A Roboteq controller c++ interface to allow a Brushless motor control on a CAN bus. Used for a FBL2360 reference.
Dependencies: CAN_FIFO_Triporteur
object_dict.h
00001 // Source : https://www.roboteq.com/index.php/docman/motor-controllers-documents-and-files/documentation/user-manual/272-roboteq-controllers-user-manual-v17/file 00002 00003 // RUNTIME commands 00004 //Command Name Index Sub(Hex) Entry Name Data Type & Access 00005 #define cCANGO 0x2000 //01 Set Motor Command, ch1 CG 00006 //02 Set Motor Command, ch2 00007 #define cP 0x2001 //01 to mm (1) Set Position, ch.1 S32 WO 00008 #define cS 0x2002 //01 to mm (1) Set Velocity S16 WO 00009 #define cC 0x2003 //01 to ee (2) Set Encoder Counter S32 WO 00010 #define cCB 0x2004 //01 to mm (1) Set Brushless Counter S32 WO 00011 #define cVAR 0x2005 //01 -vv (3) Set User Integer Variable S32 WO 00012 #define cAC 0x2006 //01 to mm (1) Set Acceleration S32 WO 00013 #define cDC 0x2007 //01 to mm (1) Set Deceleration S32 WO 00014 #define cDS 0x2008 //00 Set All Digital Out bits U8 WO 00015 #define cD1 0x2009 //00 Set Individual Digital Out bits U8 WO 00016 #define cD0 0x200A //00 Reset Individual Digital Out bits U8 WO 00017 #define cH 0x200B //01 to ee (2) Load Home Counter U8 WO 00018 #define cEX 0x200C //00 Emergency Shutdown U8 WO 00019 #define cMG 0x200D //00 Release Shutdown U8 WO 00020 #define cMS 0x200E //00 Stop in all modes U8 WO 00021 #define cPR 0x200F //01 to mm (1) Set Pos Relative S32 WO 00022 #define cPX 0x2010 //01 to mm (1) Set Next Pos Absolute S32 WO 00023 #define cPRX 0x2011 //01 to mm (1) Set Next Pos Relative S32 WO 00024 #define cAX 0x2012 //01 to mm (1) Set Next Acceleration S32 WO 00025 #define cDX 0x2013 //01 to mm (1) Set Next Deceleration S32 WO 00026 #define cSX 0x2014 //01 to mm (1) Set Next Velocity S32 WO 00027 #define cB 0x2015 //01 to bb (4) Set User Bool Variable S32 WO 00028 #define cEES 0x2017 //00 Save Config to Flash U8 WO 00029 00030 //RUNTIME Queries 00031 //Command Name Index Sub(Hex) Entry Name Data Type & Access 00032 #define qA 0x2100 //01 Read Motor Amps S16 RO 00033 #define qM 0x2101 //01 to mm (1) Read Actual Motor Command S16 RO 00034 #define qP 0x2102 //01 to mm (1) Read Applied Power Level S16 RO 00035 #define qS 0x2103 //01 to ee (2) Read Encoder Motor Speed, ch.1 S16 RO 00036 #define qC 0x2104 //01 to ee (2) Read Absolute Encoder Count S32 RO 00037 #define qCB 0x2105 //01 to mm (1) Read Absolute Brushless Counter S32 RO 00038 #define qVAR 0x2106 //01 to vv (3) Read User Integer Variable 1 S32 RO 00039 #define qSR 0x2107 //01 to ee (2) Read Encoder Motor Speed as 1/1000 of Max S16 RO 00040 #define qCR 0x2108 //01 to ee (2) Read Encoder Count Relative S32 RO 00041 #define qCBR 0x2109 //01 to mm (1) Read Brushless Count Relative S32 RO 00042 #define qBS 0x210A //01 to mm (1) Read BL Motor Speed in RPM S16 RO 00043 #define qBSR 0x210B //01 to mm (1) Read BL Motor Speed as 1/1000 of Max S16 RO 00044 #define qBA 0x210C //01 to mm (1) Read Battery Amps S16 RO 00045 #define qV 0x210D //01 to 03 Read VInt, VBat, 5VOut U16 RO 00046 #define qD 0x210E //00 Read All Digital Inputs U32 RO 00047 #define qT 0x210F //01 to tt+1(5) Read MCU and Transistor temperature S8 RO 00048 #define qF 0x2110 //01 to mm (1) Read Feedback S16 RO 00049 #define qFS 0x2111 //00 Read Status Flags U8 RO 00050 #define qFF 0x2112 //00 Read Fault Flags U8 RO 00051 #define qDO 0x2113 //00 Read Current Digital Outputs U8 RO 00052 #define qE 0x2114 //01 to mm (1) Read Closed Loop Error S32 RO 00053 #define qB 0x2115 //01 to bb (4) Read User Bool Variable S32 RO 00054 #define qCIS 0x2116 //01 to mm (1) Read Internal Serial Command S32 RO 00055 #define qCIA 0x2117 //01 to mm (1) Read Internal Analog Command S32 RO 00056 #define qCIP 0x2118 //01 to mm (1) Read Internal Pulse Command S32 RO 00057 #define qTM 0x2119 //00 Read Time U32 RO 00058 #define qK 0x211A //01 to 06 Read Spektrum Radio Capture U16 RO 00059 #define qDR 0x211B //01 to 06 Destination Pos Reached flag U8 RO 00060 #define qMGD 0x211D //01 to ss (9) Read Magsensor Track Detect U8 RO 00061 #define qMGT 0x211E //01 to ss*3(9) Read Magsensor Track Position, U8 RO 00062 #define qMGM 0x211F //01 to ss*3(9) Read Magsensor Markers U8 RO 00063 #define qMGS 0x2120 //01 to ss (9) Read Magsensor Status U16 RO 00064 #define qMGY 0x2121 //01 to ss (9) Read Magsensor Gyroscope S16 RO 00065 #define qFM 0x2122 //01 to mm (1) Read Motor Status Flags U16 RO 00066 #define qHS 0x2123 //01 to mm (1) Read Hall Sensor States U8 RO 00067 #define qDI 0x6400 //01 to dd (6) Read Individual Digital Input S32 RO 00068 #define qMGY 0x2121 //01 to 03 Read Magsensor Gyroscope S16 RO 00069 #define qFM 0x2122 //01 to mm (1) Read Motor Status Flags U16 RO 00070 #define qHS 0x2123 //01 to mm (1) Read Hall Sensor States U8 RO 00071 #define qTR 0x2125 //01 to mm (1) Read Destination Tracking S32 RO 00072 #define qANG 0x2132 //01 to mm (1) Read Rotor Angle S16 RO 00073 #define qFC 0x2135 //01 to mm (1) Read FOC Angle Correction S16 RO 00074 #define qSL 0x2136 //01 to mm(1) Read Slip S16 RO 00075 #define qAI 0x6401 //01 to aa (7) Read Analog Input S16 RO 00076 #define qAIC 0x6402 //01 to aa (7) Read Analog Input Converted S16 RO 00077 #define qPI 0x6403 //01 to pp (8) Read Pulse Input S16 RO 00078 #define qPIC 0x6404 //01 to pp (8) Read Pulse Input Converted S16 RO 00079 00080 /* Notes: Product-specific parameters. See datasheet 00081 (1) mm: Max number of motors 00082 (2) ee: Max number of encoders 00083 (3) vv: Max number of user integer variables 00084 (4) bb: Max number of user boolean variables 00085 (5) tt: Number of internal temperature sensors 00086 (6) dd: Number of digital inputs 00087 (7) aa: Number of analog inputs 00088 (8) pp: Number of pulse inputs 00089 (9) ss: Max number of Magsensors supported */
Generated on Tue Jul 12 2022 21:44:04 by
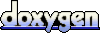