SG RFID nRF51822 fork
Fork of nRF51822 by
Embed:
(wiki syntax)
Show/hide line numbers
ble_hids.h
Go to the documentation of this file.
00001 /* Copyright (c) 2012 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 00013 /** @file 00014 * 00015 * @defgroup ble_sdk_srv_hids Human Interface Device Service 00016 * @{ 00017 * @ingroup ble_sdk_srv 00018 * @brief Human Interface Device Service module. 00019 * 00020 * @details This module implements the Human Interface Device Service with the corresponding set of 00021 * characteristics. During initialization it adds the Human Interface Device Service and 00022 * a set of characteristics as per the Human Interface Device Service specification and 00023 * the user requirements to the BLE stack database. 00024 * 00025 * If enabled, notification of Input Report characteristics is performed when the 00026 * application calls the corresponding ble_hids_xx_input_report_send() function. 00027 * 00028 * If an event handler is supplied by the application, the Human Interface Device Service 00029 * will generate Human Interface Device Service events to the application. 00030 * 00031 * @note The application must propagate BLE stack events to the Human Interface Device Service 00032 * module by calling ble_hids_on_ble_evt() from the @ref ble_stack_handler callback. 00033 * 00034 * @note Attention! 00035 * To maintain compliance with Nordic Semiconductor ASA Bluetooth profile 00036 * qualification listings, this section of source code must not be modified. 00037 */ 00038 00039 #ifndef BLE_HIDS_H__ 00040 #define BLE_HIDS_H__ 00041 00042 #include <stdint.h> 00043 #include <stdbool.h> 00044 #include "ble.h" 00045 #include "ble_srv_common.h " 00046 00047 // Report Type values 00048 #define BLE_HIDS_REP_TYPE_INPUT 1 00049 #define BLE_HIDS_REP_TYPE_OUTPUT 2 00050 #define BLE_HIDS_REP_TYPE_FEATURE 3 00051 00052 // Maximum number of the various Report Types 00053 #define BLE_HIDS_MAX_INPUT_REP 10 00054 #define BLE_HIDS_MAX_OUTPUT_REP 10 00055 #define BLE_HIDS_MAX_FEATURE_REP 10 00056 00057 // Information Flags 00058 #define HID_INFO_FLAG_REMOTE_WAKE_MSK 0x01 00059 #define HID_INFO_FLAG_NORMALLY_CONNECTABLE_MSK 0x02 00060 00061 /**@brief HID Service characteristic id. */ 00062 typedef struct 00063 { 00064 uint16_t uuid; /**< UUID of characteristic. */ 00065 uint8_t rep_type; /**< Type of report (only used for BLE_UUID_REPORT_CHAR, see @ref BLE_HIDS_REPORT_TYPE). */ 00066 uint8_t rep_index; /**< Index of the characteristic (only used for BLE_UUID_REPORT_CHAR). */ 00067 } ble_hids_char_id_t; 00068 00069 /**@brief HID Service event type. */ 00070 typedef enum 00071 { 00072 BLE_HIDS_EVT_HOST_SUSP, /**< Suspend command received. */ 00073 BLE_HIDS_EVT_HOST_EXIT_SUSP, /**< Exit suspend command received. */ 00074 BLE_HIDS_EVT_NOTIF_ENABLED, /**< Notification enabled event. */ 00075 BLE_HIDS_EVT_NOTIF_DISABLED, /**< Notification disabled event. */ 00076 BLE_HIDS_EVT_REP_CHAR_WRITE, /**< A new value has been written to an Report characteristic. */ 00077 BLE_HIDS_EVT_BOOT_MODE_ENTERED, /**< Boot mode entered. */ 00078 BLE_HIDS_EVT_REPORT_MODE_ENTERED, /**< Report mode entered. */ 00079 BLE_HIDS_EVT_REPORT_READ /**< Read with response */ 00080 } ble_hids_evt_type_t; 00081 00082 /**@brief HID Service event. */ 00083 typedef struct 00084 { 00085 ble_hids_evt_type_t evt_type; /**< Type of event. */ 00086 union 00087 { 00088 struct 00089 { 00090 ble_hids_char_id_t char_id; /**< Id of characteristic for which notification has been started. */ 00091 } notification; 00092 struct 00093 { 00094 ble_hids_char_id_t char_id; /**< Id of characteristic having been written. */ 00095 uint16_t offset; /**< Offset for the write operation. */ 00096 uint16_t len; /**< Length of the incoming data. */ 00097 uint8_t* data; /**< Incoming data, variable length */ 00098 } char_write; 00099 struct 00100 { 00101 ble_hids_char_id_t char_id; /**< Id of characteristic being read. */ 00102 } char_auth_read; 00103 } params; 00104 ble_evt_t * p_ble_evt; /**< corresponding received ble event, NULL if not relevant */ 00105 } ble_hids_evt_t; 00106 00107 // Forward declaration of the