
This is a simple program to work with RedBearLab BLE Controller App. Type something from the Terminal to send to the BLEController App or vice verse. Characteristics received from App will print on Terminal.
Dependencies: BLE_API mbed nRF51822
Fork of nRF51822_Chat_Softswitch by
main.cpp
00001 /* 00002 00003 Copyright (c) 2012-2014 RedBearLab 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 and associated documentation files (the "Software"), to deal in the Software without restriction, 00007 including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, 00009 subject to the following conditions: 00010 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR 00014 PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE 00015 FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00016 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 00018 */ 00019 00020 /* 00021 * The application works with the BLEController iOS/Android App. 00022 * Type something from the Terminal to send 00023 * to the BLEController App or vice verse. 00024 * Characteristics received from App will print on Terminal. 00025 */ 00026 00027 #include "mbed.h" 00028 #include "ble/BLE.h" 00029 #include <stdlib.h> 00030 #include <stdio.h> 00031 00032 00033 #define BLE_UUID_TXRX_SERVICE 0x0000 /**< The UUID of the Nordic UART Service. */ 00034 #define BLE_UUID_TX_CHARACTERISTIC 0x0002 /**< The UUID of the TX Characteristic. */ 00035 #define BLE_UUIDS_RX_CHARACTERISTIC 0x0003 /**< The UUID of the RX Characteristic. */ 00036 00037 #define TXRX_BUF_LEN 20 00038 00039 BLE ble; 00040 00041 Serial pc(USBTX, USBRX); 00042 00043 00044 // The Nordic UART Service 00045 static const uint8_t uart_base_uuid[] = {0x71, 0x3D, 0, 0, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00046 static const uint8_t uart_tx_uuid[] = {0x71, 0x3D, 0, 3, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00047 static const uint8_t uart_rx_uuid[] = {0x71, 0x3D, 0, 2, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00048 static const uint8_t uart_base_uuid_rev[] = {0x1E, 0x94, 0x8D, 0xF1, 0x48, 0x31, 0x94, 0xBA, 0x75, 0x4C, 0x3E, 0x50, 0, 0, 0x3D, 0x71}; 00049 00050 00051 uint8_t txPayload[TXRX_BUF_LEN] = {0,}; 00052 uint8_t rxPayload[TXRX_BUF_LEN] = {0,}; 00053 00054 static uint8_t rx_buf[TXRX_BUF_LEN]; 00055 static uint8_t rx_len=0; 00056 00057 00058 GattCharacteristic txCharacteristic (uart_tx_uuid, txPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00059 00060 GattCharacteristic rxCharacteristic (uart_rx_uuid, rxPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00061 00062 GattCharacteristic *uartChars[] = {&txCharacteristic, &rxCharacteristic}; 00063 00064 GattService uartService(uart_base_uuid, uartChars, sizeof(uartChars) / sizeof(GattCharacteristic *)); 00065 00066 int counter=10; 00067 int counter2=0; 00068 00069 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00070 { 00071 pc.printf("Disconnected \r\n"); 00072 pc.printf("Restart advertising \r\n"); 00073 ble.startAdvertising(); 00074 } 00075 00076 void WrittenHandler(const GattWriteCallbackParams *Handler) 00077 { 00078 uint8_t buf[TXRX_BUF_LEN]; 00079 uint16_t bytesRead, index; 00080 00081 if (Handler->handle == txCharacteristic.getValueAttribute().getHandle()) 00082 { 00083 ble.readCharacteristicValue(txCharacteristic.getValueAttribute().getHandle(), buf, &bytesRead); 00084 memset(txPayload, 0, TXRX_BUF_LEN); 00085 memcpy(txPayload, buf, TXRX_BUF_LEN); 00086 pc.printf("WriteHandler \r\n"); 00087 pc.printf("Length: "); 00088 pc.putc(bytesRead); 00089 pc.printf("\r\n"); 00090 pc.printf("Data: "); 00091 for(index=0; index<bytesRead; index++) 00092 { 00093 pc.putc(txPayload[index]); 00094 } 00095 pc.printf("\r\n"); 00096 } 00097 } 00098 00099 /* serial interupt (from PC) calls this function */ 00100 void uartCB(void) 00101 { 00102 rx_len = 0; 00103 /* while text in the serial buffer from the PC */ 00104 while(pc.readable()) 00105 { 00106 /* load characters from serial buffer into rx_buf array, one by one */ 00107 rx_buf[rx_len++] = pc.getc(); 00108 /*check if rx_buf array is too long, ends in 'space' or ends 'carrage return' */ 00109 if(rx_len>=20 || rx_buf[rx_len-1]=='\0' || rx_buf[rx_len-1]=='\n') 00110 { 00111 /* update the rxCharacteristic with the rx_buf data fromm the PC */ 