
DeepCover Embedded Security in IoT: Public-key Secured Data Paths
Dependencies: MaximInterface
fs.c
00001 /* 00002 * fs.c - CC31xx/CC32xx Host Driver Implementation 00003 * 00004 * Copyright (C) 2015 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * 00007 * Redistribution and use in source and binary forms, with or without 00008 * modification, are permitted provided that the following conditions 00009 * are met: 00010 * 00011 * Redistributions of source code must retain the above copyright 00012 * notice, this list of conditions and the following disclaimer. 00013 * 00014 * Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in the 00016 * documentation and/or other materials provided with the 00017 * distribution. 00018 * 00019 * Neither the name of Texas Instruments Incorporated nor the names of 00020 * its contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00026 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00030 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00031 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 00038 00039 /*****************************************************************************/ 00040 /* Include files */ 00041 /*****************************************************************************/ 00042 #include "simplelink.h" 00043 #include "protocol.h" 00044 #include "driver.h" 00045 00046 /*****************************************************************************/ 00047 /* Macro declarations */ 00048 /*****************************************************************************/ 00049 #define sl_min(a,b) (((a) < (b)) ? (a) : (b)) 00050 #define MAX_NVMEM_CHUNK_SIZE 1460 00051 00052 /*****************************************************************************/ 00053 /* Internal functions */ 00054 /*****************************************************************************/ 00055 00056 #ifndef SL_TINY 00057 00058 static _u16 _sl_Strlen(const _u8 *buffer); 00059 00060 /*****************************************************************************/ 00061 /* _sl_Strlen */ 00062 /*****************************************************************************/ 00063 static _u16 _sl_Strlen(const _u8 *buffer) 00064 { 00065 _u16 len = 0; 00066 if( buffer != NULL ) 00067 { 00068 while(*buffer++) len++; 00069 } 00070 return len; 00071 } 00072 00073 /*****************************************************************************/ 00074 /* _sl_GetCreateFsMode */ 00075 /*****************************************************************************/ 00076 _u32 _sl_GetCreateFsMode(_u32 maxSizeInBytes,_u32 accessFlags) 00077 { 00078 _u32 granIdx = 0; 00079 _u32 granNum = 0; 00080 _u32 granTable[_FS_MAX_MODE_SIZE_GRAN] = {256,1024,4096,16384,65536}; 00081 for(granIdx= _FS_MODE_SIZE_GRAN_256B ;granIdx< _FS_MAX_MODE_SIZE_GRAN;granIdx++) 00082 { 00083 if( granTable[granIdx]*255 >= maxSizeInBytes ) 00084 break; 00085 } 00086 granNum = maxSizeInBytes/granTable[granIdx]; 00087 if( maxSizeInBytes % granTable[granIdx] != 0 ) 00088 granNum++; 00089 00090 return _FS_MODE(_FS_MODE_OPEN_WRITE_CREATE_IF_NOT_EXIST, granIdx, granNum, accessFlags); 00091 } 00092 00093 #endif 00094 00095 /*****************************************************************************/ 00096 /* API functions */ 00097 /*****************************************************************************/ 00098 00099 /*****************************************************************************/ 00100 /* sl_FsOpen */ 00101 /*****************************************************************************/ 00102 typedef union 00103 { 00104 _FsOpenCommand_t Cmd; 00105 _FsOpenResponse_t Rsp; 00106 }_SlFsOpenMsg_u; 00107 00108 00109 #if _SL_INCLUDE_FUNC(sl_FsOpen) 00110 00111 static const _SlCmdCtrl_t _SlFsOpenCmdCtrl = 00112 { 00113 SL_OPCODE_NVMEM_FILEOPEN, 00114 (_SlArgSize_t)sizeof(_FsOpenCommand_t), 00115 (_SlArgSize_t)sizeof(_FsOpenResponse_t) 00116 }; 00117 00118 _i32 sl_FsOpen(const _u8 *pFileName,const _u32 AccessModeAndMaxSize, _u32 *pToken,_i32 *pFileHandle) 00119 { 00120 _SlReturnVal_t RetVal; 00121 _SlFsOpenMsg_u Msg; 00122 _SlCmdExt_t CmdExt; 00123 00124 /* verify no erorr handling in progress. if in progress than 00125 ignore the API execution and return immediately with an error */ 00126 VERIFY_NO_ERROR_HANDLING_IN_PROGRESS(); 00127 00128 CmdExt.TxPayloadLen = (_u16)((_sl_Strlen(pFileName)+4) & (~3)); /* add 4: 1 for NULL and the 3 for align */ 00129 CmdExt.RxPayloadLen = 0; 00130 CmdExt.pTxPayload = (_u8*)pFileName; 00131 CmdExt.pRxPayload = NULL; 00132 00133 Msg.Cmd.Mode = AccessModeAndMaxSize; 00134 00135 if(pToken != NULL) 00136 { 00137 Msg.Cmd.Token = *pToken; 00138 } 00139 else 00140 { 00141 Msg.Cmd.Token = 0; 00142 } 00143 00144 RetVal = _SlDrvCmdOp((_SlCmdCtrl_t *)&_SlFsOpenCmdCtrl, &Msg, &CmdExt); 00145 *pFileHandle = (_i32)Msg.Rsp.FileHandle; 00146 if (pToken != NULL) 00147 { 00148 *pToken = Msg.Rsp.Token; 00149 } 00150 00151 /* in case of an error, return the erros file handler as an error code */ 00152 if( *pFileHandle < 0 ) 00153 { 00154 return *pFileHandle; 00155 } 00156 return (_i32)RetVal; 00157 } 00158 #endif 00159 00160 /*****************************************************************************/ 00161 /* sl_FsClose */ 00162 /*****************************************************************************/ 00163 typedef union 00164 { 00165 _FsCloseCommand_t Cmd; 00166 _BasicResponse_t Rsp; 00167 }_SlFsCloseMsg_u; 00168 00169 00170 #if _SL_INCLUDE_FUNC(sl_FsClose) 00171 00172 static const _SlCmdCtrl_t _SlFsCloseCmdCtrl = 00173 { 00174 SL_OPCODE_NVMEM_FILECLOSE, 00175 (_SlArgSize_t)sizeof(_FsCloseCommand_t), 00176 (_SlArgSize_t)sizeof(_FsCloseResponse_t) 00177 }; 00178 00179 00180 _i16 