
Sweep a servo according to Proximity sensor measure
Dependencies: Servo X_NUCLEO_6180XA1 mbed
Fork of HelloWorld_6180XA1 by
main.cpp
00001 #include "mbed.h" 00002 #include "x_nucleo_6180xa1.h" 00003 #include <string.h> 00004 #include <stdlib.h> 00005 #include <stdio.h> 00006 #include <assert.h> 00007 00008 #include "Servo.h" 00009 00010 Servo myservo(D3); 00011 00012 /* This VL6180X Expansion board test application performs a range measurement and als measurement in polling mode 00013 on the onboard embedded top sensor. 00014 The result of both the measures are printed on the serial over. 00015 GetDistance and GetLux are synchronous!. So they blocks the caller until the result will be ready 00016 */ 00017 00018 #define VL6180X_I2C_SDA D14 00019 #define VL6180X_I2C_SCL D15 00020 00021 static X_NUCLEO_6180XA1 *board=NULL; 00022 00023 /*=================================== Main ================================== 00024 Prints on the serial over USB the measured distance and lux. 00025 The measures are run in single shot polling mode. 00026 =============================================================================*/ 00027 int main() 00028 { 00029 int status; 00030 uint32_t lux, dist; 00031 DevI2C *device_i2c =new DevI2C(VL6180X_I2C_SDA, VL6180X_I2C_SCL); 00032 /* creates the 6180XA1 expansion board singleton obj */ 00033 board=X_NUCLEO_6180XA1::Instance(device_i2c, A3, A2, D13, D2); 00034 /* init the 6180XA1 expansion board with default values */ 00035 status=board->InitBoard(); 00036 if(status) { printf("Failed to init board!\n\r"); return 0; } 00037 while(1) 00038 { 00039 board->sensor_top->GetDistance(&dist); 00040 board->sensor_top->GetLux(&lux); 00041 myservo = (255.0-dist)/255.0; 00042 printf ("Distance: %d, Lux: %d\n\r",dist, lux); 00043 } 00044 }
Generated on Thu Jul 14 2022 04:00:04 by
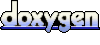