1-Wire® library for mbed. Complete 1-Wire library that supports our silicon masters along with a bit-bang master on the MAX32600MBED platform with one common interface for mbed. Slave support has also been included and more slaves will be added as time permits.
Dependents: MAXREFDES131_Qt_Demo MAX32630FTHR_iButton_uSD_Logger MAX32630FTHR_DS18B20_uSD_Logger MAXREFDES130_131_Demo ... more
DS28E15 Class Reference
Interface to the DS28E15 and DS28EL15 (low power) authenticators. More...
#include <DS28E15.h>
Inherits OneWire::DS28E15_22_25.
Public Types | |
typedef array< uint8_t, 4 > | Segment |
Holds the contents of a device memory segment. | |
typedef array< uint8_t, 32 > | Page |
Holds the contents of a device memory page. | |
typedef array< uint8_t, 32 > | Scratchpad |
Holds the contents of the device scratchpad. | |
typedef array< uint8_t, 32 > | Mac |
Container for a SHA-256 MAC. | |
typedef array< uint8_t, 2 > | ManId |
Container for a manufacturer ID. | |
Public Member Functions | |
DS28E15 (RandomAccessRomIterator &selector, bool lowVoltage=false) | |
CmdResult | writeScratchpad (const Scratchpad &data) const |
Perform Write Scratchpad operation on the device. | |
CmdResult | readScratchpad (Scratchpad &data) const |
Perform a Read Scratchpad operation on the device. | |
CmdResult | readBlockProtection (unsigned int blockNum, BlockProtection &protection) const |
Read the status of a memory protection block using the Read Status command. | |
CmdResult | readPersonality (Personality &personality) const |
Read the personality bytes using the Read Status command. | |
CmdResult | writeAuthSegment (const ISha256MacCoproc &MacCoproc, unsigned int pageNum, unsigned int segmentNum, const Segment &newData, const Segment &oldData, bool continuing=false) |
Write memory segment with authentication using the Authenticated Write Memory command. | |
CmdResult | writeAuthSegmentMac (unsigned int pageNum, unsigned int segmentNum, const Segment &newData, const Mac &mac, bool continuing=false) |
Write memory segment with authentication using the Authenticated Write Memory command. | |
CmdResult | readAllBlockProtection (array< BlockProtection, protectionBlocks > &protection) const |
Read the status of all memory protection blocks using the Read Status command. | |
CmdResult | loadSecret (bool lock) |
Perform Load and Lock Secret command on the device. | |
CmdResult | readSegment (unsigned int pageNum, unsigned int segmentNum, Segment &data, bool continuing=false) const |
Read memory segment using the Read Memory command on the device. | |
CmdResult | writeSegment (unsigned int pageNum, unsigned int segmentNum, const Segment &data, bool continuing=false) |
Write memory segment using the Write Memory command. | |
CmdResult | readPage (unsigned int pageNum, Page &rdbuf, bool continuing=false) const |
Read memory page using the Read Memory command on the device. | |
CmdResult | computeSecret (unsigned int pageNum, bool lock) |
Perform a Compute and Lock Secret command on the device. | |
CmdResult | computeReadPageMac (unsigned int pageNum, bool anon, Mac &mac) const |
Perform a Compute Page MAC command on the device. | |
CmdResult | writeBlockProtection (const BlockProtection &protection) |
Update the status of a memory protection block using the Write Page Protection command. | |
CmdResult | writeAuthBlockProtection (const ISha256MacCoproc &MacCoproc, const BlockProtection &newProtection, const BlockProtection &oldProtection) |
Update the status of a memory protection block using the Authenticated Write Page Protection command. | |
ManId | manId () const |
bool | lowVoltage () const |
RomId | romId () const |
Static Public Member Functions | |
static ISha256MacCoproc::CmdResult | computeSegmentWriteMac (const ISha256MacCoproc &MacCoproc, unsigned int pageNum, unsigned int segmentNum, const Segment &newData, const Segment &oldData, const RomId &romId, const ManId &manId, Mac &mac) |
Compute the MAC for an Authenticated Write to a memory segment. | |
static ISha256MacCoproc::CmdResult | computeProtectionWriteMac (const ISha256MacCoproc &MacCoproc, const BlockProtection &newProtection, const BlockProtection &oldProtection, const RomId &romId, const ManId &manId, Mac &mac) |
Compute the MAC for an Authenticated Write to a memory protection block. | |
