interrupt handling
Embed:
(wiki syntax)
Show/hide line numbers
readerComm.cpp
00001 /* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00002 Filename: readerComm.cpp 00003 Description: Functions used to communicate with the TRF7970 eval bd. 00004 Communication is by means of an SPI interface between 00005 the nRF51-DK board (nRF51422 MCU) and the TRF7970 eval bd. 00006 Copyright (C) 2015 Gymtrack, Inc. 00007 Author: Ron Clough 00008 Date: 2015-02-27 00009 00010 Changes: 00011 Rev Date Who Details 00012 ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ 00013 0.0 2015-02-27 RWC Original version. 00014 00015 * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */ 00016 /*========================================================================== 00017 Initialize the chipset ISO15693 and read UID: 00018 1) Reset 00019 [0x83] 00020 2) Write Modulator and SYS_CLK Control Register (0x09) (13.56Mhz SYS_CLK and default Clock 13.56Mhz)) 00021 [0x09 0x31] 00022 3) Configure Mode ISO Control Register (0x01) to 0x02 (ISO15693 high bit rate, one subcarrier, 1 out of 4) 00023 [0x01 0x02] 00024 4) Turn RF ON (Chip Status Control Register (0x00)) 00025 [0x40 r] [0x00 0x20] [0x40 r] 00026 5) Inventory Command (see Figure 5-20. Inventory Command Sent From MCU to TRF7970A) 00027 5-1) Send Inventory(8B), Wait 2ms, Read/Clear IRQ Status(0x0C=>0x6C)+dummy read, 00028 Read FIFO Status Register(0x1C/0x5C), Read Continuous FIFO from 0x1F to 0x1F+0x0A(0x1F/0x7F), 00029 Read/Clear IRQ Status(0x0C=>0x6C)+dummy read, Read FIFO Status Register(0x1C/0x5C), 00030 Reset FIFO(0x0F/0x8F), Read RSSI levels and oscillator status(0x0F/0x4F) 00031 [0x8F 0x91 0x3D 0x00 0x30 0x26 0x01 0x00] %:2 [0x6C r:2] [0x5C r] [0x7F r:10] %:10 [0x6C r:2] [0x5C r] [0x8F] [0x4F r] 00032 ==============================================================================*/ 00033 00034 00035 00036 00037 00038 00039 #include "mbed.h" 00040 #include "readerComm.h" 00041 uint8_t sg=0; 00042 DigitalOut EN(p0); // Control EN pin on TRF7970 00043 DigitalOut EN2(p4); // Control EN2 pin on TRF7970 00044 DigitalOut CS(p9); 00045 uint8_t turnRFOn[2]; 00046 uint8_t testcommand[2]; 00047 extern uint8_t noBytes; 00048 extern SPI spi; // main.cpp 00049 extern Serial pc; // main.cpp 00050 uint8_t buf[300]; // main.cpp 00051 uint8_t found=0; 00052 uint8_t rssi_flag=0; 00053 uint8_t rssi=0; 00054 extern DigitalOut BLED; 00055 DigitalOut AO_7970(p29); 00056 DigitalOut MOD_7970(p30); 00057 int nfc=0; 00058 //extern bool tagFound=0; 00059 00060 //extern DigitalOut debug1LED; 00061 //extern DigitalOut debug2LED; 00062 //extern DigitalOut ISO15693LED; 00063 //extern DigitalOut heartbeatLED; 00064 //extern DigitalOut testPin; 00065 uint8_t temp; 00066 uint8_t command[2]; 00067 00068 uint8_t WAIT=0; 00069 void trf797xDirectCommand(uint8_t *buffer) 00070 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00071 // trf797xDirectCommand() 00072 // Description: Transmit a Direct Command to the reader chip. 00073 // Parameter: *buffer = the direct command. 