Library to interface MMA8451Q sensor
Dependents: LELEC_2811_Accelerometer_2 LELEC_2811_Multiple_Sensors LELEC_2811_acquiring_data LELEC_2811_acquiring_data_working
MMA8451Q.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "MMA8451Q.h" 00020 00021 #define REG_WHO_AM_I 0x0D 00022 #define REG_CTRL_REG_1 0x2A 00023 #define REG_OUT_X_MSB 0x01 00024 #define REG_OUT_Y_MSB 0x03 00025 #define REG_OUT_Z_MSB 0x05 00026 #define REG_XYZ_DATA_CFG 0x0E 00027 00028 #define UINT14_MAX 16383 00029 00030 MMA8451Q::MMA8451Q(PinName sda, PinName scl, int addr, uint8_t fsr) : m_i2c(sda, scl), m_addr(addr) { 00031 00032 // Place the peripheral in standby mode to configure the full scale range 00033 uint8_t data[2] = {REG_CTRL_REG_1, 0x00}; 00034 writeRegs(data, 2); 00035 00036 // Set the scale 00037 setScale(fsr); 00038 00039 // Activate the peripheral 00040 data[0] = REG_CTRL_REG_1; 00041 data[1] = 0x01; 00042 writeRegs(data, 2); 00043 } 00044 00045 MMA8451Q::~MMA8451Q() { } 00046 00047 uint8_t MMA8451Q::getWhoAmI() { 00048 uint8_t who_am_i = 0; 00049 readRegs(REG_WHO_AM_I, &who_am_i, 1); 00050 return who_am_i; 00051 } 00052 00053 float MMA8451Q::getAccX() { 00054 return (float(getAccAxis(REG_OUT_X_MSB))/4096.0); 00055 } 00056 00057 float MMA8451Q::getAccY() { 00058 return (float(getAccAxis(REG_OUT_Y_MSB))/4096.0); 00059 } 00060 00061 float MMA8451Q::getAccZ() { 00062 return (float(getAccAxis(REG_OUT_Z_MSB))/4096.0); 00063 } 00064 00065 void MMA8451Q::getAccAllAxis(float * res) { 00066 res[0] = getAccX(); 00067 res[1] = getAccY(); 00068 res[2] = getAccZ(); 00069 } 00070 00071 int16_t MMA8451Q::getAccAxis(uint8_t addr) { 00072 int16_t acc; 00073 uint8_t res[2]; 00074 readRegs(addr, res, 2); 00075 00076 acc = (res[0] << 6) | (res[1] >> 2); 00077 if (acc > UINT14_MAX/2) 00078 acc -= UINT14_MAX; 00079 00080 return acc; 00081 } 00082 00083 void MMA8451Q::setScale(uint8_t fsr) 00084 { 00085 // Read register 00086 uint8_t cfg; 00087 readRegs(REG_XYZ_DATA_CFG, &cfg, 8); 00088 00089 // Mask out scale bits 00090 cfg = cfg & 0xFC; 00091 cfg = cfg | fsr; // Neat trick, see page 22. 00 = 2G, 01 = 4A, 10 = 8G 00092 00093 // Write the configuration register 00094 uint8_t data[2] = {REG_XYZ_DATA_CFG, cfg}; 00095 writeRegs(data, 8); 00096 } 00097 00098 uint8_t MMA8451Q::Read_Status() 00099 { 00100 uint8_t Status; 00101 readRegs(0, &Status, 1); 00102 return Status; 00103 } 00104 00105 void MMA8451Q::readRegs(int addr, uint8_t * data, int len) { 00106 char t[1] = {addr}; 00107 m_i2c.write(m_addr, t, 1, true); 00108 m_i2c.read(m_addr, (char *)data, len); 00109 } 00110 00111 void MMA8451Q::writeRegs(uint8_t * data, int len) { 00112 m_i2c.write(m_addr, (char *)data, len); 00113 }
Generated on Wed Jul 27 2022 10:49:26 by
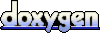