
DeepCover Embedded Security in IoT: Public-key Secured Data Paths
Dependencies: MaximInterface
socket.h
00001 /* 00002 * socket.h - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2015 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 /*****************************************************************************/ 00038 /* Include files */ 00039 /*****************************************************************************/ 00040 #include "simplelink.h" 00041 00042 #ifndef __SL_SOCKET_H__ 00043 #define __SL_SOCKET_H__ 00044 00045 00046 00047 00048 #ifdef __cplusplus 00049 extern "C" { 00050 #endif 00051 00052 /*! 00053 00054 \addtogroup socket 00055 @{ 00056 00057 */ 00058 00059 /*****************************************************************************/ 00060 /* Macro declarations */ 00061 /*****************************************************************************/ 00062 00063 #define SL_FD_SETSIZE SL_MAX_SOCKETS /* Number of sockets to select on - same is max sockets! */ 00064 #define BSD_SOCKET_ID_MASK (0x0F) /* Index using the LBS 4 bits for socket id 0-7 */ 00065 /* Define some BSD protocol constants. */ 00066 #define SL_SOCK_STREAM (1) /* TCP Socket */ 00067 #define SL_SOCK_DGRAM (2) /* UDP Socket */ 00068 #define SL_SOCK_RAW (3) /* Raw socket */ 00069 #define SL_IPPROTO_TCP (6) /* TCP Raw Socket */ 00070 #define SL_IPPROTO_UDP (17) /* UDP Raw Socket */ 00071 #define SL_IPPROTO_RAW (255) /* Raw Socket */ 00072 #define SL_SEC_SOCKET (100) /* Secured Socket Layer (SSL,TLS) */ 00073 00074 /* Address families. */ 00075 #define SL_AF_INET (2) /* IPv4 socket (UDP, TCP, etc) */ 00076 #define SL_AF_INET6 (3) /* IPv6 socket (UDP, TCP, etc) */ 00077 #define SL_AF_INET6_EUI_48 (9) 00078 #define SL_AF_RF (6) /* data include RF parameter, All layer by user (Wifi could be disconnected) */ 00079 #define SL_AF_PACKET (17) 00080 /* Protocol families, same as address families. */ 00081 #define SL_PF_INET AF_INET 00082 #define SL_PF_INET6 AF_INET6 00083 #define SL_INADDR_ANY (0) /* bind any address */ 00084 00085 /* error codes */ 00086 #define SL_SOC_ERROR (-1) /* Failure. */ 00087 #define SL_SOC_OK ( 0) /* Success. */ 00088 #define SL_INEXE (-8) /* socket command in execution */ 00089 #define SL_EBADF (-9) /* Bad file number */ 00090 #define SL_ENSOCK (-10) /* The system limit on the total number of open socket, has been reached */ 00091 #define SL_EAGAIN (-11) /* Try again */ 00092 #define SL_EWOULDBLOCK SL_EAGAIN 00093 #define SL_ENOMEM (-12) /* Out of memory */ 00094 #define SL_EACCES (-13) /* Permission denied */ 00095 #define SL_EFAULT (-14) /* Bad address */ 00096 #define SL_ECLOSE (-15) /* close socket operation failed to transmit all queued packets */ 00097 #define SL_EALREADY_ENABLED (-21) /* Transceiver - Transceiver already ON. there could be only one */ 00098 #define SL_EINVAL (-22) /* Invalid argument */ 00099 #define SL_EAUTO_CONNECT_OR_CONNECTING (-69) /* Transceiver - During connection, connected or auto mode started */ 00100 #define SL_CONNECTION_PENDING (-72) /* Transceiver - Device is connected, disconnect first to open transceiver */ 00101 #define SL_EUNSUPPORTED_ROLE (-86) /* Transceiver - Trying to start when WLAN role is AP or P2P GO */ 00102 #define SL_EDESTADDRREQ (-89) /* Destination address required */ 00103 #define SL_EPROTOTYPE (-91) /* Protocol wrong type for socket */ 00104 #define SL_ENOPROTOOPT (-92) /* Protocol not available */ 00105 #define SL_EPROTONOSUPPORT (-93) /* Protocol not supported */ 00106 #define SL_ESOCKTNOSUPPORT (-94) /* Socket type not supported */ 00107 #define SL_EOPNOTSUPP (-95) /* Operation not supported on transport endpoint */ 00108 #define SL_EAFNOSUPPORT (-97) /* Address family not supported by protocol */ 00109 #define SL_EADDRINUSE (-98) /* Address already in use */ 00110 #define SL_EADDRNOTAVAIL (-99) /* Cannot assign requested address */ 00111 #define SL_ENETUNREACH (-101) /* Network is unreachable */ 00112 #define SL_ENOBUFS (-105) /* No buffer space available */ 00113 #define SL_EOBUFF SL_ENOBUFS 00114 #define SL_EISCONN (-106) /* Transport endpoint is already connected */ 00115 #define SL_ENOTCONN (-107) /* Transport endpoint is not connected */ 00116 #define SL_ETIMEDOUT (-110) /* Connection timed out */ 00117 #define SL_ECONNREFUSED (-111) /* Connection refused */ 00118 #define SL_EALREADY (-114) /* Non blocking connect in progress, try again */ 00119 00120 #define SL_ESEC_RSA_WRONG_TYPE_E (-130) /* RSA wrong block type for RSA function */ 00121 #define SL_ESEC_RSA_BUFFER_E (-131) /* RSA buffer error, output too small or */ 00122 #define SL_ESEC_BUFFER_E (-132) /* output buffer too small or input too large */ 00123 #define SL_ESEC_ALGO_ID_E (-133) /* setting algo id error */ 00124 #define SL_ESEC_PUBLIC_KEY_E (-134) /* setting public key error */ 00125 #define SL_ESEC_DATE_E (-135) /* setting date validity error */ 00126 #define SL_ESEC_SUBJECT_E (-136) /* setting subject name error */ 00127 #define SL_ESEC_ISSUER_E (-137) /* setting issuer name error */ 00128 #define SL_ESEC_CA_TRUE_E (-138) /* setting CA basic constraint true error */ 00129 #define SL_ESEC_EXTENSIONS_E (-139) /* setting extensions error */ 00130 #define SL_ESEC_ASN_PARSE_E (-140) /* ASN parsing error, invalid input */ 00131 #define SL_ESEC_ASN_VERSION_E (-141) /* ASN version error, invalid number */ 00132 #define SL_ESEC_ASN_GETINT_E (-142) /* ASN get big _i16 error, invalid data */ 00133 #define SL_ESEC_ASN_RSA_KEY_E (-143) /* ASN key init error, invalid input */ 00134 #define SL_ESEC_ASN_OBJECT_ID_E (-144) /* ASN object id error, invalid id */ 00135 #define SL_ESEC_ASN_TAG_NULL_E (-145) /* ASN tag error, not null */ 00136 #define SL_ESEC_ASN_EXPECT_0_E (-146) /* ASN expect error, not zero */ 00137 #define SL_ESEC_ASN_BITSTR_E (-147) /* ASN bit string error, wrong id */ 00138 #define SL_ESEC_ASN_UNKNOWN_OID_E (-148) /* ASN oid error, unknown sum id */ 00139 #define SL_ESEC_ASN_DATE_SZ_E (-149) /* ASN date error, bad size */ 00140 #define SL_ESEC_ASN_BEFORE_DATE_E (-150) /* ASN date error, current date before */ 00141 #define SL_ESEC_ASN_AFTER_DATE_E (-151) /* ASN date error, current date after */ 00142 #define SL_ESEC_ASN_SIG_OID_E (-152) /* ASN signature error, mismatched oid */ 00143 #define SL_ESEC_ASN_TIME_E (-153) /* ASN time error, unknown time type */ 00144 #define SL_ESEC_ASN_INPUT_E (-154) /* ASN input error, not enough data */ 00145 #define SL_ESEC_ASN_SIG_CONFIRM_E (-155) /* ASN sig error, confirm failure */ 00146 #define SL_ESEC_ASN_SIG_HASH_E (-156) /* ASN sig error, unsupported hash type */ 00147 #define SL_ESEC_ASN_SIG_KEY_E (-157) /* ASN sig error, unsupported key type */ 00148 #define SL_ESEC_ASN_DH_KEY_E (-158) /* ASN key init error, invalid input */ 00149 #define SL_ESEC_ASN_NTRU_KEY_E (-159) /* ASN ntru key decode error, invalid input */ 00150 #define SL_ESEC_ECC_BAD_ARG_E (-170) /* ECC input argument of wrong type */ 00151 #define SL_ESEC_ASN_ECC_KEY_E (-171) /* ASN ECC bad input */ 00152 #define SL_ESEC_ECC_CURVE_OID_E (-172) /* Unsupported ECC OID curve type */ 00153 #define SL_ESEC_BAD_FUNC_ARG (-173) /* Bad function argument provided */ 00154 #define SL_ESEC_NOT_COMPILED_IN (-174) /* Feature not compiled in */ 00155 #define SL_ESEC_UNICODE_SIZE_E (-175) /* Unicode password too big */ 00156 #define SL_ESEC_NO_PASSWORD (-176) /* no password provided by user */ 00157 #define SL_ESEC_ALT_NAME_E (-177) /* alt name size problem, too big */ 00158 #define SL_ESEC_AES_GCM_AUTH_E (-180) /* AES-GCM Authentication check failure */ 00159 #define SL_ESEC_AES_CCM_AUTH_E (-181) /* AES-CCM Authentication check failure */ 00160 #define SL_SOCKET_ERROR_E (-208) /* Error state on socket */ 00161 00162 #define SL_ESEC_MEMORY_ERROR (-203) /* out of memory */ 00163 #define SL_ESEC_VERIFY_FINISHED_ERROR (-204) /* verify problem on finished */ 00164 #define SL_ESEC_VERIFY_MAC_ERROR (-205) /* verify mac problem */ 00165 #define SL_ESEC_UNKNOWN_HANDSHAKE_TYPE (-207) /* weird handshake type */ 00166 #define