00074 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00075 { 00076 *buffer = (0x80 | *buffer); // Setup command mode 00077 *buffer = (0x9F & *buffer); // Setup command mode 00078 CS = SELECT; 00079 spi.write(*buffer); 00080 CS = DESELECT; 00081 } // End of trf797xDirectCommand() 00082 00083 void trf797xWriteSingle(uint8_t *buffer, uint8_t length) 00084 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00085 // trf797xWriteSingle() 00086 // Description: Writes to specified reader registers. 00087 // Parameters: *buffer = addresses of the registers followed by the 00088 // contents to write. 00089 // length = number of registers * 2. 00090 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00091 { 00092 uint8_t i=0; 00093 00094 CS = SELECT; 00095 while(length > 0) { 00096 *buffer = (0x1F & *buffer); // Register address 00097 for(i = 0; i < 2; i++) { 00098 spi.write(*buffer); 00099 buffer++; 00100 length--; 00101 } 00102 } 00103 CS = DESELECT; 00104 00105 } // End of trf797xWriteSingle() 00106 00107 void trf797xReadSingle(uint8_t *buffer, uint8_t number) 00108 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00109 // trf797xReadSingle() 00110 // Description: Reads specified reader chip registers and 00111 // writes register contents to *buffer. 00112 // Parameters: *buffer = addresses of the registers. 00113 // number = number of registers. 00114 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00115 { 00116 CS = SELECT; 00117 while(number > 0) { 00118 *buffer = (0x40 | *buffer); // Address, read, single 00119 *buffer = (0x5F & *buffer); // Register address 00120 spi.write(*buffer); 00121 *buffer = spi.write(0x00); // *buffer <- register contents 00122 buffer++; 00123 number--; 00124 } 00125 CS = DESELECT; 00126 } // End of trf797xReadSingle() 00127 00128 void trf797xReadContinuous(uint8_t *buffer, uint8_t length) 00129 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00130 // trf797xReadContinuous() 00131 // Description: Used in SPI mode to read a specified number of 00132 // reader chip registers from a specified address upwards. 00133 // Contents of the registers are stored in *buffer. 00134 // 1) Read register(s) 00135 // 2) Write contents to *buffer 00136 // Parameters: *buffer = address of first register. 00137 // length = number of registers to read. 00138 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00139 { 00140 //==================tested wrk $sg 00141 CS = SELECT; 00142 *buffer = (0x60 | *buffer); // Address, read, continuous 00143 *buffer = (0x7F & *buffer); // Register address 00144 spi.write(*buffer); 00145 while(length > 0) { 00146 *buffer = spi.write(0x00); 00147 buffer++; 00148 length--; 00149 } 00150 // spi.write(0x00); spi.write(0x00); // 16 clock cycles, see TRF7970A FW Design Hints SLOA159 section 7.3 00151 CS = DESELECT; 00152 00153 //=====================tested it wrks $sg 00154 } // End of trf797xReadContinuous() 00155 00156 void trf797xRawWrite(uint8_t *buffer, uint8_t length) 00157 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00158 // trf797xRawWrite() 00159 // Description: