mbed.org local branch of microbit-dal. The real version lives in git at https://github.com/lancaster-university/microbit-dal
Dependencies: BLE_API nRF51822 mbed-dev-bin
Dependents: microbit Microbit IoTChallenge1 microbit ... more
MicroBitBLEManager.h
00001 /* 00002 The MIT License (MIT) 00003 00004 Copyright (c) 2016 British Broadcasting Corporation. 00005 This software is provided by Lancaster University by arrangement with the BBC. 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a 00008 copy of this software and associated documentation files (the "Software"), 00009 to deal in the Software without restriction, including without limitation 00010 the rights to use, copy, modify, merge, publish, distribute, sublicense, 00011 and/or sell copies of the Software, and to permit persons to whom the 00012 Software is furnished to do so, subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in 00015 all copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL 00020 THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00022 FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00023 DEALINGS IN THE SOFTWARE. 00024 */ 00025 00026 #ifndef MICROBIT_BLE_MANAGER_H 00027 #define MICROBIT_BLE_MANAGER_H 00028 00029 #include "mbed.h" 00030 #include "MicroBitConfig.h" 00031 00032 /* 00033 * The underlying Nordic libraries that support BLE do not compile cleanly with the stringent GCC settings we employ 00034 * If we're compiling under GCC, then we suppress any warnings generated from this code (but not the rest of the DAL) 00035 * The ARM cc compiler is more tolerant. We don't test __GNUC__ here to detect GCC as ARMCC also typically sets this 00036 * as a compatability option, but does not support the options used... 00037 */ 00038 #if !defined (__arm) 00039 #pragma GCC diagnostic push 00040 #pragma GCC diagnostic ignored "-Wunused-parameter" 00041 #endif 00042 #include "ble/BLE.h" 00043 00044 /* 00045 * Return to our predefined compiler settings. 00046 */ 00047 #if !defined (__arm) 00048 #pragma GCC diagnostic pop 00049 #endif 00050 00051 #include "ble/services/DeviceInformationService.h" 00052 #include "MicroBitDFUService.h" 00053 #include "MicroBitEventService.h" 00054 #include "MicroBitLEDService.h" 00055 #include "MicroBitAccelerometerService.h" 00056 #include "MicroBitMagnetometerService.h" 00057 #include "MicroBitButtonService.h" 00058 #include "MicroBitIOPinService.h" 00059 #include "MicroBitTemperatureService.h" 00060 #include "ExternalEvents.h" 00061 #include "MicroBitButton.h" 00062 #include "MicroBitStorage.h" 00063 00064 #define MICROBIT_BLE_PAIR_REQUEST 0x01 00065 #define MICROBIT_BLE_PAIR_COMPLETE 0x02 00066 #define MICROBIT_BLE_PAIR_PASSCODE 0x04 00067 #define MICROBIT_BLE_PAIR_SUCCESSFUL 0x08 00068 00069 #define MICROBIT_BLE_PAIRING_TIMEOUT 90 00070 #define MICROBIT_BLE_POWER_LEVELS 8 00071 #define MICROBIT_BLE_MAXIMUM_BONDS 4 00072 #define MICROBIT_BLE_ENABLE_BONDING true 00073 00074 extern const int8_t MICROBIT_BLE_POWER_LEVEL[]; 00075 00076 struct BLESysAttribute 00077 { 00078 uint8_t sys_attr[8]; 00079 }; 00080 00081 struct BLESysAttributeStore 00082 { 00083 BLESysAttribute sys_attrs[MICROBIT_BLE_MAXIMUM_BONDS]; 00084 }; 00085 00086 /** 00087 * Class definition for the MicroBitBLEManager. 00088 * 00089 */ 00090 class MicroBitBLEManager : MicroBitComponent 00091 { 00092 public: 00093 00094 // The mbed abstraction of the BlueTooth Low Energy (BLE) hardware 00095 BLEDevice *ble; 00096 00097 //an instance of MicroBitStorage used to store sysAttrs from softdevice 00098 MicroBitStorage* storage; 00099 00100 /** 00101 * Constructor. 00102 * 00103 * Configure and manage the micro:bit's Bluetooth Low Energy (BLE) stack. 00104 * 00105 * @param _storage an instance of MicroBitStorage used to persist sys attribute information. (This is required for compatability with iOS). 00106 * 00107 * @note The BLE stack *cannot* be brought up in a static context (the software simply hangs or corrupts itself). 