GroveStreams is a helper class to assist with pushing data into the GroveStreams.com IoT platform.
Embed:
(wiki syntax)
Show/hide line numbers
GroveStreams.h
00001 /* 00002 GroveStreams is a helper class to assist with pushing data into the 00003 GroveStreams.com IoT platform. 00004 00005 License: 00006 Copyright (C) 2017 GroveStreams LLC. 00007 Licensed under the Apache License, Version 2.0 (the "License"); 00008 you may not use this file except in compliance with the License. 00009 You may obtain a copy of the License at: http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 */ 00017 00018 #ifndef GROVESTREAMS_H_ 00019 #define GROVESTREAMS_H_ 00020 00021 #include "mbed.h" 00022 #include "EthernetInterface.h" 00023 #include "NetworkAPI/tcp/socket.hpp" 00024 #include "LcdDiscoF746NgTracer.h" 00025 00026 class GroveStreams 00027 { 00028 00029 protected: 00030 EthernetInterface _eth; 00031 LcdDiscoF746NgTracer* _pLcd; 00032 00033 const char* _domain; 00034 uint16_t _port; 00035 const char* _apiKey; 00036 const char* _myIPAddress; //Don't Change. Set below from DHCP. Needed by GroveStreams to verify that a device is not uploading more than once every 10s. 00037 00038 00039 public: 00040 00041 /** Constructor with apiKey and Lcd tracer 00042 * 00043 * \param apiKey the secret api_key for your GroveStreams organization 00044 * \param pLcd optional. Pass NULL or and LCD for tracing 00045 */ 00046 GroveStreams(const char* apiKey, LcdDiscoF746NgTracer* pLcd); 00047 00048 /** Constructor with apiKey and Lcd tracer. 00049 * 00050 * \param apiKey the secret api_key for your GroveStreams organization 00051 */ 00052 GroveStreams(const char* apiKey); 00053 00054 /** Destructor 00055 * 00056 */ 00057 ~GroveStreams(); 00058 00059 protected: 00060 int init(const char* apiKey, LcdDiscoF746NgTracer* pLcd); 00061 void printf(const char* format, ...); 00062 00063 public: 00064 00065 /** Send sample data to GroveStreams. Attempts to restore dropped ethernet 00066 * connections 00067 * 00068 * \param componentId The ID of the component to put the samples into 00069 * \param samples a string that is URL Encoded and contains all of the 00070 * samples. Place an ampersand at the beggining of each sample substring. 00071 * The substring is &streamId=value. 00072 * Example: &temp=6&eres=78.231&on=true&position=on+ground 00073 * \return 0 on success 00074 */ 00075 unsigned long send(const char* componentId, const char* samples); 00076 00077 00078 /** Send sample data to GroveStreams. Attempts to restore dropped ethernet 00079 * connections 00080 * 00081 * \param componentId The ID of the component to put the samples into 00082 * \param samples a string that is URL Encoded and contains all of the 00083 * samples. Place an ampersand at the beggining of each sample substring. 00084 * The substring is &streamId=value. 00085 * Example: &temp=6&eres=78.231&on=true&position=on+ground 00086 * \param resultBuffer a string that will contain the http result body 00087 * \param resultBufferSize the size of the result buffer 00088 * \return 0 on success 00089 */ 00090 unsigned long send(const char* componentId, const char* samples, char* resultBuffer, size_t resultBufferSize); 00091 00092 /** Send sample data to GroveStreams. Attempts to restore dropped ethernet 00093 * connections 00094 * 00095 * \param componentId The ID of the component to put the samples into 00096 * \param samples a string that is URL Encoded and contains all of the 00097 * samples. Place an ampersand at the beggining of each sample substring. 00098 * The substring is &streamId=value. 00099 * Example: &temp=6&eres=78.231&on=true&position=on+ground 00100 * \param componentName optional (NULL). the name assigned to a newly created component. 00101 * \param compTmplId optional (NULL). the component template ID to be used for creating a 00102 * new component 00103 * \param resultBuffer a string that will contain the http result body 00104 * \param resultBufferSize the size of the result buffer 00105 * \return 0 on success 00106 */ 00107 unsigned long send(const char* componentId, const char* samples, const char* componentName, const char* compTmplId, char* resultBuffer, size_t resultBufferSize); 00108 00109 /** Send sample data to GroveStreams. Does not attempt to restore dropped 00110 * ethernet connections. 00111 * 00112 * \param componentId The ID of the component to put the samples into 00113 * \param samples a string that is URL Encoded and contains all of the 00114 * samples. Place an ampersand at the beggining of each sample substring. 00115 * The substring is &streamId=value. 00116 * Example: &temp=6&eres=78.231&on=true&position=on+ground 00117 * \param componentName optional (NULL). the name assigned to a newly created component. 00118 * \param compTmplId optional (NULL). the component template ID to be used for creating a 00119 * new component 00120 * \param resultBuffer a string that will contain the http result body 00121 * \param resultBufferSize the size of the result buffer 00122 * \return 0 on success 00123 */ 00124 unsigned long sendNoRetry(const char* componentId, const char* samples, const char* componentName, const char* compTmplId, char* resultBuffer, size_t resultBufferSize); 00125 00126 /** Starts ethernet. 00127 * Stops ethernet if it is running before starting it. 00128 * \return 0 on success 00129 */ 00130 int startEthernet(); 00131 00132 /** Get the MAC address of your Ethernet interface 00133 * \return a pointer to a string containing the MAC address 00134 */ 00135 const char* getMACAddress(); 00136 00137 }; 00138 00139 #endif /* GROVESTREAMS_H_ */
Generated on Thu Jul 14 2022 03:13:34 by
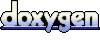