Used in SPI mode to write direct to the reader chip. 00160 // Parameters: *buffer = raw data 00161 // length = number of data bytes 00162 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00163 { 00164 CS = SELECT; 00165 while(length > 0) { 00166 temp = spi.write(*buffer); 00167 buffer++; 00168 length--; 00169 } 00170 CS = DESELECT; 00171 } // End of trf797xRawWrite() 00172 00173 void trf797xStopDecoders(void) 00174 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00175 // trf797xStopDecoders() 00176 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00177 { 00178 command[0] = STOP_DECODERS; 00179 trf797xDirectCommand(command); 00180 } 00181 00182 void trf797xRunDecoders(void) 00183 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00184 // trf797xRunDecoders() 00185 // * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * 00186 { 00187 command[0] = RUN_DECODERS; 00188 trf797xDirectCommand(command); 00189 } 00190 00191 00192 void PowerUpNFC2(void) 00193 { 00194 //CS = 1; 00195 // wait_ms(4); 00196 // EN = 1; 00197 // EN2=1; 00198 00199 CS = 0; 00200 EN2 = 0; 00201 EN = 0; 00202 wait_ms(2); 00203 CS = 1; 00204 wait_ms(3); 00205 EN2 = 1; 00206 wait_ms(1); 00207 EN = 1; 00208 } 00209 void PowerUpNFC(void) 00210 { 00211 //CS = 1; 00212 // wait_ms(4); 00213 // EN = 1; 00214 EN2 = 1; 00215 wait_ms(1); 00216 EN=1; 00217 } 00218 void PowerDownNFC(void) 00219 { 00220 ///CS=1; 00221 EN=0; 00222 EN2=0; 00223 //wait_ms(1); 00224 //EN2= 0; PowerDown Mode 00225 //EN2=1; SleepMode 00226 //CS=0; 00227 } 00228 void SleepNFC(void) 00229 { 00230 EN=0; 00231 EN2=0; 00232 } 00233 void StandByNFC(void) 00234 { 00235 turnRFOn[0] = CHIP_STATUS_CONTROL; 00236 turnRFOn[1] = CHIP_STATUS_CONTROL; 00237 00238 turnRFOn[1] &= 0x3F; 00239 turnRFOn[1] |= 0x80; 00240 trf797xWriteSingle(turnRFOn, 2); 00241 } 00242 00243 void SpiInit1(void) 00244 { 00245 spi.format(8, 1); // 8 bit data, mode = 1 (transition on rising edge, sample on falling edge) 00246 spi.frequency(250000); 00247 } 00248 00249 void SpiInit(void) 00250 { 00251 spi.format(8, 1); // 8 bit data, mode = 1 (transition on rising edge, sample on falling edge) 00252 spi.frequency(1000000); 00253 } 00254 00255 void NFCInit(void) 00256 { 00257 //testPin=1; 00258 //wait_ms(2); 00259 00260 testcommand[0] = SOFT_INIT; 00261 trf797xDirectCommand(testcommand); 00262 wait_ms(2); 00263 testcommand[0] = IDLE; 00264 trf797xDirectCommand(testcommand); 00265 wait_ms(2); 00266 //testcommand[0] =NFC_TARGET_LEVEL ; 00267 //testcommand[1] = 0x00; 00268 //trf797xWriteSingle(testcommand, 2); 00269 testcommand[0] = MODULATOR_CONTROL; 00270 testcommand[1] = 0x81; // 6.78 MHz, OOK 100% 00271 trf797xWriteSingle(testcommand, 2); 00272 //wait_ms(2); 00273 testcommand[0] = MODULATOR_CONTROL; 00274 trf797xReadSingle(testcommand, 1); 00275 testcommand[0] = REGULATOR_CONTROL ; 00276 testcommand[1] = 0x07; 00277 trf797xWriteSingle(testcommand, 2); 00278 turnRFOn[0] = CHIP_STATUS_CONTROL; 00279 turnRFOn[1] = CHIP_STATUS_CONTROL; 00280 turnRFOn[1] &= 0x3F; 00281 turnRFOn[1] |= 0x00; 00282 00283 // Oroiginal code has 0x20 !!! 