SL_ESEC_SOCKET_ERROR_E (-208) /* error state on socket */ 00167 #define SL_ESEC_SOCKET_NODATA (-209) /* expected data, not there */ 00168 #define SL_ESEC_INCOMPLETE_DATA (-210) /* don't have enough data to complete task */ 00169 #define SL_ESEC_UNKNOWN_RECORD_TYPE (-211) /* unknown type in record hdr */ 00170 #define SL_ESEC_FATAL_ERROR (-213) /* recvd alert fatal error */ 00171 #define SL_ESEC_ENCRYPT_ERROR (-214) /* error during encryption */ 00172 #define SL_ESEC_NO_PEER_KEY (-216) /* need peer's key */ 00173 #define SL_ESEC_NO_PRIVATE_KEY (-217) /* need the private key */ 00174 #define SL_ESEC_RSA_PRIVATE_ERROR (-218) /* error during rsa priv op */ 00175 #define SL_ESEC_NO_DH_PARAMS (-219) /* server missing DH params */ 00176 #define SL_ESEC_BUILD_MSG_ERROR (-220) /* build message failure */ 00177 #define SL_ESEC_BAD_HELLO (-221) /* client hello malformed */ 00178 #define SL_ESEC_DOMAIN_NAME_MISMATCH (-222) /* peer subject name mismatch */ 00179 #define SL_ESEC_WANT_READ (-223) /* want read, call again */ 00180 #define SL_ESEC_NOT_READY_ERROR (-224) /* handshake layer not ready */ 00181 #define SL_ESEC_PMS_VERSION_ERROR (-225) /* pre m secret version error */ 00182 #define SL_ESEC_VERSION_ERROR (-226) /* record layer version error */ 00183 #define SL_ESEC_WANT_WRITE (-227) /* want write, call again */ 00184 #define SL_ESEC_BUFFER_ERROR (-228) /* malformed buffer input */ 00185 #define SL_ESEC_VERIFY_CERT_ERROR (-229) /* verify cert error */ 00186 #define SL_ESEC_VERIFY_SIGN_ERROR (-230) /* verify sign error */ 00187 00188 #define SL_ESEC_LENGTH_ERROR (-241) /* record layer length error */ 00189 #define SL_ESEC_PEER_KEY_ERROR (-242) /* can't decode peer key */ 00190 #define SL_ESEC_ZERO_RETURN (-243) /* peer sent close notify */ 00191 #define SL_ESEC_SIDE_ERROR (-244) /* wrong client/server type */ 00192 #define SL_ESEC_NO_PEER_CERT (-245) /* peer didn't send key */ 00193 #define SL_ESEC_ECC_CURVETYPE_ERROR (-250) /* Bad ECC Curve Type */ 00194 #define SL_ESEC_ECC_CURVE_ERROR (-251) /* Bad ECC Curve */ 00195 #define SL_ESEC_ECC_PEERKEY_ERROR (-252) /* Bad Peer ECC Key */ 00196 #define SL_ESEC_ECC_MAKEKEY_ERROR (-253) /* Bad Make ECC Key */ 00197 #define SL_ESEC_ECC_EXPORT_ERROR (-254) /* Bad ECC Export Key */ 00198 #define SL_ESEC_ECC_SHARED_ERROR (-255) /* Bad ECC Shared Secret */ 00199 #define SL_ESEC_NOT_CA_ERROR (-257) /* Not a CA cert error */ 00200 #define SL_ESEC_BAD_PATH_ERROR (-258) /* Bad path for opendir */ 00201 #define SL_ESEC_BAD_CERT_MANAGER_ERROR (-259) /* Bad Cert Manager */ 00202 #define SL_ESEC_MAX_CHAIN_ERROR (-268) /* max chain depth exceeded */ 00203 #define SL_ESEC_SUITES_ERROR (-271) /* suites pointer error */ 00204 #define SL_ESEC_SSL_NO_PEM_HEADER (-272) /* no PEM header found */ 00205 #define SL_ESEC_OUT_OF_ORDER_E (-273) /* out of order message */ 00206 #define SL_ESEC_SANITY_CIPHER_E (-275) /* sanity check on cipher error */ 00207 #define SL_ESEC_GEN_COOKIE_E (-277) /* Generate Cookie Error */ 00208 #define SL_ESEC_NO_PEER_VERIFY (-278) /* Need peer cert verify Error */ 00209 #define SL_ESEC_UNKNOWN_SNI_HOST_NAME_E (-281) /* Unrecognized host name Error */ 00210 /* begin negotiation parameter errors */ 00211 #define SL_ESEC_UNSUPPORTED_SUITE (-290) /* unsupported cipher suite */ 00212 #define SL_ESEC_MATCH_SUITE_ERROR (-291 ) /* can't match cipher suite */ 00213 00214 /* ssl tls security start with -300 offset */ 00215 #define SL_ESEC_CLOSE_NOTIFY (-300) /* ssl/tls alerts */ 00216 #define SL_ESEC_UNEXPECTED_MESSAGE (-310) /* ssl/tls alerts */ 00217 #define SL_ESEC_BAD_RECORD_MAC (-320) /* ssl/tls alerts */ 00218 #define SL_ESEC_DECRYPTION_FAILED (-321) /* ssl/tls alerts */ 00219 #define SL_ESEC_RECORD_OVERFLOW (-322) /* ssl/tls alerts */ 00220 #define SL_ESEC_DECOMPRESSION_FAILURE (-330) /* ssl/tls alerts */ 00221 #define SL_ESEC_HANDSHAKE_FAILURE (-340) /* ssl/tls alerts */ 00222 #define SL_ESEC_NO_CERTIFICATE (-341) /* ssl/tls alerts */ 00223 #define SL_ESEC_BAD_CERTIFICATE (-342) /* ssl/tls alerts */ 00224 #define SL_ESEC_UNSUPPORTED_CERTIFICATE (-343) /* ssl/tls alerts */ 00225 #define SL_ESEC_CERTIFICATE_REVOKED (-344) /* ssl/tls alerts */ 00226 #define SL_ESEC_CERTIFICATE_EXPIRED (-345) /* ssl/tls alerts */ 00227 #define SL_ESEC_CERTIFICATE_UNKNOWN (-346) /* ssl/tls alerts */ 00228 #define SL_ESEC_ILLEGAL_PARAMETER (-347) /* ssl/tls alerts */ 00229 #define SL_ESEC_UNKNOWN_CA (-348) /* ssl/tls alerts */ 00230 #define SL_ESEC_ACCESS_DENIED (-349) /* ssl/tls alerts */ 00231 #define SL_ESEC_DECODE_ERROR (-350) /* ssl/tls alerts */ 00232 #define SL_ESEC_DECRYPT_ERROR (-351) /* ssl/tls alerts */ 00233 #define SL_ESEC_EXPORT_RESTRICTION (-360) /* ssl/tls alerts */ 00234 #define SL_ESEC_PROTOCOL_VERSION (-370) /* ssl/tls alerts */ 00235 #define SL_ESEC_INSUFFICIENT_SECURITY (-371) /* ssl/tls alerts */ 00236 #define SL_ESEC_INTERNAL_ERROR (-380) /* ssl/tls alerts */ 00237 #define SL_ESEC_USER_CANCELLED (-390) /* ssl/tls alerts */ 00238 #define SL_ESEC_NO_RENEGOTIATION (-400) /* ssl/tls alerts */ 00239 #define SL_ESEC_UNSUPPORTED_EXTENSION (-410) /* ssl/tls alerts */ 00240 #define SL_ESEC_CERTIFICATE_UNOBTAINABLE (-411) /* ssl/tls alerts */ 00241 #define SL_ESEC_UNRECOGNIZED_NAME (-412) /* ssl/tls alerts */ 00242 #define SL_ESEC_BAD_CERTIFICATE_STATUS_RESPONSE (-413) /* ssl/tls alerts */ 00243 #define SL_ESEC_BAD_CERTIFICATE_HASH_VALUE (-414) /* ssl/tls alerts */ 00244 /* propierty secure */ 00245 #define SL_ESECGENERAL (-450) /* error secure level general error */ 00246 #define SL_ESECDECRYPT (-451) /* error secure level, decrypt recv packet fail */ 00247 #define SL_ESECCLOSED (-452) /* secure layrer is closed by other size , tcp is still connected */ 00248 #define SL_ESECSNOVERIFY (-453) /* Connected without server verification */ 00249 #define SL_ESECNOCAFILE (-454) /* error secure level CA file not found*/ 00250 #define SL_ESECMEMORY (-455) /* error secure level No memory space available */ 00251 #define SL_ESECBADCAFILE (-456) /* error secure level bad CA file */ 00252 #define SL_ESECBADCERTFILE (-457) /* error secure level bad Certificate file */ 00253 #define SL_ESECBADPRIVATEFILE (-458) /* error secure level bad private file */ 00254 #define SL_ESECBADDHFILE (-459) /* error secure level bad DH file */ 00255 #define SL_ESECT00MANYSSLOPENED (-460) /* MAX SSL Sockets are opened */ 00256 #define SL_ESECDATEERROR (-461) /* connected with certificate date verification error */ 00257 #define SL_ESECHANDSHAKETIMEDOUT (-462) /* connection timed out due to handshake time */ 00258 00259 /* end error codes */ 00260 00261 /* Max payload size by protocol */ 00262 #define SL_SOCKET_PAYLOAD_TYPE_MASK (0xF0) /*4 bits type, 4 bits sockets id */ 00263 #define SL_SOCKET_PAYLOAD_TYPE_UDP_IPV4 (0x00) /* 1472 bytes */ 00264 #define SL_SOCKET_PAYLOAD_TYPE_TCP_IPV4 (0x10) /* 1460 bytes */ 00265 #define SL_SOCKET_PAYLOAD_TYPE_UDP_IPV6 (0x20) /* 1452 bytes */ 00266 #define SL_SOCKET_PAYLOAD_TYPE_TCP_IPV6 (0x30) /* 1440 bytes */ 00267 #define SL_SOCKET_PAYLOAD_TYPE_UDP_IPV4_SECURE (0x40) /* */ 00268 #define SL_SOCKET_PAYLOAD_TYPE_TCP_IPV4_SECURE (0x50) /* */ 00269 #define SL_SOCKET_PAYLOAD_TYPE_UDP_IPV6_SECURE (0x60) /* */ 00270 #define SL_SOCKET_PAYLOAD_TYPE_TCP_IPV6_SECURE (0x70) /* */ 00271 #define SL_SOCKET_PAYLOAD_TYPE_RAW_TRANCEIVER (0x80) /* 1536 bytes */ 00272 #define SL_SOCKET_PAYLOAD_TYPE_RAW_PACKET (0x90) /* 1536 bytes */ 00273 #define SL_SOCKET_PAYLOAD_TYPE_RAW_IP4 (0xa0) 00274 #define SL_SOCKET_PAYLOAD_TYPE_RAW_IP6 (SL_SOCKET_PAYLOAD_TYPE_RAW_IP4 ) 00275 00276 00277 00278 #define SL_SOL_SOCKET (1) /* Define the socket option category. */ 00279 #define SL_IPPROTO_IP (2) /* Define the IP option category. */ 00280 #define SL_SOL_PHY_OPT (3) /* Define the PHY option category. */ 00281 00282 #define SL_SO_RCVBUF (8) /* Setting TCP receive buffer size */ 00283 #define SL_SO_KEEPALIVE (9) /* Connections are kept alive with periodic messages */ 00284 #define SL_SO_RCVTIMEO (20) /* Enable receive timeout */ 00285 #define