static ISha256MacCoproc::CmdResult | computeNextSecret (ISha256MacCoproc &MacCoproc, const Page &bindingPage, unsigned int bindingPageNum, const Scratchpad &partialSecret, const RomId &romId, const ManId &manId) |
Compute the next secret from the existing secret. | |
static ISha256MacCoproc::CmdResult | computeAuthMac (const ISha256MacCoproc &MacCoproc, const Page &pageData, unsigned int pageNum, const Scratchpad &challenge, const RomId &romId, const ManId &manId, Mac &mac) |
Compute a Page MAC for authentication. | |
static ISha256MacCoproc::CmdResult | computeAuthMacAnon (const ISha256MacCoproc &MacCoproc, const Page &pageData, unsigned int pageNum, const Scratchpad &challenge, const ManId &manId, Mac &mac) |
Compute a Page MAC for authentication using anonymous mode. | |
static Segment | segmentFromPage (unsigned int segmentNum, const Page &page) |
Creates a segment representation from a subsection of the page data. | |
static void | segmentToPage (unsigned int segmentNum, const Segment &segment, Page &page) |
Copies segment data to the page. | |
Static Public Attributes | |
static const unsigned int | segmentsPerPage = (Page::csize / Segment::csize) |
Number of segments per page. | |
Protected Member Functions | |
OneWireMaster::CmdResult | selectDevice () const |
Select this slave device by ROM ID. | |
OneWireMaster & | master () const |
The 1-Wire master for this slave device. |
Detailed Description
Interface to the DS28E15 and DS28EL15 (low power) authenticators.
Definition at line 41 of file DS28E15.h.
Member Typedef Documentation
Container for a SHA-256 MAC.
Definition at line 56 of file DS28E15_22_25.h.
Container for a manufacturer ID.
Definition at line 59 of file DS28E15_22_25.h.
Holds the contents of a device memory page.
Definition at line 50 of file DS28E15_22_25.h.
typedef array<uint8_t, 32> Scratchpad [inherited] |
Holds the contents of the device scratchpad.
Definition at line 53 of file DS28E15_22_25.h.
Holds the contents of a device memory segment.
Definition at line 47 of file DS28E15_22_25.h.
Constructor & Destructor Documentation
DS28E15 | ( | RandomAccessRomIterator & | selector, |
bool | lowVoltage = false |
||
) |
Member Function Documentation
ISha256MacCoproc::CmdResult computeAuthMac | ( | const ISha256MacCoproc & | MacCoproc, |
const Page & | pageData, | ||
unsigned int | pageNum, | ||
const Scratchpad & | challenge, | ||
const RomId & | romId, | ||
const ManId & | manId, | ||
Mac & | mac | ||
) | [static, inherited] |
Compute a Page MAC for authentication.
- Parameters:
-
MacCoproc Coprocessor with Slave Secret to use for the operation. [in] pageData Data from a device memory page. pageNum Number of the page to use data from. [in] challenge Random challenge to prevent replay attacks. [in] romId 1-Wire ROM ID of the device. [in] manId Manufacturer ID of the device. [out] mac The computed MAC.
Definition at line 411 of file DS28E15_22_25.cpp.
ISha256MacCoproc::CmdResult computeAuthMacAnon | ( | const ISha256MacCoproc & | MacCoproc, |
const Page & | pageData, | ||
unsigned int | pageNum, | ||
const Scratchpad & | challenge, | ||
const ManId & | manId, | ||
Mac & | mac | ||
) | [static, inherited] |
Compute a Page MAC for authentication using anonymous mode.
- Parameters:
-
MacCoproc Coprocessor with Slave Secret to use for the operation. [in] pageData Data from a device memory page. pageNum Number of the page to use data from. [in] challenge Random challenge to prevent replay attacks. [in] manId Manufacturer ID of the device. [out] mac The computed MAC.
Definition at line 428 of file DS28E15_22_25.cpp.
ISha256MacCoproc::CmdResult computeNextSecret | ( | ISha256MacCoproc & | MacCoproc, |
const Page & | bindingPage, | ||
unsigned int | bindingPageNum, | ||
const Scratchpad & | partialSecret, | ||
const RomId & | romId, | ||
const ManId & | manId | ||
) | [static, inherited] |
Compute the next secret from the existing secret.
- Parameters:
-
MacCoproc Coprocessor with Master Secret to use for the operation. Slave Secret will be updated with the computation result. [in] bindingPage Binding data from a device memory page. bindingPageNum Number of the page where the binding data is from. [in] partialSecret Partial secret data from the device scratchpad. [in] romId 1-Wire ROM ID of the device. [in] manId Manufacturer ID of the device.
- Returns:
- The result code indicated by the coprocessor.
