Example using the support package for LPC4088 DisplayModule
Dependencies: DMBasicGUI DMSupport
AppUSBMouse.cpp
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #include "mbed.h" 00019 #include "AppUSBMouse.h" 00020 #include "lpc_swim_font.h" 00021 #include "lpc_colors.h" 00022 #include "USBMouse.h" 00023 00024 /****************************************************************************** 00025 * Defines and typedefs 00026 *****************************************************************************/ 00027 00028 /****************************************************************************** 00029 * Local variables 00030 *****************************************************************************/ 00031 00032 00033 /****************************************************************************** 00034 * Private functions 00035 *****************************************************************************/ 00036 00037 void AppUSBMouse::draw() 00038 { 00039 // Prepare fullscreen 00040 swim_window_open(_win, 00041 _disp->width(), _disp->height(), // full size 00042 (COLOR_T*)_fb, 00043 0,0,_disp->width()-1, _disp->height()-1, // window position and size 00044 0, // border 00045 BLACK, WHITE, BLACK); // colors: pen, backgr, forgr 00046 00047 swim_put_text_centered_win(_win, "(Move PC's cursor with touch display. Press USER button to exit)", _disp->height()-30); 00048 } 00049 00050 /****************************************************************************** 00051 * Public functions 00052 *****************************************************************************/ 00053 00054 AppUSBMouse::AppUSBMouse() : _disp(NULL), _win(NULL), _fb(NULL) 00055 { 00056 _coords[0].z = _coords[1].z = 0; 00057 } 00058 00059 AppUSBMouse::~AppUSBMouse() 00060 { 00061 teardown(); 00062 } 00063 00064 bool AppUSBMouse::setup() 00065 { 00066 _disp = DMBoard::instance().display(); 00067 _win = (SWIM_WINDOW_T*)malloc(sizeof(SWIM_WINDOW_T)); 00068 _fb = _disp->allocateFramebuffer(); 00069 00070 return (_win != NULL && _fb != NULL); 00071 } 00072 00073 void AppUSBMouse::runToCompletion() 00074 { 00075 bool done = false; 00076 uint32_t flags = 0; 00077 draw(); 00078 void* oldFB = _disp->swapFramebuffer(_fb); 00079 00080 DMBoard* board = &DMBoard::instance(); 00081 RtosLog* log = board->logger(); 00082 00083 USBMouse mouse; 00084 00085 // Wait for touches 00086 TouchPanel* touch = DMBoard::instance().touchPanel(); 00087 int set = 0; 00088 touch_coordinate_t* last = NULL; 00089 touch_coordinate_t* current = &_coords[set]; 00090 while(!done) { 00091 // wait for a new touch signal (signal is sent from AppLauncher, 00092 // which listens for touch events) 00093 flags = ThisThread::flags_wait_all_for(0x1, 50); 00094 if (flags == 0) { 00095 if (board->buttonPressed()) { 00096 done = true; 00097 } 00098 } else { 00099 if (touch->read(current, 1) == TouchPanel::TouchError_Ok) { 00100 if (current->z == 0) { 00101 // lifted the finger so we have to think of the next touch 00102 // event as the first one (otherwise the relative movement 00103 // will be wrong) 00104 last = NULL; 00105 } 00106 if (last != NULL) { 00107 int moveX = current->x - last->x; 00108 int moveY = current->y - last->y; 00109 if (moveX != 0 && moveY != 0) { 00110 mouse.move(current->x - last->x, current->y - last->y); 00111 //log->printf("{%3d,%3d} -> {%3d, %3d}\n", last->x, last->y, current->x, current->y); 00112 //log->printf("move(%4d, %4d)\n", moveX, moveY); 00113 } 00114 } 00115 last = current; 00116 current = &_coords[(++set)&1]; 00117 } 00118 } 00119 } 00120 00121 // User has clicked the button, restore the original FB 00122 _disp->swapFramebuffer(oldFB); 00123 swim_window_close(_win); 00124 } 00125 00126 bool AppUSBMouse::teardown() 00127 { 00128 if (_win != NULL) { 00129 free(_win); 00130 _win = NULL; 00131 } 00132 if (_fb != NULL) { 00133 free(_fb); 00134 _fb = NULL; 00135 } 00136 return true; 00137 }
Generated on Thu Jul 14 2022 09:18:18 by
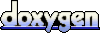