00284 trf797xReadSingle(turnRFOn, 1); 00285 turnRFOn[0] = CHIP_STATUS_CONTROL; 00286 turnRFOn[1] = CHIP_STATUS_CONTROL; 00287 turnRFOn[1] &= 0x3F; 00288 turnRFOn[1] |= 0x00; 00289 trf797xWriteSingle(turnRFOn, 2); 00290 //wait_ms(2); 00291 00292 trf797xWriteSingle(testcommand, 2); 00293 testcommand[0] = ISO_CONTROL; 00294 testcommand[1] = 0x02; // 6.78 MHz, OOK 100% 00295 trf797xWriteSingle(testcommand, 2); 00296 testcommand[0] = IRQ_MASK; 00297 testcommand[1] = 0x3F; 00298 trf797xWriteSingle(testcommand, 2); 00299 //wait_ms(6); 00300 //testPin=0; 00301 } 00302 00303 void RegisterReInitNFC(void) 00304 { 00305 //testcommand[0] =NFC_TARGET_LEVEL ; 00306 //testcommand[1] = 0x00; 00307 //trf797xWriteSingle(testcommand, 2); 00308 testcommand[0] = TX_TIMER_EPC_HIGH; 00309 testcommand[1] = 0xC1; 00310 trf797xWriteSingle(testcommand, 2); 00311 testcommand[0] = TX_TIMER_EPC_LOW ; 00312 testcommand[1] = 0xC1; 00313 trf797xWriteSingle(testcommand, 2); 00314 testcommand[0] = TX_PULSE_LENGTH_CONTROL ; 00315 testcommand[1] = 0x00; 00316 trf797xWriteSingle(testcommand, 2); 00317 testcommand[0] = RX_NO_RESPONSE_WAIT_TIME ; 00318 testcommand[1] = 0x30; 00319 trf797xWriteSingle(testcommand, 2); 00320 testcommand[0] = RX_WAIT_TIME ; 00321 testcommand[1] = 0x1F; 00322 trf797xWriteSingle(testcommand, 2); 00323 testcommand[0] = MODULATOR_CONTROL ; 00324 testcommand[1] = 0x81; //0x34 100%ook@13MHz 00325 trf797xWriteSingle(testcommand, 2); 00326 testcommand[0] = RX_SPECIAL_SETTINGS ; 00327 testcommand[1] = 0x40; 00328 trf797xWriteSingle(testcommand, 2); 00329 testcommand[0] = REGULATOR_CONTROL ; 00330 testcommand[1] = 0x07; 00331 trf797xWriteSingle(testcommand, 2); 00332 } 00333 00334 00335 void RegistersReadNFC(void) 00336 { 00337 turnRFOn[0] = CHIP_STATUS_CONTROL; 00338 trf797xReadSingle(turnRFOn, 1); 00339 testcommand[0] = ISO_CONTROL; 00340 trf797xReadSingle(testcommand, 1); 00341 testcommand[0] = TX_TIMER_EPC_HIGH; //0xC1; 00342 trf797xReadSingle(testcommand, 1); 00343 testcommand[0] = TX_TIMER_EPC_LOW ; //0xC1; 00344 trf797xReadSingle(testcommand, 1); 00345 testcommand[0] = TX_PULSE_LENGTH_CONTROL ; //0x00; 00346 trf797xReadSingle(testcommand, 1); 00347 testcommand[0] = RX_NO_RESPONSE_WAIT_TIME ; //0x30; 00348 trf797xReadSingle(testcommand, 1); 00349 testcommand[0] = RX_WAIT_TIME ; //0x1F; 00350 trf797xReadSingle(testcommand, 1); 00351 testcommand[0] = MODULATOR_CONTROL ; //0x21; 00352 trf797xReadSingle(testcommand, 1); 00353 testcommand[0] = RX_SPECIAL_SETTINGS ; //0x40; 00354 trf797xReadSingle(testcommand, 1); 00355 testcommand[0] = REGULATOR_CONTROL ; //0x87; 00356 trf797xReadSingle(testcommand, 1); 00357 } 00358 00359 void InventoryReqNFC(void) 00360 { 00361 //send inventory command================================================== 00362 buf[0]=0x8F; //Send Inventory(8B)[0x8F 0x91 0x3D 0x00 0x30 0x26 0x01 0x00] 00363 buf[1]=0x91; 00364 buf[2]=0x3D; 00365 buf[3]=0x00; 00366 buf[4]=0x30; 00367 buf[5]=0x26; 00368 buf[6]=0x01; 00369 buf[7]=0x00; 00370 trf797xRawWrite(&buf[0],8); 00371 //read