SL_SO_NONBLOCKING (24) /* Enable . disable nonblocking mode */ 00286 #define SL_SO_SECMETHOD (25) /* security metohd */ 00287 #define SL_SO_SECURE_MASK (26) /* security mask */ 00288 #define SL_SO_SECURE_FILES (27) /* security files */ 00289 #define SL_SO_CHANGE_CHANNEL (28) /* This option is available only when transceiver started */ 00290 #define SL_SO_SECURE_FILES_PRIVATE_KEY_FILE_NAME (30) /* This option used to configue secure file */ 00291 #define SL_SO_SECURE_FILES_CERTIFICATE_FILE_NAME (31) /* This option used to configue secure file */ 00292 #define SL_SO_SECURE_FILES_CA_FILE_NAME (32) /* This option used to configue secure file */ 00293 #define SL_SO_SECURE_FILES_DH_KEY_FILE_NAME (33) /* This option used to configue secure file */ 00294 #define SO_SECURE_DOMAIN_NAME_VERIFICATION (35) 00295 #define SECURE_MAX_DOMAIN_LENGTH (64) 00296 00297 #define SL_IP_MULTICAST_IF (60) /* Specify outgoing multicast interface */ 00298 #define SL_IP_MULTICAST_TTL (61) /* Specify the TTL value to use for outgoing multicast packet. */ 00299 #define SL_IP_ADD_MEMBERSHIP (65) /* Join IPv4 multicast membership */ 00300 #define SL_IP_DROP_MEMBERSHIP (66) /* Leave IPv4 multicast membership */ 00301 #define SL_IP_HDRINCL (67) /* Raw socket IPv4 header included. */ 00302 #define SL_IP_RAW_RX_NO_HEADER (68) /* Proprietary socket option that does not includeIPv4/IPv6 header (and extension headers) on received raw sockets*/ 00303 #define SL_IP_RAW_IPV6_HDRINCL (69) /* Transmitted buffer over IPv6 socket contains IPv6 header. */ 00304 00305 #define SL_SO_PHY_RATE (100) /* WLAN Transmit rate */ 00306 #define SL_SO_PHY_TX_POWER (101) /* TX Power level */ 00307 #define SL_SO_PHY_NUM_FRAMES_TO_TX (102) /* Number of frames to transmit */ 00308 #define SL_SO_PHY_PREAMBLE (103) /* Preamble for transmission */ 00309 00310 #define SL_SO_SEC_METHOD_SSLV3 (0) /* security metohd SSL v3*/ 00311 #define SL_SO_SEC_METHOD_TLSV1 (1) /* security metohd TLS v1*/ 00312 #define SL_SO_SEC_METHOD_TLSV1_1 (2) /* security metohd TLS v1_1*/ 00313 #define SL_SO_SEC_METHOD_TLSV1_2 (3) /* security metohd TLS v1_2*/ 00314 #define SL_SO_SEC_METHOD_SSLv3_TLSV1_2 (4) /* use highest possible version from SSLv3 - TLS 1.2*/ 00315 #define SL_SO_SEC_METHOD_DLSV1 (5) /* security metohd DTL v1 */ 00316 00317 #define SL_SEC_MASK_SSL_RSA_WITH_RC4_128_SHA (1 << 0) 00318 #define SL_SEC_MASK_SSL_RSA_WITH_RC4_128_MD5 (1 << 1) 00319 #define SL_SEC_MASK_TLS_RSA_WITH_AES_256_CBC_SHA (1 << 2) 00320 #define SL_SEC_MASK_TLS_DHE_RSA_WITH_AES_256_CBC_SHA (1 << 3) 00321 #define SL_SEC_MASK_TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA (1 << 4) 00322 #define SL_SEC_MASK_TLS_ECDHE_RSA_WITH_RC4_128_SHA (1 << 5) 00323 #define SL_SEC_MASK_TLS_RSA_WITH_AES_128_CBC_SHA256 (1 << 6) 00324 #define SL_SEC_MASK_TLS_RSA_WITH_AES_256_CBC_SHA256 (1 << 7) 00325 #define SL_SEC_MASK_TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA256 (1 << 8) 00326 #define SL_SEC_MASK_TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA256 (1 << 9) 00327 00328 00329 #define SL_SEC_MASK_SECURE_DEFAULT ((SL_SEC_MASK_TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA256 << 1) - 1) 00330 00331 #define SL_MSG_DONTWAIT (0x00000008) /* Nonblocking IO */ 00332 00333 /* AP DHCP Server - IP Release reason code */ 00334 #define SL_IP_LEASE_PEER_RELEASE (0) 00335 #define SL_IP_LEASE_PEER_DECLINE (1) 00336 #define SL_IP_LEASE_EXPIRED (2) 00337 00338 /* possible types when receiving SL_SOCKET_ASYNC_EVENT*/ 00339 #define SSL_ACCEPT (0) /* accept failed due to ssl issue ( tcp pass) */ 00340 #define RX_FRAGMENTATION_TOO_BIG (1) /* connection less mode, rx packet fragmentation > 16K, packet is being released */ 00341 #define OTHER_SIDE_CLOSE_SSL_DATA_NOT_ENCRYPTED (2) /* remote side down from secure to unsecure */ 00342 00343 00344 00345 #ifdef SL_INC_STD_BSD_API_NAMING 00346 00347 #define FD_SETSIZE SL_FD_SETSIZE 00348 00349 #define SOCK_STREAM SL_SOCK_STREAM 00350 #define SOCK_DGRAM SL_SOCK_DGRAM 00351 #define SOCK_RAW SL_SOCK_RAW 00352 #define IPPROTO_TCP SL_IPPROTO_TCP 00353 #define IPPROTO_UDP SL_IPPROTO_UDP 00354 #define IPPROTO_RAW SL_IPPROTO_RAW 00355 00356 #define AF_INET SL_AF_INET 00357 #define AF_INET6 SL_AF_INET6 00358 #define AF_INET6_EUI_48 SL_AF_INET6_EUI_48 00359 #define AF_RF SL_AF_RF 00360 #define AF_PACKET SL_AF_PACKET 00361 00362 #define PF_INET SL_PF_INET 00363 #define PF_INET6 SL_PF_INET6 00364 00365 #define INADDR_ANY SL_INADDR_ANY 00366 #define ERROR SL_SOC_ERROR 00367 #define INEXE SL_INEXE 00368 #define EBADF SL_EBADF 00369 #define ENSOCK SL_ENSOCK 00370 #define EAGAIN SL_EAGAIN 00371 #define EWOULDBLOCK SL_EWOULDBLOCK 00372 #define ENOMEM SL_ENOMEM 00373 #define EACCES SL_EACCES 00374 #define EFAULT SL_EFAULT 00375 #define EINVAL SL_EINVAL 00376 #define EDESTADDRREQ SL_EDESTADDRREQ 00377 #define EPROTOTYPE SL_EPROTOTYPE 00378 #define ENOPROTOOPT SL_ENOPROTOOPT 00379 #define EPROTONOSUPPORT SL_EPROTONOSUPPORT 00380 #define ESOCKTNOSUPPORT SL_ESOCKTNOSUPPORT 00381 #define EOPNOTSUPP SL_EOPNOTSUPP 00382 #define EAFNOSUPPORT SL_EAFNOSUPPORT 00383 #define EADDRINUSE SL_EADDRINUSE 00384 #define EADDRNOTAVAIL SL_EADDRNOTAVAIL 00385 #define ENETUNREACH SL_ENETUNREACH 00386 #define ENOBUFS SL_ENOBUFS 00387 #define EOBUFF SL_EOBUFF 00388 #define EISCONN SL_EISCONN 00389 #define ENOTCONN SL_ENOTCONN 00390 #define ETIMEDOUT SL_ETIMEDOUT 00391 #define ECONNREFUSED SL_ECONNREFUSED 00392 00393 #define SOL_SOCKET SL_SOL_SOCKET 00394 #define IPPROTO_IP SL_IPPROTO_IP 00395 #define SO_KEEPALIVE SL_SO_KEEPALIVE 00396 00397 #define SO_RCVTIMEO SL_SO_RCVTIMEO 00398 #define SO_NONBLOCKING SL_SO_NONBLOCKING 00399 00400 #define IP_MULTICAST_IF SL_IP_MULTICAST_IF 00401 #define IP_MULTICAST_TTL SL_IP_MULTICAST_TTL 00402 #define IP_ADD_MEMBERSHIP SL_IP_ADD_MEMBERSHIP 00403 #define IP_DROP_MEMBERSHIP SL_IP_DROP_MEMBERSHIP 00404 00405 #define socklen_t SlSocklen_t 00406 #define timeval SlTimeval_t 00407 #define sockaddr SlSockAddr_t 00408 #define in6_addr SlIn6Addr_t 00409 #define sockaddr_in6 SlSockAddrIn6_t 00410 #define in_addr SlInAddr_t 00411 #define sockaddr_in SlSockAddrIn_t 00412 00413 #define MSG_DONTWAIT SL_MSG_DONTWAIT 00414 00415 #define FD_SET SL_FD_SET 00416 #define FD_CLR SL_FD_CLR 00417 #define FD_ISSET SL_FD_ISSET 00418 #define FD_ZERO SL_FD_ZERO 00419 #define fd_set SlFdSet_t 00420 00421 /*#define socket sl_Socket 00422 #define close sl_Close 00423 #define accept sl_Accept 00424 #define bind sl_Bind 00425 #define listen sl_Listen 00426 #define connect sl_Connect 00427 #define select sl_Select 00428 #define setsockopt sl_SetSockOpt 00429 #define getsockopt sl_GetSockOpt 00430 #define recv sl_Recv 00431 #define recvfrom sl_RecvFrom 00432 #define write sl_Write 00433 #define send sl_Send 00434 #define sendto sl_SendTo 00435 #define gethostbyname sl_NetAppDnsGetHostByName 00436 #define htonl sl_Htonl 00437 #define ntohl sl_Ntohl 00438 #define htons sl_Htons 00439 #define ntohs sl_Ntohs*/ 00440 #endif 00441 00442 /*****************************************************************************/ 00443 /* Structure/Enum declarations */ 00444 /*****************************************************************************/ 00445 00446 /* Internet address */ 00447 typedef struct SlInAddr_t 00448 { 00449 #ifndef s_addr 00450 _u32 s_addr; /* Internet address 32 bits */ 00451 #else 00452 union S_un { 00453 struct { _u8 s_b1,s_b2,s_b3,s_b4; } S_un_b; 00454 struct { _u8 s_w1,s_w2; } S_un_w; 00455 _u32 S_addr; 00456 } S_un; 00457 #endif 00458 }SlInAddr_t; 00459 00460 00461 /* sockopt */ 00462 typedef struct 00463 { 00464 _u32 KeepaliveEnabled; /* 0 = disabled;1 = enabled; default = 1*/ 00465 }SlSockKeepalive_t; 00466 00467 typedef struct 00468 { 00469 _u32 ReuseaddrEnabled; /* 0 = disabled; 1 = enabled; default = 1*/ 00470 }SlSockReuseaddr_t; 00471 00472 typedef struct 00473 { 00474 _u32 Winsize; /* receive window size for tcp sockets */ 00475 }SlSockWinsize_t; 00476 00477 typedef struct 00478 { 00479 _u32 NonblockingEnabled;/* 0 = disabled;1 = enabled;default = 1*/ 00480 }SlSockNonblocking_t; 00481 00482 00483 typedef struct 00484 { 00485 _u8 sd; 00486 _u8 type; 00487 _i16 val; 00488 _u8* pExtraInfo; 00489 } SlSocketAsyncEvent_t; 00490 00491 typedef struct 00492 { 00493 _i16 status; 00494 _u8 sd; 00495 _u8 padding; 00496 } SlSockTxFailEventData_t; 00497 00498 00499 typedef union 00500 { 00501 SlSockTxFailEventData_t SockTxFailData; 00502 SlSocketAsyncEvent_t SockAsyncData; 00503 } SlSockEventData_u; 00504 00505 00506 typedef struct 00507 { 00508 _u32 Event; 00509 SlSockEventData_u socketAsyncEvent; 00510 } SlSockEvent_t; 00511 00512 00513 00514 00515 00516 00517 typedef struct 00518 { 00519 _u32 secureMask; 00520 } SlSockSecureMask; 00521 00522 typedef struct 00523 { 00524 _u8 secureMethod; 00525 } SlSockSecureMethod; 00526 00527 typedef enum 00528 { 00529 SL_BSD_SECURED_PRIVATE_KEY_IDX = 0, 00530 SL_BSD_SECURED_CERTIFICATE_IDX, 00531 SL_BSD_SECURED_CA_IDX, 00532 SL_BSD_SECURED_DH_IDX 00533 }slBsd_secureSocketFilesIndex_e; 00534 00535 typedef struct 00536 { 00537 SlInAddr_t imr_multiaddr; /* The IPv4 multicast address to join */ 00538 SlInAddr_t imr_interface; /* The interface to use for this group */ 00539 } SlSockIpMreq; 00540 00541 00542 /* sockopt */ 00543 typedef _u32 SlTime_t; 00544 typedef _u32 SlSuseconds_t; 00545 00546 typedef struct SlTimeval_t 00547 { 00548 SlTime_t tv_sec; /* Seconds */ 00549 SlSuseconds_t tv_usec; /* Microseconds */ 00550 }SlTimeval_t; 00551 00552 typedef _u16 SlSocklen_t; 00553 00554 /* IpV4 socket address */ 00555 typedef struct SlSockAddr_t 00556 { 00557 _u16 sa_family; /* Address family (e.g. , AF_INET) */ 00558 _u8 sa_data[14]; /* Protocol- specific address information*/ 00559 }SlSockAddr_t; 00560 00561 00562 /* IpV6 or Ipv6 EUI64 */ 00563 typedef struct SlIn6Addr_t 00564 { 00565 union 00566 { 00567 _u8 _S6_u8[16]; 00568 _u32 _S6_u32[4]; 00569 } _S6_un; 00570 }SlIn6Addr_t; 00571 00572 typedef struct SlSockAddrIn6_t 00573 { 00574 _u16 sin6_family; /* AF_INET6 || AF_INET6_EUI_48*/ 00575 _u16 sin6_port; /* Transport layer port. */ 00576 _u32 sin6_flowinfo; /* IPv6 flow information. */ 00577 SlIn6Addr_t sin6_addr; /* IPv6 address. */ 00578 _u32 sin6_scope_id; /* set of interfaces for a scope. */ 00579 }SlSockAddrIn6_t; 00580 00581 /* Socket address, Internet style. */ 00582 00583 typedef struct SlSockAddrIn_t 00584 { 00585 _u16 sin_family; /* Internet Protocol (AF_INET). */ 00586 _u16 sin_port; /* Address port (16 bits). */ 00587 SlInAddr_t sin_addr; /* Internet address (32 bits). */ 00588 _i8 sin_zero[8]; /* Not used. */ 00589 }SlSockAddrIn_t; 00590 00591 typedef struct 00592 { 00593 _u32 ip; 00594 _u32 gateway; 00595 _u32 dns; 00596 }SlIpV4AcquiredAsync_t; 00597 00598 typedef struct 00599 { 00600 _u32 type; 00601 _u32 ip[4]; 00602 _u32 gateway[4]; 00603 _u32 dns[4]; 00604 }SlIpV6AcquiredAsync_t; 00605 00606 typedef struct 00607 { 00608 _u32 ip_address; 00609 _u32 lease_time; 00610 _u8 mac[6]; 00611 _u16 padding; 00612 }SlIpLeasedAsync_t; 00613 00614 typedef struct 00615 { 00616 _u32 ip_address; 00617 _u8 mac[6]; 00618 _u16 reason; 00619 }SlIpReleasedAsync_t; 00620 00621 00622 typedef union 00623 { 00624 SlIpV4AcquiredAsync_t ipAcquiredV4; /*SL_NETAPP_IPV4_IPACQUIRED_EVENT*/ 00625 SlIpV6AcquiredAsync_t ipAcquiredV6; /*SL_NETAPP_IPV6_IPACQUIRED_EVENT*/ 00626 _u32 sd; /*SL_SOCKET_TX_FAILED_EVENT*/ 00627 SlIpLeasedAsync_t ipLeased; /* SL_NETAPP_IP_LEASED_EVENT */ 00628 SlIpReleasedAsync_t ipReleased; /* SL_NETAPP_IP_RELEASED_EVENT */ 00629 } SlNetAppEventData_u; 00630 00631 typedef struct 00632 { 00633 _u32 Event; 00634 SlNetAppEventData_u EventData; 00635 }SlNetAppEvent_t; 00636 00637 00638 typedef struct sock_secureFiles 00639 { 00640 _u8 secureFiles[4]; 00641 }SlSockSecureFiles_t; 00642 00643 00644 typedef struct SlFdSet_t /* The select socket array manager */ 00645 { 00646 _u32 fd_array[(SL_FD_SETSIZE + (_u8)31)/(_u8)32]; /* Bit map of SOCKET Descriptors */ 00647 } SlFdSet_t; 00648 00649 typedef struct 00650 { 00651 _u8 rate; /* Recevied Rate */ 00652 _u8 channel; /* The received channel*/ 00653 _i8 rssi; /* The computed RSSI value in db of current frame */ 00654 _u8 padding; /* pad to align to 32 bits */ 00655 _u32 timestamp; /* Timestamp in microseconds, */ 00656 }SlTransceiverRxOverHead_t; 00657 00658 00659 00660 /*****************************************************************************/ 00661 /* Function prototypes */ 00662 /*****************************************************************************/ 00663 00664 /*! 00665 00666 \brief create an endpoint for communication 00667 00668 The socket function creates a new socket of a certain socket type, identified 00669 by an integer number, and allocates system resources to it. 00670 This function is called by the application layer to obtain a socket handle. 00671 00672 \param[in] domain specifies the protocol family of the created socket. 00673 For example: 00674 AF_INET for network protocol IPv4 00675 AF_RF for starting transceiver mode. Notes: 00676 - sending and receiving any packet overriding 802.11 header 00677 - for optimized power consumption the socket will be started in TX 00678 only mode until receive command is activated 00679 AF_INET6 for IPv6 00680 00681 00682 \param[in] type specifies the communication semantic, one of: 00683 SOCK_STREAM (reliable stream-oriented service or Stream Sockets) 00684 SOCK_DGRAM (datagram service or Datagram Sockets) 00685 SOCK_RAW (raw protocols atop the network layer) 00686 when used with AF_RF: 00687 SOCK_DGRAM - L2 socket 00688 SOCK_RAW - L1 socket - bypass WLAN CCA (Clear Channel Assessment) 00689 00690 \param[in] protocol specifies a particular transport to be used with 00691 the socket. 00692 The most common are IPPROTO_TCP, IPPROTO_SCTP, IPPROTO_UDP, 00693 IPPROTO_DCCP. 00694 The value 0 may be used to select a default 00695 protocol from the selected domain and type 00696 00697 00698 \return On success, socket handle that is used for consequent socket operations. 00699 A successful return code should be a positive number (int16) 00700 On error, a negative (int16) value will be returned specifying the error code. 00701 SL_EAFNOSUPPORT - illegal domain parameter 00702 SL_EPROTOTYPE - illegal type parameter 00703 SL_EACCES - permission denied 00704 SL_ENSOCK - exceeded maximal number of socket 00705 SL_ENOMEM - memory allocation error 00706 SL_EINVAL - error in socket configuration 00707 SL_EPROTONOSUPPORT - illegal protocol parameter 00708 SL_EOPNOTSUPP - illegal combination of protocol and type parameters 00709 00710 00711 \sa sl_Close 00712 \note belongs to \ref basic_api 00713 \warning 00714 */ 00715 #if _SL_INCLUDE_FUNC(sl_Socket) 00716 _i16 sl_Socket(_i16 Domain, _i16 Type, _i16 Protocol); 00717 #endif 00718 00719 /*! 00720 \brief gracefully close socket 00721 00722 This function causes the system to release resources allocated to a socket. \n 00723 In case of TCP, the connection is terminated. 00724 00725 \param[in] sd socket handle (received in sl_Socket) 00726 00727 \return On success, zero is returned. 00728 On error, a negative number is returned. 00729 00730 \sa sl_Socket 00731 \note belongs to \ref ext_api 00732 \warning 00733 */ 00734 #if _SL_INCLUDE_FUNC(sl_Close) 00735 _i16 sl_Close(_i16 sd); 00736 #endif 00737 00738 /*! 00739 \brief Accept a connection on a socket 00740 00741 This function is used with connection-based socket types (SOCK_STREAM). 00742 It extracts the first connection request on the queue of pending 00743 connections, creates a new connected socket, and returns a new file 00744 descriptor referring to that socket. 00745 The newly created socket is not in the listening state. The 00746 original socket sd is unaffected by this call. 00747 The argument sd is a socket that has been created with 00748 sl_Socket(), bound to a local address with sl_Bind(), and is 00749 listening for connections after a sl_Listen(). The argument \b 00750 \e addr is a pointer to a sockaddr structure. This structure 00751 is filled in with the address of the peer socket, as known to 00752 the communications layer. The exact format of the address 00753 returned addr is determined by the socket's address family. 