ble_hids_t type. 00108 typedef struct ble_hids_s ble_hids_t; 00109 00110 /**@brief HID Service event handler type. */ 00111 typedef void (*ble_hids_evt_handler_t) (ble_hids_t * p_hids, ble_hids_evt_t * p_evt); 00112 00113 /**@brief HID Information characteristic value. */ 00114 typedef struct 00115 { 00116 uint16_t bcd_hid; /**< 16-bit unsigned integer representing version number of base USB HID Specification implemented by HID Device */ 00117 uint8_t b_country_code; /**< Identifies which country the hardware is localized for. Most hardware is not localized and thus this value would be zero (0). */ 00118 uint8_t flags; /**< See http://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.hid_information.xml */ 00119 ble_srv_security_mode_t security_mode; /**< Security mode for the HID Information characteristic. */ 00120 } ble_hids_hid_information_t; 00121 00122 /**@brief HID Service Input Report characteristic init structure. This contains all options and 00123 * data needed for initialization of one Input Report characteristic. */ 00124 typedef struct 00125 { 00126 uint16_t max_len; /**< Maximum length of characteristic value. */ 00127 ble_srv_report_ref_t rep_ref; /**< Value of the Report Reference descriptor. */ 00128 ble_srv_cccd_security_mode_t security_mode; /**< Security mode for the HID Input Report characteristic, including cccd. */ 00129 uint8_t read_resp : 1; /**< Should application generate a response to read requests. */ 00130 } ble_hids_inp_rep_init_t; 00131 00132 /**@brief HID Service Output Report characteristic init structure. This contains all options and 00133 * data needed for initialization of one Output Report characteristic. */ 00134 typedef struct 00135 { 00136 uint16_t max_len; /**< Maximum length of characteristic value. */ 00137 ble_srv_report_ref_t rep_ref; /**< Value of the Report Reference descriptor. */ 00138 ble_srv_cccd_security_mode_t security_mode; /**< Security mode for the HID Output Report characteristic, including cccd. */ 00139 uint8_t read_resp : 1; /**< Should application generate a response to read requests. */ 00140 } ble_hids_outp_rep_init_t; 00141 00142 /**@brief HID Service Feature Report characteristic init structure. This contains all options and 00143 * data needed for initialization of one Feature Report characteristic. */ 00144 typedef struct 00145 { 00146 uint16_t max_len; /**< Maximum length of characteristic value. */ 00147 ble_srv_report_ref_t rep_ref; /**< Value of the Report Reference descriptor. */ 00148 ble_srv_cccd_security_mode_t security_mode; /**< Security mode for the HID Service Feature Report characteristic, including cccd. */ 00149 uint8_t read_resp : 1; /**< Should application generate a response to read requests. */ 00150 } ble_hids_feature_rep_init_t; 00151 00152 /**@brief HID Service Report Map characteristic init structure. This contains all options and data 00153 * needed for initialization of the Report Map characteristic. */ 00154 typedef struct 00155 { 00156 uint8_t * p_data; /**< Report map data. */ 00157 uint16_t data_len; /**< Length of report map data. */ 00158 uint8_t ext_rep_ref_num; /**< Number of Optional External Report Reference descriptors. */ 00159 ble_uuid_t * p_ext_rep_ref; /**< Optional External Report Reference descriptor (will be added if != NULL). */ 00160 ble_srv_security_mode_t security_mode; /**< Security mode for the HID Service Report Map characteristic. */ 00161 } ble_hids_rep_map_init_t; 00162 00163 /**@brief HID Report characteristic structure. */ 00164 typedef struct 00165 { 00166 ble_gatts_char_handles_t char_handles; /**< Handles related to the Report characteristic. */ 00167 uint16_t ref_handle; /**< Handle of the Report Reference descriptor. */ 00168 } ble_hids_rep_char_t; 00169 00170 /**@brief HID Service init structure. This contains all options and data needed for initialization 00171 * of the service. */ 00172 typedef struct 00173 { 00174 ble_hids_evt_handler_t evt_handler; /**< Event handler to be called for handling events in the HID Service. */ 00175 ble_srv_error_handler_t error_handler; /**< Function to be called in case of an error. */ 00176 bool