sl_FsClose(const _i32 FileHdl, const _u8* pCeritificateFileName,const _u8* pSignature ,const _u32 SignatureLen) 00181 { 00182 _SlFsCloseMsg_u Msg; 00183 _SlCmdExt_t ExtCtrl; 00184 00185 _SlDrvMemZero(&Msg, (_u16)sizeof(_FsCloseCommand_t)); 00186 00187 /* verify no erorr handling in progress. if in progress than 00188 ignore the API execution and return immediately with an error */ 00189 VERIFY_NO_ERROR_HANDLING_IN_PROGRESS(); 00190 00191 Msg.Cmd.FileHandle = (_u32)FileHdl; 00192 if( pCeritificateFileName != NULL ) 00193 { 00194 Msg.Cmd.CertificFileNameLength = (_u32)((_sl_Strlen(pCeritificateFileName)+4) & (~3)); /* add 4: 1 for NULL and the 3 for align */ 00195 } 00196 Msg.Cmd.SignatureLen = SignatureLen; 00197 00198 ExtCtrl.TxPayloadLen = (_u16)(((SignatureLen+3) & (~3))); /* align */ 00199 ExtCtrl.pTxPayload = (_u8*)pSignature; 00200 ExtCtrl.RxPayloadLen = (_i16)Msg.Cmd.CertificFileNameLength; 00201 ExtCtrl.pRxPayload = (_u8*)pCeritificateFileName; /* Add signature */ 00202 00203 if(ExtCtrl.pRxPayload != NULL && ExtCtrl.RxPayloadLen != 0) 00204 { 00205 ExtCtrl.RxPayloadLen = ExtCtrl.RxPayloadLen * (-1); 00206 } 00207 00208 VERIFY_RET_OK(_SlDrvCmdOp((_SlCmdCtrl_t *)&_SlFsCloseCmdCtrl, &Msg, &ExtCtrl)); 00209 00210 return (_i16)((_i16)Msg.Rsp.status); 00211 } 00212 #endif 00213 00214 00215 /*****************************************************************************/ 00216 /* sl_FsRead */ 00217 /*****************************************************************************/ 00218 typedef union 00219 { 00220 _FsReadCommand_t Cmd; 00221 _FsReadResponse_t Rsp; 00222 }_SlFsReadMsg_u; 00223 00224 #if _SL_INCLUDE_FUNC(sl_FsRead) 00225 00226 00227 static const _SlCmdCtrl_t _SlFsReadCmdCtrl = 00228 { 00229 SL_OPCODE_NVMEM_FILEREADCOMMAND, 00230 (_SlArgSize_t)sizeof(_FsReadCommand_t), 00231 (_SlArgSize_t)sizeof(_FsReadResponse_t) 00232 }; 00233 00234 _i32 sl_FsRead(const _i32 FileHdl,_u32 Offset, _u8* pData,_u32 Len) 00235 { 00236 _SlFsReadMsg_u Msg; 00237 _SlCmdExt_t ExtCtrl; 00238 _u16 ChunkLen; 00239 _SlReturnVal_t RetVal =0; 00240 _i32 RetCount = 0; 00241 00242 /* verify no erorr handling in progress. if in progress than 00243 ignore the API execution and return immediately with an error */ 00244 VERIFY_NO_ERROR_HANDLING_IN_PROGRESS(); 00245 00246 ExtCtrl.TxPayloadLen = 0; 00247 ExtCtrl.pTxPayload = NULL; 00248 00249 ChunkLen = (_u16)sl_min(MAX_NVMEM_CHUNK_SIZE,Len); 00250 ExtCtrl.RxPayloadLen = (_i16)ChunkLen; 00251 ExtCtrl.pRxPayload = (_u8 *)(pData); 00252 Msg.Cmd.Offset = Offset; 00253 Msg.Cmd.Len = ChunkLen; 00254 Msg.Cmd.FileHandle = (_u32)FileHdl; 00255 do 00256 { 00257 RetVal = _SlDrvCmdOp((_SlCmdCtrl_t *)&_SlFsReadCmdCtrl, &Msg, &ExtCtrl); 00258 if(SL_OS_RET_CODE_OK == RetVal) 00259 { 00260 if( Msg.Rsp.status < 0) 00261 { 00262 if( RetCount > 0) 00263 { 00264 return RetCount; 00265 } 00266 else 