Library for MAX14871 Shield, MAXREFDES89#
Dependents: MAXREFDES89_MAX14871_Shield_Demo MAXREFDES89_Test_Program Line_Following_Bot Line_Following_Bot_Pololu
max14871_shield.h
00001 /******************************************************************//** 00002 * @file max14871_shield.h 00003 * Copyright (C) 2015 Maxim Integrated Products, Inc., All Rights Reserved. 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a 00006 * copy of this software and associated documentation files (the "Software"), 00007 * to deal in the Software without restriction, including without limitation 00008 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00009 * and/or sell copies of the Software, and to permit persons to whom the 00010 * Software is furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included 00013 * in all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00016 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00017 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00018 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00019 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00020 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00021 * OTHER DEALINGS IN THE SOFTWARE. 00022 * 00023 * Except as contained in this notice, the name of Maxim Integrated 00024 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00025 * Products, Inc. Branding Policy. 00026 * 00027 * The mere transfer of this software does not imply any licenses 00028 * of trade secrets, proprietary technology, copyrights, patents, 00029 * trademarks, maskwork rights, or any other form of intellectual 00030 * property whatsoever. Maxim Integrated Products, Inc. retains all 00031 * ownership rights. 00032 **********************************************************************/ 00033 00034 00035 #ifndef MAX14871_SHIELD_H 00036 #define MAX14871_SHIELD_H 00037 00038 #include "mbed.h" 00039 #include "max7300.h" 00040 #include "max5387.h" 00041 00042 00043 /** 00044 * @brief MAX14871 Full-Bridge DC Motor Driver MBED Shield Library 00045 * 00046 * @details The MAXREFDES89# provides an ideal interface for anyone 00047 * developing with brushed DC motors. The design is an 00048 * mbed®-compatible, Arduino® form factor shield for the rapid 00049 * development of brushed DC motor applications. The shield contains 00050 * four MAX14871 full-bridge DC motor drivers for driving up to 4 00051 * motors. A MAX17501 DC-DC converter allows the system to operate 00052 * from a single 7 to 36VDC power supply. Four MAX4704 4:1 00053 * multiplexers permit setting the current regulation mode of the 00054 * MAX14871, while two MAX5387 digital potentiometers provide the 00055 * ability to set the motor current limit. A MAX7300 GPIO expander 00056 * supports interfacing each motor driver circuit to the 00057 * mbed-enabled microcontroller platform. 00058 * 00059 * @code 00060 * @endcode 00061 */ 00062 class Max14871_Shield 00063 { 00064 public: 00065 00066 /** 00067 * @brief Motor driver channels 00068 * @details Simple enumeration of channels available on the shield 00069 */ 00070 typedef enum 00071 { 00072 MD1 = 1, 00073 MD2, 00074 MD3, 00075 MD4 00076 }max14871_motor_driver_t; 00077 00078 00079 /** 00080 * @brief Operating modes 00081 * @details Motor operating modes offered on each channel 00082 */ 00083 typedef enum 00084 { 00085 COAST = 1, 00086 BRAKE, 00087 REVERSE, 00088 FORWARD 00089 }max14871_operating_mode_t; 00090 00091 00092 /** 00093 * @brief Current regulation modes 00094 * @details Three different current regulation modes offered with 00095 * either an internal or external reference for setting the regulation limit. 00096 * This is not the fault current which is fixed, see datasheet. 00097 */ 00098 typedef enum 00099 { 00100 RIPPLE_25_INTERNAL_REF = 1, 00101 RIPPLE_25_EXTERNAL_REF, 00102 TCOFF_FAST_INTERNAL_REF, 00103 TCOFF_SLOW_INTERNAL_REF, 00104 TCOFF_FAST_EXTERNAL_REF, 00105 TCOFF_SLOW_EXTERNAL_REF 00106 }max14871_current_regulation_mode_t; 00107 00108 00109 /**********************************************************//** 00110 * @brief Constructor for Max14871_Shield Class. 