Updated i2c interface to work correctly with mBed and Hexiwear
Dependents: Hexi_Click_IRThermo_Example
Fork of MLX90614 by
mlx90614.cpp
00001 //Melexis Infrared Thermometer MLX90614 Library 00002 00003 //***************************************************************** 00004 // Build : 27/10/16 Dave Clarke 00005 // Only read thermo data. 00006 // 00007 // This program does not check CRC. 00008 // If you want to check CRC, please do it your self :) 00009 //****************************************************************// 00010 00011 #include "mlx90614.h" 00012 00013 MLX90614::MLX90614(I2C* i2c, uint8_t addr){ 00014 00015 this->i2caddress = addr; 00016 this->i2c = i2c; 00017 00018 } 00019 00020 00021 bool MLX90614::getTemp(float* temp_val){ 00022 00023 bool ch; // Check flag for ack 00024 char data[3]; // Raw data storage 00025 char ram[1] = {0x07}; // RAM address what temperature is stored 00026 char readaddr = 0xB5; // Read Data address 00027 uint16_t temp_thermo; 00028 00029 ch = i2c->write(i2caddress, ram, 1, true); // ping i2c with address and RAM location 00030 if (ch) return false; // check for ack 00031 00032 ch = i2c->read(readaddr, data , 3); // read raw temperature value 00033 if (ch) return false; // check for ack 00034 00035 temp_thermo |= data[1] << 8; // make MSB 00036 temp_thermo |= data[0]; // make LSB 00037 00038 *temp_val = ((float)temp_thermo * 0.02) - 273; //Convert kelvin to degree Celsius 00039 00040 return true; //load data successfully, return true 00041 } 00042 00043
Generated on Fri Jul 15 2022 02:23:28 by
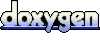