rssi register and interchange rx input to main and aux receiver blocks 00372 //{add code block here 00373 // } 00374 wait_ms(2); 00375 testcommand[0] = IRQ_STATUS; 00376 trf797xReadSingle(testcommand,1); 00377 testcommand[0] = IRQ_STATUS; 00378 trf797xReadSingle(testcommand,1); 00379 wait_ms(5); 00380 //testcommand[0] = CHECK_EXTERNAL_RF; 00381 //trf797xDirectCommand(testcommand); 00382 //wait_ms(1); 00383 testcommand[0] = RSSI_LEVELS; //Read RSSI levels and oscillator status(0x0F/0x4F) 00384 trf797xReadSingle(testcommand, 1); 00385 printf("RSSI:%X \r\n", testcommand[0]); 00386 /*====================================read tag ID 00387 testcommand[0] = IRQ_STATUS; 00388 trf797xReadSingle(testcommand,1); 00389 testcommand[0] = IRQ_STATUS; 00390 trf797xReadSingle(testcommand,1); 00391 testcommand[0] = FIFO_COUNTER; //Read FIFO Status Register(0x1C/0x5C) 00392 trf797xReadSingle(testcommand, 1); 00393 testcommand[0] = 0x7F & testcommand[0]; // Determine the number of bytes left in FIFO 00394 buf[0] = FIFO; 00395 trf797xReadContinuous(&buf[0], testcommand[0]); 00396 testcommand[0] = RSSI_LEVELS; //Read RSSI levels and oscillator status(0x0F/0x4F) 00397 trf797xReadSingle(testcommand, 1); 00398 testcommand[0] = IRQ_STATUS; 00399 trf797xReadSingle(testcommand,1); 00400 testcommand[0] = IRQ_STATUS; 00401 trf797xReadSingle(testcommand,1); 00402 testcommand[0] = RESET; //Reset FIFO(0x0F/0x8F) 00403 trf797xDirectCommand(testcommand); 00404 trf797xStopDecoders(); 00405 trf797xRunDecoders(); */ 00406 //=====================================read Tag ID 00407 //wait(1); 00408 } 00409 00410 void FindNFC(uint8_t *irqStatus) 00411 { 00412 //found=0; 00413 //static uint8_t sg; 00414 //printf("%X \r\n", *irqStatus); 00415 00416 switch(*irqStatus) { 00417 case BIT0: 00418 found=BIT0; 00419 WAIT=10; 00420 break; 00421 case BIT1: 00422 found=BIT1; 00423 break; 00424 // case BIT2://found=BIT2; // break; 00425 //case BIT3:found=BIT3; WAIT=0;break; 00426 // case BIT4:found=BIT4; WAIT=0;break; 00427 // case BIT5:found=BIT5; WAIT=0;break; 00428 case 0x40: 00429 found=0x40; 00430 break; 00431 case BIT7: 00432 found=BIT7; 00433 break; 00434 default: 00435 found=0; 00436 } 00437 00438 00439 } 00440 void handlerNFC(void) 00441 { 00442 testcommand[0] = IRQ_STATUS; 00443 trf797xReadSingle(testcommand,1); 00444 FindNFC(testcommand); 00445 //wait_ms(1); 00446 //=============================use if trf7970a irq_status is not cleared 00447 //testcommand[0] = IRQ_STATUS; 00448 //trf797xReadSingle(testcommand,1); 00449 //============================= 00450 } 00451 00452 00453 void MemReadReqNFC(void) 00454 { 00455 testcommand[0] = RX_NO_RESPONSE_WAIT_TIME ; 00456 testcommand[1] = 0xFF; 00457 trf797xWriteSingle(testcommand, 2); 00458 //send inventory command================================================== 00459 buf[0]=0x8F; //Send Inventory(8B)[0x8F 0x91 0x3D 0x00 0x30 0x26 0x01 0x00] 00460 buf[1]=0x91; 00461 buf[2]=0x3D; 00462 buf[3]=0x00; 00463 buf[4]=0x30; 00464 buf[5]=0x02; 00465 buf[6]=0x20; 00466 buf[7]=0x00; 00467 trf797xRawWrite(&buf[0],8); 