
HeptaSat
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "MPL3115A2.h" 00003 00004 I2C i2c(p28, p27); // sda, scl 00005 00006 // Comment out all of the references to 'pc' on this page if you don't have the 00007 // serial debug driver for your mbed board installed on your computer. If you do, 00008 // I personally like to use Putty as the terminal window to capture debug messages. 00009 Serial pc(USBTX, USBRX); // tx, rx 00010 00011 // Again, remove the '&pc' parameter is you're not debugging. 00012 MPL3115A2 sensor(&i2c, &pc); 00013 00014 DigitalOut myled(LED1); // Sanity check to make sure the program is working. 00015 DigitalOut powerPin(p21); // <-- I powered the sensor from a pin. You don't have to. 00016 00017 int main() { 00018 00019 powerPin = 1; 00020 wait_ms(300); 00021 00022 pc.printf("** MPL3115A2 SENSOR **\r\n"); 00023 sensor.init(); 00024 00025 Altitude a; 00026 Temperature t; 00027 Pressure p; 00028 00029 // Offsets for Dacula, GA 00030 sensor.setOffsetAltitude(83); 00031 sensor.setOffsetTemperature(20); 00032 sensor.setOffsetPressure(-32); 00033 00034 while(1) 00035 { 00036 sensor.readAltitude(&a); 00037 sensor.readTemperature(&t); 00038 00039 sensor.setModeStandby(); 00040 sensor.setModeBarometer(); 00041 sensor.setModeActive(); 00042 sensor.readPressure(&p); 00043 00044 pc.printf("Altitude: %sft, Temp: %sºF, Pressure: %sPa\r\n", a.print(), t.print(), p.print()); 00045 00046 sensor.setModeStandby(); 00047 sensor.setModeAltimeter(); 00048 sensor.setModeActive(); 00049 00050 wait(1.0); 00051 } 00052 }
Generated on Sun Aug 7 2022 04:42:49 by
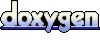