Update version of EALib.
Dependencies: FATFileSystem
Fork of EALib by
LcdController Class Reference
A LCD controller interface. More...
#include <LcdController.h>
Data Structures | |
class | Config |
LCD configuration. More... | |
Public Member Functions | |
LcdController () | |
Create a LCD controller interface. | |
int | open (LcdController::Config *cfg) |
Open and initialize the LCD controller with the given LCD configuration. | |
int | close () |
Close the LCD controller. | |
int | setFrameBuffer (uint32_t address) |
Set and activate the address of the frame buffer to use. | |
int | setPower (bool on) |
Turn on/off the power to the display. |
Detailed Description
A LCD controller interface.
#include "mbed.h" #include "LcdController.h" LcdController::Config innolux( 45, 17, 2, 800, 22, 22, 2, 480, false, false, true, true, true, LcdController::Bpp_16_565, 36000000, LcdController::Tft, false); int main(void) { LcdController lcd; lcd.open(&innolux); lcd.setFrameBuffer(frameBuffer); lcd.setPower(true); // draw on the frame buffer ... }
Definition at line 58 of file LcdController.h.
Constructor & Destructor Documentation
LcdController | ( | ) |
Create a LCD controller interface.
Definition at line 156 of file LcdController.cpp.
Member Function Documentation
int close | ( | ) |
Close the LCD controller.
- Returns:
- 0 on success 1 on failure
Definition at line 177 of file LcdController.cpp.
int open | ( | LcdController::Config * | cfg ) |
Open and initialize the LCD controller with the given LCD configuration.
- Parameters:
-
cfg LCD configuration
- Returns:
- 0 on success 1 on failure
Definition at line 160 of file LcdController.cpp.
int setFrameBuffer | ( | uint32_t | address ) |
Set and activate the address of the frame buffer to use.
It is the content of the frame buffer that is shown on the display. All the drawing on the frame buffer can be done 'offline' and whenever it should be shown this function can be called with the address of the offline frame buffer.
- Parameters:
-
address Memory address of the frame buffer
- Returns:
- 0 on success 1 on failure
Definition at line 194 of file LcdController.cpp.
int setPower | ( | bool | on ) |
Turn on/off the power to the display.
- Parameters:
-
on true to turn on, false to turn off
- Returns:
- 0 on success 1 on failure
Definition at line 202 of file LcdController.cpp.
Generated on Thu Jul 14 2022 09:42:14 by
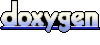