00267 { 00268 return Msg.Rsp.status; 00269 } 00270 } 00271 RetCount += (_i32)Msg.Rsp.status; 00272 Len -= ChunkLen; 00273 Offset += ChunkLen; 00274 Msg.Cmd.Offset = Offset; 00275 ExtCtrl.pRxPayload += ChunkLen; 00276 ChunkLen = (_u16)sl_min(MAX_NVMEM_CHUNK_SIZE,Len); 00277 ExtCtrl.RxPayloadLen = (_i16)ChunkLen; 00278 Msg.Cmd.Len = ChunkLen; 00279 Msg.Cmd.FileHandle = (_u32)FileHdl; 00280 } 00281 else 00282 { 00283 return RetVal; 00284 } 00285 }while(ChunkLen > 0); 00286 00287 return (_i32)RetCount; 00288 } 00289 #endif 00290 00291 /*****************************************************************************/ 00292 /* sl_FsWrite */ 00293 /*****************************************************************************/ 00294 typedef union 00295 { 00296 _FsWriteCommand_t Cmd; 00297 _FsWriteResponse_t Rsp; 00298 }_SlFsWriteMsg_u; 00299 00300 00301 #if _SL_INCLUDE_FUNC(sl_FsWrite) 00302 00303 static const _SlCmdCtrl_t _SlFsWriteCmdCtrl = 00304 { 00305 SL_OPCODE_NVMEM_FILEWRITECOMMAND, 00306 (_SlArgSize_t)sizeof(_FsWriteCommand_t), 00307 (_SlArgSize_t)sizeof(_FsWriteResponse_t) 00308 }; 00309 00310 _i32 sl_FsWrite(const _i32 FileHdl,_u32 Offset, _u8* pData,_u32 Len) 00311 { 00312 _SlFsWriteMsg_u Msg; 00313 _SlCmdExt_t ExtCtrl; 00314 _u16 ChunkLen; 00315 _SlReturnVal_t RetVal; 00316 _i32 RetCount = 0; 00317 00318 /* verify no erorr handling in progress. if in progress than 00319 ignore the API execution and return immediately with an error */ 00320 VERIFY_NO_ERROR_HANDLING_IN_PROGRESS(); 00321 00322 ExtCtrl.RxPayloadLen = 0; 00323 ExtCtrl.pRxPayload = NULL; 00324 00325 ChunkLen = (_u16)sl_min(MAX_NVMEM_CHUNK_SIZE,Len); 00326 ExtCtrl.TxPayloadLen = ChunkLen; 00327 ExtCtrl.pTxPayload = (_u8 *)(pData); 00328 Msg.Cmd.Offset = Offset; 00329 Msg.Cmd.Len = ChunkLen; 00330 Msg.Cmd.FileHandle = (_u32)FileHdl; 00331 00332 do 00333 { 00334 00335 RetVal = _SlDrvCmdOp((_SlCmdCtrl_t *)&_SlFsWriteCmdCtrl, &Msg, &ExtCtrl); 00336 if(SL_OS_RET_CODE_OK == RetVal) 00337 { 00338 if( Msg.Rsp.status < 0) 00339 { 00340 if( RetCount > 0) 00341 { 00342 return RetCount; 00343 } 00344 else 00345 { 00346 return Msg.Rsp.status; 00347 } 00348 } 00349 00350 RetCount += (_i32)Msg.Rsp.status; 00351 Len -= ChunkLen; 00352 Offset += ChunkLen; 00353 Msg.Cmd.Offset = Offset; 00354 ExtCtrl.pTxPayload += ChunkLen; 00355 ChunkLen = (_u16)sl_min(MAX_NVMEM_CHUNK_SIZE,Len); 00356 ExtCtrl.TxPayloadLen = ChunkLen; 00357 Msg.Cmd.Len = ChunkLen; 00358 Msg.Cmd.FileHandle = (_u32)FileHdl; 00359 } 00360 else 00361 { 00362 return RetVal; 00363 } 00364 }while(ChunkLen > 0); 00365 00366 return (_i32)RetCount; 00367 } 00368 #endif 00369 00370 /*****************************************************************************/ 00371 /* sl_FsGetInfo */ 00372 /*****************************************************************************/ 00373 typedef union 00374 { 00375 _FsGetInfoCommand_t Cmd; 00376 _FsGetInfoResponse_t Rsp; 00377 }_SlFsGetInfoMsg_u; 00378 00379 00380 #if _SL_INCLUDE_FUNC(sl_FsGetInfo) 00381 00382 00383 static const _SlCmdCtrl_t _SlFsGetInfoCmdCtrl = 00384 { 00385 SL_OPCODE_NVMEM_FILEGETINFOCOMMAND, 00386 (_SlArgSize_t)sizeof(_FsGetInfoCommand_t), 00387 (_SlArgSize_t)sizeof(_FsGetInfoResponse_t) 00388 }; 00389 00390 _i16 sl_FsGetInfo(const _u8 *pFileName,const _u32 Token,SlFsFileInfo_t* pFsFileInfo) 00391 { 00392 _SlFsGetInfoMsg_u Msg; 00393 _SlCmdExt_t CmdExt; 00394 00395 /* verify no erorr handling in progress. if in progress than 00396 ignore the API execution and return immediately with an error */ 00397 VERIFY_NO_ERROR_HANDLING_IN_PROGRESS(); 00398 00399 CmdExt.TxPayloadLen = (_u16)((_sl_Strlen(pFileName)+4) & (~3)); /* add 4: 1 for NULL and the 3 for align */ 00400 CmdExt.RxPayloadLen = 0; 00401 CmdExt.pTxPayload = (_u8*)pFileName; 00402 CmdExt.pRxPayload = NULL; 00403 Msg.Cmd.Token = Token; 00404 00405 VERIFY_RET_OK(_SlDrvCmdOp((_SlCmdCtrl_t *)&_SlFsGetInfoCmdCtrl, &Msg, &CmdExt)); 00406 00407 pFsFileInfo->flags = Msg.Rsp.flags; 00408 pFsFileInfo->FileLen = Msg.Rsp.FileLen; 00409 pFsFileInfo->AllocatedLen = Msg.Rsp.AllocatedLen; 00410 pFsFileInfo->Token[0] = Msg.Rsp.Token[0]; 00411 pFsFileInfo->Token[1] = Msg.Rsp.Token[1]; 00412 pFsFileInfo->Token[2] = Msg.Rsp.Token[2]; 00413 pFsFileInfo->Token[3] = Msg.Rsp.Token[3]; 00414 return (_i16)((_i16)Msg.Rsp.Status); 00415 } 00416 #endif 00417 00418 /*****************************************************************************/ 00419 /* sl_FsDel */ 00420 /*****************************************************************************/ 00421 typedef union 00422 { 00423 _FsDeleteCommand_t Cmd; 00424 _FsDeleteResponse_t Rsp; 00425 }_SlFsDeleteMsg_u; 00426 00427 00428 #if _SL_INCLUDE_FUNC(sl_FsDel) 00429 00430 static const _SlCmdCtrl_t _SlFsDeleteCmdCtrl = 00431 { 00432 SL_OPCODE_NVMEM_FILEDELCOMMAND, 00433 (_SlArgSize_t)sizeof(_FsDeleteCommand_t), 00434 (_SlArgSize_t)sizeof(_FsDeleteResponse_t) 00435 }; 00436 00437 _i16 sl_FsDel(const _u8 *pFileName,const _u32 Token) 00438 { 00439 _SlFsDeleteMsg_u Msg; 00440 _SlCmdExt_t CmdExt; 00441 00442 /* verify no erorr handling in progress. if in progress than 00443 ignore the API execution and return immediately with an error */ 00444 VERIFY_NO_ERROR_HANDLING_IN_PROGRESS(); 00445 CmdExt.TxPayloadLen = (_u16)((_sl_Strlen(pFileName)+4) & (~3)); /* add 4: 1 for NULL and the 3 for align */ 00446 CmdExt.RxPayloadLen = 0; 00447 CmdExt.pTxPayload = (_u8*)pFileName; 00448 CmdExt.pRxPayload = NULL; 00449 Msg.Cmd.Token = Token; 00450 00451 00452 VERIFY_RET_OK(_SlDrvCmdOp((_SlCmdCtrl_t *)&_SlFsDeleteCmdCtrl, &Msg, &CmdExt)); 00453 00454 return (_i16)((_i16)Msg.Rsp.status); 00455 } 00456 #endif
Generated on Tue Jul 12 2022 12:06:48 by
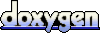