
working - MUX,3cannel,Reset
Dependencies: MAX30003 max32630fthr DS1307
main.cpp
00001 00002 #include "MAX30003.h" 00003 #include "mbed.h" 00004 #include "max32630fthr.h" 00005 #include "ds1307.h" 00006 //#include <BufferedSerial.h> 00007 #include <string> 00008 //#include <Serial.h> 00009 00010 #define TARGET_TX_PIN P3_1 00011 #define TARGET_RX_PIN P3_0 00012 00013 00014 //Timer timer_fast; 00015 Timer t; 00016 MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00017 00018 void task_fast(void); 00019 //DigitalOut ledA(LED2); 00020 00021 DigitalOut S0(P4_0); 00022 DigitalOut S1(P5_6); 00023 //DigitalOut S2(P7_2); 00024 //DigitalOut S3(P7_2); 00025 00026 //channel feedback 00027 DigitalIn A(P6_0); //P17 00028 DigitalIn B(P3_5); //P18 00029 DigitalIn C(P3_4); //P19 00030 00031 00032 void ecg_config(MAX30003 &ecgAFE); 00033 //BufferedSerial pc(P3_1,P3_0); // Use USB debug probe for serial link static Unbuffered 00034 static BufferedSerial pc(TARGET_TX_PIN, TARGET_RX_PIN, 230400); // 230400 works well 00035 00036 //Serial uart_1(USBTX, USBRX); // Use USB debug probe for serial link static Unbuffered 00037 // 00038 //Serial pc(P3_1,P3_0); 00039 volatile bool ecgFIFOIntFlag = 0; 00040 volatile bool timerflag = 0; 00041 00042 FileHandle *mbed::mbed_override_console(int fd) 00043 { 00044 return &pc; 00045 } 00046 00047 void ecgFIFO_callback_1() { // Triggered when the ECG FIFO is about to be full 00048 00049 ecgFIFOIntFlag = 1; 00050 } 00051 00052 time_t asUnixTime(int year, int mon, int mday, int hour, int min, int sec) { 00053 struct tm t; 00054 t.tm_year = year - 1900; 00055 t.tm_mon = mon - 1; // convert to 0 based month 00056 t.tm_mday = mday; 00057 t.tm_hour = hour; 00058 t.tm_min = min; 00059 t.tm_sec = sec; 00060 t.tm_isdst = -1; // Is Daylight saving time on? 1 = yes, 0 = no, -1 = unknown 00061 00062 return mktime(&t); // returns seconds elapsed since January 1, 1970 (begin of the Epoch) 00063 } 00064 int main(void) 00065 { 00066 uint16_t tic=0; 00067 00068 /* S1S0 00069 00 - C0 00070 01 - C1 00071 10 - C2 00072 11 - C3 */ 00073 00074 00075 S0=0; //C0 00076 S1=0; 00077 // S2=0; 00078 // S3=0; 00079 00080 00081 00082 // Constants 00083 const int EINT_STATUS_MASK = 1 << 23; 00084 const int FIFO_OVF_MASK = 0x7; 00085 const int FIFO_VALID_SAMPLE_MASK = 0x0; 00086 const int FIFO_FAST_SAMPLE_MASK = 0x1; 00087 const int ETAG_BITS_MASK = 0x7; 00088 00089 // timer_fast.start(); 00090 DigitalOut rLed(LED2, LED_ON); 00091 // pc.baud(9600); 00092 00093 //pc.set_baud(115200); // Baud rate = 115200 00094 //pc.set_format( 00095 // /* bits */ 8, 00096 // /* parity */ BufferedSerial::None, 00097 // /* stop bit */ 1 //1 00098 //); 00099 //uart_1.baud(115200); 00100 00101 //--------------------------------- ECG Initialization ---------------------------------------------// 00102 InterruptIn ecgFIFO_int(P5_4); // Config P5_4 as int. in for the 00103 ecgFIFO_int.fall(&ecgFIFO_callback_1); // ecg FIFO interrupt at falling edge 00104 00105 SPI spiBus(SPI2_MOSI, SPI2_MISO, SPI2_SCK); // SPI bus, P5_1 = MOSI, 00106 // P5_2 = MISO, P5_0 = SCK 00107 00108 MAX30003 ecgAFE(spiBus, P5_3); // New MAX30003 on spiBus, CS = P5_3 00109 00110 ecg_config(ecgAFE); // Config ECG 00111 00112 00113 ecgAFE.writeRegister( MAX30003::SYNCH , 0); 00114 00115 //-------------------------------------------------------------------------------------------------// 