00754 The \b \e addrlen argument is a value-result argument: it 00755 should initially contain the size of the structure pointed to 00756 by addr, on return it will contain the actual length (in 00757 bytes) of the address returned. 00758 00759 \param[in] sd socket descriptor (handle) 00760 \param[out] addr the argument addr is a pointer 00761 to a sockaddr structure. This 00762 structure is filled in with the 00763 address of the peer socket, as 00764 known to the communications 00765 layer. The exact format of the 00766 address returned addr is 00767 determined by the socket's 00768 address\n 00769 sockaddr:\n - code for the 00770 address format. On this version 00771 only AF_INET is supported.\n - 00772 socket address, the length 00773 depends on the code format 00774 \param[out] addrlen the addrlen argument is a value-result 00775 argument: it should initially contain the 00776 size of the structure pointed to by addr 00777 00778 \return On success, a socket handle. 00779 On a non-blocking accept a possible negative value is SL_EAGAIN. 00780 On failure, negative value. 00781 SL_POOL_IS_EMPTY may be return in case there are no resources in the system 00782 In this case try again later or increase MAX_CONCURRENT_ACTIONS 00783 00784 \sa sl_Socket sl_Bind sl_Listen 00785 \note belongs to \ref server_side 00786 \warning 00787 */ 00788 #if _SL_INCLUDE_FUNC(sl_Accept) 00789 _i16 sl_Accept(_i16 sd, SlSockAddr_t *addr, SlSocklen_t *addrlen); 00790 #endif 00791 00792 /*! 00793 \brief assign a name to a socket 00794 00795 This function gives the socket the local address addr. 00796 addr is addrlen bytes long. Traditionally, this is called 00797 When a socket is created with socket, it exists in a name 00798 space (address family) but has no name assigned. 00799 It is necessary to assign a local address before a SOCK_STREAM 00800 socket may receive connections. 00801 00802 \param[in] sd socket descriptor (handle) 00803 \param[in] addr specifies the destination 00804 addrs\n sockaddr:\n - code for 00805 the address format. On this 00806 version only AF_INET is 00807 supported.\n - socket address, 00808 the length depends on the code 00809 format 00810 \param[in] addrlen contains the size of the structure pointed to by addr 00811 00812 \return On success, zero is returned. On error, a negative error code is returned. 00813 00814 \sa sl_Socket sl_Accept sl_Listen 00815 \note belongs to \ref basic_api 00816 \warning 00817 */ 00818 #if _SL_INCLUDE_FUNC(sl_Bind) 00819 _i16 sl_Bind(_i16 sd, const SlSockAddr_t *addr, _i16 addrlen); 00820 #endif 00821 00822 /*! 00823 \brief listen for connections on a socket 00824 00825 The willingness to accept incoming connections and a queue 00826 limit for incoming connections are specified with listen(), 00827 and then the connections are accepted with accept. 00828 The listen() call applies only to sockets of type SOCK_STREAM 00829 The backlog parameter defines the maximum length the queue of 00830 pending connections may grow to. 00831 00832 \param[in] sd socket descriptor (handle) 00833 \param[in] backlog specifies the listen queue depth. 00834 00835 00836 \return On success, zero is returned. On error, a negative error code is returned. 00837 00838 \sa sl_Socket sl_Accept sl_Bind 00839 \note belongs to \ref server_side 00840 \warning 00841 */ 00842 #if _SL_INCLUDE_FUNC(sl_Listen) 00843 _i16 sl_Listen(_i16 sd, _i16 backlog); 00844 #endif 00845 00846 /*! 00847 \brief Initiate a connection on a socket 00848 00849 Function connects the socket referred to by the socket 00850 descriptor sd, to the address specified by addr. The addrlen 00851 argument specifies the size of addr. The format of the 00852 address in addr is determined by the address space of the 00853 socket. If it is of type SOCK_DGRAM, this call specifies the 00854 peer with which the socket is to be associated; this address 00855 is that to which datagrams are to be sent, and the only 00856 address from which datagrams are to be received. If the 00857 socket is of type SOCK_STREAM, this call attempts to make a 00858 connection to another socket. The other socket is specified 00859 by address, which is an address in the communications space 00860 of the socket. 00861 00862 00863 \param[in] sd socket descriptor (handle) 00864 \param[in] addr specifies the destination addr\n 00865 sockaddr:\n - code for the 00866 address format. On this version 00867 only AF_INET is supported.\n - 00868 socket address, the length 00869 depends on the code format 00870 00871 \param[in] addrlen contains the size of the structure pointed 00872 to by addr 00873 00874 \return On success, a socket handle. 00875 On a non-blocking connect a possible negative value is SL_EALREADY. 00876 On failure, negative value. 00877 SL_POOL_IS_EMPTY may be return in case there are no resources in the system 00878 In this case try again later or increase MAX_CONCURRENT_ACTIONS 00879 00880 \sa sl_Socket 00881 \note belongs to \ref client_side 00882 \warning 00883 */ 00884 #if _SL_INCLUDE_FUNC(sl_Connect) 00885 _i16 sl_Connect(_i16 sd, const SlSockAddr_t *addr, _i16 addrlen); 00886 #endif 00887 00888 /*! 00889 \brief Monitor socket activity 00890 00891 Select allow a program to monitor multiple file descriptors, 00892 waiting until one or more of the file descriptors become 00893 "ready" for some class of I/O operation 00894 00895 00896 \param[in] nfds the highest-numbered file descriptor in any of the 00897 three sets, plus 1. 00898 \param[out] readsds socket descriptors list for read monitoring and accept monitoring 00899 \param[out] writesds socket descriptors list for connect monitoring only, write monitoring is not supported, non blocking connect is supported 00900 \param[out] exceptsds socket descriptors list for exception monitoring, not supported. 00901 \param[in] timeout is an upper bound on the amount of time elapsed 00902 before select() returns. Null or above 0xffff seconds means 00903 infinity timeout. The minimum timeout is 10 milliseconds, 00904 less than 10 milliseconds will be set automatically to 10 milliseconds. 00905 Max microseconds supported is 0xfffc00. 00906 00907 \return On success, select() returns the number of 00908 file descriptors contained in the three returned 00909 descriptor sets (that is, the total number of bits that 00910 are set in readfds, writefds, exceptfds) which may be 00911 zero if the timeout expires before anything interesting 00912 happens. On error, a negative value is returned. 00913 readsds - return the sockets on which Read request will 00914 return without delay with valid data. 00915 writesds - return the sockets on which Write request 00916 will return without delay. 00917 exceptsds - return the sockets closed recently. 00918 SL_POOL_IS_EMPTY may be return in case there are no resources in the system 00919 In this case try again later or increase MAX_CONCURRENT_ACTIONS 00920 00921 \sa sl_Socket 00922 \note If the timeout value set to less than 5ms it will automatically set 00923 to 5ms to prevent overload of the system 00924 belongs to \ref basic_api 00925 00926 Only one sl_Select can be handled at a time. 00927 Calling this API while the same command is called from another thread, may result 00928 in one of the two scenarios: 00929 1. The command will wait (internal) until the previous command finish, and then be executed. 00930 2. There are not enough resources and SL_POOL_IS_EMPTY error will return. 00931 In this case, MAX_CONCURRENT_ACTIONS can be increased (result in memory increase) or try 00932 again later to issue the command. 00933 00934 \warning 00935 */ 00936 #if _SL_INCLUDE_FUNC(sl_Select) 00937 _i16 sl_Select(_i16 nfds, SlFdSet_t *readsds, SlFdSet_t *writesds, SlFdSet_t *exceptsds, struct SlTimeval_t *timeout); 00938 00939 00940 /*! 