
panneau
Dependencies: mbed
LEDMatrix.h
00001 /** 00002 * LED Matrix library for http://www.seeedstudio.com/depot/ultrathin-16x32-red-led-matrix-panel-p-1582.html 00003 * The LED Matrix panel has 32x16 pixels. Several panel can be combined together as a large screen. 00004 * 00005 * Coordinate & Connection (mbed -> panel 0 -> panel 1 -> ...) 00006 * (0, 0) (0, 0) 00007 * +--------+--------+--------+ +--------+--------+ 00008 * | 5 | 3 | 1 | | 1 | 0 | 00009 * | | | | | | |<----- mbed 00010 * +--------+--------+--------+ +--------+--------+ 00011 * | 4 | 2 | 0 | (64, 16) 00012 * | | | |<----- mbed 00013 * +--------+--------+--------+ 00014 * (96, 32) 00015 * Copyright (c) 2013 Seeed Technology Inc. 00016 * @auther Yihui Xiong 00017 * @date Nov 7, 2013 00018 * @license Apache 00019 */ 00020 00021 00022 #ifndef __LED_MATRIX_H__ 00023 #define __LED_MATRIX_H__ 00024 00025 #include "mbed.h" 00026 #include "fonts.h" 00027 00028 class LEDMatrix { 00029 public: 00030 LEDMatrix(PinName pinA, PinName pinB, PinName pinC, PinName pinD, PinName pinOE, 00031 PinName pinR1, PinName pinR2, PinName pinB1, PinName pinB2, PinName pinG1, PinName pinG2, 00032 PinName pinG3,PinName pinR3, PinName pinB3, PinName pinR4, PinName pinG4,PinName pinB4, 00033 PinName pinSTB, PinName pinCLK); 00034 00035 /** 00036 * set the display's display buffer and number, the buffer's size must be not less than 512 * number / 8 bytes 00037 * @param displaybuf display buffer 00038 * @param number panels' number 00039 */ 00040 void begin(uint8_t *displaybuf, uint16_t width, uint16_t height); 00041 00042 /** 00043 * draw a point 00044 * @param x x 00045 * @param y y 00046 * @param pixel 0: led off, >0: led on 00047 */ 00048 void drawPoint(uint16_t x, uint16_t y, uint8_t pixel); 00049 00050 /** 00051 * draw a rect 00052 * @param (x1, y1) top-left position 00053 * @param (x2, y2) bottom-right position, not included in the rect 00054 * @param pixel 0: rect off, >0: rect on 00055 */ 00056 void drawRect(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint8_t pixel); 00057 00058 int drawChar(uint16_t x, uint16_t y, char c, uint8_t pixel, Font *font); 00059 00060 void drawCharString (uint16_t x, uint16_t y, char *c, uint8_t pixel, Font *font); 00061 00062 void drawCharBig(uint16_t x, uint16_t y, char c, uint8_t pixel); 00063 00064 /** 00065 * draw a image 00066 * @param (x1, y1) top-left position 00067 * @param (x2, y2) bottom-right position, not included in the rect 00068 * @param pixels contents, 1 bit to 1 led 00069 */ 00070 void drawImage(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint8_t *image); 00071 00072 /** 00073 * Set screen buffer to zero 00074 */ 00075 void clear(); 00076 00077 /** 00078 * turn off 1/16 leds and turn on another 1/16 leds 00079 */ 00080 void scan(); 00081 00082 void reverse(); 00083 00084 uint8_t isReversed(); 00085 00086 void on(); 00087 00088 void off(); 00089 00090 void swap(); 00091 00092 int synchro(); 00093 00094 private: 00095 DigitalOut a, b, c, d, oe, r1, r2, b1, b2, g1, g2, g3, r3, b3, r4, g4, b4, stb, clk; 00096 uint8_t *displaybuf, *memoBuf, *drawBuf; 00097 uint16_t width; 00098 uint16_t height; 00099 uint8_t mask; 00100 uint8_t state; 00101 int bufferIndex; 00102 volatile int flagSwap; 00103 }; 00104 00105 #endif
Generated on Fri Aug 5 2022 10:36:44 by
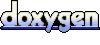