
DeepCover Embedded Security in IoT: Public-key Secured Data Paths
Dependencies: MaximInterface
itoa.h
00001 // Tencent is pleased to support the open source community by making RapidJSON available. 00002 // 00003 // Copyright (C) 2015 THL A29 Limited, a Tencent company, and Milo Yip. All rights reserved. 00004 // 00005 // Licensed under the MIT License (the "License"); you may not use this file except 00006 // in compliance with the License. You may obtain a copy of the License at 00007 // 00008 // http://opensource.org/licenses/MIT 00009 // 00010 // Unless required by applicable law or agreed to in writing, software distributed 00011 // under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR 00012 // CONDITIONS OF ANY KIND, either express or implied. See the License for the 00013 // specific language governing permissions and limitations under the License. 00014 00015 #ifndef RAPIDJSON_ITOA_ 00016 #define RAPIDJSON_ITOA_ 00017 00018 #include "../rapidjson.h" 00019 00020 RAPIDJSON_NAMESPACE_BEGIN 00021 namespace internal { 00022 00023 inline const char* GetDigitsLut() { 00024 static const char cDigitsLut[200] = { 00025 '0','0','0','1','0','2','0','3','0','4','0','5','0','6','0','7','0','8','0','9', 00026 '1','0','1','1','1','2','1','3','1','4','1','5','1','6','1','7','1','8','1','9', 00027 '2','0','2','1','2','2','2','3','2','4','2','5','2','6','2','7','2','8','2','9', 00028 '3','0','3','1','3','2','3','3','3','4','3','5','3','6','3','7','3','8','3','9', 00029 '4','0','4','1','4','2','4','3','4','4','4','5','4','6','4','7','4','8','4','9', 00030 '5','0','5','1','5','2','5','3','5','4','5','5','5','6','5','7','5','8','5','9', 00031 '6','0','6','1','6','2','6','3','6','4','6','5','6','6','6','7','6','8','6','9', 00032 '7','0','7','1','7','2','7','3','7','4','7','5','7','6','7','7','7','8','7','9', 00033 '8','0','8','1','8','2','8','3','8','4','8','5','8','6','8','7','8','8','8','9', 00034 '9','0','9','1','9','2','9','3','9','4','9','5','9','6','9','7','9','8','9','9' 00035 }; 00036 return cDigitsLut; 00037 } 00038 00039 inline char* u32toa(uint32_t value, char* buffer) { 00040 const char* cDigitsLut = GetDigitsLut(); 00041 00042 if (value < 10000) { 00043 const uint32_t d1 = (value / 100) << 1; 00044 const uint32_t d2 = (value % 100) << 1; 00045 00046 if (value >= 1000) 00047 *buffer++ = cDigitsLut[d1]; 00048 if (value >= 100) 00049 *buffer++ = cDigitsLut[d1 + 1]; 00050 if (value >= 10) 00051 *buffer++ = cDigitsLut[d2]; 00052 *buffer++ = cDigitsLut[d2 + 1]; 00053 } 00054 else if (value < 100000000) { 00055 // value = bbbbcccc 00056 const uint32_t b = value / 10000; 00057 const uint32_t c = value % 10000; 00058 00059 const uint32_t d1 = (b / 100) << 1; 00060 const uint32_t d2 = (b % 100) << 1; 00061 00062 const uint32_t d3 = (c / 100) << 1; 00063 const uint32_t d4 = (c % 100) << 1; 00064 00065 if (value >= 10000000) 00066 *buffer++ = cDigitsLut[d1]; 00067 if (value >= 1000000) 00068 *buffer++ = cDigitsLut[d1 + 1]; 00069 if (value >= 100000) 00070 *buffer++ = cDigitsLut[d2]; 00071 *buffer++ = cDigitsLut[d2 + 1]; 00072 00073 *buffer++ = cDigitsLut[d3]; 00074 *buffer++ = cDigitsLut[d3 + 1]; 00075 *buffer++ = cDigitsLut[d4]; 00076 *buffer++ = cDigitsLut[d4 + 1]; 00077 } 00078 else { 00079 // value = aabbbbcccc in decimal 00080 00081 const uint32_t a = value / 100000000; // 1 to 42 00082 value %= 100000000; 00083 00084 if (a >= 10) { 00085 const unsigned i = a << 1; 00086 *buffer++ = cDigitsLut[i]; 00087 *buffer++ = cDigitsLut[i + 1]; 00088 } 00089 else 00090 *buffer++ = static_cast<char>('0' + static_cast<char>(a)); 00091 00092 const uint32_t b = value / 10000; // 0 to 9999 00093 const uint32_t c = value % 10000; // 0 to 9999 00094 00095 const uint32_t d1 = (b / 100) << 1; 00096 const uint32_t d2 = (b % 100) << 1; 00097 00098 const uint32_t d3 = (c / 100) << 1; 00099 const uint32_t d4 = (c % 100) << 1; 00100 00101 *buffer++ = cDigitsLut[d1]; 00102 *buffer++ = cDigitsLut[d1 + 1]; 00103 *buffer++ = cDigitsLut[d2]; 00104 *buffer++ = cDigitsLut[d2 + 1]; 00105 *buffer++ = cDigitsLut[d3]; 00106 *buffer++ = cDigitsLut[d3 + 1]; 00107 *buffer++ = cDigitsLut[d4]; 00108 *buffer++ = cDigitsLut[d4 + 1]; 00109 } 00110 return buffer; 00111 } 00112 00113 inline char* i32toa(int32_t value, char* buffer) { 00114 uint32_t u = static_cast<uint32_t>(value); 00115 if (value < 0) { 00116 *buffer++ = '-'; 00117 u = ~u + 1; 00118 } 00119 00120 return u32toa(u, buffer); 00121 } 00122 00123 inline char* u64toa(uint64_t value, char* buffer) { 00124 const char* cDigitsLut = GetDigitsLut(); 00125 const uint64_t kTen8 = 100000000; 00126 const uint64_t kTen9 = kTen8 * 10; 00127 const uint64_t kTen10 = kTen8 * 100; 00128 const uint64_t kTen11 = kTen8 * 1000; 00129 const uint64_t kTen12 = kTen8 * 10000; 00130 const uint64_t kTen13 = kTen8 * 100000; 00131 const uint64_t kTen14 = kTen8 * 1000000; 00132 const uint64_t kTen15 = kTen8 * 10000000; 00133 const uint64_t kTen16 = kTen8 * kTen8; 00134 00135 if (value < kTen8) { 00136 uint32_t v = static_cast<uint32_t>(value); 00137 if (v < 10000) { 00138 const uint32_t d1 = (v / 100) << 1; 00139 const uint32_t d2 = (v % 100) << 1; 00140 00141 if (v >= 1000) 00142 *buffer++ = cDigitsLut[d1]; 00143 if (v >= 100) 00144 *buffer++ = cDigitsLut[d1 + 1]; 00145 if (v >= 10) 00146 *buffer++ = cDigitsLut[d2]; 00147 *buffer++ = cDigitsLut[d2 + 1]; 00148 } 00149 else { 00150 // value = bbbbcccc 00151 const uint32_t b = v / 10000; 00152 const uint32_t c = v % 10000; 00153 00154 const uint32_t d1 = (b / 100) << 1; 00155 const uint32_t d2 = (b % 100) << 1; 00156 00157 const uint32_t d3 = (c / 100) << 1; 00158 const uint32_t d4 = (c % 100) << 1; 00159 00160 if (value >= 10000000) 00161 *buffer++ = cDigitsLut[d1]; 00162 if (value >= 1000000) 00163 *buffer++ = cDigitsLut[d1 + 1]; 00164 if (value >= 100000) 00165 *buffer++ = cDigitsLut[d2]; 00166 *buffer++ = cDigitsLut[d2 + 1]; 00167 00168 *buffer++ = cDigitsLut[d3]; 00169 *buffer++ = cDigitsLut[d3 + 1]; 00170 *buffer++ = cDigitsLut[d4]; 00171 *buffer++ = cDigitsLut[d4 + 1]; 00172 } 00173 } 00174 else if (value < kTen16) { 00175 const uint32_t v0 = static_cast<uint32_t>(value / kTen8); 00176 const uint32_t v1 = static_cast<uint32_t>(value % kTen8); 00177 00178 const uint32_t b0 = v0 / 10000; 00179 const uint32_t c0 = v0 % 10000; 00180 00181 const uint32_t d1 = (b0 / 100) << 1; 00182 const uint32_t d2 = (b0 % 100) << 1; 00183 00184 const uint32_t d3 = (c0 / 100) << 1; 00185 const uint32_t d4 = (c0 % 100) << 1; 00186 00187 const uint32_t b1 = v1 / 10000; 00188 const uint32_t c1 = v1 % 10000; 00189 00190 const uint32_t d5 = (b1 / 100) << 1; 00191 const uint32_t d6 = (b1 % 100) << 1; 00192 00193 const uint32_t d7 = (c1 / 100) << 1; 00194 const