
This is a basic code to be used for Sequana BLE Lab exercises.
Embed:
(wiki syntax)
Show/hide line numbers
SequanaPrimaryService.cpp
00001 /* 00002 * Copyright (c) 2017-2019 Future Electronics 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <mbed.h> 00018 #include "ble/BLE.h" 00019 #include "UUID.h" 00020 00021 #include "SequanaPrimaryService.h" 00022 00023 00024 namespace sequana { 00025 00026 const UUID PrimaryService::UUID_SEQUANA_PRIMARY_SERVICE("F79B4EB2-1B6E-41F2-8D65-D346B4EF5685"); 00027 00028 UUID UUID_TEMPERATURE_CHAR(GattCharacteristic::UUID_TEMPERATURE_CHAR); 00029 UUID UUID_ACCELLEROMETER_CHAR("F79B4EB5-1B6E-41F2-8D65-D346B4EF5685"); 00030 UUID UUID_MAGNETOMETER_CHAR("F79B4EB7-1B6E-41F2-8D65-D346B4EF5685"); 00031 UUID UUID_AIR_QUALITY_CHAR("f79B4EBA-1B6E-41F2-8D65-D346B4EF5685"); 00032 UUID UUID_PARTICULATE_MATTER_CHAR("F79B4EBB-1B6B-41F2-8D65-D346B4EF5685"); 00033 UUID UUID_OTHER_ENV_CHAR("F79B4EBC-1B6E-41F2-8D65-D346B4EF5685"); 00034 00035 int16_t tempValue = 0; 00036 00037 SingleCharParams accMagSensorCharacteristics[2] = { 00038 { &UUID_ACCELLEROMETER_CHAR, 0, 6 }, 00039 { &UUID_MAGNETOMETER_CHAR, 6, 6 } 00040 }; 00041 00042 00043 PrimaryService::PrimaryService(BLE &ble, 00044 Kmx65Sensor &kmx65) : 00045 _ble(ble), 00046 _accMagSensorMeasurement(ble, 00047 accMagSensorCharacteristics, 00048 kmx65) 00049 { 00050 GattCharacteristic *sequanaChars[] = { 00051 _accMagSensorMeasurement.get_characteristic(0), 00052 _accMagSensorMeasurement.get_characteristic(1) 00053 }; 00054 00055 GattService sequanaService(UUID_SEQUANA_PRIMARY_SERVICE, sequanaChars, sizeof(sequanaChars) / sizeof(GattCharacteristic *)); 00056 00057 _ble.gattServer().addService(sequanaService); 00058 } 00059 00060 } //namespace 00061
Generated on Thu Jul 14 2022 02:36:35 by
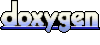