Definition at line 1164 of file DS28E15_22_25.cpp.
ISha256MacCoproc::CmdResult computeProtectionWriteMac | ( | const ISha256MacCoproc & | MacCoproc, |
const BlockProtection & | newProtection, | ||
const BlockProtection & | oldProtection, | ||
const RomId & | romId, | ||
const ManId & | manId, | ||
Mac & | mac | ||
) | [static, inherited] |
Compute the MAC for an Authenticated Write to a memory protection block.
- Parameters:
-
MacCoproc Coprocessor with Slave Secret to use for the operation. [in] newProtection New protection status to write. [in] oldProtection Existing protection status in device. [in] romId 1-Wire ROM ID of the device. [in] manId Manufacturer ID of the device. [out] mac The computed MAC.
- Returns:
- The result code indicated by the coprocessor.
Definition at line 891 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult computeReadPageMac | ( | unsigned int | pageNum, |
bool | anon, | ||
Mac & | mac | ||
) | const [inherited] |
Perform a Compute Page MAC command on the device.
Read back the MAC and verify the CRC16.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
pageNum Page number to use for the computation. anon True to compute in anonymous mode where ROM ID is not used. [out] mac The device computed MAC.
Definition at line 435 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult computeSecret | ( | unsigned int | pageNum, |
bool | lock | ||
) | [inherited] |
Perform a Compute and Lock Secret command on the device.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
pageNum Page number to use as the binding data. lock Prevent further changes to the secret on the device after computing.
Definition at line 491 of file DS28E15_22_25.cpp.
ISha256MacCoproc::CmdResult computeSegmentWriteMac | ( | const ISha256MacCoproc & | MacCoproc, |
unsigned int | pageNum, | ||
unsigned int | segmentNum, | ||
const Segment & | newData, | ||
const Segment & | oldData, | ||
const RomId & | romId, | ||
const ManId & | manId, | ||
Mac & | mac | ||
) | [static, inherited] |
Compute the MAC for an Authenticated Write to a memory segment.
- Parameters:
-
MacCoproc Coprocessor with Slave Secret to use for the computation. pageNum Page number for write operation. segmentNum Segment number within page for write operation. [in] newData New data to write to the segment. [in] oldData Existing data contained in the segment. [in] romId 1-Wire ROM ID of the device. [in] manId Manufacturer ID of the device. [out] mac The computed MAC.
- Returns:
- The result code indicated by the coprocessor.
Definition at line 870 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult loadSecret | ( | bool | lock ) | [inherited] |
Perform Load and Lock Secret command on the device.
- Note:
- The secret should already be stored in the scratchpad on the device.
- Parameters:
-
lock Prevent further changes to the secret on the device after loading.
Definition at line 649 of file DS28E15_22_25.cpp.
bool lowVoltage | ( | ) | const [inherited] |
Enable low voltage timing
Definition at line 237 of file DS28E15_22_25.h.
ManId manId | ( | ) | const [inherited] |
Manufacturer ID
Definition at line 231 of file DS28E15_22_25.h.
OneWireMaster& master | ( | ) | const [protected, inherited] |
The 1-Wire master for this slave device.
Definition at line 77 of file OneWireSlave.h.
OneWireSlave::CmdResult readAllBlockProtection | ( | array< BlockProtection, protectionBlocks > & | protection ) | const |
Read the status of all memory protection blocks using the Read Status command.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
[out] protection Receives protection statuses read from device.
Definition at line 1224 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult readBlockProtection | ( | unsigned int | blockNum, |
BlockProtection & | protection | ||
) | const |
Read the status of a memory protection block using the Read Status command.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
blockNum Block number to to read status of. [out] protection Receives protection status read from device.
Definition at line 1204 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult readPage | ( | unsigned int | pageNum, |
Page & | rdbuf, | ||
bool | continuing = false |
||
) | const [inherited] |
Read memory page using the Read Memory command on the device.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
pageNum Page number for write operation. [out] rdbuf Buffer to read data from the page into. continuing True if continuing a previous Read Memory command. False to begin a new command.
Definition at line 695 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult readPersonality | ( | Personality & | personality ) | const |
Read the personality bytes using the Read Status command.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
[out] personality Receives personality read from device.
Definition at line 1209 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult readScratchpad | ( | Scratchpad & | data ) | const |
Perform a Read Scratchpad operation on the device.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
[out] data Buffer to read data from the scratchpad into.