00116 00117 uint32_t ecgFIFO, readECGSamples, idx, ETAG[32], status; 00118 int16_t ecgSample[32]; 00119 //bool timerflag = false; 00120 int16_t ecgSample_1sec[256]; 00121 // uint8_t ecg_1 = 0; 00122 // uint8_t ecg_2 = 0; 00123 uint16_t onesec_counter = 0; 00124 uint16_t onesec_counter_temp = 0; 00125 00126 // int16_t sample = 300; 00127 // uint8_t final[10]; 00128 00129 uint16_t checksum_ = 0; 00130 uint16_t mod_checksum = 0; 00131 00132 uint8_t p_1 = 0; 00133 uint8_t p_2 = 0; 00134 uint8_t p_3 = 0; 00135 uint8_t p_4 = 0; 00136 // uint8_t channel_num= 1; 00137 uint8_t channel_num[1]= {0}; 00138 uint8_t data_len_1 = 0; 00139 uint8_t data_len_2 = 0; 00140 uint32_t packet_1 = 0; 00141 uint8_t cksm_1 = 0; 00142 uint8_t cksm_2 = 0; 00143 uint8_t header_device_id[3] = {0,0,210}; 00144 //uint8_t header_device_id[3] = {0,0,1}; //for rahul 00145 uint8_t header_packet_type[2] = {0,2}; 00146 00147 uint8_t ending[5] = {'@','#','%','!','7'}; 00148 00149 bool flip = true; 00150 int sampleps = 0; 00151 00152 char buf[20]; 00153 // pc.write("Welcome",8*sizeof(char)); 00154 // printf("In the main loop"); //printf("In the main loop"); 00155 bool flag_first = false; 00156 bool timestamp_reader = false; 00157 00158 00159 //---------------------------------software RTC Start---------------------------------------------// 00160 do 00161 { 00162 if (pc.readable()) 00163 { 00164 scanf("%s",buf); 00165 //printf("The entered string is %s : \n ",buf);*/ 00166 // buf[20] = 1621297647; 00167 packet_1 = atoi(buf); 00168 set_time(packet_1); 00169 00170 if ((packet_1 % 60) == 0) 00171 { 00172 for (int u = 0;u<3;u++) 00173 { 00174 if (pc.readable()) 00175 { 00176 scanf("%s",buf); 00177 printf("Entered the minute string %s : \n ",buf); 00178 00179 packet_1 = atoi(buf); 00180 set_time(packet_1); 00181 } 00182 } 00183 timestamp_reader = true; 00184 } 00185 else 00186 { 00187 //printf("The timestamp is not divisible by 60 \n"); 00188 } 00189 flag_first = true; 00190 00191 } 00192 00193 } while (timestamp_reader==false); // take the third timestamp 00194 00195 //---------------------------------software RTC End---------------------------------------------// 00196 00197 00198 while(1) 00199 { 00200 00201 00202 if ((onesec_counter>=112)) 00203 { 00204 tic++; 00205 00206 pc.write((uint8_t *)header_device_id, sizeof(header_device_id)); // device ID 00207 00208 pc.write((uint8_t *)header_packet_type, sizeof(header_packet_type)); // packet type 00209 00210 time_t seconds = time(NULL); 00211 packet_1 = seconds; 00212 00213 //time_t seconds = time(NULL); // if remove it the timestamp will be static 00214 //packet_1 ++; 00215 // printf("Time as seconds since January 1, 1970 = %d\n", packet_1); 00216 p_1 = packet_1 & 0xff; 00217 p_2 = (packet_1 >> 8) & 0xff; 00218 p_3 = (packet_1 >> 16) & 0xff; 00219 p_4 = (packet_1 >> 24) & 0xff; 00220 // checksum_ = checksum_ + (packet_1 & 0xffff) + ((packet_1 >> 16) & 0xffff) ; 00221 checksum_ = checksum_ + p_1 + p_2 + p_3 + p_4 ; 00222 uint8_t header_packet_id[4] = {p_4,p_3,p_2,p_1}; 00223 pc.write((uint8_t *)header_packet_id, sizeof(header_packet_id)); // packet ID 00224 00225 00226 pc.write((uint8_t *)channel_num, sizeof(channel_num)); 00227 00228 onesec_counter_temp = onesec_counter * 2; 00229 data_len_1 = onesec_counter_temp & 0xff; 00230 data_len_2 = (onesec_counter_temp >> 8) & 0xff; 00231 checksum_ = checksum_ + data_len_1 + data_len_2; 00232 //pc.write((uint32_t *)packet_1, sizeof(packet_1)); 00233 uint8_t header_ecg_datalen[2] = {data_len_2,data_len_1}; 00234 pc.write((uint8_t *)header_ecg_datalen, sizeof(header_ecg_datalen)); 00235 00236 mod_checksum = checksum_ % 65536 ; 00237 cksm_1 = mod_checksum & 0xff; 00238 cksm_2 = (mod_checksum >> 8) & 0xff; 00239 uint8_t header_ecg_checksum[2] = {cksm_2,cksm_1}; 00240 pc.write((uint8_t *)header_ecg_checksum, sizeof(header_ecg_checksum)); 00241 00242 pc.write((int16_t *)ecgSample_1sec,onesec_counter * sizeof(int16_t)); 00243 // printf("Samples per second %d \n", (onesec_counter)); 00244 onesec_counter = 0; 00245 // memset(ecgSample_1sec, 0, sizeof(ecgSample_1sec)); 00246 00247 pc.write((uint8_t *)ending, sizeof(ending)); 00248 checksum_ = 0; 00249 //t.stop(); 00250 // auto us = t.elapsed_time().count(); 00251 // float time_taken = us/1000000; 00252 // printf("Timer time: %lu ms \n", (time_taken*100)); 00253 // t.reset(); 00254 // timer_fast.reset(); 00255 00256 00257 if (tic==10) //C1 00258 { 00259 S0=1; 00260 S1=0; 00261 // S2=0; 00262 // S3=0; 00263 channel_num[0]= {1}; 00264 } 00265 if (tic==20) //C2 00266 { 00267 S0=0; 00268 S1=1; 00269 // S2=0; 00270 // S3=0; 00271 channel_num[0]= {2}; 00272 } 00273 00274 if (tic==30) // C0 00275 { 00276 S0=0; 00277 S1=0; 00278 // S2=0; 00279 // S3=0; 00280 channel_num[0]= {0}; 00281 tic=0;} 00282 00283 00284 // for channel selection 00285 00286 if (A==0) // 00287 {tic=50; 00288 S0=0; 00289 S1=0; 00290 // S2=0; 00291 // S3=0; 00292 channel_num[0]= {0};} 00293 00294 if (B==0) // 00295 {tic=60; 00296 S0=1; 00297 S1=0; 00298 // S2=0; 00299 // S3=0; 00300 channel_num[0]= {1};} 00301 00302 if (C==0) // 00303 {tic=70; 00304 S0=0; 00305 S1=1; 00306 // S2=0; 00307 // S3=0; 00308 channel_num[0]= {2};} 00309 00310 00311 00312 00313 00314 00315 00316 00317 00318 } 00319 // Read back ECG samples from the FIFO 00320 else if((ecgFIFOIntFlag==1))// && (timerflag == 0)) 00321 { 00322 00323 ecgFIFOIntFlag = 0; 00324 status = ecgAFE.readRegister( MAX30003::STATUS ); // Read the STATUS register 00325 00326 // Check if EINT interrupt asserted 00327 if ( ( status & EINT_STATUS_MASK ) == EINT_STATUS_MASK ) 00328 { 00329 00330 readECGSamples = 0; // Reset sample counter 00331 00332 do { 00333 ecgFIFO = ecgAFE.readRegister( MAX30003::ECG_FIFO ); // Read FIFO 00334 ecgSample[readECGSamples] = ecgFIFO >> 8; // Isolate voltage data 00335 ecgSample[readECGSamples] = ((ecgSample[readECGSamples]<<8)&0xFF00)|((ecgSample[readECGSamples]>>8)&0x00FF); 00336 ETAG[readECGSamples] = ( ecgFIFO >> 3 ) & ETAG_BITS_MASK; // Isolate ETAG 00337 readECGSamples++; // Increment sample counter 00338 00339 // Check that sample is not last sample in FIFO 00340 } while ( ETAG[readECGSamples-1] == FIFO_VALID_SAMPLE_MASK || 00341 ETAG[readECGSamples-1] == FIFO_FAST_SAMPLE_MASK ); 00342 00343 // Check if FIFO has overflowed 00344 if( ETAG[readECGSamples - 1] == FIFO_OVF_MASK ) 00345 { 00346 ecgAFE.writeRegister( MAX30003::FIFO_RST , 0); // Reset FIFO 00347 rLed = 1;//notifies the user that an over flow occured 00348 } 00349 //uint8_t header_ecg_checksum[2] = {'%','%'}; 00350 //pc.write((uint8_t *)header_ecg_checksum, sizeof(header_ecg_checksum)); 00351 00352 //pc.write((int16_t *)ecgSample,readECGSamples * sizeof(int16_t )); 00353 //memcpy(ecgSample_1sec , ecgSample, sizeof(ecgSample)); 