00111 * 00112 * @details Allows user to use existing I2C object 00113 * 00114 * On Entry: 00115 * @param[in] i2c_bus - pointer to existing I2C object 00116 * @param[in] default_config - true if board uses default 00117 * pwm channels and I2C addressing 00118 * 00119 * On Exit: 00120 * 00121 * @return None 00122 **************************************************************/ 00123 Max14871_Shield(I2C *i2c_bus, bool default_config = true); 00124 00125 00126 /**********************************************************//** 00127 * @brief Constructor for Max14871_Shield Class. 00128 * 00129 * @details Allows user to create a new I2C object if not 00130 * already using one 00131 * 00132 * On Entry: 00133 * @param[in] sda - sda pin of I2C bus 00134 * @param[in] scl - scl pin of I2C bus 00135 * @param[in] default_config - true if board uses default 00136 * pwm channels and I2C addressing 00137 * 00138 * On Exit: 00139 * 00140 * @return None 00141 **************************************************************/ 00142 Max14871_Shield(PinName sda, PinName scl, bool default_config = true); 00143 00144 00145 /**********************************************************//** 00146 * @brief Default destructor for Max14871_Shield Class. 00147 * 00148 * @details Destroys I2C object if owner 00149 * 00150 * On Entry: 00151 * 00152 * On Exit: 00153 * 00154 * @return None 00155 **************************************************************/ 00156 ~Max14871_Shield(); 00157 00158 00159 /**********************************************************//** 00160 * @brief Set the operating mode of the motor driver 00161 * 00162 * @details Configures the /EN and DIR pins of the motor driver 00163 * via the MAX7300 GPIO Expander 00164 * 00165 * On Entry: 00166 * @param[in] md - 1 of 4 motor drivers on the shield 00167 * @param[in] mode - 1 of 4 operating modes of the motor driver 00168 * 00169 * On Exit: 00170 * 00171 * @return 0 on success, non-0 on failure 00172 **************************************************************/ 00173 int16_t set_operating_mode(max14871_motor_driver_t md, 00174 max14871_operating_mode_t mode); 00175 00176 00177 /**********************************************************//** 00178 * @brief 00179 * 00180 * @details 00181 * 00182 * On Entry: 00183 * @param[in] md - 1 of 4 motor drivers on the shield 00184 * @param[in] mode - 1 of 6 current regulation modes of the 00185 * motor driver 00186 * @param[in] vref - sets maximum motor current, Max of 2.0V 00187 * 00188 * On Exit: 00189 * 00190 * @return 0 on success, non-0 on failure 00191 **************************************************************/ 00192 int16_t set_current_regulation_mode (max14871_motor_driver_t md, 00193 max14871_current_regulation_mode_t mode, 00194 float vref = 1.0); 00195 00196 00197 /**********************************************************//** 00198 * @brief Sets pwm channel for given motor driver 00199 * 00200 * @details Must use default, or alternate channel for specific 00201 * motor driver. Function allows for mix of default and 00202 * alternates for each motor driver vs all default or 00203 * all alternate. 00204 * 00205 * On Entry: 00206 * @param[in] md - 1 of 4 motor drivers on the shield 00207 * @param[in] ch - PWM channel using Arduino naming convention 00208 * 00209 * On Exit: 00210 * 00211 * @return 0 on success, non-0 on failure 00212 **************************************************************/ 00213 int16_t set_pwm_channel(max14871_motor_driver_t md, PinName ch); 00214 00215 00216 /**********************************************************//** 00217 * @brief Sets period of pwm signal for selected motor driver 00218 * 00219 * @details period must be in micro-seconds 00220 * 00221 * On Entry: 00222 * @param[in] md - 1 of 4 motor drivers on the shield 00223 * @param[in] period - PWM period specified