
panneau
Dependencies: mbed
main.cpp
00001 /** 00002 * LED Matrix library for http://www.seeedstudio.com/depot/ultrathin-16x32-red-led-matrix-panel-p-1582.html 00003 * The LED Matrix panel has 32x16 pixels. Several panel can be combined together as a large screen. 00004 * 00005 * Coordinate & Connection (mbed -> panel 0 -> panel 1 -> ...) 00006 * (0, 0) (0, 0) 00007 * +--------+--------+--------+ +--------+--------+ 00008 * | 5 | 3 | 1 | | 1 | 0 | 00009 * | | | | | | |<----- mbed 00010 * +--------+--------+--------+ +--------+--------+ 00011 * | 4 | 2 | 0 | (64, 16) 00012 * | | | |<----- mbed 00013 * +--------+--------+--------+ 00014 * (96, 32) 00015 * 00016 */ 00017 00018 #include "mbed.h" 00019 #include "LEDMatrix.h" 00020 #include "smallFont.h" 00021 00022 #define WIDTH 128 00023 #define HEIGHT 64 00024 00025 BusOut leds(LED1, LED2, LED3, LED4); 00026 //stb c'est le latch 00027 // LEDMatrix(a, b, c, d, oe_1, r3, r4, b3, b4, g3, g4, g1, r1, b1, r2, g2, b2, stb_1, clk_1,); 00028 LEDMatrix matrix(p12, p13, p14, p15, p18, p25, p23, p24, p21, p26, p22, p9, p8, p10, p7, p11, p6, p17, p16); 00029 Ticker scanner; 00030 00031 // Display Buffer 00032 uint8_t displaybuf[2][WIDTH *HEIGHT] = { 00033 0x00 00034 }; 00035 00036 // Instantiation de Led 00037 DigitalOut Led(LED1); 00038 00039 // Initialisation de la liaison Bluetooth 00040 Serial Bluetooth(p28, p27); // tx, rx 00041 00042 // 16 * 8 digital font 00043 const uint8_t digitals[] = { 00044 0x00, 0x1C, 0x36, 0x63, 0x63, 0x63, 0x63, 0x63, 0x63, 0x63, 0x36, 0x1C, 0x00, 0x00, 0x00, 0x00, // 0 00045 0x00, 0x18, 0x78, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x7E, 0x00, 0x00, 0x00, 0x00, // 1 00046 0x00, 0x3E, 0x63, 0x63, 0x63, 0x06, 0x06, 0x0C, 0x18, 0x30, 0x63, 0x7F, 0x00, 0x00, 0x00, 0x00, // 2 00047 0x00, 0x3E, 0x63, 0x63, 0x06, 0x1C, 0x06, 0x03, 0x03, 0x63, 0x66, 0x3C, 0x00, 0x00, 0x00, 0x00, // 3 00048 0x00, 0x06, 0x0E, 0x1E, 0x36, 0x36, 0x66, 0x66, 0x7F, 0x06, 0x06, 0x1F, 0x00, 0x00, 0x00, 0x00, // 4 00049 0x00, 0x7F, 0x60, 0x60, 0x60, 0x7C, 0x76, 0x03, 0x03, 0x63, 0x66, 0x3C, 0x00, 0x00, 0x00, 0x00, // 5 00050 0x00, 0x1E, 0x36, 0x60, 0x60, 0x7C, 0x76, 0x63, 0x63, 0x63, 0x36, 0x1C, 0x00, 0x00, 0x00, 0x00, // 6 00051 0x00, 0x7F, 0x66, 0x66, 0x0C, 0x0C, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x00, 0x00, 0x00, 0x00, // 7 00052 0x00, 0x3E, 0x63, 0x63, 0x63, 0x36, 0x1C, 0x36, 0x63, 0x63, 0x63, 0x3E, 0x00, 0x00, 0x00, 0x00, // 8 00053 0x00, 0x1C, 0x36, 0x63, 0x63, 0x63, 0x37, 0x1F, 0x03, 0x03, 0x36, 0x3C, 0x00, 0x00, 0x00, 0x00, // 9 00054 }; 00055 00056 // (x, y) top-left position, x should be multiple of 8 00057 void drawDigital(uint16_t x, uint16_t y, uint8_t n) 00058 { 00059 /* if ((n >= 10) || (0 != (x % 8))) { 00060 return; 00061 } 00062 00063 uint8_t *pDst = displaybuf + y * (WIDTH / 8) + x / 8; 00064 const uint8_t *pSrc = digitals + n * 16; 00065 for (uint8_t i = 0; i < 16; i++) { 00066 *pDst = *pSrc; 00067 pDst += WIDTH / 8; 00068 pSrc++; 00069 }*/ 00070 } 00071 00072 00073 void scan() 00074 { 00075 matrix.scan(); 00076 } 00077 00078 void