
各ピンへのread/writeを提供するサーバサンプル
Dependencies: NySNICInterface mbed-rtos mbed
RPCObject.cpp
00001 #include "RPCObject.h" 00002 #include "parse_pins.h" 00003 #include "mbed.h" 00004 #include "HTTPServer.h" 00005 00006 00007 RPCObject::RPCObject() 00008 { 00009 } 00010 00011 00012 int RPCObject::decode(char* request, char* reply) 00013 { 00014 char* clz = strtok(request+1,"/"); 00015 char* pin = strtok(NULL, "/"); 00016 char* val = strtok(NULL, "/"); 00017 00018 if(!strcmp(clz, "DigitalIn")){ 00019 type = RPC_PIN_DIGITAL_IN; 00020 printf(" type is DigitalIn. \r\n"); 00021 } else if(!strcmp(clz, "DigitalOut")){ 00022 type = RPC_PIN_DIGITAL_OUT; 00023 printf(" type is DigitalOut. \r\n"); 00024 } else if(!strcmp(clz, "DigitalInOut")){ 00025 type = RPC_PIN_DIGITAL_INOUT; 00026 printf(" type is DigitalInOut. \r\n"); 00027 } else { 00028 type = RPC_PIN_UNKNOWN; 00029 printf("Unsupported type name: %s. \r\n", clz); 00030 sprintf(reply, "Unsupported type name: %s. \r\n", clz); 00031 return HTTP_400_BADREQUEST; 00032 } 00033 00034 pin_name = parse_pins(pin); 00035 if(pin_name == NC){ 00036 printf("Unsupported pin name: %s. \n", pin); 00037 sprintf(reply, "Unsupported pin name: %s. \r\n", pin); 00038 return HTTP_400_BADREQUEST; 00039 } 00040 00041 if(!val || val[0] == '\0'){ 00042 value = -1; 00043 } 00044 else if(!strcmp(val, "delete")){ 00045 value = -2; 00046 } 00047 else { 00048 value = (val[0] - '0') ? 1 : 0; 00049 } 00050 00051 return 0; 00052 } 00053 00054 00055 bool RPCObject::create_pin_object(char* reply) 00056 { 00057 RPCClass* pinobj; 00058 00059 if(pinObjects.find(pin_name) != pinObjects.end()){ 00060 printf("The pin already exists.\r\n"); 00061 strcat(reply, "The pin already exists. "); 00062 return false; 00063 } 00064 00065 switch(type){ 00066 case RPC_PIN_DIGITAL_IN: 00067 printf("DigitalIn.\r\n"); 00068 pinobj = new RPCDigitalIn(pin_name); 00069 break; 00070 case RPC_PIN_DIGITAL_OUT: 00071 printf("DigitalOut.\r\n"); 00072 pinobj = new RPCDigitalOut(pin_name); 00073 break; 00074 case RPC_PIN_DIGITAL_INOUT: 00075 printf("DigitalInOut.\r\n"); 00076 pinobj = new RPCDigitalInOut(pin_name); 00077 break; 00078 default: 00079 printf(" Unsupported type.\r\n"); 00080 strcat(reply, "Unsupported type. "); 00081 return false; 00082 } 00083 00084 pinObjects[pin_name] = pinobj; 00085 00086 return true; 00087 }
Generated on Sun Jul 17 2022 16:34:37 by
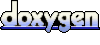