
Basic touch buttons example for Hexiwear
Dependencies: Hexi_KW40Z
main.cpp
00001 #include "mbed.h" 00002 #include "Hexi_KW40Z.h" 00003 00004 #define LED_ON 0 00005 #define LED_OFF 1 00006 00007 void StartHaptic(void); 00008 void StopHaptic(void const *n); 00009 00010 DigitalOut redLed(LED1); 00011 DigitalOut greenLed(LED2); 00012 DigitalOut blueLed(LED3); 00013 DigitalOut haptic(PTB9); 00014 00015 /* Define timer for haptic feedback */ 00016 RtosTimer hapticTimer(StopHaptic, osTimerOnce); 00017 00018 /* Instantiate the Hexi KW40Z Driver (UART TX, UART RX) */ 00019 KW40Z kw40z_device(PTE24, PTE25); 00020 00021 void ButtonUp(void) 00022 { 00023 StartHaptic(); 00024 00025 redLed = LED_ON; 00026 greenLed = LED_OFF; 00027 blueLed = LED_OFF; 00028 } 00029 00030 void ButtonDown(void) 00031 { 00032 StartHaptic(); 00033 00034 redLed = LED_OFF; 00035 greenLed = LED_ON; 00036 blueLed = LED_OFF; 00037 } 00038 00039 void ButtonRight(void) 00040 { 00041 StartHaptic(); 00042 00043 redLed = LED_OFF; 00044 greenLed = LED_OFF; 00045 blueLed = LED_ON; 00046 } 00047 00048 void ButtonLeft(void) 00049 { 00050 StartHaptic(); 00051 00052 redLed = LED_ON; 00053 greenLed = LED_ON; 00054 blueLed = LED_OFF; 00055 } 00056 00057 void ButtonSlide(void) 00058 { 00059 StartHaptic(); 00060 00061 redLed = LED_ON; 00062 greenLed = LED_ON; 00063 blueLed = LED_ON; 00064 } 00065 00066 int main() 00067 { 00068 /* Register callbacks to application functions */ 00069 kw40z_device.attach_buttonUp(&ButtonUp); 00070 kw40z_device.attach_buttonDown(&ButtonDown); 00071 kw40z_device.attach_buttonLeft(&ButtonLeft); 00072 kw40z_device.attach_buttonRight(&ButtonRight); 00073 kw40z_device.attach_buttonSlide(&ButtonSlide); 00074 00075 while (true) { 00076 Thread::wait(500); 00077 } 00078 } 00079 00080 void StartHaptic(void) 00081 { 00082 hapticTimer.start(50); 00083 haptic = 1; 00084 } 00085 00086 void StopHaptic(void const *n) { 00087 haptic = 0; 00088 hapticTimer.stop(); 00089 }
Generated on Tue Jul 12 2022 12:29:01 by
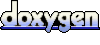