00941 \brief Select's SlFdSet_t SET function 00942 00943 Sets current socket descriptor on SlFdSet_t container 00944 */ 00945 void SL_FD_SET(_i16 fd, SlFdSet_t *fdset); 00946 00947 /*! 00948 \brief Select's SlFdSet_t CLR function 00949 00950 Clears current socket descriptor on SlFdSet_t container 00951 */ 00952 void SL_FD_CLR(_i16 fd, SlFdSet_t *fdset); 00953 00954 00955 /*! 00956 \brief Select's SlFdSet_t ISSET function 00957 00958 Checks if current socket descriptor is set (TRUE/FALSE) 00959 00960 \return Returns TRUE if set, FALSE if unset 00961 00962 */ 00963 _i16 SL_FD_ISSET(_i16 fd, SlFdSet_t *fdset); 00964 00965 /*! 00966 \brief Select's SlFdSet_t ZERO function 00967 00968 Clears all socket descriptors from SlFdSet_t 00969 */ 00970 void SL_FD_ZERO(SlFdSet_t *fdset); 00971 00972 00973 00974 #endif 00975 00976 /*! 00977 \brief set socket options 00978 00979 This function manipulate the options associated with a socket. 00980 Options may exist at multiple protocol levels; they are always 00981 present at the uppermost socket level. 00982 00983 When manipulating socket options the level at which the option resides 00984 and the name of the option must be specified. To manipulate options at 00985 the socket level, level is specified as SOL_SOCKET. To manipulate 00986 options at any other level the protocol number of the appropriate proto- 00987 col controlling the option is supplied. For example, to indicate that an 00988 option is to be interpreted by the TCP protocol, level should be set to 00989 the protocol number of TCP; 00990 00991 The parameters optval and optlen are used to access optval - 00992 ues for setsockopt(). For getsockopt() they identify a 00993 buffer in which the value for the requested option(s) are to 00994 be returned. For getsockopt(), optlen is a value-result 00995 parameter, initially containing the size of the buffer 00996 pointed to by option_value, and modified on return to 00997 indicate the actual size of the value returned. If no option 00998 value is to be supplied or returned, option_value may be 00999 NULL. 01000 01001 \param[in] sd socket handle 01002 \param[in] level defines the protocol level for this option 01003 - <b>SL_SOL_SOCKET</b> Socket level configurations (L4, transport layer) 01004 - <b>SL_IPPROTO_IP</b> IP level configurations (L3, network layer) 01005 - <b>SL_SOL_PHY_OPT</b> Link level configurations (L2, link layer) 01006 \param[in] optname defines the option name to interrogate 01007 - <b>SL_SOL_SOCKET</b> 01008 - <b>SL_SO_KEEPALIVE</b> \n 01009 Enable/Disable periodic keep alive. 01010 Keeps TCP connections active by enabling the periodic transmission of messages \n 01011 Timeout is 5 minutes.\n 01012 Default: Enabled \n 01013 This options takes SlSockKeepalive_t struct as parameter 01014 - <b>SL_SO_RCVTIMEO</b> \n 01015 Sets the timeout value that specifies the maximum amount of time an input function waits until it completes. \n 01016 Default: No timeout \n 01017 This options takes SlTimeval_t struct as parameter 01018 - <b>SL_SO_RCVBUF</b> \n 01019 Sets tcp max recv window size. \n 01020 This options takes SlSockWinsize_t struct as parameter 01021 - <b>SL_SO_NONBLOCKING</b> \n 01022 Sets socket to non-blocking operation Impacts: connect, accept, send, sendto, recv and recvfrom. \n 01023 Default: Blocking. 01024 This options takes SlSockNonblocking_t struct as parameter 01025 - <b>SL_SO_SECMETHOD</b> \n 01026 Sets method to tcp secured socket (SL_SEC_SOCKET) \n 01027 Default: SL_SO_SEC_METHOD_SSLv3_TLSV1_2 \n 01028 This options takes SlSockSecureMethod struct as parameter 01029 - <b>SL_SO_SEC_MASK</b> \n 01030 Sets specific cipher to tcp secured socket (SL_SEC_SOCKET) \n 01031 Default: "Best" cipher suitable to method \n 01032 This options takes SlSockSecureMask struct as parameter 01033 - <b>SL_SO_SECURE_FILES_CA_FILE_NAME</b> \n 01034 Map secured socket to CA file by name \n 01035 This options takes <b>_u8</b> buffer as parameter 01036 - <b>SL_SO_SECURE_FILES_PRIVATE_KEY_FILE_NAME</b> \n 01037 Map secured socket to private key by name \n 01038 This options takes <b>_u8</b> buffer as parameter 01039 - <b>SL_SO_SECURE_FILES_CERTIFICATE_FILE_NAME</b> \n 01040 Map secured socket to certificate file by name \n 01041 This options takes <b>_u8</b> buffer as parameter 01042 - <b>SL_SO_SECURE_FILES_DH_KEY_FILE_NAME</b> \n 01043 Map secured socket to Diffie Hellman file by name \n 01044 This options takes <b>_u8</b> buffer as parameter 01045 - <b>SL_SO_CHANGE_CHANNEL</b> \n 01046 Sets channel in transceiver mode. 01047 This options takes <b>_u32</b> as channel number parameter 01048 - <b>SL_IPPROTO_IP</b> 01049 - <b>SL_IP_MULTICAST_TTL</b> \n 01050 Set the time-to-live value of outgoing multicast packets for this socket. \n 01051 This options takes <b>_u8</b> as parameter 01052 - <b>SL_IP_ADD_MEMBERSHIP</b> \n 01053 UDP socket, Join a multicast group. \n 01054 This options takes SlSockIpMreq struct as parameter 01055 - <b>SL_IP_DROP_MEMBERSHIP</b> \n 01056 UDP socket, Leave a multicast group \n 01057 This options takes SlSockIpMreq struct as parameter 01058 - <b>SL_IP_RAW_RX_NO_HEADER</b> \n 01059 Raw socket remove IP header from received data. \n 01060 Default: data includes ip header \n 01061 This options takes <b>_u32</b> as parameter 01062 - <b>SL_IP_HDRINCL</b> \n 01063 RAW socket only, the IPv4 layer generates an IP header when sending a packet unless \n 01064 the IP_HDRINCL socket option is enabled on the socket. \n 01065 When it is enabled, the packet must contain an IP header. \n 01066 Default: disabled, IPv4 header generated by Network Stack \n 01067 This options takes <b>_u32</b> as parameter 01068 - <b>SL_IP_RAW_IPV6_HDRINCL</b> (inactive) \n 01069 RAW socket only, the IPv6 layer generates an IP header when sending a packet unless \n 01070 the IP_HDRINCL socket option is enabled on the socket. When it is enabled, the packet must contain an IP header \n 01071 Default: disabled, IPv4 header generated by Network Stack \n 01072 This options takes <b>_u32</b> as parameter 01073 - <b>SL_SOL_PHY_OPT</b> 01074 - <b>SL_SO_PHY_RATE</b> \n 01075 RAW socket, set WLAN PHY transmit rate \n 01076 The values are based on RateIndex_e \n 01077 This options takes <b>_u32</b> as parameter 01078 - <b>SL_SO_PHY_TX_POWER</b> \n 01079 RAW socket, set WLAN PHY TX power \n 01080 Valid rage is 1-15 \n 01081 This options takes <b>_u32</b> as parameter 01082 - <b>SL_SO_PHY_NUM_FRAMES_TO_TX</b> \n 01083 RAW socket, set number of frames to transmit in transceiver mode. 01084 Default: 1 packet 01085 This options takes <b>_u32</b> as parameter 01086 - <b>SL_SO_PHY_PREAMBLE</b> \n 01087 RAW socket, set WLAN PHY preamble for Long/Short\n 01088 This options takes <b>_u32</b> as parameter 01089 01090 \param[in] optval specifies a value for the option 01091 \param[in] optlen specifies the length of the 01092 option value 01093 01094 \return On success, zero is returned. 01095 On error, a negative value is returned. 01096 \sa sl_getsockopt 01097 \note belongs to \ref basic_api 01098 \warning 01099 \par Examples: 01100 \par 01101 <b> SL_SO_KEEPALIVE: </b>(disable Keepalive) 01102 \code 01103 SlSockKeepalive_t enableOption; 01104 enableOption.KeepaliveEnabled = 0; 01105 sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_KEEPALIVE, (_u8 *)&enableOption,sizeof(enableOption)); 01106 \endcode 01107 \par 01108 <b> SL_SO_RCVTIMEO: </b> 01109 \code 01110 struct SlTimeval_t timeVal; 01111 timeVal.tv_sec = 1; // Seconds 01112 timeVal.tv_usec = 0; // Microseconds. 