
panneau
Dependencies: mbed
LEDMatrix.cpp
00001 /** 00002 * LED Matrix library for http://www.seeedstudio.com/depot/ultrathin-16x32-red-led-matrix-panel-p-1582.html 00003 * The LED Matrix panel has 32x16 pixels. Several panel can be combined together as a large screen. 00004 * 00005 * Coordinate & Connection (mbed -> panel 0 -> panel 1 -> ...) 00006 * (0, 0) (0, 0) 00007 * +--------+--------+--------+ +--------+--------+ 00008 * | 5 | 3 | 1 | | 1 | 0 | 00009 * | | | | | | |<----- mbed 00010 * +--------+--------+--------+ +--------+--------+ 00011 * | 4 | 2 | 0 | (64, 16) 00012 * | | | |<----- mbed 00013 * +--------+--------+--------+ 00014 * (96, 32) 00015 * Copyright (c) 2013 Seeed Technology Inc. 00016 * @auther Yihui Xiong 00017 * @date Nov 8, 2013 00018 * @license Apache 00019 */ 00020 00021 #include "LEDMatrix.h" 00022 #include "mbed.h" 00023 00024 #if 0 00025 #define ASSERT(e) if (!(e)) { Serial.println(#e); while (1); } 00026 #else 00027 #define ASSERT(e) 00028 #endif 00029 00030 LEDMatrix::LEDMatrix(PinName pinA, PinName pinB, PinName pinC, PinName pinD, PinName pinOE, 00031 PinName pinR1, PinName pinR2, PinName pinB1, PinName pinB2, PinName pinG1, PinName pinG2, 00032 PinName pinG3,PinName pinR3, PinName pinB3, PinName pinR4, PinName pinG4,PinName pinB4, 00033 PinName pinSTB, PinName pinCLK) : 00034 a(pinA), b(pinB), c(pinC), d(pinD), oe(pinOE), r1(pinR1), r2(pinR2), b1(pinB1), b2(pinB2), g1(pinG1), g2(pinG2), g3(pinG3), r3(pinR3), b3(pinB3),r4(pinR4), g4(pinG4), b4(pinB4), stb(pinSTB), clk(pinCLK) 00035 { 00036 oe = 1; 00037 clk = 1; 00038 stb = 1; 00039 mask = 0xff; 00040 state = 0; 00041 bufferIndex = 0; 00042 flagSwap = 0; 00043 } 00044 00045 void LEDMatrix::begin(uint8_t *displaybuf, uint16_t width, uint16_t height) 00046 { 00047 this->displaybuf = displaybuf; 00048 memoBuf = displaybuf; 00049 drawBuf = memoBuf + width * height; 00050 this->width = width; 00051 this->height = height; 00052 00053 state = 1; 00054 } 00055 00056 void LEDMatrix::drawPoint(uint16_t x, uint16_t y, uint8_t pixel) 00057 { 00058 if (x>=width) return; 00059 if (y>=height) return; 00060 drawBuf[x+ width * y] = pixel & 0x07; 00061 } 00062 00063 void LEDMatrix::drawRect(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint8_t pixel) 00064 { 00065 for (uint16_t x = x1; x < x2; x++) { 00066 for (uint16_t y = y1; y < y2; y++) { 00067 drawPoint(x, y, pixel); 00068 } 00069 } 00070 } 00071 00072 int LEDMatrix::drawChar(uint16_t x, uint16_t y, char c, uint8_t pixel, Font *font) 00073 { 00074 int i, j,k, col, max = 0; 00075 for (i=0; i<font->hauteur(); i++) { 00076 for (k=0; k<font->largeur(); k++) { 00077 col = font->octet(c, i, k); 00078 for (j=8; j>=0; j--) { 00079 if (col & 0x01 != 0) { 00080 int dx = j+8*k; 00081 if (dx > max) max = dx; 00082 drawPoint(x + dx, y+i, pixel); 00083 } 00084 col = col >> 1; 00085 } 00086 } 00087 } 00088 if (c==' ') { 00089 return 5*font->largeur(); 00090 } else { 00091 return max + font->largeur(); 00092 } 00093 } 00094 00095 00096 void LEDMatrix::drawCharString (uint16_t x, uint16_t y, char *c, uint8_t pixel,Font *font) 00097 { 00098 int i=0; 00099 while(c[i] != '\0') { 00100 int dx = drawChar(x,y,c[i],pixel,font); 00101 i++; 00102 x += dx; 00103 } 00104 } 00105 00106 00107 void LEDMatrix::drawImage(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint8_t *image) 