00108 * Hence, the init() member function should be used to initialise the BLE stack. 00109 */ 00110 MicroBitBLEManager(MicroBitStorage& _storage); 00111 00112 /** 00113 * Constructor. 00114 * 00115 * Configure and manage the micro:bit's Bluetooth Low Energy (BLE) stack. 00116 * 00117 * @note The BLE stack *cannot* be brought up in a static context (the software simply hangs or corrupts itself). 00118 * Hence, the init() member function should be used to initialise the BLE stack. 00119 */ 00120 MicroBitBLEManager(); 00121 00122 /** 00123 * Post constructor initialisation method as the BLE stack cannot be brought 00124 * up in a static context. 00125 * 00126 * @param deviceName The name used when advertising 00127 * @param serialNumber The serial number exposed by the device information service 00128 * @param messageBus An instance of an EventModel, used during pairing. 00129 * @param enableBonding If true, the security manager enabled bonding. 00130 * 00131 * @code 00132 * bleManager.init(uBit.getName(), uBit.getSerial(), uBit.messageBus, true); 00133 * @endcode 00134 */ 00135 void init(ManagedString deviceName, ManagedString serialNumber, EventModel& messageBus, bool enableBonding); 00136 00137 /** 00138 * Change the output power level of the transmitter to the given value. 00139 * 00140 * @param power a value in the range 0..7, where 0 is the lowest power and 7 is the highest. 00141 * 00142 * @return MICROBIT_OK on success, or MICROBIT_INVALID_PARAMETER if the value is out of range. 00143 * 00144 * @code 00145 * // maximum transmission power. 00146 * bleManager.setTransmitPower(7); 00147 * @endcode 00148 */ 00149 int setTransmitPower(int power); 00150 00151 /** 00152 * Enter pairing mode. This is mode is called to initiate pairing, and to enable FOTA programming 00153 * of the micro:bit in cases where BLE is disabled during normal operation. 00154 * 00155 * @param display An instance of MicroBitDisplay used when displaying pairing information. 00156 * @param authorizationButton The button to use to authorise a pairing request. 00157 * 00158 * @code 00159 * // initiate pairing mode 00160 * bleManager.pairingMode(uBit.display, uBit.buttonA); 00161 * @endcode 00162 */ 00163 void pairingMode(MicroBitDisplay &display, MicroBitButton &authorisationButton); 00164 00165 /** 00166 * When called, the micro:bit will begin advertising for a predefined period, 00167 * MICROBIT_BLE_ADVERTISING_TIMEOUT seconds to bonded devices. 00168 */ 00169 void advertise(); 00170 00171 /** 00172 * Determines the number of devices currently bonded with this micro:bit. 00173 * @return The number of active bonds. 00174 */ 00175 int getBondCount(); 00176 00177 /** 00178 * A request to pair has been received from a BLE device. 00179 * If we're in pairing mode, display the passkey to the user. 00180 * Also, purge the bonding table if it has reached capacity. 00181 * 00182 * @note for internal use only. 00183 */ 00184 void pairingRequested(ManagedString passKey); 00185 00186 /** 00187 * A pairing request has been sucessfully completed. 00188 * If we're in pairing mode, display a success or failure message. 00189 * 00190 * @note for internal use only. 00191 */ 00192 void pairingComplete(bool success); 00193 00194 /** 00195 * Periodic callback in thread context. 00196 * We use this here purely to safely issue a disconnect operation after a pairing operation is complete. 00197 */ 00198 void idleTick(); 00199 00200 private: 00201 00202 /** 00203 * Displays the device's ID code as a histogram on the provided MicroBitDisplay instance. 00204 * 00205 * @param display The display instance used for displaying the histogram. 00206 */ 00207 void showNameHistogram(MicroBitDisplay &display); 00208 00209 int pairingStatus; 00210 ManagedString passKey; 00211 ManagedString deviceName; 00212 00213 }; 00214 00215 #endif
Generated on Tue Jul 12 2022 15:22:55 by
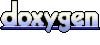