Adaptation of the official mbed USBHost repository to work with the LPC4088 Display Module
Dependents: DMSupport DMSupport DMSupport DMSupport
Fork of DM_USBHost by
USBHost.cpp
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #include "USBHost.h" 00019 #include "USBHostHub.h" 00020 00021 USBHost * USBHost::instHost = NULL; 00022 00023 #define DEVICE_CONNECTED_EVENT (1 << 0) 00024 #define DEVICE_DISCONNECTED_EVENT (1 << 1) 00025 #define TD_PROCESSED_EVENT (1 << 2) 00026 00027 #define MAX_TRY_ENUMERATE_HUB 3 00028 00029 #define MIN(a, b) ((a > b) ? b : a) 00030 00031 /** 00032 * How interrupts are processed: 00033 * - new device connected: 00034 * - a message is queued in queue_usb_event with the id DEVICE_CONNECTED_EVENT 00035 * - when the usb_thread receives the event, it: 00036 * - resets the device 00037 * - reads the device descriptor 00038 * - sets the address of the device 00039 * - if it is a hub, enumerates it 00040 * - device disconnected: 00041 * - a message is queued in queue_usb_event with the id DEVICE_DISCONNECTED_EVENT 00042 * - when the usb_thread receives the event, it: 00043 * - free the device and all its children (hub) 00044 * - td processed 00045 * - a message is queued in queue_usb_event with the id TD_PROCESSED_EVENT 00046 * - when the usb_thread receives the event, it: 00047 * - call the callback attached to the endpoint where the td is attached 00048 */ 00049 void USBHost::usb_process() { 00050 00051 bool controlListState; 00052 bool bulkListState; 00053 bool interruptListState; 00054 USBEndpoint * ep; 00055 uint8_t i, j, res, timeout_set_addr = 10; 00056 #define SAFE_USB_PROCESS_BUFF_SIZE (8) 00057 uint8_t* buf = getSafeMem(SAFE_USB_PROCESS_BUFF_SIZE); 00058 bool too_many_hub; 00059 int idx; 00060 00061 #if DEBUG_TRANSFER 00062 uint8_t * buf_transfer; 00063 #endif 00064 00065 #if MAX_HUB_NB 00066 uint8_t k; 00067 #endif 00068 00069 while(1) { 00070 osEvent evt = mail_usb_event.get(); 00071 00072 if (evt.status == osEventMail) { 00073 00074 message_t * usb_msg = (message_t*)evt.value.p; 00075 00076 switch (usb_msg->event_id) { 00077 00078 // a new device has been connected 00079 case DEVICE_CONNECTED_EVENT: 00080 too_many_hub = false; 00081 buf[4] = 0; 00082 00083 do 00084 { 00085 Lock lock(this); 00086 00087 for (i = 0; i < MAX_DEVICE_CONNECTED; i++) { 00088 if (!deviceInUse[i]) { 00089 USB_DBG_EVENT("new device connected: %p\r\n", &devices[i]); 00090 devices[i].init(usb_msg->hub, usb_msg->port, usb_msg->lowSpeed); 00091 deviceReset[i] = false; 00092 deviceInited[i] = true; 00093 break; 00094 } 00095 } 00096 00097 if (i == MAX_DEVICE_CONNECTED) { 00098 USB_ERR("Too many device connected!!