00108 { 00109 ASSERT(0 == ((x2 - x1) % 8)); 00110 00111 for (uint16_t x = x1; x < x2; x++) { 00112 for (uint16_t y = y1; y < y2; y++) { 00113 uint8_t *byte = image + x * 8 + y / 8; 00114 uint8_t bit = 7 - (y % 8); 00115 uint8_t pixel = (*byte >> bit) & 1; 00116 00117 drawPoint(x, y, pixel); 00118 } 00119 } 00120 } 00121 00122 void LEDMatrix::clear() 00123 { 00124 uint8_t *ptr = drawBuf; 00125 for (uint16_t i = 0; i < (width * height); i++) { 00126 *ptr = 0x00; 00127 ptr++; 00128 } 00129 } 00130 00131 void LEDMatrix::reverse() 00132 { 00133 mask = ~mask; 00134 } 00135 00136 uint8_t LEDMatrix::isReversed() 00137 { 00138 return mask; 00139 } 00140 00141 void LEDMatrix::scan() 00142 { 00143 static uint8_t row = 0; 00144 00145 if (!state) { 00146 return; 00147 } 00148 00149 int debut1 = 2048 + row * width ; //1024 00150 int debut2 = 6144 + row * width ; //3072 00151 int debut3 = 2112 + row * width ; 00152 int debut4 = 6208 + row * width ; 00153 00154 for (uint8_t i = 0; i <64 ; i++) { 00155 clk = 0; 00156 r1 = displaybuf[debut1+i] & 0x01; 00157 r2 = displaybuf[debut2+i] & 0x01; 00158 r3 = displaybuf[debut3+i] & 0x01; 00159 r4 = displaybuf[debut4+i] & 0x01; 00160 g1 = (displaybuf[debut1+i] & 0x02) >> 1; 00161 g2 = (displaybuf[debut2+i] & 0x02) >> 1; 00162 g3 = (displaybuf[debut3+i] & 0x02) >> 1; 00163 g4 = (displaybuf[debut4+i] & 0x02) >> 1; 00164 b1 = (displaybuf[debut1+i] & 0x04) >> 2; 00165 b2 = (displaybuf[debut2+i] & 0x04) >> 2; 00166 b3 = (displaybuf[debut3+i] & 0x04) >> 2; 00167 b4 = (displaybuf[debut4+i] & 0x04) >> 2; 00168 clk = 1; 00169 } 00170 00171 debut1 = 0 + row * width;//0 00172 debut2 = 4096 + row * width;//2048 00173 debut3 = 64 + row * width; 00174 debut4 = 4160 + row * width; 00175 00176 for (uint8_t i = 0; i <64 ; i++) { 00177 clk = 0; 00178 r1 = displaybuf[debut1+i] & 0x01; 00179 r2 = displaybuf[debut2+i] & 0x01; 00180 r3 = displaybuf[debut3+i] & 0x01; 00181 r4 = displaybuf[debut4+i] & 0x01; 00182 g1 = (displaybuf[debut1+i] & 0x02) >> 1; 00183 g2 = (displaybuf[debut2+i] & 0x02) >> 1; 00184 g3 = (displaybuf[debut3+i] & 0x02) >> 1; 00185 g4 = (displaybuf[debut4+i] & 0x02) >> 1; 00186 b1 = (displaybuf[debut1+i] & 0x04) >> 2; 00187 b2 = (displaybuf[debut2+i] & 0x04) >> 2; 00188 b3 = (displaybuf[debut3+i] & 0x04) >> 2; 00189 b4 = (displaybuf[debut4+i] & 0x04) >> 2; 00190 clk = 1; 00191 } 00192 00193 oe = 1; // disable display 00194 00195 // select row 00196 a = (row & 0x01); 00197 b = (row & 0x02); 00198 c = (row & 0x04); 00199 d = (row & 0x08); 00200 00201 // latch data 00202 stb = 0; 00203 stb = 1; 00204 00205 oe = 0; // enable display 00206 00207 row=(row + 1) & 0x0F; 00208 00209 if (row==0) { 00210 if (flagSwap == 1) { 00211 if (bufferIndex==0) { 00212 bufferIndex = 1; 00213 displaybuf = memoBuf + width*height; 00214 drawBuf = memoBuf; 00215 } else { 00216 bufferIndex = 0; 00217 displaybuf = memoBuf; 00218 drawBuf = memoBuf + width*height; 00219 } 00220 flagSwap = 0; 00221 } 00222 } 00223 } 00224 00225 void LEDMatrix::on() 00226 { 00227 state = 1; 00228 } 00229 00230 void LEDMatrix::off() 00231 { 00232 state = 0; 00233 oe = 1; 00234 } 00235 00236 void LEDMatrix::swap() 00237 { 00238 flagSwap = 1; 00239 } 00240 00241 int LEDMatrix::synchro() 00242 { 00243 return !flagSwap; 00244 }
Generated on Fri Aug 5 2022 10:36:44 by
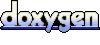