
20211204
Dependencies: mbed
main.cpp
00001 #include "mbed.h" 00002 DigitalOut condition(LED1); 00003 Serial gs(USBTX,USBRX,9600); 00004 Timer sattime; 00005 int rcmd = 0, cmdflag = 0; //command variable 00006 00007 //getting command and command flag 00008 void commandget() 00009 { 00010 rcmd = gs.getc(); 00011 cmdflag = 1; 00012 } 00013 //interrupting process by command receive 00014 void receive(int rcmd, int cmdflag) 00015 { 00016 gs.attach(commandget,Serial::RxIrq); 00017 } 00018 //initializing 00019 void initialize() 00020 { 00021 rcmd = 0; 00022 cmdflag = 0; 00023 condition = 0; 00024 } 00025 00026 int main() 00027 { 00028 gs.printf("From Sat : Nominal Operation\r\n"); 00029 int flag = 0; //condition flag 00030 float batvol, temp; //voltage, temperature 00031 sattime.start(); 00032 receive(rcmd,cmdflag); //interrupting 00033 for(int i=0;i<50;i++){ 00034 //satellite condition led 00035 condition = !condition; 00036 00037 //senssing HK data(dummy data) 00038 batvol = 3.7; 00039 temp = 28.5; 00040 00041 //Transmitting HK data to Ground Station(GS) 00042 gs.printf("HEPTASAT::Condition = %d, Time = %f [s], batvol = %2f [V], temp = %2f [deg C]\r\n",flag,sattime.read(),batvol,temp); 00043 wait_ms(1000); 00044 00045 if(cmdflag == 1){ 00046 if(rcmd == 'a'){ 00047 for(int j=0;j<5;j++){ 00048 gs.printf("Hello World!\r\n"); 00049 condition = 1; 00050 wait_ms(1000); 00051 } 00052 } 00053 initialize(); //initializing 00054 } 00055 } 00056 sattime.stop(); 00057 gs.printf("From Sat : End of operation\r\n"); 00058 }
Generated on Thu Jul 14 2022 10:33:07 by
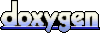