] = characteristic; 00062 gattCharacteristics[runningIndex] = characteristic->getGattCharacteristic(); 00063 runningIndex++; 00064 } 00065 00066 /** 00067 * @brief Initialize the advertising, characteristics, service for this platform 00068 */ 00069 void BluetoothLE::initService(uint8_t *serialNumber, uint8_t *deviceName, 00070 int nameSize, uint8_t *serviceUUID) { 00071 ble->init(); 00072 ble->onDisconnection(this, &BluetoothLE::disconnectionCallback); 00073 00074 ble->gap().setAddress((BLEProtocol::AddressType_t)0, serialNumber); 00075 00076 // Setup Advertising 00077 ble->accumulateAdvertisingPayload( 00078 GapAdvertisingData::BREDR_NOT_SUPPORTED | 00079 GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00080 ble->accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, 00081 (uint8_t *)deviceName, nameSize); 00082 ble->setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00083 ble->setAdvertisingInterval(1600); // 1000ms; in multiples of 0.625ms. 00084 GattService envService(serviceUUID, gattCharacteristics, 00085 numberOfCharacteristics); 00086 ble->gattServer().addService(envService); 00087 ble->onDataWritten(this, &BluetoothLE::onDataWritten); 00088 // Start Advertising 00089 ble->startAdvertising(); 00090 } 00091 00092 /** 00093 * @brief Called on BLE connection 00094 */ 00095 void BluetoothLE::connectionCallback( 00096 const Gap::ConnectionCallbackParams_t *params) { 00097 } 00098 00099 /** 00100 * @brief Start advertising on a disconnect 00101 */ 00102 void BluetoothLE::disconnectionCallback( 00103 const Gap::DisconnectionCallbackParams_t *params) { 00104 _isConnected = false; 00105 ble->startAdvertising(); 00106 } 00107 00108 /** 00109 * @brief Called when the client writes to a writable characteristic 00110 * @param params Pointer to a structure that contains details on what was 00111 * written 00112 */ 00113 void BluetoothLE::onDataWritten(const GattWriteCallbackParams *params) { 00114 int i; 00115 int index = 0; 00116 // match the characteristic handle 00117 printf("BluetoothLE::onDataWritten "); 00118 for (i = 0; i < numberOfCharacteristics; i++) { 00119 if (params->handle == gattCharacteristics[i]->getValueHandle()) { 00120 characteristics[i]->copyDataWritten(params); 00121 index = i; 00122 break; 00123 } 00124 } 00125 (*_onDataWritten)(index); 00126 } 00127 00128 /** 00129 * @brief Update the characteristic notification 00130 * @param index Index of the characteristic 00131 * @param data Pointer to the byte data to update the charateristic payload 00132 */ 00133 void BluetoothLE::notifyCharacteristic(int index, uint8_t *data) { 00134 for (int i = 0; i < characteristics[index]->getPayloadLength(); i++) { 00135 characteristics[index]->getPayloadBytes()[i] = data[i]; 00136 } 00137 characteristics[index]->update(); 00138 } 00139 00140 /** 00141 * @brief Update the characteristic notification 00142 * @param index Index of the characteristic 00143 * @param data Pointer to the byte data to update the charateristic payload 00144 */ 00145 void BluetoothLE::notifyCharacteristicTest(int index) { 00146 for (int i = 0; i < characteristics[index]->getPayloadLength(); i++) { 00147 characteristics[index]->getPayloadBytes()[i]++; 00148 } 00149 characteristics[index]->update(); 00150 } 00151 00152 uint8_t *BluetoothLE::getDataWritten(int index, int *length) { 00153 return characteristics[index]->getDataWritten(length); 00154 } 00155 00156 /** 00157 * @brief Function to query if a BLE connection is active 00158 * @return true if BLE connected, false if BLE is not connected 00159 */ 00160 bool BluetoothLE::isConnected(void) { 00161 return (ble->getGapState().connected == 1 ? true : false); 00162 }
Generated on Tue Jul 12 2022 17:59:19 by
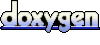