Evrythng API for W5500, WIZ550io
Dependencies: W5500Interface
Dependents: EvrythngApiExampleW5500
Fork of EvrythngApi by
EvrythngApi.h
00001 /* 00002 * (c) Copyright 2012 EVRYTHNG Ltd London / Zurich 00003 * www.evrythng.com 00004 * 00005 * --- DISCLAIMER --- 00006 * 00007 * EVRYTHNG provides this source code "as is" and without warranty of any kind, 00008 * and hereby disclaims all express or implied warranties, including without 00009 * limitation warranties of merchantability, fitness for a particular purpose, 00010 * performance, accuracy, reliability, and non-infringement. 00011 * 00012 * Author: Michel Yerly 00013 * 00014 */ 00015 #ifndef EVRYTHNGAPI_H 00016 #define EVRYTHNGAPI_H 00017 00018 // not changed code, but import different library, W5500Interface. 00019 #include "EthernetInterface.h" 00020 #include <string> 00021 00022 #include <stdint.h> 00023 00024 enum HttpMethod { 00025 GET, PUT, POST, DELETE 00026 }; 00027 00028 00029 /* 00030 * Class to communicate with EVRYTHNG engine. 00031 */ 00032 class EvrythngApi 00033 { 00034 public: 00035 00036 /* 00037 * Constructor 00038 */ 00039 EvrythngApi(const std::string& token, const std::string& host = "api.evrythng.com", int port = 80); 00040 00041 /* 00042 * Destructor 00043 */ 00044 virtual ~EvrythngApi(); 00045 00046 /* 00047 * Reads the current value of a thng's property. The value read is put 00048 * in the value parameter. 00049 * Returns 0 on success, or an error code on error. Error codes are 00050 * described in evry_error.h. 00051 */ 00052 int getThngPropertyValue(const std::string& thngId, const std::string& key, std::string& value); 00053 00054 /* 00055 * Sets the value of a thng's property. 00056 * Returns 0 on success, or an error code on error. Error codes are 00057 * described in evry_error.h. 00058 */ 00059 int setThngPropertyValue(const std::string& thngId, const std::string& key, const std::string& value, int64_t timestamp); 00060 00061 private: 00062 std::string token; 00063 std::string host; 00064 int port; 00065 00066 int httpRequest(HttpMethod method, const std::string& path, const std::string& content, std::string& out, int& codeOut); 00067 00068 int httpPut(const std::string& path, const std::string& json, std::string& out, int& codeOut); 00069 int httpGet(const std::string& path, std::string& out, int& codeOut); 00070 int httpPost(const std::string& path, const std::string& json, std::string& out, int& codeOut); 00071 int httpDelete(const std::string& path, std::string& out, int& codeOut); 00072 }; 00073 00074 #endif
Generated on Wed Jul 20 2022 03:34:21 by
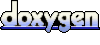