
各ピンへのread/writeを提供するサーバサンプル
Dependencies: NySNICInterface mbed-rtos mbed
RPCObject.h
00001 #ifndef RPCOBJECT 00002 #define RPCOBJECT 00003 00004 #include <map> 00005 #include "mbed.h" 00006 00007 enum RPC_PIN_TYPE { 00008 RPC_PIN_DIGITAL_IN, 00009 RPC_PIN_DIGITAL_OUT, 00010 RPC_PIN_DIGITAL_INOUT, 00011 RPC_PIN_UNKNOWN 00012 }; 00013 00014 struct rpc_arg 00015 { 00016 char *name; 00017 char *val; 00018 }; 00019 00020 class RPCClass 00021 { 00022 public : 00023 virtual int read()= 0; 00024 virtual void write(int value) = 0; 00025 }; 00026 00027 class RPCDigitalIn : public RPCClass 00028 { 00029 public : 00030 RPCDigitalIn(PinName pin) :i(pin){} 00031 virtual int read(void){return i.read();} 00032 virtual void write(int value){} 00033 00034 private : 00035 DigitalIn i; 00036 }; 00037 00038 class RPCDigitalOut : public RPCClass 00039 { 00040 public : 00041 RPCDigitalOut(PinName pin) :o(pin){} 00042 virtual int read(void){return o.read();} 00043 virtual void write(int value){o.write(value);} 00044 00045 private : 00046 DigitalOut o; 00047 }; 00048 00049 class RPCDigitalInOut : public RPCClass 00050 { 00051 public : 00052 RPCDigitalInOut(PinName pin) :o(pin){} 00053 virtual int read(void){return o.read();} 00054 virtual void write(int value){o.write(value);} 00055 00056 private : 00057 DigitalInOut o; 00058 }; 00059 00060 class RPCObject 00061 { 00062 public : 00063 RPCObject(); 00064 int decode(char *request, char* reply); 00065 00066 RPC_PIN_TYPE get_type() const { return type; } 00067 PinName get_pin_name() const { return pin_name; } 00068 int get_value() const { return value; } 00069 bool create_pin_object(char* reply); 00070 std::map<PinName, RPCClass*> pinObjects; 00071 00072 private : 00073 RPC_PIN_TYPE type; 00074 char obj_name[20]; 00075 PinName pin_name; 00076 int value; 00077 }; 00078 #endif 00079
Generated on Sun Jul 17 2022 16:34:37 by
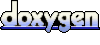