AKM AK9752 Ultra-small IR Sensor IC with I2C I/F
Embed:
(wiki syntax)
Show/hide line numbers
AK9752.h
00001 /************************************* 00002 * AK9752 00003 * AKM Ultra-small IR Sensor IC with I2C I/F 00004 **/ 00005 #ifndef _AK9752_H_ 00006 #define _AK9752_H_ 00007 #include "mbed.h" 00008 00009 class AK9752 00010 { 00011 public: 00012 /** 00013 * AK9752 constructor 00014 * 00015 * @param sda SDA pin 00016 * @param sdl SCL pin 00017 * @param addr addr of the I2C peripheral 00018 */ 00019 AK9752(PinName sda, PinName scl, int addr); 00020 00021 /** 00022 * AK9752 destructor 00023 */ 00024 ~AK9752(); 00025 00026 uint8_t getCompanyCode(void) ; /* 0x48 expected */ 00027 uint8_t getDeviceID(void) ; /* 0x14 expected */ 00028 bool dataReady(void) ; /* returns ST1[0], read ST2 to clear */ 00029 uint8_t getIntcause(void) ; /* get REG_INTCAUSE (0x05) */ 00030 int16_t getRawIR(void) ; /* raw data, must be mulitplied by 0.4578 for pA */ 00031 float getIR(void) ; 00032 int16_t getRawTMP(void) ; /* raw data, must be multiplied by 0.0019837 and + 25 */ 00033 float getTMP(void) ; 00034 bool dataOverRun(void) ; /* check data over run and clear data ready */ 00035 void getTHIR(int16_t *high, int16_t *low) ; 00036 void setTHIR(int16_t high, int16_t low) ; 00037 void getTHTMP(int16_t *high, int16_t *low) ; 00038 void setTHTMP(int16_t high, int16_t low) ; 00039 uint8_t getINTEN(void) ; 00040 void setINTEN(uint8_t value) ; 00041 uint8_t getCNTL1(void) ; 00042 void setCNTL1(uint8_t value) ; 00043 uint8_t getCNTL2(void) ; 00044 void setCNTL2(uint8_t value) ; 00045 void software_reset(void) ; 00046 00047 private: 00048 I2C m_i2c; 00049 int m_addr; 00050 int readRegs(int addr, uint8_t * data, int len); 00051 int writeRegs(uint8_t * data, int len); 00052 }; 00053 00054 #endif /* _AK9752_H_ */
Generated on Tue Jul 12 2022 22:52:49 by
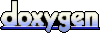