10000 microseconds resolution 01113 sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_RCVTIMEO, (_u8 *)&timeVal, sizeof(timeVal)); // Enable receive timeout 01114 \endcode 01115 \par 01116 <b> SL_SO_RCVBUF: </b> 01117 \code 01118 SlSockWinsize_t size; 01119 size.Winsize = 3000; // bytes 01120 sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_RCVBUF, (_u8 *)&size, sizeof(size)); 01121 \endcode 01122 \par 01123 <b> SL_SO_NONBLOCKING: </b> 01124 \code 01125 SlSockNonblocking_t enableOption; 01126 enableOption.NonblockingEnabled = 1; 01127 sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_NONBLOCKING, (_u8 *)&enableOption,sizeof(enableOption)); // Enable/disable nonblocking mode 01128 \endcode 01129 \par 01130 <b> SL_SO_SECMETHOD:</b> 01131 \code 01132 SlSockSecureMethod method; 01133 method.secureMethod = SL_SO_SEC_METHOD_SSLV3; // security method we want to use 01134 SockID = sl_Socket(SL_AF_INET,SL_SOCK_STREAM, SL_SEC_SOCKET); 01135 sl_SetSockOpt(SockID, SL_SOL_SOCKET, SL_SO_SECMETHOD, (_u8 *)&method, sizeof(method)); 01136 \endcode 01137 \par 01138 <b> SL_SO_SECURE_MASK:</b> 01139 \code 01140 SlSockSecureMask cipher; 01141 cipher.secureMask = SL_SEC_MASK_SSL_RSA_WITH_RC4_128_SHA; // cipher type 01142 SockID = sl_Socket(SL_AF_INET,SL_SOCK_STREAM, SL_SEC_SOCKET); 01143 sl_SetSockOpt(SockID, SL_SOL_SOCKET, SL_SO_SEC_MASK,(_u8 *)&cipher, sizeof(cipher)); 01144 \endcode 01145 \par 01146 <b> SL_SO_SECURE_FILES_CA_FILE_NAME:</b> 01147 \code 01148 sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_SECURE_FILES_CA_FILE_NAME,"exuifaxCaCert.der",strlen("exuifaxCaCert.der")); 01149 \endcode 01150 01151 \par 01152 <b> SL_SO_SECURE_FILES_PRIVATE_KEY_FILE_NAME:</b> 01153 \code 01154 sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_SECURE_FILES_PRIVATE_KEY_FILE_NAME,"myPrivateKey.der",strlen("myPrivateKey.der")); 01155 \endcode 01156 01157 \par 01158 <b> SL_SO_SECURE_FILES_CERTIFICATE_FILE_NAME:</b> 01159 \code 01160 sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_SECURE_FILES_CERTIFICATE_FILE_NAME,"myCertificate.der",strlen("myCertificate.der")); 01161 \endcode 01162 01163 \par 01164 <b> SL_SO_SECURE_FILES_DH_KEY_FILE_NAME:</b> 01165 \code 01166 sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_SECURE_FILES_DH_KEY_FILE_NAME,"myDHinServerMode.der",strlen("myDHinServerMode.der")); 01167 \endcode 01168 01169 \par 01170 <b> SL_IP_MULTICAST_TTL:</b> 01171 \code 01172 _u8 ttl = 20; 01173 sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_MULTICAST_TTL, &ttl, sizeof(ttl)); 01174 \endcode 01175 01176 \par 01177 <b> SL_IP_ADD_MEMBERSHIP:</b> 01178 \code 01179 SlSockIpMreq mreq; 01180 sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_ADD_MEMBERSHIP, &mreq, sizeof(mreq)); 01181 \endcode 01182 01183 \par 01184 <b> SL_IP_DROP_MEMBERSHIP:</b> 01185 \code 01186 SlSockIpMreq mreq; 01187 sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_DROP_MEMBERSHIP, &mreq, sizeof(mreq)); 01188 \endcode 01189 01190 \par 01191 <b> SL_SO_CHANGE_CHANNEL:</b> 01192 \code 01193 _u32 newChannel = 6; // range is 1-13 01194 sl_SetSockOpt(SockID, SL_SOL_SOCKET, SL_SO_CHANGE_CHANNEL, &newChannel, sizeof(newChannel)); 01195 \endcode 01196 01197 \par 01198 <b> SL_IP_RAW_RX_NO_HEADER:</b> 01199 \code 01200 _u32 header = 1; // remove ip header 01201 sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_RAW_RX_NO_HEADER, &header, sizeof(header)); 01202 \endcode 01203 01204 \par 01205 <b> SL_IP_HDRINCL:</b> 01206 \code 01207 _u32 header = 1; 01208 sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_HDRINCL, &header, sizeof(header)); 01209 \endcode 01210 \par 01211 <b> SL_IP_RAW_IPV6_HDRINCL:</b> 01212 \code 01213 _u32 header = 1; 01214 sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_RAW_IPV6_HDRINCL, &header, sizeof(header)); 01215 \endcode 01216 01217 \par 01218 <b> SL_SO_PHY_RATE:</b> 01219 \code 01220 _u32 rate = 6; // see wlan.h RateIndex_e for values 01221 sl_SetSockOpt(SockID, SL_SOL_PHY_OPT, SL_SO_PHY_RATE, &rate, sizeof(rate)); 01222 \endcode 01223 01224 \par 01225 <b> SL_SO_PHY_TX_POWER:</b> 01226 \code 01227 _u32 txpower = 1; // valid range is 1-15 01228 sl_SetSockOpt(SockID, SL_SOL_PHY_OPT, SL_SO_PHY_TX_POWER, &txpower, sizeof(txpower)); 01229 \endcode 01230 01231 \par 01232 <b> SL_SO_PHY_NUM_FRAMES_TO_TX:</b> 01233 \code 01234 _u32 numframes = 1; 01235 sl_SetSockOpt(SockID, SL_SOL_PHY_OPT, SL_SO_PHY_NUM_FRAMES_TO_TX, &numframes, sizeof(numframes)); 01236 \endcode 01237 01238 \par 01239 <b> SL_SO_PHY_PREAMBLE:</b> 01240 \code 01241 _u32 preamble = 1; 01242 sl_SetSockOpt(SockID, SL_SOL_PHY_OPT, SL_SO_PHY_PREAMBLE, &preamble, sizeof(preamble)); 01243 \endcode 01244 01245 */ 01246 #if _SL_INCLUDE_FUNC(sl_SetSockOpt) 01247 _i16 sl_SetSockOpt(_i16 sd, _i16 level, _i16 optname, const void *optval, SlSocklen_t optlen); 01248 #endif 01249 01250 /*! 01251 \brief Get socket options 01252 01253 This function manipulate the options associated with a socket. 01254 Options may exist at multiple protocol levels; they are always 01255 present at the uppermost socket level. 01256 01257 When manipulating socket options the level at which the option resides 01258 and the name of the option must be specified. To manipulate options at 01259 the socket level, level is specified as SOL_SOCKET. To manipulate 01260 options at any other level the protocol number of the appropriate proto- 01261 col controlling the option is supplied. For example, to indicate that an 01262 option is to be interpreted by the TCP protocol, level should be set to 01263 the protocol number of TCP; 01264 01265 The parameters optval and optlen are used to access optval - 01266 ues for setsockopt(). For getsockopt() they identify a 01267 buffer in which the value for the requested option(s) are to 01268 be returned. For getsockopt(), optlen is a value-result 01269 parameter, initially containing the size of the buffer 01270 pointed to by option_value, and modified on return to 01271 indicate the actual size of the value returned. If no option 01272 value is to be supplied or returned, option_value may be 01273 NULL. 01274 01275 01276 \param[in] sd socket handle 01277 \param[in] level defines the protocol level for this option 01278 \param[in] optname defines the option name to interrogate 01279 \param[out] optval specifies a value for the option 01280 \param[out] optlen specifies the length of the 01281 option value 01282 01283 \return On success, zero is returned. 01284 On error, a negative value is returned. 01285 \sa sl_SetSockOpt 01286 \note See sl_SetSockOpt 01287 belongs to \ref ext_api 01288 \warning 01289 */ 01290 #if _SL_INCLUDE_FUNC(sl_GetSockOpt) 01291 _i16 sl_GetSockOpt(_i16 sd, _i16 level, _i16 optname, void *optval, SlSocklen_t *optlen); 01292 #endif 01293 01294 /*! 01295 \brief read data from TCP socket 01296 01297 function receives a message from a connection-mode socket 01298 01299 \param[in] sd socket handle 01300 \param[out] buf Points to the buffer where the 01301 message should be stored. 01302 \param[in] Len Specifies the length in bytes of 01303 the buffer pointed to by the buffer argument. 01304 Range: 1-16000 bytes 01305 \param[in] flags Specifies the type of message 01306 reception. On this version, this parameter is not 01307 supported. 01308 01309 \return return the number of bytes received, 01310 or a negative value if an error occurred. 01311 using a non-blocking recv a possible negative value is SL_EAGAIN. 01312 SL_POOL_IS_EMPTY may be return in case there are no resources in the system 01313 In this case try again later or increase MAX_CONCURRENT_ACTIONS 01314 01315 \sa sl_RecvFrom 01316 \note belongs to \ref recv_api 01317 \warning 01318 \par Examples: 01319 \code An example of receiving data using TCP socket: 01320 01321 SlSockAddrIn_t Addr; 01322 SlSockAddrIn_t LocalAddr; 01323 _i16 AddrSize = sizeof(SlSockAddrIn_t); 01324 _i16 SockID, newSockID; 01325 _i16 Status; 01326 _i8 Buf[RECV_BUF_LEN]; 01327 01328 LocalAddr.sin_family = SL_AF_INET; 01329 LocalAddr.sin_port = sl_Htons(5001); 01330 LocalAddr.sin_addr.s_addr = 0; 01331 01332 Addr.sin_family = SL_AF_INET; 01333 Addr.sin_port = sl_Htons(5001); 01334 Addr.sin_addr.s_addr = sl_Htonl(SL_IPV4_VAL(10,1,1,200)); 01335 01336 SockID = sl_Socket(SL_AF_INET,SL_SOCK_STREAM, 0); 01337 Status = sl_Bind(SockID, (SlSockAddr_t *)&LocalAddr, AddrSize); 01338 Status = sl_Listen(SockID, 0); 01339 newSockID = sl_Accept(SockID, (SlSockAddr_t*)&Addr, (SlSocklen_t*) &AddrSize); 01340 Status = sl_Recv(newSockID, Buf, 1460, 0); 01341 \endcode 01342 \code Example code for Rx transceiver mode using a raw socket 01343 _i8 buffer[1536]; 01344 _i16 sd; 01345 _u16 size; 01346 SlTransceiverRxOverHead_t *transHeader; 01347 sd = sl_Socket(SL_AF_RF,SL_SOCK_RAW,11); // channel 11 01348 while(1) 01349 { 01350 size = sl_Recv(sd,buffer,1536,0); 01351 transHeader = (SlTransceiverRxOverHead_t *)buffer; 01352 printf("RSSI is %d frame type is 0x%x size %d\n",transHeader->rssi,buffer[sizeof(SlTransceiverRxOverHead_t)],size); 01353 } 01354 \endcode 01355 */ 01356 #if _SL_INCLUDE_FUNC(sl_Recv) 01357 _i16 sl_Recv(_i16 sd, void *buf, _i16 Len, _i16 flags); 01358 #endif 01359 01360 /*! 