\r\n"); 00099 continue; 00100 } 00101 00102 if (!controlEndpointAllocated) { 00103 control = newEndpoint(CONTROL_ENDPOINT, OUT, 0x08, 0x00); 00104 addEndpoint(NULL, 0, (USBEndpoint*)control); 00105 controlEndpointAllocated = true; 00106 } 00107 00108 #if MAX_HUB_NB 00109 if (usb_msg->hub_parent) 00110 devices[i].setHubParent((USBHostHub *)(usb_msg->hub_parent)); 00111 #endif 00112 00113 for (j = 0; j < timeout_set_addr; j++) { 00114 00115 resetDevice(&devices[i]); 00116 00117 // set size of control endpoint 00118 devices[i].setSizeControlEndpoint(8); 00119 00120 devices[i].activeAddress(false); 00121 00122 // get first 8 bit of device descriptor 00123 // and check if we deal with a hub 00124 USB_DBG("usb_thread read device descriptor on dev: %p\r\n", &devices[i]); 00125 res = getDeviceDescriptor(&devices[i], buf, SAFE_USB_PROCESS_BUFF_SIZE); 00126 00127 if (res != USB_TYPE_OK) { 00128 USB_ERR("usb_thread could not read dev descr"); 00129 continue; 00130 } 00131 00132 // set size of control endpoint 00133 devices[i].setSizeControlEndpoint(buf[7]); 00134 00135 // second step: set an address to the device 00136 res = setAddress(&devices[i], devices[i].getAddress()); 00137 00138 if (res != USB_TYPE_OK) { 00139 USB_ERR("SET ADDR FAILED"); 00140 continue; 00141 } 00142 devices[i].activeAddress(true); 00143 USB_DBG("Address of %p: %d", &devices[i], devices[i].getAddress()); 00144 00145 // try to read again the device descriptor to check if the device 00146 // answers to its new address 00147 res = getDeviceDescriptor(&devices[i], buf, SAFE_USB_PROCESS_BUFF_SIZE); 00148 00149 if (res == USB_TYPE_OK) { 00150 break; 00151 } 00152 00153 ThisThread::sleep_for(100); 00154 } 00155 00156 USB_INFO("New device connected: %p [hub: %d - port: %d]", &devices[i], usb_msg->hub, usb_msg->port); 00157 00158 #if MAX_HUB_NB 00159 if (buf[4] == HUB_CLASS) { 00160 for (k = 0; k < MAX_HUB_NB; k++) { 00161 if (hub_in_use[k] == false) { 00162 for (uint8_t j = 0; j < MAX_TRY_ENUMERATE_HUB; j++) { 00163 if (hubs[k].connect(&devices[i])) { 00164 devices[i].hub = &hubs[k]; 00165 hub_in_use[k] = true; 00166 break; 00167 } 00168 } 00169 if (hub_in_use[k] == true) 00170 break; 00171 } 00172 } 00173 00174 if (k == MAX_HUB_NB) { 00175 USB_ERR("Too many hubs connected!!\r\n"); 00176 too_many_hub = true; 00177 } 00178 } 00179 00180 if (usb_msg->hub_parent) 00181 ((USBHostHub *)(usb_msg->hub_parent))->deviceConnected(&devices[i]); 00182 #endif 00183 00184 if ((i < MAX_DEVICE_CONNECTED) && !too_many_hub) { 00185 deviceInUse[i] = true; 00186 } 00187 00188 } while(0); 00189 00190 if (listener != 0) { 00191 listener->set(listenerSignal); 00192 } 00193 00194 break; 00195 00196 // a device has been disconnected 00197 case DEVICE_DISCONNECTED_EVENT: 00198 00199 do 00200 { 00201 Lock lock(this); 00202 00203 controlListState = disableList(CONTROL_ENDPOINT); 00204 bulkListState = disableList(BULK_ENDPOINT); 00205 interruptListState = disableList(INTERRUPT_ENDPOINT); 00206 00207 idx = findDevice(usb_msg->hub, usb_msg->port, (USBHostHub *)(usb_msg->hub_parent)); 00208 if (idx != -1) { 00209 freeDevice((USBDeviceConnected*)&devices[idx]); 00210 } 00211 00212 if (controlListState) enableList(CONTROL_ENDPOINT); 00213 if (bulkListState) enableList(BULK_ENDPOINT); 