Light_and_Temp_Control
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /*This program monitoring the light and the temperature and send a serial command (if the user chose it) and turn on a light signal*/ 00002 00003 #include "mbed.h" 00004 #include "Servo.h" 00005 00006 Servo Servo_Temp(D13); 00007 Servo Servo_Light(D14); 00008 AnalogIn temperature(A0); 00009 AnalogIn light(A1); 00010 DigitalOut temp_alert(D0); 00011 DigitalOut light_alert(D1); 00012 int i; 00013 Serial pc(USBTX, USBRX); // tx, rx 00014 00015 int main() 00016 { 00017 temp_alert=0; 00018 light_alert=0; 00019 pc.printf("Press 'y' to receive commands or 'n' for not receive\n"); 00020 char mode = pc.getc(); 00021 while(1) { 00022 if (temperature>5) { //High Temperature 00023 i=0; 00024 while(i<10) { 00025 temp_alert=1; 00026 wait(1); 00027 temp_alert=0; 00028 i++; 00029 } 00030 temp_alert=1; 00031 Servo_Temp.position(0); 00032 if(mode=='y') 00033 pc.printf("High Temperature"); 00034 00035 } else 00036 temp_alert=0; 00037 Servo_Temp.position(100); 00038 00039 if (light<1) { //Low Light 00040 i=0; 00041 while(i<10) { 00042 light_alert=1; 00043 wait(1); 00044 light_alert=0; 00045 i++; 00046 } 00047 light_alert=1; 00048 Servo_Light.position(0); 00049 if(mode=='y') 00050 pc.printf("Low Light"); 00051 } else 00052 light_alert=0; 00053 Servo_Light.position(100); 00054 wait(1); 00055 } 00056 }
Generated on Tue Jul 19 2022 08:22:35 by
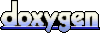