Lancaster University's fork of the mbed BLE API. Lives on github, https://github.com/lancaster-university/BLE_API
Dependents: microbit-dal microbit-dal microbit-ble-open microbit-dal ... more
Fork of BLE_API by
DiscoveredCharacteristic.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __DISCOVERED_CHARACTERISTIC_H__ 00018 #define __DISCOVERED_CHARACTERISTIC_H__ 00019 00020 #include "UUID.h" 00021 #include "Gap.h" 00022 #include "GattAttribute.h" 00023 #include "GattClient.h" 00024 #include "CharacteristicDescriptorDiscovery.h" 00025 #include "ble/DiscoveredCharacteristicDescriptor.h" 00026 00027 /** 00028 * @brief Representation of a characteristic discovered during a GattClient 00029 * discovery procedure (see GattClient::launchServiceDiscovery ). 00030 * 00031 * @detail Provide detailed informations about a discovered characteristic like: 00032 * - Its UUID (see #getUUID). 00033 * - The most important handles of the characteristic definition 00034 * (see #getDeclHandle, #getValueHandle, #getLastHandle ) 00035 * - Its properties (see #getProperties). 00036 * This class also provide functions to operate on the characteristic: 00037 * - Read the characteristic value (see #read) 00038 * - Writing a characteristic value (see #write or #writeWoResponse) 00039 * - Discover descriptors inside the characteristic definition. These descriptors 00040 * extends the characteristic. More information about descriptor usage is 00041 * available in DiscoveredCharacteristicDescriptor class. 00042 */ 00043 class DiscoveredCharacteristic { 00044 public: 00045 struct Properties_t { 00046 uint8_t _broadcast :1; /**< Broadcasting the value permitted. */ 00047 uint8_t _read :1; /**< Reading the value permitted. */ 00048 uint8_t _writeWoResp :1; /**< Writing the value with Write Command permitted. */ 00049 uint8_t _write :1; /**< Writing the value with Write Request permitted. */ 00050 uint8_t _notify :1; /**< Notifications of the value permitted. */ 00051 uint8_t _indicate :1; /**< Indications of the value permitted. */ 00052 uint8_t _authSignedWrite :1; /**< Writing the value with Signed Write Command permitted. */ 00053 00054 public: 00055 bool broadcast(void) const {return _broadcast; } 00056 bool read(void) const {return _read; } 00057 bool writeWoResp(void) const {return _writeWoResp; } 00058 bool write(void) const {return _write; } 00059 bool notify(void) const {return _notify; } 00060 bool indicate(void) const {return _indicate; } 00061 bool authSignedWrite(void) const {return _authSignedWrite;} 00062 00063 /** 00064 * @brief "Equal to" operator for DiscoveredCharacteristic::Properties_t 00065 * 00066 * @param lhs[in] The left hand side of the equality expression 00067 * @param rhs[in] The right hand side of the equality expression 00068 * 00069 * @return true if operands are equals, false otherwise. 00070 */ 00071 friend bool operator==(Properties_t lhs, Properties_t rhs) { 00072 return lhs._broadcast == rhs._broadcast && 00073 lhs._read == rhs._read && 00074 lhs._writeWoResp == rhs._writeWoResp && 00075 lhs._write == rhs._write && 00076 lhs._notify == rhs._notify && 00077 lhs._indicate == rhs._indicate && 00078 lhs._authSignedWrite == rhs._authSignedWrite; 00079 } 00080 00081 /** 00082 * @brief "Not equal to" operator for DiscoveredCharacteristic::Properties_t 00083 * 00084 * @param lhs The right hand side of the expression 00085 * @param rhs The left hand side of the expression 00086 * 00087 * @return true if operands are not equals, false otherwise. 00088 */ 00089 friend bool operator!=(Properties_t lhs, Properties_t rhs) { 00090 return !(lhs == rhs); 00091 } 00092 00093 private: 00094 operator uint8_t() const; /* Disallow implicit conversion into an integer. */ 00095 operator unsigned() const; /* Disallow implicit conversion into an integer. */ 00096 }; 00097 00098 /** 00099 * Initiate (or continue) a read for the value attribute, optionally at a 00100 * given offset. If the characteristic or descriptor to be read is longer 00101 * than ATT_MTU - 1, this function must be called multiple times with 00102 * appropriate offset to read the complete value. 00103 * 00104 * @param offset[in] The position - in the characteristic value bytes stream - where 00105 * the read operation begin. 