01361 \brief read data from socket 01362 01363 function receives a message from a connection-mode or 01364 connectionless-mode socket 01365 01366 \param[in] sd socket handle 01367 \param[out] buf Points to the buffer where the message should be stored. 01368 \param[in] Len Specifies the length in bytes of the buffer pointed to by the buffer argument. 01369 Range: 1-16000 bytes 01370 \param[in] flags Specifies the type of message 01371 reception. On this version, this parameter is not 01372 supported. 01373 \param[in] from pointer to an address structure 01374 indicating the source 01375 address.\n sockaddr:\n - code 01376 for the address format. On this 01377 version only AF_INET is 01378 supported.\n - socket address, 01379 the length depends on the code 01380 format 01381 \param[in] fromlen source address structure 01382 size. This parameter MUST be set to the size of the structure pointed to by addr. 01383 01384 01385 \return return the number of bytes received, 01386 or a negative value if an error occurred. 01387 using a non-blocking recv a possible negative value is SL_EAGAIN. 01388 SL_RET_CODE_INVALID_INPUT (-2) will be returned if fromlen has incorrect length. 01389 SL_POOL_IS_EMPTY may be return in case there are no resources in the system 01390 In this case try again later or increase MAX_CONCURRENT_ACTIONS 01391 01392 \sa sl_Recv 01393 \note belongs to \ref recv_api 01394 \warning 01395 \par Example: 01396 \code An example of receiving data: 01397 01398 SlSockAddrIn_t Addr; 01399 SlSockAddrIn_t LocalAddr; 01400 _i16 AddrSize = sizeof(SlSockAddrIn_t); 01401 _i16 SockID; 01402 _i16 Status; 01403 _i8 Buf[RECV_BUF_LEN]; 01404 01405 LocalAddr.sin_family = SL_AF_INET; 01406 LocalAddr.sin_port = sl_Htons(5001); 01407 LocalAddr.sin_addr.s_addr = 0; 01408 01409 SockID = sl_Socket(SL_AF_INET,SL_SOCK_DGRAM, 0); 01410 Status = sl_Bind(SockID, (SlSockAddr_t *)&LocalAddr, AddrSize); 01411 Status = sl_RecvFrom(SockID, Buf, 1472, 0, (SlSockAddr_t *)&Addr, (SlSocklen_t*)&AddrSize); 01412 01413 \endcode 01414 */ 01415 #if _SL_INCLUDE_FUNC(sl_RecvFrom) 01416 _i16 sl_RecvFrom(_i16 sd, void *buf, _i16 Len, _i16 flags, SlSockAddr_t *from, SlSocklen_t *fromlen); 01417 #endif 01418 01419 /*! 01420 \brief write data to TCP socket 01421 01422 This function is used to transmit a message to another socket. 01423 Returns immediately after sending data to device. 01424 In case of TCP failure an async event SL_SOCKET_TX_FAILED_EVENT is going to 01425 be received. 01426 In case of a RAW socket (transceiver mode), extra 4 bytes should be reserved at the end of the 01427 frame data buffer for WLAN FCS 01428 01429 \param[in] sd socket handle 01430 \param[in] buf Points to a buffer containing 01431 the message to be sent 01432 \param[in] Len message size in bytes. Range: 1-1460 bytes 01433 \param[in] flags Specifies the type of message 01434 transmission. On this version, this parameter is not 01435 supported for TCP. 01436 For transceiver mode, the SL_RAW_RF_TX_PARAMS macro can be used to determine 01437 transmission parameters (channel,rate,tx_power,preamble) 01438 01439 01440 \return Return the number of bytes transmitted, 01441 or -1 if an error occurred 01442 01443 \sa sl_SendTo 01444 \note belongs to \ref send_api 01445 \warning 01446 \par Example: 01447 \code An example of sending data: 01448 01449 SlSockAddrIn_t Addr; 01450 _i16 AddrSize = sizeof(SlSockAddrIn_t); 01451 _i16 SockID; 01452 _i16 Status; 01453 _i8 Buf[SEND_BUF_LEN]; 01454 01455 Addr.sin_family = SL_AF_INET; 01456 Addr.sin_port = sl_Htons(5001); 01457 Addr.sin_addr.s_addr = sl_Htonl(SL_IPV4_VAL(10,1,1,200)); 01458 01459 SockID = sl_Socket(SL_AF_INET,SL_SOCK_STREAM, 0); 01460 Status = sl_Connect(SockID, (SlSockAddr_t *)&Addr, AddrSize); 01461 Status = sl_Send(SockID, Buf, 1460, 0 ); 01462 01463 \endcode 01464 */ 01465 #if _SL_INCLUDE_FUNC(sl_Send ) 01466 _i16 sl_Send(_i16 sd, const void *buf, _i16 Len, _i16 flags); 01467 #endif 01468 01469 /*! 01470 \brief write data to socket 01471 01472 This function is used to transmit a message to another socket 01473 (connection less socket SOCK_DGRAM, SOCK_RAW). 01474 Returns immediately after sending data to device. 01475 In case of transmission failure an async event SL_SOCKET_TX_FAILED_EVENT is going to 01476 be received. 01477 01478 \param[in] sd socket handle 01479 \param[in] buf Points to a buffer containing 01480 the message to be sent 01481 \param[in] Len message size in bytes. Range: 1-1460 bytes 01482 \param[in] flags Specifies the type of message 01483 transmission. On this version, this parameter is not 01484 supported 01485 \param[in] to pointer to an address structure 01486 indicating the destination 01487 address.\n sockaddr:\n - code 01488 for the address format. On this 01489 version only AF_INET is 01490 supported.\n - socket address, 01491 the length depends on the code 01492 format 01493 \param[in] tolen destination address structure size 01494 01495 \return Return the number of transmitted bytes, 01496 or -1 if an error occurred 01497 01498 \sa sl_Send 01499 \note belongs to \ref send_api 01500 \warning 01501 \par Example: 01502 \code An example of sending data: 01503 01504 SlSockAddrIn_t Addr; 01505 _i16 AddrSize = sizeof(SlSockAddrIn_t); 01506 _i16 SockID; 01507 _i16 Status; 01508 _i8 Buf[SEND_BUF_LEN]; 01509 01510 Addr.sin_family = SL_AF_INET; 01511 Addr.sin_port = sl_Htons(5001); 01512 Addr.sin_addr.s_addr = sl_Htonl(SL_IPV4_VAL(10,1,1,200)); 01513 01514 SockID = sl_Socket(SL_AF_INET,SL_SOCK_DGRAM, 0); 01515 Status = sl_SendTo(SockID, Buf, 1472, 0, (SlSockAddr_t *)&Addr, AddrSize); 01516 01517 \endcode 01518 */ 01519 #if _SL_INCLUDE_FUNC(sl_SendTo) 01520 _i16 sl_SendTo(_i16 sd, const void *buf, _i16 Len, _i16 flags, const SlSockAddr_t *to, SlSocklen_t tolen); 01521 #endif 01522 01523 /*! 01524 \brief Reorder the bytes of a 32-bit unsigned value 01525 01526 This function is used to Reorder the bytes of a 32-bit unsigned value from processor order to network order. 01527 01528 \param[in] var variable to reorder 01529 01530 \return Return the reorder variable, 01531 01532 \sa sl_SendTo sl_Bind sl_Connect sl_RecvFrom sl_Accept 01533 \note belongs to \ref send_api 01534 \warning 01535 */ 01536 #if _SL_INCLUDE_FUNC(sl_Htonl ) 01537 _u32 sl_Htonl( _u32 val ); 01538 01539 #define sl_Ntohl sl_Htonl /* Reorder the bytes of a 16-bit unsigned value from network order to processor orde. */ 01540 #endif 01541 01542 /*! 01543 \brief Reorder the bytes of a 16-bit unsigned value 01544 01545 This function is used to Reorder the bytes of a 16-bit unsigned value from processor order to network order. 01546 01547 \param[in] var variable to reorder 01548 01549 \return Return the reorder variable, 01550 01551 \sa sl_SendTo sl_Bind sl_Connect sl_RecvFrom sl_Accept 01552 \note belongs to \ref send_api 01553 \warning 01554 */ 01555 #if _SL_INCLUDE_FUNC(sl_Htons ) 01556 _u16 sl_Htons( _u16 val ); 01557 01558 #define sl_Ntohs sl_Htons /* Reorder the bytes of a 16-bit unsigned value from network order to processor orde. */ 01559 #endif 01560 01561 /*! 01562 01563 Close the Doxygen group. 01564 @} 01565 01566 */ 01567 01568 01569 #ifdef __cplusplus 01570 } 01571 #endif /* __cplusplus */ 01572 01573 #endif /* __SOCKET_H__ */ 01574 01575
Generated on Tue Jul 12 2022 12:06:49 by
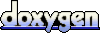