An mbed wrapper around the helium-client to communicate with the Helium Atom
Channel Class Reference
A Channel to an IoT platform. More...
#include <Helium.h>
Public Member Functions | |
Channel (Helium *helium) | |
Construct a channel. | |
int | begin (const char *name, int8_t *result) |
Begin communicating over a channel. | |
int | send (void const *data, size_t len, int8_t *result) |
Send data to this channel. | |
int | begin (const char *name, uint16_t *token) |
Asynchronous begin method. | |
int | send (void const *data, size_t len, uint16_t *token) |
Asynchornous send method. | |
int | poll_result (uint16_t token, int8_t *result, uint32_t retries=HELIUM_POLL_RETRIES_5S) |
Poll for a result token. | |
int | poll_data (void *data, size_t len, size_t *used, uint32_t retries=HELIUM_POLL_RETRIES_5S) |
Poll for data on a channel. | |
Data Fields | |
Helium * | helium |
The Helium Atom this channel uses to communicate. | |
Friends | |
class | Config |
Detailed Description
A Channel to an IoT platform.
Channels represent a delivery mechanism from the device to a number of supported back-end IoT platforms.
To use a channel, make sure you have it set up in your Helium Dashboard and then call Channel::begin with the channel name as you configured it on the Helium Dashboard. This will automatically ensure that the device is securely authenticated and registered with the channel.
Definition at line 131 of file Helium.h.
Constructor & Destructor Documentation
Construct a channel.
- Parameters:
-
helium The Helium Atom to communicate with
Definition at line 113 of file Helium.cpp.
Member Function Documentation
int begin | ( | const char * | name, |
int8_t * | result | ||
) |
Begin communicating over a channel.
Always call this method before calling Channel::send. Beginning communication will ensure that the device is authenticated and registered with the channel with the given name as configured in the Helium Dashboard.
The `result` value will be 0 if the channel was created successfully and non-`0` otherwise.
- Parameters:
-
name The name of the channel to authenticate with. result An out parameter for the result of the request.
Definition at line 125 of file Helium.cpp.
int begin | ( | const char * | name, |
uint16_t * | token | ||
) |
Asynchronous begin method.
The Channel::begin(const char *) method is a synchronous version of this method. Sending a request to a channel returns a `token` which can be used in a subsequent call to poll() to check for results from the remote channel.
- Parameters:
-
name The name of the channel to authenticate with. token The output parameter for the pending result token.
Definition at line 119 of file Helium.cpp.
int poll_data | ( | void * | data, |
size_t | len, | ||
size_t * | used, | ||
uint32_t | retries = HELIUM_POLL_RETRIES_5S |
||
) |
Poll for data on a channel.
This polls the Helium Atom for the given number of retries for any data on the channel.
If successful the result will be helium_status_OK and the given `data` buffer will be filled with the received data. The `used` out parameter will be set to the number of bytes read. Note that the maximum number of bytes that can be sent is set by HELIUM_MAX_DATA_SIZE.
- Parameters:
-
[out] data The data buffer to fill with received data len The available length of the data buffer [out] used On success the number of bytes used up in data retries The number of times to retry
Definition at line 169 of file Helium.cpp.
int poll_result | ( | uint16_t | token, |
int8_t * | result, | ||
uint32_t | retries = HELIUM_POLL_RETRIES_5S |
||
) |
Poll for a result token.
This polls the Helium Atom for the given number of retries for the result of a given `token`. Use HELIUM_POLL_RETRIES_5S for the recommended number of retries.
If succcessful the result will be helium_status_OK and the result value will be set to the result of the original request that the token represents.
- Parameters:
-
token The token to check for result[out] The result of the given request token retries The number of times to retry
Definition at line 163 of file Helium.cpp.
int send | ( | void const * | data, |
size_t | len, | ||
int8_t * | result | ||
) |
Send data to this channel.
Send data to a given channel. The given data is sent to the configured channel and the result as returned by the channel is put in the `result` output variable. The result value is `0` for success and non-`0` if an error occurred.
- Note:
- The maximum number of bytes that can be transmitted is limited to HELIUM_MAX_DATA_SIZE (about 100 bytes).
- Parameters:
-
data The data bytes to send len The number of bytes in data to send result An out parameter for the result returned by the channel
Definition at line 151 of file Helium.cpp.
int send | ( | void const * | data, |
size_t | len, | ||
uint16_t * | token | ||
) |
Asynchornous send method.
This is the asynchronous version of the send(void const *data, size_t int8_t *) method.
- Parameters:
-
data The data bytes to send len The number of bytes in data to send token The output parameter for the pending result token
Definition at line 144 of file Helium.cpp.
Field Documentation
Generated on Tue Jul 12 2022 12:08:02 by
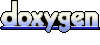