in seconds 00224 * 00225 * On Exit: 00226 * 00227 * @return 0 on success, non-0 on failure 00228 **************************************************************/ 00229 int16_t set_pwm_period(max14871_motor_driver_t md, float period); 00230 00231 00232 /**********************************************************//** 00233 * @brief Sets duty cycle of pwm signal for selected motor driver 00234 * 00235 * @details duty cycle must be in micro-seconds 00236 * 00237 * On Entry: 00238 * @param[in] md - 1 of 4 motor drivers on the shield 00239 * @param[in] duty_cycle - duty cycle of the pwm signal specified 00240 * as a percentage 00241 * 00242 * On Exit: 00243 * 00244 * @return 0 on success, non-0 on failure 00245 **************************************************************/ 00246 int16_t set_pwm_duty_cycle(max14871_motor_driver_t md, float duty_cycle); 00247 00248 00249 /**********************************************************//** 00250 * @brief Get operating mode of selected motor driver 00251 * 00252 * @details 00253 * 00254 * On Entry: 00255 * @param[in] md - 1 of 4 motor drivers on the shield 00256 * 00257 * On Exit: 00258 * 00259 * @return Operating mode of selected motor driver 00260 **************************************************************/ 00261 max14871_operating_mode_t get_operating_mode(max14871_motor_driver_t md); 00262 00263 00264 /**********************************************************//** 00265 * @brief Get current regulation mode of selected motor driver 00266 * 00267 * @details 00268 * 00269 * On Entry: 00270 * @param[in] md - 1 of 4 motor drivers on the shield 00271 * 00272 * On Exit: 00273 * 00274 * @return Current regulation mode of selected motor driver 00275 **************************************************************/ 00276 max14871_current_regulation_mode_t get_current_regulation_mode(max14871_motor_driver_t md); 00277 00278 00279 /**********************************************************//** 00280 * @brief Get duty cycle of selected motor driver 00281 * 00282 * @details 00283 * 00284 * On Entry: 00285 * @param[in] md - 1 of 4 motor drivers on the shield 00286 * 00287 * On Exit: 00288 * 00289 * @return Duty cycle of selected motor driver 00290 **************************************************************/ 00291 float get_pwm_duty_cycle(max14871_motor_driver_t md); 00292 00293 00294 /**********************************************************//** 00295 * @brief Get pwm period of selected motor driver 00296 * 00297 * @details 00298 * 00299 * On Entry: 00300 * @param[in] md - 1 of 4 motor drivers on the shield 00301 * 00302 * On Exit: 00303 * 00304 * @return pwm period of selected motor driver 00305 **************************************************************/ 00306 float get_pwm_period(max14871_motor_driver_t md); 00307 00308 00309 /**********************************************************//** 00310 * @brief Get external voltage reference of selected motor driver 00311 * 00312 * @details 00313 * 00314 * On Entry: 00315 * @param[in] md - 1 of 4 motor drivers on the shield 00316 * 00317 * On Exit: 00318 * 00319 * @return External voltage reference of selected motor driver 00320 **************************************************************/ 00321 float get_external_voltage_ref(max14871_motor_driver_t md); 00322 00323 00324 private: 00325 00326 struct motor_data_s 00327 { 00328 max14871_operating_mode_t op_mode; 00329 max14871_current_regulation_mode_t i_reg_mode; 00330 float duty_cycle; 00331 float period; 00332 float v_ref; 00333 }; 00334 00335 struct motor_data_s _motor_data_array[4]; 00336 00337 I2C *_p_i2c; 00338 Max7300 *_p_io_expander; 00339 Max5387 *_p_digi_pot1; 00340 Max5387 *_p_digi_pot2; 00341 PwmOut *_p_pwm1; 00342 PwmOut *_p_pwm2; 00343 PwmOut *_p_pwm3; 00344 PwmOut *_p_pwm4; 00345 00346 bool _i2c_owner; 00347 00348 void init_board(void); 00349 }; 00350 00351 #endif/* MAX14871_SHIELD_H */
Generated on Thu Jul 14 2022 04:04:30 by
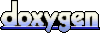