00214 if (interruptListState) enableList(INTERRUPT_ENDPOINT); 00215 00216 } while(0); 00217 00218 if (listener != 0) { 00219 listener->set(listenerSignal); 00220 } 00221 00222 break; 00223 00224 // a td has been processed 00225 // call callback on the ed associated to the td 00226 // we are not in ISR -> users can use printf in their callback method 00227 case TD_PROCESSED_EVENT: 00228 ep = (USBEndpoint *) ((HCTD *)usb_msg->td_addr)->ep; 00229 if (usb_msg->td_state == USB_TYPE_IDLE) { 00230 USB_DBG_EVENT("call callback on td %p [ep: %p state: %s - dev: %p - %s]", usb_msg->td_addr, ep, ep->getStateString(), ep->dev, ep->dev->getName(ep->getIntfNb())); 00231 00232 #if DEBUG_TRANSFER 00233 if (ep->getDir() == IN) { 00234 buf_transfer = ep->getBufStart(); 00235 USB_DBG_X("READ SUCCESS [%d bytes transferred - td: 0x%08X] on ep: [%p - addr: %02X]: ", ep->getLengthTransferred(), usb_msg->td_addr, ep, ep->getAddress()); 00236 for (int i = 0; i < ep->getLengthTransferred(); i++) 00237 USB_DBG_X("%02X ", buf_transfer[i]); 00238 USB_DBG_X("\r\n\r\n"); 00239 } 00240 #endif 00241 ep->call(); 00242 } else { 00243 idx = findDevice(ep->dev); 00244 if (idx != -1) { 00245 if (deviceInUse[idx]) { 00246 USB_WARN("td %p processed but not in idle state: %s [ep: %p - dev: %p - %s]", usb_msg->td_addr, ep->getStateString(), ep, ep->dev, ep->dev->getName(ep->getIntfNb())); 00247 ep->setState(USB_TYPE_IDLE); 00248 } 00249 } 00250 } 00251 break; 00252 } 00253 00254 mail_usb_event.free(usb_msg); 00255 } 00256 } 00257 } 00258 00259 /* static */void USBHost::usb_process_static(void const * arg) { 00260 ((USBHost *)arg)->usb_process(); 00261 } 00262 00263 USBHost::USBHost() : usbThread(osPriorityNormal, USB_THREAD_STACK) 00264 { 00265 headControlEndpoint = NULL; 00266 headBulkEndpoint = NULL; 00267 headInterruptEndpoint = NULL; 00268 tailControlEndpoint = NULL; 00269 tailBulkEndpoint = NULL; 00270 tailInterruptEndpoint = NULL; 00271 00272 lenReportDescr = 0; 00273 00274 setupPacket = getSafeMem(SAFE_USB_HOST_SETUP_PACKET_BUFF_SIZE); 00275 data = getSafeMem(SAFE_USB_HOST_DATA_BUFF_SIZE); 00276 00277 controlEndpointAllocated = false; 00278 00279 for (uint8_t i = 0; i < MAX_DEVICE_CONNECTED; i++) { 00280 deviceInUse[i] = false; 00281 devices[i].setAddress(i + 1); 00282 deviceReset[i] = false; 00283 deviceInited[i] = false; 00284 for (uint8_t j = 0; j < MAX_INTF; j++) 00285 deviceAttachedDriver[i][j] = false; 00286 } 00287 00288 #if MAX_HUB_NB 00289 for (uint8_t i = 0; i < MAX_HUB_NB; i++) { 00290 hubs[i].setHost(this); 00291 hub_in_use[i] = false; 00292 } 00293 #endif 00294 00295 listener = NULL; 00296 listenerSignal = 0; 00297 00298 usbThread.start(callback(USBHost::usb_process_static, (void *)this)); 00299 } 00300 00301 USBHost::Lock::Lock(USBHost* pHost) : m_pHost(pHost) 00302 { 00303 m_pHost->usb_mutex.lock(); 00304 } 00305 00306 USBHost::Lock::~Lock() 00307 { 00308 m_pHost->usb_mutex.unlock(); 00309 } 00310 00311 void USBHost::transferCompleted(volatile