
DeepCover Embedded Security in IoT: Public-key Secured Data Paths
Dependencies: MaximInterface
Graphic.hpp
00001 /******************************************************************************* 00002 * Copyright (C) Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 00033 #ifndef GRAPHIC_HPP 00034 #define GRAPHIC_HPP 00035 00036 #include <stddef.h> 00037 #include <vector> 00038 #include "Keys.hpp" 00039 00040 class Bitmap; 00041 00042 /// @brief Base class for all graphical elements. 00043 /// @details 00044 /// Includes unique parent-child relationships for creating trees of graphical 00045 /// objects. 00046 class Graphic { 00047 public: 00048 typedef std::vector<Graphic *> ChildContainer; 00049 00050 Graphic(); 00051 virtual ~Graphic(); 00052 00053 /// @name Parent 00054 /// @brief 00055 /// Get or set the parent graphic of this graphic. Set to NULL if this graphic 00056 /// has no parent. 00057 /// @{ 00058 00059 Graphic * parent() { return parent_; } 00060 00061 const Graphic * parent() const { return parent_; } 00062 00063 /// @note Adds this graphic to the parent's list of children. 00064 /// @sa childrenChanged 00065 void setParent (Graphic * parent); 00066 00067 /// @} 00068 00069 /// @brief List of child graphics for this parent. 00070 /// @note 00071 /// Children should be added and removed by calling setParent. Children will 00072 /// be removed automatically when they are destroyed. 00073 const ChildContainer & children() const { return children_; } 00074 00075 /// @brief Check if this graphic is focused. 00076 /// @returns True if focused. 00077 bool focused() const; 00078 00079 /// @brief Set this graphic as the focused graphic. 00080 /// @details 00081 /// The focused graphic is the first to receive input events such as key 00082 /// presses. 00083 /// @sa focusChanged 00084 void setFocused(); 00085 00086 /// @brief Coordinates of this graphic in pixels. 00087 /// @details 00088 /// Coordinates are relative to the top-left corner of the parent graphic. 00089 /// @{ 00090 00091 int x() const { return x_; } 00092 int y() const { return y_; } 00093 00094 /// @} 00095 00096 /// @brief Displayed dimensions of this graphic in pixels. 00097 /// @{ 00098 00099 int width() const { return width_; } 00100 int height() const { return height_; } 00101 00102 /// @} 00103 00104 /// @brief Move graphic to a new location measured in pixels. 00105 /// @details 00106 /// Coordinates are relative to the top-left corner of the parent graphic. 00107 /// @sa moved 00108 void move(int x, int y); 00109 00110 /// @brief 00111 /// Resize graphic to a new size measure in pixels. Minimum width and height 00112 /// is 1. 00113 /// @sa resized 00114 void resize(int width, int height); 00115 00116 /// @brief Render this graphic onto a bitmap. 00117 /// @{ 00118 00119 void render(Bitmap & bitmap, int xOffset, int yOffset) const; 00120 void render(Bitmap & bitmap) const; 00121 00122 /// @} 00123 00124 /// @brief 00125 /// Update this graphic and all child graphics. Checks if graphic has been 00126 /// invalidated and should be redrawn. 00127 /// @param canvas Canvas used for rendering. May be set to NULL to defer redraw. 00128 /// @returns True if the canvas has been updated. 00129 /// @sa updated 00130 bool update(Bitmap * canvas); 00131 00132 /// @brief Process a key-press input event. 00133 /// @details 00134 /// The event will first be directed to the focused graphic. Processing will 00135 /// proceed to each parent graphic until it has been handled. 00136 /// @sa doProcessKey 00137 /// @returns True if the key event was handled. 00138 bool processKey(Key key); 00139 00140 protected: 00141 /// @brief Mark the visual region as invalid. 00142 /// @note Indicates a redraw is necessary during next update. 00143 void invalidate() { valid_ = false; } 00144 00145 /// Event handler for when a child is added or removed. 00146 virtual void childrenChanged(); 00147 00148 /// @brief Event handler for when this graphic has been focused or unfocused. 00149 /// @param focused True if focused or false if unfocused. 00150 virtual void focusChanged(bool focused); 00151 00152 /// Event handler for when this graphic has been moved. 00153 virtual void moved(); 00154 00155 /// Event handler for when this graphic has been resized. 00156 virtual void resized(); 00157 00158 /// Event handler for when this graphic has been updated. 00159 virtual void updated(); 00160 00161 /// @brief Render this graphic onto a bitmap. 00162 /// @details The default implementation renders each child in order. 00163 virtual void doRender(Bitmap & bitmap, int xOffset, int yOffset) const; 00164 00165 /// @brief Process a key-press input event. 00166 /// @returns 00167 /// True if the key event was handled. False if the key event should be 00168 /// propagated. 00169 virtual bool doProcessKey(Key); 00170 00171 private: 00172 Graphic * parent_; 00173 ChildContainer children_; 00174 Graphic * focusedChild_; 00175 int x_; 00176 int y_; 00177 int width_; 00178 int height_; 00179 bool valid_; 00180 00181 void updateAll(); 00182 bool redrawInvalid(Bitmap & canvas); 00183 void setAllValid(); 00184 00185 // Uncopyable 00186 Graphic(const Graphic &); 00187 const Graphic & operator=(const Graphic &); 00188 }; 00189 00190 #endif
Generated on Tue Jul 12 2022 12:06:48 by
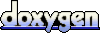