00354 00355 //memcpy(ecgSample_1sec + (onesec_counter * sizeof(int16_t)), ecgSample, sizeof(ecgSample)); 00356 00357 if (flip) 00358 { 00359 sampleps = 12; 00360 //printf("Flipped 12\r\n"); 00361 } 00362 else 00363 { 00364 sampleps = 13; 00365 // printf("Flipped 13\r\n"); 00366 } 00367 00368 for( idx = 0; idx < sampleps; idx++ ) 00369 { 00370 //pc.printf("%6d\r\n", ecgSample[idx]); 00371 ecgSample_1sec[onesec_counter] = ecgSample[idx]; 00372 00373 cksm_1 = ecgSample[idx] & 0xff; 00374 cksm_2 = (ecgSample[idx] >> 8) & 0xff; 00375 checksum_ += cksm_1 + cksm_2; 00376 onesec_counter++; 00377 } 00378 flip =!flip; 00379 rLed = ! rLed; 00380 } 00381 00382 } 00383 } 00384 } 00385 00386 00387 00388 00389 void ecg_config(MAX30003& ecgAFE) { 00390 00391 // Reset ECG to clear registers 00392 ecgAFE.writeRegister( MAX30003::SW_RST , 0); 00393 00394 // General config register setting 00395 MAX30003::GeneralConfiguration_u CNFG_GEN_r; 00396 CNFG_GEN_r.bits.en_ecg = 1; // Enable ECG channel 00397 CNFG_GEN_r.bits.rbiasn = 1; // Enable resistive bias on negative input 00398 CNFG_GEN_r.bits.rbiasp = 1; // Enable resistive bias on positive input 00399 CNFG_GEN_r.bits.en_rbias = 1; // Enable resistive bias 00400 CNFG_GEN_r.bits.imag = 2; // Current magnitude = 10nA 00401 CNFG_GEN_r.bits.en_dcloff = 1; // Enable DC lead-off detection 00402 //CNFG_GEN_r.bits.fmstr = 1; //125 sps FMSTR 1 00403 ecgAFE.writeRegister( MAX30003::CNFG_GEN , CNFG_GEN_r.all); 00404 00405 00406 // ECG Config register setting 00407 MAX30003::ECGConfiguration_u CNFG_ECG_r; 00408 CNFG_ECG_r.bits.dlpf = 1; // Digital LPF cutoff = 40Hz 00409 CNFG_ECG_r.bits.dhpf = 1; // Digital HPF cutoff = 0.5Hz 00410 CNFG_ECG_r.bits.gain = 3; // ECG gain = 160V/V 00411 CNFG_ECG_r.bits.rate = 2; // Sample rate = 128 sps 00412 ecgAFE.writeRegister( MAX30003::CNFG_ECG , CNFG_ECG_r.all); 00413 00414 00415 //R-to-R configuration 00416 MAX30003::RtoR1Configuration_u CNFG_RTOR_r; 00417 CNFG_RTOR_r.bits.en_rtor = 1; // Enable R-to-R detection 00418 ecgAFE.writeRegister( MAX30003::CNFG_RTOR1 , CNFG_RTOR_r.all); 00419 00420 00421 //Manage interrupts register setting 00422 MAX30003::ManageInterrupts_u MNG_INT_r; 00423 MNG_INT_r.bits.efit = 0b00011; // Assert EINT w/ 4 unread samples 00424 MNG_INT_r.bits.clr_rrint = 0b01; // Clear R-to-R on RTOR reg. read back 00425 ecgAFE.writeRegister( MAX30003::MNGR_INT , MNG_INT_r.all); 00426 00427 00428 //Enable interrupts register setting 00429 MAX30003::EnableInterrupts_u EN_INT_r; 00430 EN_INT_r.all = 0; 00431 EN_INT_r.bits.en_eint = 1; // Enable EINT interrupt 00432 EN_INT_r.bits.en_rrint = 0; // Disable R-to-R interrupt 00433 EN_INT_r.bits.intb_type = 3; // Open-drain NMOS with internal pullup 00434 ecgAFE.writeRegister( MAX30003::EN_INT , EN_INT_r.all); 00435 00436 00437 //Dyanmic modes config 00438 MAX30003::ManageDynamicModes_u MNG_DYN_r; 00439 MNG_DYN_r.bits.fast = 0; // Fast recovery mode disabled 00440 ecgAFE.writeRegister( MAX30003::MNGR_DYN , MNG_DYN_r.all); 00441 00442 // MUX Config 00443 MAX30003::MuxConfiguration_u CNFG_MUX_r; 00444 CNFG_MUX_r.bits.openn = 0; // Connect ECGN to AFE channel 00445 CNFG_MUX_r.bits.openp = 0; // Connect ECGP to AFE channel 00446 ecgAFE.writeRegister( MAX30003::CNFG_EMUX , CNFG_MUX_r.all); 00447 00448 return; 00449 } 00450
Generated on Tue Aug 23 2022 05:32:45 by
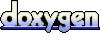