setup() 00079 { 00080 // Initialisation Communication et premier message Bluetooth 00081 Bluetooth.baud(9600);//vitesse de communication 00082 Bluetooth.format(8,SerialBase::None,1);// 00083 00084 Bluetooth.printf("\r\n Setup OK !! \r\n"); 00085 00086 // Clignotement led 00087 Led = 1; 00088 wait(1); 00089 Led = 0; 00090 wait(1); 00091 Led = 1; 00092 } //End setup 00093 00094 int main() 00095 { 00096 setup(); 00097 int i, j, c=1; 00098 00099 char carac; 00100 initFonts(); 00101 00102 matrix.begin((uint8_t *)displaybuf, WIDTH, HEIGHT); 00103 scanner.attach(scan, 0.0005); 00104 00105 //defilement point 00106 /* for (i=0; i<64; i++) { 00107 for (j=0; j<128; j++) { 00108 matrix.clear(); 00109 matrix.drawPoint(j,i,4); 00110 matrix.swap(); 00111 while (!matrix.synchro()); 00112 } 00113 } 00114 while (1); 00115 */ 00116 /* while(1) { 00117 if(Bluetooth.readable()) // établir la connection 00118 { 00119 carac = Bluetooth.getc(); //On stocke le caractère envoyé dans la variable qu'on tape sur l'interface 00120 00121 switch(carac) { 00122 00123 case 'A' : 00124 00125 for (i=138; i>-180; i-=2) { 00126 matrix.clear(); 00127 matrix.drawCharString(i,5,"Le meilleur ",c,fonts[1]); 00128 matrix.swap(); 00129 wait(0.05); 00130 while (!matrix.synchro()); 00131 } 00132 c++; 00133 if (c==8) c=1; 00134 break; 00135 00136 case 'B' : 00137 00138 for (i=138; i>-180; i-=2) { 00139 matrix.clear(); 00140 matrix.drawCharString(i,5,"IUT Cachan ",c,fonts[1]); 00141 matrix.swap(); 00142 wait(0.05); 00143 while (!matrix.synchro()); 00144 } 00145 c++; 00146 if (c==8) c=1; 00147 break; 00148 } 00149 } 00150 } // end while */ 00151 00152 // matrix.clear(); 00153 00154 uint8_t count = 0; 00155 00156 //France 00157 /* matrix.drawRect(0,0,21,64,4); 00158 matrix.drawRect(21,0,43,64,7); 00159 matrix.drawRect(43,0,64,64,1);*/ 00160 00161 matrix.drawCharString(78,0,"3",7,fonts[2]); 00162 matrix.drawCharString(14,0,"3",7,fonts[2]); 00163 matrix.swap(); 00164 while (!matrix.synchro()); 00165 wait(1); 00166 matrix.clear(); 00167 00168 matrix.drawCharString(78,0,"2",7,fonts[2]); 00169 matrix.drawCharString(14,0,"2",7,fonts[2]); 00170 matrix.swap(); 00171 while (!matrix.synchro()); 00172 wait(1); 00173 matrix.clear(); 00174 00175 matrix.drawCharString(78,0,"1",7,fonts[2]); 00176 matrix.drawCharString(14,0,"1",7,fonts[2]); 00177 matrix.swap(); 00178 while (!matrix.synchro()); 00179 wait(1); 00180 00181 00182 while (1) { 00183 00184 00185 00186 00187 00188 for (i=200; i>-400; i-=4) { 00189 matrix.clear(); 00190 matrix.drawCharString(i,0,"Comment est votre blanquette ? ",c,fonts[1]); 00191 matrix.drawCharString(i,32," ma blanquette est bonne ",c,fonts[1]); 00192 matrix.swap(); 00193 wait(0.05); 00194 while (!matrix.synchro()); 00195 } 00196 c++; 00197 if (c==8) c=1; 00198 00199 // for (i=64; i>-192; i--) { 00200 00201 // matrix.drawChar(0,0,'A',2,fonts[0]); 00202 //matrix.drawChar(10,0,'A',2,fonts[1]); 00203 //matrix.drawChar(20,0,'A',3,fonts[2]); 00204 00205 } 00206 00207 } 00208 00209
Generated on Fri Aug 5 2022 10:36:44 by
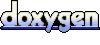