C code and C++ library, driver software for Maxim Integrated DS1775, DS75 thermometer and thermostat temperature sensor. Code supports continuous or shut-down/standby, hysteresis, alarm limits, comparator or interrupt mode, fault filtering, and active low/high. Compact 5-pin SOT23 packaging
Dependents: DS1775_Digital_Thermostat_Temperature
ds1775_c.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2019 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "ds1775.h" 00034 #include "ds1775_c.h" 00035 #include "mbed.h" 00036 // #include "USBSerial.h" 00037 00038 00039 /******************************************************************************/ 00040 /* C version for DS1775 driver */ 00041 /******************************************************************************/ 00042 00043 /* @var ds1775_write_address, ds1775_read_address 00044 * @brief I2C address 00045 */ 00046 uint8_t ds1775_write_address, ds1775_read_address; 00047 00048 int ds1775_init(uint8_t slave_address) 00049 { 00050 ds1775_write_address = (slave_address <<1); 00051 ds1775_read_address = ((slave_address << 1) | 1); 00052 return DS1775_NO_ERROR; 00053 } 00054 00055 /******************************************************************************/ 00056 int ds1775_read_cfg_reg(uint8_t *value, I2C &i2c_bus) 00057 { 00058 int32_t ret; 00059 char data[1] = {0}; 00060 char reg = DS1775_REG_CONFIGURATION; 00061 00062 /* write to the Register Select, true is for repeated start */ 00063 ret = i2c_bus.write(ds1775_write_address, ®, 1, true); 00064 if (ret == 0) { 00065 ret = i2c_bus.read(ds1775_read_address, data, 1, false); 00066 if (ret == 0) { 00067 *value = data[0]; 00068 return DS1775_NO_ERROR; 00069 } else { 00070 printf("%s: failed to read data: ret: %d\r\n", __func__, ret); 00071 } 00072 } else { 00073 printf("%s: failed to write to Register Select: ret: %d\r\n", 00074 __func__, ret); 00075 } 00076 return DS1775_ERROR; 00077 } 00078 00079 00080 /******************************************************************************/ 00081 int ds1775_read_reg16(int16_t *value, char reg, I2C &i2c_bus) 00082 { 00083 int32_t ret; 00084 char data[2] = {0, 0}; 00085 ds1775_raw_data tmp; 00086 00087 if (reg == DS1775_REG_TEMPERATURE || 00088 reg == DS1775_REG_THYST_LOW_TRIP || reg == DS1775_REG_TOS_HIGH_TRIP) { 00089 /* write to the Register Select, true is for repeated start */ 00090 ret = i2c_bus.write(ds1775_write_address, ®, 1, true); 00091 /* read the two bytes of data */ 00092 if (ret == 0) { 00093 ret = i2c_bus.read(ds1775_read_address, data, 2, false); 00094 if (ret == 0) { 00095 tmp.msb = data[0]; 00096 tmp.lsb = data[1]; 00097 *value = tmp.swrd; 00098 return DS1775_NO_ERROR; 00099 } else { 00100 printf( 00101 "%s: failed to read data: ret: %d\r\n", __func__, ret); 00102 } 00103 } else { 00104 printf("%s: failed to write to Register Select: ret: %d\r\n", 00105 __func__, ret); 00106 } 00107 } else { 00108 printf("%s: register address is not correct: register: %d\r\n", 00109 __func__, reg); 00110 } 00111 return DS1775_ERROR; 00112 } 00113 00114 float ds1775_read_reg_as_temperature(uint8_t reg, I2C &i2c_bus) 00115 { 00116 ds1775_raw_data tmp; 00117 float temperature; 00118 if (reg == DS1775_REG_TEMPERATURE || 00119 reg == DS1775_REG_THYST_LOW_TRIP || reg == DS1775_REG_TOS_HIGH_TRIP) { 00120 ds1775_read_reg16(&tmp.swrd, reg, i2c_bus); 00121 temperature = (float)tmp.swrd; /* values are 2's complement */ 00122 temperature *= DS1775_CF_LSB; 00123 return temperature; 00124 } else { 00125 printf("%s: register is invalid, %d r\n", __func__, reg); 00126 return 0; 00127 } 00128 } 00129 00130 00131 /******************************************************************************/ 00132 int ds1775_write_reg16(int16_t value, char reg, I2C &i2c_bus) 00133 { 00134 int32_t ret; 00135 char cmd[3]; 00136 ds1775_raw_data tmp; 00137 00138 if (reg >= DS1775_REG_THYST_LOW_TRIP && reg <= DS1775_REG_MAX) { 00139 cmd[0] = reg; 00140 tmp.swrd = value; 00141 cmd[1] = tmp.msb; 00142 cmd[2] = tmp.lsb; 00143 ret = i2c_bus.write(ds1775_write_address, cmd, 3, false); 00144 if (ret == 0) { 00145 return DS1775_NO_ERROR; 00146 } else { 00147 printf("%s: I2C write error %d\r\n",__func__, ret); 00148 return DS1775_ERROR; 00149 } 00150 } else { 00151 printf("%s: register value invalid %x\r\n",__func__, reg); 00152 return DS1775_ERROR; 00153 } 00154 } 00155 00156 00157 int ds1775_write_cfg_reg(uint8_t cfg, I2C &i2c_bus) 00158 { 00159 int32_t ret; 00160 char cmd[2]; 00161 00162 cmd[0] = DS1775_REG_CONFIGURATION; 00163 cmd[1] = cfg; 00164 ret = i2c_bus.write(ds1775_write_address, cmd, 2, false); 00165 if (ret == 0) { 00166 return DS1775_NO_ERROR; 00167 } else { 00168 printf("%s: I2C write error %d\r\n",__func__, ret); 00169 return DS1775_ERROR; 00170 } 00171 } 00172 00173 00174 int ds1775_write_trip_low_thyst(float temperature, I2C &i2c_bus) 00175 { 00176 ds1775_raw_data raw; 00177 temperature /= DS1775_CF_LSB; 00178 raw.swrd = int16_t(temperature); 00179 return ds1775_write_reg16(raw.swrd, DS1775_REG_THYST_LOW_TRIP, i2c_bus); 00180 } 00181 00182 00183 int ds1775_write_trip_high_tos(float temperature, I2C &i2c_bus) 00184 { 00185 ds1775_raw_data raw; 00186 temperature /= DS1775_CF_LSB; 00187 raw.swrd = int16_t(temperature); 00188 return ds1775_write_reg16(raw.swrd, DS1775_REG_TOS_HIGH_TRIP, i2c_bus); 00189 } 00190 00191 00192 float ds1775_celsius_to_fahrenheit(float temp_c) 00193 { 00194 float temp_f; 00195 temp_f = ((temp_c * 9)/5) + 32; 00196 return temp_f; 00197 }
Generated on Fri Jul 15 2022 10:27:39 by
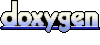