00106 * 00107 * @return BLE_ERROR_NONE if a read has been initiated, or 00108 * BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or 00109 * BLE_STACK_BUSY if some client procedure is already in progress, or 00110 * BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties. 00111 */ 00112 ble_error_t read(uint16_t offset = 0) const; 00113 00114 /** 00115 * @brief Same as #read(uint16_t) const but allow the user to register a callback 00116 * which will be fired once the read is done. 00117 * 00118 * @param offset[in] The position - in the characteristic value bytes stream - where 00119 * the read operation begin. 00120 * @param onRead[in] Continuation of the read operation 00121 */ 00122 ble_error_t read(uint16_t offset, const GattClient::ReadCallback_t & onRead) const; 00123 00124 /** 00125 * Perform a write without response procedure. 00126 * 00127 * @param[in] length 00128 * The amount of data being written. 00129 * @param[in] value 00130 * The bytes being written. 00131 * 00132 * @note It is important to note that a write without response will generate 00133 * an onDataSent() callback when the packet has been transmitted. There 00134 * will be a BLE-stack specific limit to the number of pending 00135 * writeWoResponse operations; the user may want to use the onDataSent() 00136 * callback for flow-control. 00137 * 00138 * @retval BLE_ERROR_NONE Successfully started the Write procedure, or 00139 * BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or 00140 * BLE_STACK_BUSY if some client procedure is already in progress, or 00141 * BLE_ERROR_NO_MEM if there are no available buffers left to process the request, or 00142 * BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties. 00143 */ 00144 ble_error_t writeWoResponse(uint16_t length, const uint8_t *value) const; 00145 00146 /** 00147 * Initiate a GATT Characteristic Descriptor Discovery procedure for descriptors within this characteristic. 00148 * 00149 * @param[in] onDescriptorDiscovered This callback will be called every time a descriptor is discovered 00150 * @param[in] onTermination This callback will be called when the discovery process is over. 00151 * 00152 * @return BLE_ERROR_NONE if descriptor discovery is launched successfully; else an appropriate error. 00153 */ 00154 ble_error_t discoverDescriptors(const CharacteristicDescriptorDiscovery::DiscoveryCallback_t& onDescriptorDiscovered, 00155 const CharacteristicDescriptorDiscovery::TerminationCallback_t& onTermination) const; 00156 00157 /** 00158 * Perform a write procedure. 00159 * 00160 * @param[in] length 00161 * The amount of data being written. 00162 * @param[in] value 00163 * The bytes being written. 00164 * 00165 * @note It is important to note that a write will generate 00166 * an onDataWritten() callback when the peer acknowledges the request. 00167 * 00168 * @retval BLE_ERROR_NONE Successfully started the Write procedure, or 00169 * BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or 00170 * BLE_STACK_BUSY if some client procedure is already in progress, or 00171 * BLE_ERROR_NO_MEM if there are no available buffers left to process the request, or 00172 * BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties. 00173 */ 00174 ble_error_t write(uint16_t length, const uint8_t *value) const; 00175 00176 /** 00177 * Same as #write(uint16_t, const uint8_t *) const but register a callback 00178 * which will be called once the data has been written. 00179 * 00180 * @param[in] length The amount of bytes to write. 00181 * @param[in] value The bytes to write. 00182 * @param[in] onRead Continuation callback for the write operation 00183 */ 00184 ble_error_t write(uint16_t length, const uint8_t *value, const GattClient::WriteCallback_t & onWrite) const; 00185 00186 void setupLongUUID(UUID::LongUUIDBytes_t longUUID, UUID::ByteOrder_t order = UUID::MSB) { 00187 uuid.setupLong(longUUID, order); 00188 } 00189 00190 public: 00191 /** 00192 * @brief Get the UUID of the discovered characteristic 00193 * @return the UUID of this characteristic 00194 */ 00195 const UUID& getUUID(void) const { 00196 return uuid; 00197 } 00198 00199 /** 00200 * @brief Get the properties of this characteristic 00201 * @return the set of properties of this characteristic 00202 */ 00203 const Properties_t& getProperties(void) const { 00204 return props; 00205 } 00206 00207 /** 00208 * @brief Get the declaration handle of this characteristic. 