
Reads data from arduino
Dependencies: mbed SDFileSystem FXOS8700Q
main.cpp
00001 #include "mbed.h" 00002 #include <string> 00003 #include "FXOS8700Q.h" 00004 #include "SDFileSystem.h" 00005 /*------------------------------------------------------------------------------ 00006 Before to use this example, ensure that you an hyperterminal installed on your 00007 computer. More info here: https://developer.mbed.org/handbook/Terminals 00008 00009 The default serial comm port uses the SERIAL_TX and SERIAL_RX pins (see their 00010 definition in the PinNames.h file). 00011 00012 The default serial configuration in this case is 9600 bauds, 8-bit data, no parity 00013 00014 If you want to change the baudrate for example, you have to redeclare the 00015 serial object in your code: 00016 00017 Serial pc(SERIAL_TX, SERIAL_RX); 00018 00019 Then, you can modify the baudrate and print like this: 00020 00021 pc.baud(115200); 00022 pc.printf("Hello World !\n"); 00023 ------------------------------------------------------------------------------*/ 00024 00025 DigitalOut led(LED1); 00026 00027 #define UART3_tx PTC17 00028 #define UART3_rx PTC16 00029 // 00030 //#define UART3_tx D8 00031 //#define UART3_rx D2 00032 Serial s_com(UART3_tx, UART3_rx); // tx, rx read gps in 00033 SDFileSystem sd(PTE3, PTE1, PTE2, PTE4, "sd"); // MOSI, MISO, SCK, CS 00034 FILE *fp; 00035 00036 00037 void readData(); 00038 void sensor_data(); 00039 void log_data(); 00040 00041 char rca1[128]; 00042 //string rca2; 00043 string data; 00044 00045 int main() 00046 { 00047 00048 00049 printf("Hello World !\n"); 00050 acc.enable(); //start accelerometer 00051 mag.enable(); 00052 00053 00054 fp = fopen("/sd/sensors.txt", "r"); 00055 if (fp != NULL) { 00056 fclose(fp); 00057 remove("/sd/sensors.txt"); 00058 //pc.printf("Remove an existing file with the same name \n"); 00059 } 00060 00061 while(1) { 00062 while (s_com.readable()) { 00063 // rca = s_com.getc(); 00064 // printf("%c",rca); 00065 readData(); 00066 sensor_data(); 00067 00068 } 00069 } 00070 } 00071 00072 void readData() 00073 { 00074 s_com.scanf("%s",rca1); 00075 string rca2(rca1); 00076 rca2 += "\n"; 00077 log_data(rca2); 00078 printf(rca2.c_str()); 00079 } 00080 00081 void sensor_data() 00082 { 00083 00084 //get mag+accel data 00085 motion_data_units_t acc_data, mag_data; 00086 00087 acc.getAxis(acc_data); 00088 mag.getAxis(mag_data); 00089 pc.printf("\rACC: X=%1.4ff Y=%1.4ff Z=%1.4ff ", acc_data.x, acc_data.y, acc_data.z); 00090 pc.printf(" MAG: X=%4.1ff Y=%4.1ff Z=%4.1ff \r\n", mag_data.x, mag_data.y, mag_data.z); 00091 data = ("ACC: "+ acc_data.x + ", "+ acc_data.y + ", " + acc_data.z + "\n" + 00092 "MAG: " + mag_data.x + ", " + mag_data.y + ", " + mag_data.z + "\n"); 00093 log_data(data); 00094 00095 // wait(0.5); 00096 00097 } 00098 00099 00100 void log_data(string data) 00101 { 00102 00103 // printf("\nWriting data to the sd card \n"); 00104 fp = fopen("/sd/sensors.txt", "w"); 00105 if (fp == NULL) { 00106 //pc.printf("Unable to write the file \n"); 00107 } else { 00108 fprintf(fp, data); 00109 fclose(fp); 00110 //pc.printf("File successfully written! \n"); 00111 } 00112 00113 00114 }
Generated on Wed Jul 20 2022 05:15:51 by
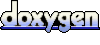