uint32_t addr) 00312 { 00313 uint8_t state; 00314 00315 if(addr == 0) 00316 return; 00317 00318 volatile HCTD* tdList = NULL; 00319 00320 //First we must reverse the list order and dequeue each TD 00321 do { 00322 volatile HCTD* td = (volatile HCTD*)addr; 00323 addr = (uint32_t)td->nextTD; //Dequeue from physical list 00324 td->nextTD = tdList; //Enqueue into reversed list 00325 tdList = td; 00326 } while(addr); 00327 00328 while(tdList != NULL) { 00329 volatile HCTD* td = tdList; 00330 tdList = (volatile HCTD*)td->nextTD; //Dequeue element now as it could be modified below 00331 if (td->ep != NULL) { 00332 USBEndpoint * ep = (USBEndpoint *)(td->ep); 00333 00334 if (((HCTD *)td)->control >> 28) { 00335 state = ((HCTD *)td)->control >> 28; 00336 } else { 00337 if (td->currBufPtr) 00338 ep->setLengthTransferred((uint32_t)td->currBufPtr - (uint32_t)ep->getBufStart()); 00339 state = 16 /*USB_TYPE_IDLE*/; 00340 } 00341 00342 ep->unqueueTransfer(td); 00343 00344 if (ep->getType() != CONTROL_ENDPOINT) { 00345 // callback on the processed td will be called from the usb_thread (not in ISR) 00346 message_t * usb_msg = mail_usb_event.alloc(); 00347 usb_msg->event_id = TD_PROCESSED_EVENT; 00348 usb_msg->td_addr = (void *)td; 00349 usb_msg->td_state = state; 00350 mail_usb_event.put(usb_msg); 00351 } 00352 ep->setState(state); 00353 ep->ep_queue.put((uint8_t*)1); 00354 } 00355 } 00356 } 00357 00358 USBHost * USBHost::getHostInst() 00359 { 00360 if (instHost == NULL) { 00361 instHost = new USBHost(); 00362 instHost->init(); 00363 } 00364 00365 return instHost; 00366 } 00367 00368 00369 /* 00370 * Called when a device has been connected 00371 * Called in ISR!!!! (no printf) 00372 */ 00373 /* virtual */ void USBHost::deviceConnected(int hub, int port, bool lowSpeed, USBHostHub * hub_parent) 00374 { 00375 // be sure that the new device connected is not already connected... 00376 int idx = findDevice(hub, port, hub_parent); 00377 if (idx != -1) { 00378 if (deviceInited[idx]) 00379 return; 00380 } 00381 00382 message_t * usb_msg = mail_usb_event.alloc(); 00383 usb_msg->event_id = DEVICE_CONNECTED_EVENT; 00384 usb_msg->hub = hub; 00385 usb_msg->port = port; 00386 usb_msg->lowSpeed = lowSpeed; 00387 usb_msg->hub_parent = hub_parent; 00388 mail_usb_event.put(usb_msg); 00389 } 00390 00391 /* 00392 * Called when a device has been disconnected 00393 * Called in ISR!!!! (no printf) 00394 */ 00395 /* virtual */ void USBHost::deviceDisconnected(int hub, int port, USBHostHub * hub_parent, volatile uint32_t addr) 00396 { 00397 // be sure that the device disconnected is connected... 00398 int idx = findDevice(hub, port, hub_parent); 00399 if (idx != -1) { 00400 if (!deviceInUse[idx]) 00401 return; 00402 } else { 00403 return; 00404 } 00405 00406 message_t * usb_msg = mail_usb_event.alloc(); 00407 usb_msg->event_id = DEVICE_DISCONNECTED_EVENT; 00408 usb_msg->hub = hub; 00409 usb_msg->port = port; 00410 usb_msg->hub_parent = hub_parent; 00411 mail_usb_event.put(usb_msg); 00412 } 00413 00414 void USBHost::freeDevice(USBDeviceConnected * dev) 00415 { 00416 USBEndpoint * ep = NULL; 00417 HCED * ed = NULL; 00418 00419 #if MAX_HUB_NB 00420 if (dev->getClass() == HUB_CLASS) { 00421 if (dev->hub == NULL) { 00422 USB_ERR("HUB NULL!!!!!