Basic services for RF XBee modules
Dependents: frdm_MasterVehicle
xbee.cpp
00001 #include "mbed.h" 00002 00003 #define XBEE_SERVER 1 00004 #define XBEE_CLIENT 1 00005 #define SERIAL_BAUDRATE 115200 00006 #define BUFFER_SIZE 9 00007 #define BUFFER_FORMAT "%X%X%X%X%X%X%X%X%X" 00008 #define BUFFER_FORMAT_RX "%c%c%c%c%c%c%c%c%c" 00009 #define BUFFER_RX_DATA_WR &CommandRx[0],&CommandRx[1],&CommandRx[2],&CommandRx[3],\ 00010 &CommandRx[4],&CommandRx[5],&CommandRx[6],&CommandRx[7],\ 00011 &CommandRx[8] 00012 #define BUFFER_RX_DATA_RD CommandRx[0],CommandRx[1],CommandRx[2],CommandRx[3],\ 00013 CommandRx[4],CommandRx[5],CommandRx[6],CommandRx[7],\ 00014 CommandRx[8] 00015 00016 Serial PC(USBTX, USBRX); 00017 DigitalOut Indicator(LED_GREEN); 00018 int XBee_u16Loop; 00019 00020 #if XBEE_SERVER == 1 00021 Serial XBeeServer(PTC17, PTC16); /* Tx, Rx*/ 00022 char CommandTx[BUFFER_SIZE+1]={0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, '\0'}; 00023 DigitalOut RSTServer(PTB9); 00024 int XBee_u16TxResult; 00025 int XBee_u8TxRq; 00026 #endif 00027 00028 #if XBEE_CLIENT == 1 00029 Serial XBeeClient(PTE24, PTE25); /* Tx, Rx*/ 00030 DigitalOut RSTClient(PTA1); 00031 char CommandRx[BUFFER_SIZE+1]; 00032 volatile int XBee_u8RxResult; 00033 int XBee_boRxIndication = 0; 00034 int XBee_boRxAllowed = 1; 00035 00036 /* This function is called when a character goes into the RX buffer.*/ 00037 void XBeeClientRx() { 00038 if(XBee_boRxAllowed == 1) 00039 { 00040 PC.printf("\r\nRx... "); 00041 XBee_u8RxResult = 0; 00042 XBee_u8RxResult = XBeeClient.scanf( BUFFER_FORMAT_RX, BUFFER_RX_DATA_WR ); 00043 if(XBee_u8RxResult>0) 00044 { 00045 XBee_boRxIndication = 1; 00046 } 00047 PC.printf("\r\nRx done [%i]. ",XBee_u8RxResult); 00048 PC.printf( BUFFER_FORMAT, BUFFER_RX_DATA_RD ); 00049 } 00050 return; 00051 } 00052 #endif 00053 00054 void XBee_vInit(void) 00055 { 00056 XBee_u16Loop = 0; 00057 00058 PC.baud(SERIAL_BAUDRATE); 00059 00060 #if XBEE_SERVER == 1 00061 PC.printf("\r\nSetting server XBee..."); 00062 XBeeServer.baud(SERIAL_BAUDRATE); 00063 XBee_u16TxResult = 0; 00064 XBee_u8TxRq = 0; 00065 #endif 00066 00067 #if XBEE_CLIENT == 1 00068 PC.printf("\r\nSetting client XBee..."); 00069 XBeeClient.baud(SERIAL_BAUDRATE); 00070 XBeeClient.attach(&XBeeClientRx, Serial::RxIrq); 00071 XBee_u8RxResult = 0; 00072 XBee_boRxAllowed = 1; 00073 #endif 00074 00075 /* XBee reset*/ 00076 #if XBEE_SERVER == 1 00077 RSTServer = 0; 00078 #endif 00079 #if XBEE_CLIENT == 1 00080 RSTClient = 0; 00081 #endif 00082 wait_ms(1); 00083 #if XBEE_SERVER == 1 00084 RSTServer = 1; 00085 #endif 00086 #if XBEE_CLIENT == 1 00087 RSTClient = 1; 00088 #endif 00089 wait_ms(1); 00090 } 00091 00092 void XBee_vMain(void) 00093 { 00094 XBee_u16Loop++; 00095 PC.printf("\r\n[%i]:",XBee_u16Loop); 00096 00097 if(XBee_u8TxRq == 1) 00098 { 00099 /* a Tx job request is active */ 00100 PC.printf("\r\nTx... "); 00101 /* disable rx */ 00102 XBee_boRxAllowed = 0; 00103 for (int i=0; i<BUFFER_SIZE; i++) 00104 { 00105 while (true) 00106 { 00107 if (XBeeServer.writeable()) 00108 { 00109 XBee_u16TxResult= XBeeServer.putc(CommandTx[i]); 00110 PC.printf("%X ", XBee_u16TxResult); 00111 break; 00112 } 00113 } 00114 } 00115 /* tx done */ 00116 PC.printf("\r\nTx done. "); 00117 XBee_u8TxRq = 0; 00118 /* enable rx */ 00119 XBee_boRxAllowed = 1; 00120 } 00121 } 00122 00123 #if 0 00124 00125 int main() 00126 { 00127 char TxJob[BUFFER_SIZE+1] = {0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, '\0'}; 00128 00129 XBee_vInit(); 00130 00131 while (true) { 00132 wait(0.2f); // wait a small period of time 00133 Indicator = !Indicator; // toggle a led 00134 00135 XBee_vMain(); 00136 00137 memcpy(CommandTx,TxJob,9); 00138 XBee_u8TxRq = 1; 00139 00140 for(int i=0;i<BUFFER_SIZE;i++) 00141 { 00142 TxJob[i]++; 00143 } 00144 00145 } 00146 } 00147 #endif 00148
Generated on Sat Jul 23 2022 03:38:06 by
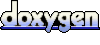