Lancaster University fork of the Nordic nrf51-SDK repository, which actually lives on github: https://github.com/lancaster-university/nrf51-sdk
dfu_bank_internal.h
00001 /* 00002 * Copyright (c) Nordic Semiconductor ASA 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, this 00009 * list of conditions and the following disclaimer. 00010 * 00011 * 2. Redistributions in binary form must reproduce the above copyright notice, this 00012 * list of conditions and the following disclaimer in the documentation and/or 00013 * other materials provided with the distribution. 00014 * 00015 * 3. Neither the name of Nordic Semiconductor ASA nor the names of other 00016 * contributors to this software may be used to endorse or promote products 00017 * derived from this software without specific prior written permission. 00018 * 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00021 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00022 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00023 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00024 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00025 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00026 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00027 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00028 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00029 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00030 * 00031 */ 00032 00033 /**@file 00034 * 00035 * @defgroup dfu_bank_internal Device Firmware Update internal header for bank handling in DFU. 00036 * @{ 00037 * 00038 * @brief Device Firmware Update Bank handling module interface. 00039 * 00040 * @details This header is intended for shared definition and functions between single and dual bank 00041 * implementations used for DFU support. It is not supposed to be used for external access 00042 * to the DFU module. 00043 * 00044 */ 00045 #ifndef DFU_BANK_INTERNAL_H__ 00046 #define DFU_BANK_INTERNAL_H__ 00047 00048 #include <dfu_types.h > 00049 00050 /**@brief States of the DFU state machine. */ 00051 typedef enum 00052 { 00053 DFU_STATE_INIT_ERROR, /**< State for: dfu_init(...) error. */ 00054 DFU_STATE_IDLE, /**< State for: idle. */ 00055 DFU_STATE_PREPARING, /**< State for: preparing, indicates that the flash is being erased and no data packets can be processed. */ 00056 DFU_STATE_RDY, /**< State for: ready. */ 00057 DFU_STATE_RX_INIT_PKT, /**< State for: receiving initialization packet. */ 00058 DFU_STATE_RX_DATA_PKT, /**< State for: receiving data packet. */ 00059 DFU_STATE_VALIDATE, /**< State for: validate. */ 00060 DFU_STATE_WAIT_4_ACTIVATE /**< State for: waiting for dfu_image_activate(). */ 00061 } dfu_state_t; 00062 00063 #define APP_TIMER_PRESCALER 0 /**< Value of the RTC1 PRESCALER register. */ 00064 #define DFU_TIMEOUT_INTERVAL APP_TIMER_TICKS(120000, APP_TIMER_PRESCALER) /**< DFU timeout interval in units of timer ticks. */ 00065 00066 #define IS_UPDATING_SD(START_PKT) ((START_PKT).dfu_update_mode & DFU_UPDATE_SD) /**< Macro for determining if a SoftDevice update is ongoing. */ 00067 #define IS_UPDATING_BL(START_PKT) ((START_PKT).dfu_update_mode & DFU_UPDATE_BL) /**< Macro for determining if a Bootloader update is ongoing. */ 00068 #define IS_UPDATING_APP(START_PKT) ((START_PKT).dfu_update_mode & DFU_UPDATE_APP) /**< Macro for determining if a Application update is ongoing. */ 00069 #define IMAGE_WRITE_IN_PROGRESS() (m_data_received > 0) /**< Macro for determining if an image write is in progress. */ 00070 #define IS_WORD_SIZED(SIZE) ((SIZE & (sizeof(uint32_t) - 1)) == 0) /**< Macro for checking that the provided is word sized. */ 00071 00072 /**@cond NO_DOXYGEN */ 00073 static uint32_t m_data_received; /**< Amount of received data. */ 00074 /**@endcond */ 00075 00076 /**@brief Type definition of function used for preparing of the bank before receiving of a 00077 * software image. 00078 * 00079 * @param[in] image_size Size of software image being received. 00080 */ 00081 typedef void (*dfu_bank_prepare_t)(uint32_t image_size); 00082 00083 /**@brief Type definition of function used for handling clear complete of the bank before 00084 * receiving of a software image. 00085 */ 00086 typedef void (*dfu_bank_cleared_t)(void); 00087 00088 /**@brief Type definition of function used for activating of the software image received. 00089 * 00090 * @return NRF_SUCCESS If the image has been successfully activated any other NRF_ERROR code in 00091 * case of a failure. 00092 */ 00093 typedef uint32_t (*dfu_bank_activate_t)(void); 00094 00095 /**@brief Structure for holding of function pointers for needed prepare and activate procedure for 00096 * the requested update procedure. 00097 */ 00098 typedef struct 00099 { 00100 dfu_bank_prepare_t prepare; /**< Function pointer to the prepare function called on start of update procedure. */ 00101 dfu_bank_cleared_t cleared; /**< Function pointer to the cleared function called after prepare function completes. */ 00102 dfu_bank_activate_t activate; /**< Function pointer to the activate function called on finalizing the update procedure. */ 00103 } dfu_bank_func_t; 00104 00105 #endif // DFU_BANK_INTERNAL_H__ 00106 00107 /** @} */
Generated on Tue Jul 12 2022 15:07:13 by
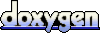