00209 * @detail The declaration handle is the first handle of a characteristic 00210 * definition. The value accessible at this handle contains the following 00211 * informations: 00212 * - The characteristics properties (see Properties_t). This value can 00213 * be accessed by using #getProperties . 00214 * - The characteristic value attribute handle. This field can be accessed 00215 * by using #getValueHandle . 00216 * - The characteristic UUID, this value can be accessed by using the 00217 * function #getUUID . 00218 * @return the declaration handle of this characteristic. 00219 */ 00220 GattAttribute::Handle_t getDeclHandle(void) const { 00221 return declHandle; 00222 } 00223 00224 /** 00225 * @brief Return the handle used to access the value of this characteristic. 00226 * @details This handle is the one provided in the characteristic declaration 00227 * value. Usually, it is equal to #getDeclHandle() + 1. But it is not always 00228 * the case. Anyway, users are allowed to use #getDeclHandle() + 1 to access 00229 * the value of a characteristic. 00230 * @return The handle to access the value of this characteristic. 00231 */ 00232 GattAttribute::Handle_t getValueHandle(void) const { 00233 return valueHandle; 00234 } 00235 00236 /** 00237 * @brief Return the last handle of the characteristic definition. 00238 * @details A Characteristic definition can contain a lot of handles: 00239 * - one for the declaration (see #getDeclHandle) 00240 * - one for the value (see #getValueHandle) 00241 * - zero of more for the characteristic descriptors. 00242 * This handle is the last handle of the characteristic definition. 00243 * @return The last handle of this characteristic definition. 00244 */ 00245 GattAttribute::Handle_t getLastHandle(void) const { 00246 return lastHandle; 00247 } 00248 00249 /** 00250 * @brief Return the GattClient which can operate on this characteristic. 00251 * @return The GattClient which can operate on this characteristic. 00252 */ 00253 GattClient* getGattClient() { 00254 return gattc; 00255 } 00256 00257 /** 00258 * @brief Return the GattClient which can operate on this characteristic. 00259 * @return The GattClient which can operate on this characteristic. 00260 */ 00261 const GattClient* getGattClient() const { 00262 return gattc; 00263 } 00264 00265 /** 00266 * @brief Return the connection handle to the GattServer which contain 00267 * this characteristic. 00268 * @return the connection handle to the GattServer which contain 00269 * this characteristic. 00270 */ 00271 Gap::Handle_t getConnectionHandle() const { 00272 return connHandle; 00273 } 00274 00275 /** 00276 * @brief "Equal to" operator for DiscoveredCharacteristic 00277 * 00278 * @param lhs[in] The left hand side of the equality expression 00279 * @param rhs[in] The right hand side of the equality expression 00280 * 00281 * @return true if operands are equals, false otherwise. 00282 */ 00283 friend bool operator==(const DiscoveredCharacteristic& lhs, const DiscoveredCharacteristic& rhs) { 00284 return lhs.gattc == rhs.gattc && 00285 lhs.uuid == rhs.uuid && 00286 lhs.props == rhs.props && 00287 lhs.declHandle == rhs.declHandle && 00288 lhs.valueHandle == rhs.valueHandle && 00289 lhs.lastHandle == rhs.lastHandle && 00290 lhs.connHandle == rhs.connHandle; 00291 } 00292 00293 /** 00294 * @brief "Not equal to" operator for DiscoveredCharacteristic 00295 * 00296 * @param lhs[in] The right hand side of the expression 00297 * @param rhs[in] The left hand side of the expression 00298 * 00299 * @return true if operands are not equals, false otherwise. 00300 */ 00301 friend bool operator !=(const DiscoveredCharacteristic& lhs, const DiscoveredCharacteristic& rhs) { 00302 return !(lhs == rhs); 00303 } 00304 00305 public: 00306 DiscoveredCharacteristic() : gattc(NULL), 00307 uuid(UUID::ShortUUIDBytes_t(0)), 00308 props(), 00309 declHandle(GattAttribute::INVALID_HANDLE), 00310 valueHandle(GattAttribute::INVALID_HANDLE), 00311 lastHandle(GattAttribute::INVALID_HANDLE), 00312 connHandle() { 00313 /* empty */ 00314 } 00315 00316 protected: 00317 GattClient *gattc; 00318 00319 protected: 00320 UUID uuid; 00321 Properties_t props; 00322 GattAttribute::Handle_t declHandle; 00323 GattAttribute::Handle_t valueHandle; 00324 GattAttribute::Handle_t lastHandle; 00325 00326 Gap::Handle_t connHandle; 00327 }; 00328 00329 #endif /*__DISCOVERED_CHARACTERISTIC_H__*/
Generated on Tue Jul 12 2022 17:17:58 by
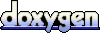