
p
Dependencies: SDFileSystem SPI_TFT_ILI9341 TFT_fonts mbed
main.cpp
00001 #include "mbed.h" 00002 #include "SPI_TFT_ILI9341.h" 00003 #include "stdio.h" 00004 #include "string" 00005 #include "Arial12x12.h" 00006 #include "Arial24x23.h" 00007 #include "Arial28x28.h" 00008 #include "font_big.h" 00009 #include "SDFileSystem.h" 00010 PwmOut mypwm(PWM_OUT); 00011 SDFileSystem sd(SPI_MOSI, SPI_MISO, SPI_SCK, D7, "sd"); 00012 DigitalOut myled(LED1); 00013 PwmOut RED(D8); 00014 PwmOut GREEN(D10); 00015 PwmOut BLUE(D9); 00016 InterruptIn button(USER_BUTTON); 00017 extern unsigned char p1[]; 00018 extern unsigned char p2[]; 00019 extern unsigned char p3[]; 00020 char List[4][8]={"track01","track02","track03","track04"}; 00021 Serial pc(SERIAL_TX,SERIAL_RX); 00022 // the TFT is connected to SPI pin 11-14 00023 int mark=10,list_nowplay=0; 00024 SPI_TFT_ILI9341 TFT(SPI_MOSI,SPI_MISO,SPI_SCK,SPI_CS, D9, D8,"TFT"); // mosi, miso, sclk, cs, reset, dc 00025 00026 void letplay() 00027 { 00028 TFT.cls(); 00029 TFT.foreground(White); 00030 TFT.background(Black); 00031 TFT.cls(); 00032 TFT.set_orientation(1); 00033 TFT.Bitmap(60,1,200,173,p1); 00034 } 00035 void angry() 00036 { 00037 TFT.cls(); 00038 TFT.foreground(White); 00039 TFT.background(Black); 00040 TFT.cls(); 00041 TFT.set_orientation(1); 00042 TFT.Bitmap(60,1,200,173,p2); 00043 } 00044 void cry() 00045 { 00046 TFT.cls(); 00047 TFT.foreground(White); 00048 TFT.background(Black); 00049 TFT.cls(); 00050 TFT.set_orientation(1); 00051 TFT.Bitmap(60,1,200,173,p3); 00052 } 00053 void print_list() 00054 { 00055 int i=0,j=0; 00056 TFT.claim(stdout); 00057 TFT.cls(); 00058 TFT.foreground(White); 00059 TFT.background(Black); 00060 TFT.cls(); 00061 00062 TFT.set_orientation(3); 00063 TFT.set_font((unsigned char*) Arial28x28); 00064 TFT.locate(150,120); 00065 TFT.printf("Manual Mode:"); 00066 TFT.cls(); 00067 TFT.set_orientation(3); 00068 TFT.set_font((unsigned char*) Arial24x23); 00069 do 00070 { 00071 TFT.locate(5,j); 00072 TFT.printf("%2d . %s\r\n", i,List[i]); 00073 i++; 00074 j=j+23; 00075 }while(i<4); 00076 00077 } 00078 void select_list() 00079 { 00080 //i=10; 00081 if(mark>=96) 00082 { 00083 mark=10; 00084 } 00085 TFT.cls(); 00086 print_list(); 00087 TFT.set_orientation(0); 00088 TFT.fillcircle(mark,20,10,Red); 00089 00090 mark=mark+23; 00091 list_nowplay++; 00092 //pc.printf("%d\n",list_nowplay); 00093 } 00094 void SmoothRed() 00095 { 00096 int i,j; 00097 for(i=0.0;i<=1.0;i+=0.1) 00098 { 00099 RED.write(i); 00100 wait(1); 00101 for(j=0.0;j<=1.0;j+=0.1) 00102 { 00103 GREEN.write(j); 00104 wait(1); 00105 } 00106 } 00107 00108 /* for(i=1.0;i>=0;i-=0.1) 00109 { 00110 RED.write(i); 00111 wait(1); 00112 }*/ 00113 00114 } 00115 00116 int main() 00117 { 00118 // print_list(); 00119 // select_list(); 00120 // button.rise(&select_list); 00121 while(1) 00122 { 00123 SmoothRed(); 00124 wait(2); 00125 } 00126 00127 }
Generated on Thu Jul 14 2022 18:08:54 by
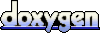