00112 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), rx_buf, rx_len); 00113 /* print debug info and reset */ 00114 pc.printf("RecHandler \r\n"); 00115 pc.printf("Length: "); 00116 pc.putc(rx_len); 00117 pc.printf("\r\n"); 00118 rx_len = 0; 00119 break; 00120 } 00121 } 00122 } 00123 00124 void dummyticker(void) { 00125 00126 rx_len = 0; 00127 char counterString[2]; 00128 00129 counter2++; 00130 if (counter2 > 2){ 00131 counter2 = 1; 00132 } 00133 00134 /* this could be done more efficiently using sprinf */ 00135 if ( counter2 == 1) { 00136 pc.printf("press1\n"); 00137 /* this only seems to work with exactly 20 characters */ 00138 char rx_message[] = "SWITCH1-901234567-"; 00139 sprintf(counterString, "%d\n", counter); 00140 strncat(rx_message, counterString,20); 00141 pc.printf(rx_message); 00142 00143 while(rx_len<20 && rx_buf[rx_len-1]!='\0' && rx_buf[rx_len-1]!='\n'){ 00144 00145 rx_buf[rx_len] = (int) rx_message[rx_len++]; 00146 } 00147 00148 } 00149 if ( counter2 == 2) { 00150 pc.printf("press2\n"); 00151 char rx_message[] = "SWITCH2-901234567-"; 00152 sprintf(counterString, "%d\n", counter); 00153 strncat(rx_message, counterString,20); 00154 pc.printf(rx_message); 00155 00156 while(rx_len<20 && rx_buf[rx_len-1]!='\0' && rx_buf[rx_len-1]!='\n'){ 00157 00158 rx_buf[rx_len] = (int) rx_message[rx_len++]; 00159 } 00160 } 00161 00162 if ( counter2 == 3) { 00163 pc.printf("press2\n"); 00164 char rx_message[] = "SWITCH2-901234567-"; 00165 sprintf(counterString, "%d\n", counter); 00166 strncat(rx_message, counterString,20); 00167 pc.printf(rx_message); 00168 00169 while(rx_len<20 && rx_buf[rx_len-1]!='\0' && rx_buf[rx_len-1]!='\n'){ 00170 00171 rx_buf[rx_len] = (int) rx_message[rx_len++]; 00172 } 00173 } 00174 00175 if ( counter2 == 4) { 00176 pc.printf("press2\n"); 00177 char rx_message[] = "SWITCH2-901234567-"; 00178 sprintf(counterString, "%d\n", counter); 00179 strncat(rx_message, counterString,20); 00180 pc.printf(rx_message); 00181 00182 while(rx_len<20 && rx_buf[rx_len-1]!='\0' && rx_buf[rx_len-1]!='\n'){ 00183 00184 rx_buf[rx_len] = (int) rx_message[rx_len++]; 00185 } 00186 } 00187 00188 if ( counter2 == 5) { 00189 pc.printf("press2\n"); 00190 char rx_message[] = "SWITCH2-901234567-"; 00191 sprintf(counterString, "%d\n", counter); 00192 strncat(rx_message, counterString,20); 00193 pc.printf(rx_message); 00194 00195 while(rx_len<20 && rx_buf[rx_len-1]!='\0' && rx_buf[rx_len-1]!='\n'){ 00196 00197 rx_buf[rx_len] = (int) rx_message[rx_len++]; 00198 } 00199 } 00200 00201 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), rx_buf, rx_len); 00202 00203 pc.printf("button press\n"); 00204 pc.printf("Length: "); 00205 pc.putc(rx_len); 00206 pc.printf("\r\n"); 00207 rx_len = 0; 00208 counter++; 00209 if (counter > 99) { 00210 counter = 0; 00211 } 00212 } 00213 00214 int main(void) 00215 { 00216 ble.init(); 00217 ble.onDisconnection(disconnectionCallback); 00218 00219 /* bluetooth data interrupt */ 00220 /* when data sent from client (phone) call 'WrittenHandler' */ 00221 ble.onDataWritten(WrittenHandler); 00222 00223 pc.baud(9600); 00224 pc.printf("SimpleChat Init \r\n"); 00225 00226 /* serial interupt*/ 00227 /* when text typed into PC terminal call 'uartCB' */ 00228 pc.attach( uartCB , pc.RxIrq); 00229 00230 00231 00232 // setup advertising 00233 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00234 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00235 ble.accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, 00236 (const uint8_t *)"Biscuit", sizeof("Biscuit") - 1); 00237 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00238 (const uint8_t *)uart_base_uuid_rev, sizeof(uart_base_uuid)); 00239 // 100ms; in multiples of 0.625ms. 00240 ble.setAdvertisingInterval(160); 00241 00242 ble.addService(uartService); 00243 00244 ble.startAdvertising(); 00245 pc.printf("Advertising Start \r\n"); 00246 00247 /* ticker interupt */ 00248 /* timer will call 'dummyticker' every X seconds */ 00249 Ticker ticker; 00250 ticker.attach(dummyticker, 3); 00251 00252 while(1) 00253 { 00254 ble.waitForEvent(); 00255 } 00256 } 00257 00258 00259 00260 00261 00262 00263 00264 00265 00266 00267 00268 00269 00270 00271 00272 00273
Generated on Tue Aug 16 2022 12:07:02 by
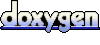