
Contains code to drive a small brushed DC motor with a Freescale FRDM-17510 MPC17510 H-bridge driver evaluation board, and a Freedom FRDM-KL25Z. Configures the KL25Z as USB HID device and requres a GUI on the PC.
main.cpp
00001 #include "mbed.h" 00002 #include "USBHID.h" 00003 00004 // We declare a USBHID device. 00005 // HID In/Out Reports are 64 Bytes long 00006 // Vendor ID (VID): 0x15A2 00007 // Product ID (PID): 0x0138 00008 // Serial Number: 0x0001 00009 USBHID hid(64, 64, 0x15A2, 0x0138, 0x0001, true); 00010 00011 //Setup Digital Outputs for the LEDs on the FRDM 00012 DigitalOut red_led(LED1); 00013 DigitalOut green_led(LED2); 00014 DigitalOut blue_led(LED3); 00015 00016 //Setup PWM and Digital Outputs from FRDM-KL25Z to FRDM-17510 00017 PwmOut IN1(PTD4); // Pin IN1 input to MPC17510 (FRDM PIN Name) 00018 PwmOut IN2(PTA12); // Pin IN2 input to MPC17510 (FRDM PIN Name) 00019 DigitalOut EN(PTC7); // Pin EN input to MPC17510 (FRDM PIN Name) 00020 DigitalOut GINB(PTC0); // Pin GIN_B input to MPC17510 (FRDM PIN Name) 00021 DigitalOut READY(PTC5); // Pin READY input to Motor Control Board (FRDM PIN Name) 00022 00023 //Variables 00024 int pwm_freq_lo; 00025 int pwm_freq_hi; 00026 int frequencyHz; 00027 int storedFreq; 00028 int storedDuty; 00029 int runstop = 0; 00030 int runStopChange; 00031 int direction = 1; 00032 int directionChange; 00033 int braking; 00034 int brakingChange; 00035 int dutycycle; 00036 int motorState = 0; 00037 int ramptime = 0; 00038 00039 //storage for send and receive data 00040 HID_REPORT send_report; 00041 HID_REPORT recv_report; 00042 00043 bool initflag = true; 00044 00045 // USB COMMANDS 00046 // These are sent from the PC 00047 #define WRITE_LED 0x20 00048 #define WRITE_GEN_EN 0x40 00049 #define WRITE_DUTY_CYCLE 0x50 00050 #define WRITE_PWM_FREQ 0x60 00051 #define WRITE_RUN_STOP 0x70 00052 #define WRITE_DIRECTION 0x71 00053 #define WRITE_BRAKING 0x90 00054 #define WRITE_RESET 0xA0 00055 00056 00057 // MOTOR STATES 00058 #define STOP 0x00 00059 #define RUN 0x02 00060 #define RESET 0x05 00061 00062 // LED CONSTANTS 00063 #define LEDS_OFF 0x00 00064 #define RED 0x01 00065 #define GREEN 0x02 00066 #define BLUE 0x03 00067 #define READY_LED 0x04 00068 00069 // LOGICAL CONSTANTS 00070 #define OFF 0x00 00071 #define ON 0x01 00072 00073 //Functions 00074 void set_PWM_Freq(int freq); 00075 void set_Duty_Cycle(int dutycycle); 00076 00077 00078 int main() 00079 { 00080 send_report.length = 64; 00081 recv_report.length = 64; 00082 00083 red_led = 1; // Red LED = OFF 00084 green_led = 1; // Green LED = OFF 00085 blue_led = 0; // Blue LED = ON 00086 00087 motorState = RESET; 00088 initflag = true; 00089 00090 IN1.period(1); //initially set period to 1 second 00091 IN2.period(1); 00092 00093 IN1.write(0); //set PWM percentage to 0 00094 IN2.write(0); 00095 00096 00097 while(1) 00098 { 00099 //try to read a msg 00100 if(hid.readNB(&recv_report)) 00101 { 00102 if(initflag == true) 00103 { 00104 initflag = false; 00105 blue_led = 1; //turn off blue LED 00106 } 00107 switch(recv_report.data[0]) //byte 0 of recv_report.data is command 00108 { 00109 //----------------------------------------------------------------------------------------------------------------- 00110 // COMMAND PARSER 00111 //----------------------------------------------------------------------------------------------------------------- 00112 //////// 00113 case WRITE_LED: 00114 switch(recv_report.data[1]) 00115 { 00116 case LEDS_OFF: 00117 red_led = 1; 00118 green_led = 1; 00119 blue_led = 1; 00120 break; 00121 case RED: 00122 if(recv_report.data[2] == 1){red_led = 0;} else {red_led = 1;} 00123 break; 00124 case GREEN: 00125 if(recv_report.data[2] == 1){green_led = 0;} else {green_led = 1;} 00126 break; 00127 case BLUE: 00128 if(recv_report.data[2] == 1){blue_led = 0;} else {blue_led = 1;} 00129 break; 00130 default: 00131 break; 00132 00133 00134 }// End recv report data[1] 00135 break; 00136 //////// 00137 00138 //////// 00139 case WRITE_DUTY_CYCLE: 00140 dutycycle = recv_report.data[1]; 00141 set_Duty_Cycle(dutycycle); 00142 break; 00143 //////// 00144 case WRITE_PWM_FREQ: //PWM frequency can be larger than 1 byte 00145 pwm_freq_lo = recv_report.data[1]; //so we have to re-assemble the number 00146 pwm_freq_hi = recv_report.data[2] * 100; 00147 frequencyHz = pwm_freq_lo + pwm_freq_hi; 00148 set_PWM_Freq(frequencyHz); 00149 break; 00150 //////// 00151 case WRITE_RUN_STOP: 00152 if(recv_report.data[1] == 1) 00153 { 00154 if(runstop != 1) 00155 { 00156 red_led = 1; 00157 blue_led = 1; 00158 green_led = 0; 00159 READY = 1; 00160 runstop = 1; 00161 runStopChange = 1; 00162 motorState = RUN; 00163 frequencyHz = storedFreq; 00164 set_PWM_Freq(frequencyHz); 00165 dutycycle = storedDuty; 00166 set_Duty_Cycle(dutycycle); 00167 } 00168 00169 } 00170 else 00171 { 00172 if(runstop != 0) 00173 { 00174 runstop = 0; 00175 runStopChange = 1; 00176 motorState = STOP; 00177 red_led = 0; 00178 green_led = 1; 00179 blue_led = 1; 00180 storedFreq = frequencyHz; 00181 storedDuty = dutycycle; 00182 READY = 0; 00183 } 00184 } 00185 break; 00186 //////// 00187 case WRITE_DIRECTION: 00188 00189 00190 if(recv_report.data[1] == 1) 00191 { 00192 if(direction != 1) 00193 { 00194 direction = 1; 00195 directionChange = 1; 00196 } 00197 } 00198 else 00199 { 00200 if(direction != 0) 00201 { 00202 direction = 0; 00203 directionChange = 1; 00204 } 00205 } 00206 break; 00207 //////// 00208 case WRITE_BRAKING: 00209 if(recv_report.data[1] == 1) 00210 { 00211 braking = 1; 00212 } 00213 else 00214 { 00215 braking = 0; 00216 } 00217 break; 00218 //////// 00219 default: 00220 break; 00221 }// End Switch recv report data[0] 00222 00223 //----------------------------------------------------------------------------------------------------------------- 00224 // end command parser 00225 //----------------------------------------------------------------------------------------------------------------- 00226 00227 send_report.data[0] = recv_report.data[0]; // Echo Command 00228 send_report.data[1] = recv_report.data[1]; // Echo Subcommand 1 00229 send_report.data[2] = recv_report.data[2]; // Echo Subcommand 2 00230 send_report.data[3] = 0x00; 00231 send_report.data[4] = 0x00; 00232 send_report.data[5] = 0x00; 00233 send_report.data[6] = 0x00; 00234 send_report.data[7] = 0x00; 00235 00236 //Send the report 00237 hid.send(&send_report); 00238 }// End If(hid.readNB(&recv_report)) 00239 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00240 //End of USB message handling 00241 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00242 00243 //************************************************************************************************************************ 00244 // This is handling of PWM, braking, direction 00245 //***********************************************************************************************************************/ 00246 00247 switch (motorState) 00248 { 00249 case STOP: 00250 READY = 0; 00251 00252 if(braking == 1) 00253 { 00254 EN = 0; 00255 IN1.period(1); 00256 IN2.period(1); 00257 IN1.write(0); 00258 IN2.write(0); 00259 } 00260 else 00261 { 00262 EN = 0; 00263 IN1.period(1); 00264 IN2.period(1); 00265 IN1.write(0); 00266 IN2.write(0); 00267 EN = 1; 00268 } 00269 break; 00270 00271 case RUN: 00272 if(runStopChange != 0) 00273 { 00274 READY = 1; 00275 EN = 1; 00276 directionChange = 0; 00277 runStopChange = 0; 00278 set_PWM_Freq(frequencyHz); 00279 set_Duty_Cycle(dutycycle); 00280 } 00281 break; 00282 00283 case RESET: 00284 IN1.write(0.0); 00285 IN2.write(0.0); 00286 IN1.period(0.0); 00287 IN2.period(0.0); 00288 runStopChange = false; 00289 motorState = STOP; 00290 break; 00291 00292 }// end switch motorState 00293 /////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00294 }// End While Loop 00295 }// End Main 00296 00297 00298 //////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00299 //////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00300 00301 void set_PWM_Freq(int freq) 00302 { 00303 storedFreq = freq; 00304 float period = 1.0/freq; 00305 00306 if(direction == 1) 00307 { 00308 IN1.period(period); 00309 IN2.period(0.0); 00310 } 00311 else 00312 { 00313 IN1.period(0.0); 00314 IN2.period(period); 00315 } 00316 } 00317 00318 void set_Duty_Cycle(int dc) 00319 { 00320 storedDuty = dc; 00321 if(direction == 1) 00322 { 00323 red_led = 0; 00324 green_led = 1; 00325 IN1.write(float(dc/100.0)); 00326 IN2.write(0); 00327 } 00328 else 00329 { 00330 red_led = 1; 00331 green_led = 0; 00332 IN2.write(float(dc/100.00)); 00333 IN1.write(0); 00334 } 00335 }
Generated on Sat Jul 30 2022 04:07:15 by
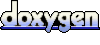