Simple temperature and humidity program example for Hexiwear featuring UART
Fork of HTU21D by
HTU21D.cpp
00001 // @author Alex Lipford 00002 // Georgia Institute of Technology 00003 // ECE 4180 Embeded Systems Design 00004 // Professor Hamblen 00005 // 10/19/2014 00006 00007 // @section LICENSE 00008 // ---------------------------------------------------------------------------- 00009 // "THE BEER-WARE LICENSE" (Revision 42): 00010 // <alexlipford@gmail.com> wrote this file. As long as you retain this notice you 00011 // can do whatever you want with this stuff. If we meet some day, and you think 00012 // this stuff is worth it, you can buy me a beer in return. 00013 // ---------------------------------------------------------------------------- 00014 00015 // @section DESCRIPTION 00016 // HTU21D Humidity and Temperature sensor. 00017 // Datasheet, specs, and information: 00018 // https://www.sparkfun.com/products/12064 00019 00020 00021 #include "HTU21D.h" 00022 00023 HTU21D::HTU21D(PinName sda, PinName scl) { 00024 00025 i2c_ = new I2C(sda, scl); 00026 //400KHz, as specified by the datasheet. 00027 i2c_->frequency(400000); 00028 } 00029 00030 int HTU21D::sample_ctemp(void) { 00031 00032 char tx[1]; 00033 char rx[2]; 00034 00035 tx[0] = TRIGGER_TEMP_MEASURE; // Triggers a temperature measure by feeding correct opcode. 00036 i2c_->write((HTU21D_I2C_ADDRESS << 1) & 0xFE, tx, 1); 00037 wait_ms(50); // Per datasheet, wait long enough for device to sample temperature 00038 00039 // Reads triggered measure 00040 i2c_->read((HTU21D_I2C_ADDRESS << 1) | 0x01, rx, 2); 00041 wait_ms(1); 00042 00043 // Algorithm from datasheet to compute temperature. 00044 unsigned int rawTemperature = ((unsigned int) rx[0] << 8) | (unsigned int) rx[1]; 00045 rawTemperature &= 0xFFFC; 00046 00047 float tempTemperature = rawTemperature / (float)65536; //2^16 = 65536 00048 float realTemperature = -46.85 + (175.72 * tempTemperature); //From page 14 00049 00050 return (int)realTemperature; 00051 } 00052 00053 int HTU21D::sample_ftemp(void){ 00054 int temptemp = sample_ctemp(); 00055 int ftemp = temptemp * 1.8 + 32; 00056 00057 return ftemp; 00058 } 00059 00060 int HTU21D::sample_ktemp(void){ 00061 int temptemp = sample_ctemp(); 00062 int ktemp = temptemp + 274; 00063 00064 return ktemp; 00065 } 00066 00067 int HTU21D::sample_humid(void) { 00068 00069 char tx[1]; 00070 char rx[2]; 00071 00072 00073 tx[0] = TRIGGER_HUMD_MEASURE; // Triggers a humidity measure by feeding correct opcode. 00074 i2c_->write((HTU21D_I2C_ADDRESS << 1) & 0xFE, tx, 1); 00075 wait_ms(16); // Per datasheet, wait long enough for device to sample humidity 00076 00077 // Reads triggered measure 00078 i2c_->read((HTU21D_I2C_ADDRESS << 1) | 0x01, rx, 2); 00079 wait_ms(1); 00080 00081 //Algorithm from datasheet. 00082 unsigned int rawHumidity = ((unsigned int) rx[0] << 8) | (unsigned int) rx[1]; 00083 00084 rawHumidity &= 0xFFFC; //Zero out the status bits but keep them in place 00085 00086 //Given the raw humidity data, calculate the actual relative humidity 00087 float tempRH = rawHumidity / (float)65536; //2^16 = 65536 00088 float rh = -6 + (125 * tempRH); //From page 14 00089 00090 return (int)rh; 00091 } 00092
Generated on Tue Jul 12 2022 21:43:35 by
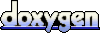