Definition at line 1199 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult readSegment | ( | unsigned int | pageNum, |
unsigned int | segmentNum, | ||
Segment & | data, | ||
bool | continuing = false |
||
) | const [inherited] |
Read memory segment using the Read Memory command on the device.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
pageNum Page number for read operation. segmentNum Segment number within page for read operation. [out] data Buffer to read data from the segment into. continuing True if continuing a previous Read Memory command. False to begin a new command.
Definition at line 1050 of file DS28E15_22_25.cpp.
RomId romId | ( | ) | const [inherited] |
1-Wire ROM ID for this slave device.
Definition at line 59 of file OneWireSlave.h.
DS28E15_22_25::Segment segmentFromPage | ( | unsigned int | segmentNum, |
const Page & | page | ||
) | [static, inherited] |
Creates a segment representation from a subsection of the page data.
- Parameters:
-
segmentNum Segment number within page to copy from.
- Returns:
- The copied segment data.
Definition at line 60 of file DS28E15_22_25.cpp.
void segmentToPage | ( | unsigned int | segmentNum, |
const Segment & | segment, | ||
Page & | page | ||
) | [static, inherited] |
Copies segment data to the page.
- Parameters:
-
segmentNum Segment number within the page to copy to. [in] segment Segment to copy from.
Definition at line 72 of file DS28E15_22_25.cpp.
OneWireMaster::CmdResult selectDevice | ( | ) | const [protected, inherited] |
Select this slave device by ROM ID.
Definition at line 74 of file OneWireSlave.h.
OneWireSlave::CmdResult writeAuthBlockProtection | ( | const ISha256MacCoproc & | MacCoproc, |
const BlockProtection & | newProtection, | ||
const BlockProtection & | oldProtection | ||
) | [inherited] |
Update the status of a memory protection block using the Authenticated Write Page Protection command.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
MacCoproc Coprocessor with Slave Secret to use for the operation. [in] newProtection New protection status to write. [in] oldProtection Existing protection status in device. continuing True to continue a previous Authenticated Write Page Protection command. False to begin a new command.
Definition at line 155 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult writeAuthSegment | ( | const ISha256MacCoproc & | MacCoproc, |
unsigned int | pageNum, | ||
unsigned int | segmentNum, | ||
const Segment & | newData, | ||
const Segment & | oldData, | ||
bool | continuing = false |
||
) |
Write memory segment with authentication using the Authenticated Write Memory command.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
MacCoproc Coprocessor to use for Write MAC computation. pageNum Page number for write operation. segmentNum Segment number within page for write operation. [in] newData New data to write to the segment. [in] oldData Existing data contained in the segment. continuing True to continue writing with the next sequential segment. False to begin a new command.
Definition at line 1214 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult writeAuthSegmentMac | ( | unsigned int | pageNum, |
unsigned int | segmentNum, | ||
const Segment & | newData, | ||
const Mac & | mac, | ||
bool | continuing = false |
||
) |
Write memory segment with authentication using the Authenticated Write Memory command.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
pageNum Page number for write operation. segmentNum Segment number within page for write operation. [in] newData New data to write to the segment. [in] mac Write MAC computed for this operation. continuing True to continue writing with the next sequential segment. False to begin a new command.
Definition at line 1219 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult writeBlockProtection | ( | const BlockProtection & | protection ) | [inherited] |
Update the status of a memory protection block using the Write Page Protection command.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
[in] Desired protection status for the block. It is not possible to disable existing protections. continuing True to continue a previous Write Page Protection command. False to begin a new command.
Definition at line 238 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult writeScratchpad | ( | const Scratchpad & | data ) | const |
Perform Write Scratchpad operation on the device.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
[in] data Data to write to the scratchpad.
Definition at line 1194 of file DS28E15_22_25.cpp.
OneWireSlave::CmdResult writeSegment | ( | unsigned int | pageNum, |
unsigned int | segmentNum, | ||
const Segment & | data, | ||
bool | continuing = false |
||
) | [inherited] |
Write memory segment using the Write Memory command.
- Note:
- 1-Wire ROM selection should have already occurred.
- Parameters:
-
pageNum Page number for write operation. segmentNum Segment number within page for write operation. [in] data Data to write to the memory segment. continuing True to continue writing with the next sequential segment. False to begin a new command.
Definition at line 1086 of file DS28E15_22_25.cpp.
Field Documentation
const unsigned int segmentsPerPage = (Page::csize / Segment::csize) [static, inherited] |
Number of segments per page.
Definition at line 217 of file DS28E15_22_25.h.
Generated on Tue Jul 12 2022 15:46:21 by
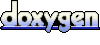