SG RFID nRF51822 fork
Fork of nRF51822 by
Embed:
(wiki syntax)
Show/hide line numbers
ble_hrs_c.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2012 Nordic Semiconductor. All Rights Reserved. 00003 * 00004 * The information contained herein is confidential property of Nordic Semiconductor. The use, 00005 * copying, transfer or disclosure of such information is prohibited except by express written 00006 * agreement with Nordic Semiconductor. 00007 * 00008 */ 00009 00010 /**@file 00011 * 00012 * @defgroup ble_sdk_srv_hrs_c Heart Rate Service Client 00013 * @{ 00014 * @ingroup ble_sdk_srv 00015 * @brief Heart Rate Service Client module. 00016 * 00017 * @details This module contains the APIs and types exposed by the Heart Rate Service Client 00018 * module. These APIs and types can be used by the application to perform discovery of 00019 * Heart Rate Service at the peer and interact with it. 00020 * 00021 * @warning Currently this module only has support for Heart Rate Measurement characteristic. This 00022 * means that it will be able to enable notification of the characteristic at the peer and 00023 * be able to receive Heart Rate Measurement notifications from the peer. It does not 00024 * support the Body Sensor Location and the Heart Rate Control Point characteristics. 00025 * When a Heart Rate Measurement is received, this module will decode only the 00026 * Heart Rate Measurement Value (both 8 bit and 16 bit) field from it and provide it to 00027 * the application. 00028 * 00029 * @note The application must propagate BLE stack events to this module by calling 00030 * ble_hrs_c_on_ble_evt(). 00031 * 00032 */ 00033 00034 #ifndef BLE_HRS_C_H__ 00035 #define BLE_HRS_C_H__ 00036 00037 #include <stdint.h> 00038 #include "ble.h" 00039 00040 /** 00041 * @defgroup hrs_c_enums Enumerations 00042 * @{ 00043 */ 00044 00045 /**@brief HRS Client event type. */ 00046 typedef enum 00047 { 00048 BLE_HRS_C_EVT_DISCOVERY_COMPLETE = 1, /**< Event indicating that the Heart Rate Service has been discovered at the peer. */ 00049 BLE_HRS_C_EVT_HRM_NOTIFICATION /**< Event indicating that a notification of the Heart Rate Measurement characteristic has been received from the peer. */ 00050 } ble_hrs_c_evt_type_t; 00051 00052 /** @} */ 00053 00054 /** 00055 * @defgroup hrs_c_structs Structures 00056 * @{ 00057 */ 00058 00059 /**@brief Structure containing the heart rate measurement received from the peer. */ 00060 typedef struct 00061 { 00062 uint16_t hr_value; /**< Heart Rate Value. */ 00063 } ble_hrm_t; 00064 00065 /**@brief Heart Rate Event structure. */ 00066 typedef struct 00067 { 00068 ble_hrs_c_evt_type_t evt_type; /**< Type of the event. */ 00069 union 00070 { 00071 ble_hrm_t hrm; /**< Heart rate measurement received. This will be filled if the evt_type is @ref BLE_HRS_C_EVT_HRM_NOTIFICATION. */ 00072 } params; 00073 } ble_hrs_c_evt_t; 00074 00075 /** @} */ 00076 00077 /** 00078 * @defgroup hrs_c_types Types 00079 * @{ 00080 */ 00081 00082 // Forward declaration of the ble_bas_t type. 00083 typedef struct ble_hrs_c_s ble_hrs_c_t; 00084 00085 /**@brief Event handler type. 00086 * 00087 * @details This is the type of the event handler that should be provided by the application 00088 * of this module in order to receive events. 00089 */ 00090 typedef void (* ble_hrs_c_evt_handler_t) (ble_hrs_c_t * p_ble_hrs_c, ble_hrs_c_evt_t * p_evt); 00091 00092 /** @} */ 00093 00094 /** 00095 * @addtogroup hrs_c_structs 00096 * @{ 00097 */ 00098 00099 /**@brief Heart Rate Client structure. 00100 */ 00101 typedef struct ble_hrs_c_s 00102 { 00103 uint16_t conn_handle; /**< Connection handle as provided by the SoftDevice. */ 00104 uint16_t hrm_cccd_handle; /**< Handle of the CCCD of the Heart Rate Measurement characteristic. */ 00105 uint16_t hrm_handle; /**< Handle of the Heart Rate Measurement characteristic as provided by the SoftDevice. */ 00106 ble_hrs_c_evt_handler_t evt_handler; /**< Application event handler to be called when there is an event related to the heart rate service. */ 00107 } ble_hrs_c_t; 00108 00109 /**@brief Heart Rate Client initialization structure. 00110 */ 00111 typedef struct 00112 { 00113 ble_hrs_c_evt_handler_t evt_handler; /**< Event handler to be called by the Heart Rate Client module whenever there is an event related to the Heart Rate Service. */ 00114 } ble_hrs_c_init_t; 00115 00116 /** @} */ 00117 00118 /** 00119 * @defgroup hrs_c_functions Functions 00120 * @{ 00121 */ 00122 00123 /**@brief Function for initializing the heart rate client module. 00124 * 00125 * @details This function will register with the DB Discovery module. There it 00126 * registers for the Heart Rate Service. Doing so will make the DB Discovery 00127 * module look for the presence of a Heart Rate Service instance at the peer when a 00128 * discovery is started. 00129 * 00130 * @param[in] p_ble_hrs_c Pointer to the heart rate client structure. 00131 * @param[in] p_ble_hrs_c_init Pointer to the heart rate initialization structure containing the 00132 * initialization information. 00133 * 00134 * @retval NRF_SUCCESS On successful initialization. Otherwise an error code. This function 00135 * propagates the error code returned by the Database Discovery module API 00136 * @ref ble_db_discovery_evt_register. 00137 */ 00138 uint32_t ble_hrs_c_init(ble_hrs_c_t * p_ble_hrs_c, ble_hrs_c_init_t * p_ble_hrs_c_init); 00139 00140 /**@brief Function for handling BLE events from the SoftDevice. 00141 * 00142 * @details This function will handle the BLE events received from the SoftDevice. If a BLE 00143 * event is relevant to the Heart Rate Client module, then it uses it to update 00144 * interval variables and, if necessary, send events to the application. 00145 * 00146 * @param[in] p_ble_hrs_c Pointer to the heart rate client structure. 00147 * @param[in] p_ble_evt Pointer to the BLE event. 00148 */ 00149 void ble_hrs_c_on_ble_evt(ble_hrs_c_t * p_ble_hrs_c, const ble_evt_t * p_ble_evt); 00150 00151 00152 /**@brief Function for requesting the peer to start sending notification of Heart Rate 00153 * Measurement. 00154 * 00155 * @details This function will enable to notification of the Heart Rate Measurement at the peer 00156 * by writing to the CCCD of the Heart Rate Measurement Characteristic. 00157 * 00158 * @param p_ble_hrs_c Pointer to the heart rate client structure. 00159 * 00160 * @retval NRF_SUCCESS If the SoftDevice has been requested to write to the CCCD of the peer. 00161 * Otherwise, an error code. This function propagates the error code returned 00162 * by the SoftDevice API @ref sd_ble_gattc_write. 00163 */ 00164 uint32_t ble_hrs_c_hrm_notif_enable(ble_hrs_c_t * p_ble_hrs_c); 00165 00166 /** @} */ // End tag for Function group. 00167 00168 #endif // BLE_HRS_C_H__ 00169 00170 /** @} */ // End tag for the file.
Generated on Tue Jul 12 2022 15:07:43 by
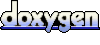