is_kb; /**< TRUE if device is operating as a keyboard, FALSE if it is not. */ 00177 bool is_mouse; /**< TRUE if device is operating as a mouse, FALSE if it is not. */ 00178 uint8_t inp_rep_count; /**< Number of Input Report characteristics. */ 00179 ble_hids_inp_rep_init_t * p_inp_rep_array; /**< Information about the Input Report characteristics. */ 00180 uint8_t outp_rep_count; /**< Number of Output Report characteristics. */ 00181 ble_hids_outp_rep_init_t * p_outp_rep_array; /**< Information about the Output Report characteristics. */ 00182 uint8_t feature_rep_count; /**< Number of Feature Report characteristics. */ 00183 ble_hids_feature_rep_init_t * p_feature_rep_array; /**< Information about the Feature Report characteristics. */ 00184 ble_hids_rep_map_init_t rep_map; /**< Information nedeed for initialization of the Report Map characteristic. */ 00185 ble_hids_hid_information_t hid_information; /**< Value of the HID Information characteristic. */ 00186 uint8_t included_services_count; /**< Number of services to include in HID service. */ 00187 uint16_t * p_included_services_array; /**< Array of services to include in HID service. */ 00188 ble_srv_security_mode_t security_mode_protocol; /**< Security settings for HID service protocol attribute */ 00189 ble_srv_security_mode_t security_mode_ctrl_point; /**< Security settings for HID service Control Point attribute */ 00190 ble_srv_cccd_security_mode_t security_mode_boot_mouse_inp_rep; /**< Security settings for HID service Mouse input report attribute */ 00191 ble_srv_cccd_security_mode_t security_mode_boot_kb_inp_rep; /**< Security settings for HID service Keyboard input report attribute */ 00192 ble_srv_security_mode_t security_mode_boot_kb_outp_rep; /**< Security settings for HID service Keyboard output report attribute */ 00193 } ble_hids_init_t; 00194 00195 /**@brief HID Service structure. This contains various status information for the service. */ 00196 typedef struct ble_hids_s 00197 { 00198 ble_hids_evt_handler_t evt_handler; /**< Event handler to be called for handling events in the HID Service. */ 00199 ble_srv_error_handler_t error_handler; /**< Function to be called in case of an error. */ 00200 uint16_t service_handle; /**< Handle of HID Service (as provided by the BLE stack). */ 00201 ble_gatts_char_handles_t protocol_mode_handles; /**< Handles related to the Protocol Mode characteristic (will only be created if ble_hids_init_t.is_kb or ble_hids_init_t.is_mouse is set). */ 00202 uint8_t inp_rep_count; /**< Number of Input Report characteristics. */ 00203 ble_hids_rep_char_t inp_rep_array[BLE_HIDS_MAX_INPUT_REP]; /**< Information about the Input Report characteristics. */ 00204 uint8_t outp_rep_count; /**< Number of Output Report characteristics. */ 00205 ble_hids_rep_char_t outp_rep_array[BLE_HIDS_MAX_OUTPUT_REP]; /**< Information about the Output Report characteristics. */ 00206 uint8_t feature_rep_count; /**< Number of Feature Report characteristics. */ 00207 ble_hids_rep_char_t feature_rep_array[BLE_HIDS_MAX_FEATURE_REP]; /**< Information about the Feature Report characteristics. */ 00208 ble_gatts_char_handles_t rep_map_handles; /**< Handles related to the Report Map characteristic. */ 00209 uint16_t rep_map_ext_rep_ref_handle; /**< Handle of the Report Map External Report Reference descriptor. */ 00210 ble_gatts_char_handles_t boot_kb_inp_rep_handles; /**< Handles related to the Boot Keyboard Input Report characteristic (will only be created if ble_hids_init_t.is_kb is set). */ 00211 ble_gatts_char_handles_t boot_kb_outp_rep_handles; /**< Handles related to the Boot Keyboard Output Report characteristic (will only be created if ble_hids_init_t.is_kb is set). */ 00212 ble_gatts_char_handles_t boot_mouse_inp_rep_handles; /**< Handles related to the Boot Mouse Input Report characteristic (will only be created if ble_hids_init_t.is_mouse is set). */ 00213 ble_gatts_char_handles_t hid_information_handles; /**< Handles related to the Report Map characteristic. */ 00214 ble_gatts_char_handles_t hid_control_point_handles; /**< Handles related to the Report Map characteristic. */ 00215 uint16_t conn_handle; /**< Handle of the current connection (as provided by the BLE stack, is BLE_CONN_HANDLE_INVALID if not in a connection). */ 00216 } ble_hids_t; 00217 00218 /**@brief Function for initializing the HID Service. 