00468 00469 //read rssi register and interchange rx input to main and aux receiver blocks 00470 //{add code block here 00471 // } 00472 //wait_ms(2); 00473 //testPin=1; 00474 //=========================use to clear irq_status register of trf7970a 00475 //testcommand[0] = IRQ_STATUS; 00476 //trf797xReadSingle(testcommand,1); 00477 //testcommand[0] = IRQ_STATUS; 00478 //trf797xReadSingle(testcommand,1); 00479 //========================== 00480 wait_ms(5); 00481 //testcommand[0] = CHECK_EXTERNAL_RF; 00482 //trf797xDirectCommand(testcommand); 00483 //wait_us(100); 00484 testcommand[0] = RSSI_LEVELS; 00485 //Read RSSI levels and oscillator status(0x0F/0x4F) 00486 trf797xReadSingle(testcommand, 1); 00487 rssi=testcommand[0]; 00488 00489 printf("RSSI:%X \r\n", testcommand[0]); 00490 } 00491 void ReadNFC(void) 00492 { 00493 /*========================================== read irqstatus reg. of trf7970a 00494 //testcommand[0] = IRQ_STATUS; clear irqstatus reg of trf7970a 00495 //trf797xReadSingle(testcommand,1); 00496 //testcommand[0] = IRQ_STATUS; 00497 //trf797xReadSingle(testcommand,1); 00498 ===========================================*/ 00499 00500 noBytes = FIFO_COUNTER; //Read FIFO Status Register(0x1C/0x5C) 00501 trf797xReadSingle(&noBytes, 1); 00502 noBytes = 0x7F & noBytes; // Determine the number of bytes left in FIFO 00503 buf[0] = FIFO; 00504 trf797xReadContinuous(&buf[0],noBytes); 00505 //==use if trf7970a irq_status is not cleared 00506 //testcommand[0] = IRQ_STATUS; 00507 //trf797xReadSingle(testcommand,1); 00508 //testcommand[0] = IRQ_STATUS; 00509 //trf797xReadSingle(testcommand,1); 00510 //========================================= 00511 testcommand[0] = RESET; //Reset FIFO(0x0F/0x8F) 00512 trf797xDirectCommand(testcommand); 00513 00514 //wait(1); 00515 } 00516 00517 00518 00519 00520 00521 00522 00523 00524 00525 bool PollNFC(void) 00526 { 00527 printf("PollNFC \r\n"); 00528 //CS=1; 00529 // PowerUpNFC(); 00530 //////wait_ms(1); 00531 // NFCInit(); 00532 // RegisterReInitNFC(); 00533 MemReadReqNFC(); 00534 printf("back from MemReadReqNFC \r\n"); 00535 if (rssi>0x50) 00536 {rssi_flag=1;} 00537 else{rssi_flag=0;} 00538 nfc++; 00539 //InventoryReqNFC(); 00540 wait_ms(WAIT); 00541 00542 switch(found) { 00543 case BIT0: 00544 printf("no response:"); 00545 printf("%X \r\n",found); 00546 found=0; 00547 WAIT=0; 00548 break; 00549 // //case BIT1: 00550 // //case BIT2: 00551 // //case BIT3: 00552 //// case BIT4: 00553 //// case BIT5: 00554 case BIT6: 00555 ReadNFC(); 00556 //PowerDownNFC(); //SleepNFC() //StandByNFC() 00557 found=1; 00558 WAIT=0; 00559 break; 00560 case BIT7: 00561 printf("tx complete:"); 00562 printf("%X \r\n",found); 00563 found=0; 00564 WAIT=0; 00565 break; 00566 default: 00567 if((nfc>10)&&(rssi_flag=1)); 00568 {rssi_flag=0; 00569 nfc=0; 00570 PowerUpNFC(); 00571 NFCInit(); 00572 RegisterReInitNFC(); 00573 printf("resetting 7970 \r\n:"); 00574 found=0; 00575 } 00576 } 00577 00578 return found; 00579 00580 }
Generated on Tue Jul 26 2022 13:02:03 by
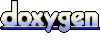