
Project with example IPSO resources for LED bar, Gas Sensor, and Light Sensor
Dependencies: EthernetInterface LED_Bar mbed-rtos mbed nsdl_lib
LEDbar.cpp
00001 // Light resource implementation 00002 00003 #include "mbed.h" 00004 #include "nsdl_support.h" 00005 #include "LEDbar.h" 00006 #include "LED_Bar.h" 00007 00008 #define LEDBAR_RES_ID "11101/0/5901" 00009 #define LEDBAR_RES_RT "urn:X-mbed:LEDbar" 00010 00011 extern Serial pc; 00012 char leds[] = {"0000000000"}; //GGGGGGGGYR 00013 00014 LED_Bar bar(D7, D6); 00015 00016 uint8_t ledbar_max_age = 0; 00017 uint8_t ledbar_content_type = 0; 00018 00019 void set_leds(char *leds) 00020 { 00021 int i; 00022 for (i=0; i<=9; i++) { 00023 if(leds[i] == '1') 00024 bar.setSingleLed((9-i),1); 00025 else if(leds[i] == '0') 00026 bar.setSingleLed((9-i),0); 00027 } 00028 } 00029 00030 00031 /* Only GET and PUT method allowed */ 00032 static uint8_t LEDbar_resource_cb(sn_coap_hdr_s *received_coap_ptr, sn_nsdl_addr_s *address, sn_proto_info_s * proto) 00033 { 00034 sn_coap_hdr_s *coap_res_ptr = 0; 00035 00036 //pc.printf("LED Strip callback\r\n"); 00037 00038 if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_GET) 00039 { 00040 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CONTENT); 00041 00042 coap_res_ptr->payload_len = strlen(leds); 00043 coap_res_ptr->payload_ptr = (uint8_t*)leds; 00044 00045 coap_res_ptr->content_type_ptr = &ledbar_content_type; 00046 coap_res_ptr->content_type_len = sizeof(ledbar_content_type); 00047 00048 coap_res_ptr->options_list_ptr = (sn_coap_options_list_s*)nsdl_alloc(sizeof(sn_coap_options_list_s)); 00049 if(!coap_res_ptr->options_list_ptr) 00050 { 00051 pc.printf("cant alloc option list for max-age\r\n"); 00052 coap_res_ptr->options_list_ptr = NULL; //FIXME report error and recover 00053 } 00054 memset(coap_res_ptr->options_list_ptr, 0, sizeof(sn_coap_options_list_s)); 00055 coap_res_ptr->options_list_ptr->max_age_ptr = &ledbar_max_age; 00056 coap_res_ptr->options_list_ptr->max_age_len = sizeof(ledbar_max_age); 00057 00058 sn_nsdl_send_coap_message(address, coap_res_ptr); 00059 nsdl_free(coap_res_ptr->options_list_ptr); 00060 coap_res_ptr->options_list_ptr = NULL; 00061 coap_res_ptr->content_type_ptr = NULL;// parser_release below tries to free this memory 00062 00063 } 00064 else if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_PUT) 00065 { 00066 //pc.printf("PUT: %d bytes\r\n", received_coap_ptr->payload_len); 00067 if(received_coap_ptr->payload_len == 10) 00068 { 00069 memcpy(leds, (char *)received_coap_ptr->payload_ptr, received_coap_ptr->payload_len); 00070 00071 leds[received_coap_ptr->payload_len] = '\0'; 00072 pc.printf("PUT: %s\r\n",leds); 00073 00074 //call LED strup update function here 00075 set_leds(leds); 00076 00077 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CHANGED); 00078 sn_nsdl_send_coap_message(address, coap_res_ptr); 00079 } 00080 } 00081 00082 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00083 return 0; 00084 } 00085 00086 int create_LEDbar_resource(sn_nsdl_resource_info_s *resource_ptr) 00087 { 00088 nsdl_create_dynamic_resource(resource_ptr, sizeof(LEDBAR_RES_ID)-1, (uint8_t*)LEDBAR_RES_ID, sizeof(LEDBAR_RES_RT)-1, (uint8_t*)LEDBAR_RES_RT, 0, &LEDbar_resource_cb, (SN_GRS_GET_ALLOWED | SN_GRS_PUT_ALLOWED)); 00089 set_leds("0000000000"); 00090 return 0; 00091 }
Generated on Tue Jul 12 2022 23:49:31 by
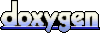