00219 * 00220 * @param[out] p_hids HID Service structure. This structure will have to be supplied by the 00221 * application. It will be initialized by this function, and will later be 00222 * used to identify this particular service instance. 00223 * @param[in] p_hids_init Information needed to initialize the service. 00224 * 00225 * @return NRF_SUCCESS on successful initialization of service, otherwise an error code. 00226 */ 00227 uint32_t ble_hids_init(ble_hids_t * p_hids, const ble_hids_init_t * p_hids_init); 00228 00229 /**@brief Function for handling the Application's BLE Stack events. 00230 * 00231 * @details Handles all events from the BLE stack of interest to the HID Service. 00232 * 00233 * @param[in] p_hids HID Service structure. 00234 * @param[in] p_ble_evt Event received from the BLE stack. 00235 */ 00236 void ble_hids_on_ble_evt(ble_hids_t * p_hids, ble_evt_t * p_ble_evt); 00237 00238 /**@brief Function for sending Input Report. 00239 * 00240 * @details Sends data on an Input Report characteristic. 00241 * 00242 * @param[in] p_hids HID Service structure. 00243 * @param[in] rep_index Index of the characteristic (corresponding to the index in 00244 * ble_hids_t.inp_rep_array as passed to ble_hids_init()). 00245 * @param[in] len Length of data to be sent. 00246 * @param[in] p_data Pointer to data to be sent. 00247 * 00248 * @return NRF_SUCCESS on successful sending of input report, otherwise an error code. 00249 */ 00250 uint32_t ble_hids_inp_rep_send(ble_hids_t * p_hids, 00251 uint8_t rep_index, 00252 uint16_t len, 00253 uint8_t * p_data); 00254 00255 /**@brief Function for sending Boot Keyboard Input Report. 00256 * 00257 * @details Sends data on an Boot Keyboard Input Report characteristic. 00258 * 00259 * @param[in] p_hids HID Service structure. 00260 * @param[in] len Length of data to be sent. 00261 * @param[in] p_data Pointer to data to be sent. 00262 * 00263 * @return NRF_SUCCESS on successful sending of the report, otherwise an error code. 00264 */ 00265 uint32_t ble_hids_boot_kb_inp_rep_send(ble_hids_t * p_hids, 00266 uint16_t len, 00267 uint8_t * p_data); 00268 00269 /**@brief Function for sending Boot Mouse Input Report. 00270 * 00271 * @details Sends data on an Boot Mouse Input Report characteristic. 00272 * 00273 * @param[in] p_hids HID Service structure. 00274 * @param[in] buttons State of mouse buttons. 00275 * @param[in] x_delta Horizontal movement. 00276 * @param[in] y_delta Vertical movement. 00277 * @param[in] optional_data_len Length of optional part of Boot Mouse Input Report. 00278 * @param[in] p_optional_data Optional part of Boot Mouse Input Report. 00279 * 00280 * @return NRF_SUCCESS on successful sending of the report, otherwise an error code. 00281 */ 00282 uint32_t ble_hids_boot_mouse_inp_rep_send(ble_hids_t * p_hids, 00283 uint8_t buttons, 00284 int8_t x_delta, 00285 int8_t y_delta, 00286 uint16_t optional_data_len, 00287 uint8_t * p_optional_data); 00288 00289 /**@brief Function for getting the current value of Output Report from the stack. 00290 * 00291 * @details Fetches the current value of the output report characteristic from the stack. 00292 * 00293 * @param[in] p_hids HID Service structure. 00294 * @param[in] rep_index Index of the characteristic (corresponding to the index in 00295 * ble_hids_t.outp_rep_array as passed to ble_hids_init()). 00296 * @param[in] len Length of output report needed. 00297 * @param[in] offset Offset in bytes to read from. 00298 * @param[out] p_outp_rep Pointer to the output report. 00299 * 00300 * @return NRF_SUCCESS on successful read of the report, otherwise an error code. 00301 */ 00302 uint32_t ble_hids_outp_rep_get(ble_hids_t * p_hids, 00303 uint8_t rep_index, 00304 uint16_t len, 00305 uint8_t offset, 00306 uint8_t * p_outp_rep); 00307 00308 #endif // BLE_HIDS_H__ 00309 00310 /** @} */
Generated on Tue Jul 12 2022 15:07:43 by
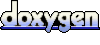