uint32_t d8 = (c1 % 100) << 1; 00195 00196 if (value >= kTen15) 00197 *buffer++ = cDigitsLut[d1]; 00198 if (value >= kTen14) 00199 *buffer++ = cDigitsLut[d1 + 1]; 00200 if (value >= kTen13) 00201 *buffer++ = cDigitsLut[d2]; 00202 if (value >= kTen12) 00203 *buffer++ = cDigitsLut[d2 + 1]; 00204 if (value >= kTen11) 00205 *buffer++ = cDigitsLut[d3]; 00206 if (value >= kTen10) 00207 *buffer++ = cDigitsLut[d3 + 1]; 00208 if (value >= kTen9) 00209 *buffer++ = cDigitsLut[d4]; 00210 if (value >= kTen8) 00211 *buffer++ = cDigitsLut[d4 + 1]; 00212 00213 *buffer++ = cDigitsLut[d5]; 00214 *buffer++ = cDigitsLut[d5 + 1]; 00215 *buffer++ = cDigitsLut[d6]; 00216 *buffer++ = cDigitsLut[d6 + 1]; 00217 *buffer++ = cDigitsLut[d7]; 00218 *buffer++ = cDigitsLut[d7 + 1]; 00219 *buffer++ = cDigitsLut[d8]; 00220 *buffer++ = cDigitsLut[d8 + 1]; 00221 } 00222 else { 00223 const uint32_t a = static_cast<uint32_t>(value / kTen16); // 1 to 1844 00224 value %= kTen16; 00225 00226 if (a < 10) 00227 *buffer++ = static_cast<char>('0' + static_cast<char>(a)); 00228 else if (a < 100) { 00229 const uint32_t i = a << 1; 00230 *buffer++ = cDigitsLut[i]; 00231 *buffer++ = cDigitsLut[i + 1]; 00232 } 00233 else if (a < 1000) { 00234 *buffer++ = static_cast<char>('0' + static_cast<char>(a / 100)); 00235 00236 const uint32_t i = (a % 100) << 1; 00237 *buffer++ = cDigitsLut[i]; 00238 *buffer++ = cDigitsLut[i + 1]; 00239 } 00240 else { 00241 const uint32_t i = (a / 100) << 1; 00242 const uint32_t j = (a % 100) << 1; 00243 *buffer++ = cDigitsLut[i]; 00244 *buffer++ = cDigitsLut[i + 1]; 00245 *buffer++ = cDigitsLut[j]; 00246 *buffer++ = cDigitsLut[j + 1]; 00247 } 00248 00249 const uint32_t v0 = static_cast<uint32_t>(value / kTen8); 00250 const uint32_t v1 = static_cast<uint32_t>(value % kTen8); 00251 00252 const uint32_t b0 = v0 / 10000; 00253 const uint32_t c0 = v0 % 10000; 00254 00255 const uint32_t d1 = (b0 / 100) << 1; 00256 const uint32_t d2 = (b0 % 100) << 1; 00257 00258 const uint32_t d3 = (c0 / 100) << 1; 00259 const uint32_t d4 = (c0 % 100) << 1; 00260 00261 const uint32_t b1 = v1 / 10000; 00262 const uint32_t c1 = v1 % 10000; 00263 00264 const uint32_t d5 = (b1 / 100) << 1; 00265 const uint32_t d6 = (b1 % 100) << 1; 00266 00267 const uint32_t d7 = (c1 / 100) << 1; 00268 const uint32_t d8 = (c1 % 100) << 1; 00269 00270 *buffer++ = cDigitsLut[d1]; 00271 *buffer++ = cDigitsLut[d1 + 1]; 00272 *buffer++ = cDigitsLut[d2]; 00273 *buffer++ = cDigitsLut[d2 + 1]; 00274 *buffer++ = cDigitsLut[d3]; 00275 *buffer++ = cDigitsLut[d3 + 1]; 00276 *buffer++ = cDigitsLut[d4]; 00277 *buffer++ = cDigitsLut[d4 + 1]; 00278 *buffer++ = cDigitsLut[d5]; 00279 *buffer++ = cDigitsLut[d5 + 1]; 00280 *buffer++ = cDigitsLut[d6]; 00281 *buffer++ = cDigitsLut[d6 + 1]; 00282 *buffer++ = cDigitsLut[d7]; 00283 *buffer++ = cDigitsLut[d7 + 1]; 00284 *buffer++ = cDigitsLut[d8]; 00285 *buffer++ = cDigitsLut[d8 + 1]; 00286 } 00287 00288 return buffer; 00289 } 00290 00291 inline char* i64toa(int64_t value, char* buffer) { 00292 uint64_t u = static_cast<uint64_t>(value); 00293 if (value < 0) { 00294 *buffer++ = '-'; 00295 u = ~u + 1; 00296 } 00297 00298 return u64toa(u, buffer); 00299 } 00300 00301 } // namespace internal 00302 RAPIDJSON_NAMESPACE_END 00303 00304 #endif // RAPIDJSON_ITOA_
Generated on Tue Jul 12 2022 12:06:48 by
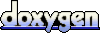