\r\n"); 00423 } else { 00424 dev->hub->hubDisconnected(); 00425 for (uint8_t i = 0; i < MAX_HUB_NB; i++) { 00426 if (dev->hub == &hubs[i]) { 00427 hub_in_use[i] = false; 00428 break; 00429 } 00430 } 00431 } 00432 } 00433 00434 // notify hub parent that this device has been disconnected 00435 if (dev->getHubParent()) 00436 dev->getHubParent()->deviceDisconnected(dev); 00437 00438 #endif 00439 00440 int idx = findDevice(dev); 00441 if (idx != -1) { 00442 deviceInUse[idx] = false; 00443 deviceReset[idx] = false; 00444 00445 for (uint8_t j = 0; j < MAX_INTF; j++) { 00446 deviceAttachedDriver[idx][j] = false; 00447 if (dev->getInterface(j) != NULL) { 00448 USB_DBG("FREE INTF %d on dev: %p, %p, nb_endpot: %d, %s", j, (void *)dev->getInterface(j), dev, dev->getInterface(j)->nb_endpoint, dev->getName(j)); 00449 for (int i = 0; i < dev->getInterface(j)->nb_endpoint; i++) { 00450 if ((ep = dev->getEndpoint(j, i)) != NULL) { 00451 ed = (HCED *)ep->getHCED(); 00452 ed->control |= (1 << 14); //sKip bit 00453 unqueueEndpoint(ep); 00454 00455 freeTD((volatile uint8_t*)ep->getTDList()[0]); 00456 freeTD((volatile uint8_t*)ep->getTDList()[1]); 00457 00458 freeED((uint8_t *)ep->getHCED()); 00459 } 00460 printList(BULK_ENDPOINT); 00461 printList(INTERRUPT_ENDPOINT); 00462 } 00463 USB_INFO("Device disconnected [%p - %s - hub: %d - port: %d]", dev, dev->getName(j), dev->getHub(), dev->getPort()); 00464 } 00465 } 00466 dev->disconnect(); 00467 } 00468 } 00469 00470 00471 void USBHost::unqueueEndpoint(USBEndpoint * ep) 00472 { 00473 USBEndpoint * prec = NULL; 00474 USBEndpoint * current = NULL; 00475 00476 for (int i = 0; i < 2; i++) { 00477 current = (i == 0) ? (USBEndpoint*)headBulkEndpoint : (USBEndpoint*)headInterruptEndpoint; 00478 prec = current; 00479 while (current != NULL) { 00480 if (current == ep) { 00481 if (current->nextEndpoint() != NULL) { 00482 prec->queueEndpoint(current->nextEndpoint()); 00483 if (current == headBulkEndpoint) { 00484 updateBulkHeadED((uint32_t)current->nextEndpoint()->getHCED()); 00485 headBulkEndpoint = current->nextEndpoint(); 00486 } else if (current == headInterruptEndpoint) { 00487 updateInterruptHeadED((uint32_t)current->nextEndpoint()->getHCED()); 00488 headInterruptEndpoint = current->nextEndpoint(); 00489 } 00490 } 00491 // here we are dequeuing the queue of ed 00492 // we need to update the tail pointer 00493 else { 00494 prec->queueEndpoint(NULL); 00495 if (current == headBulkEndpoint) { 00496 updateBulkHeadED(0); 00497 headBulkEndpoint = current->nextEndpoint(); 00498 } else if (current == headInterruptEndpoint) { 00499 updateInterruptHeadED(0); 00500 headInterruptEndpoint = current->nextEndpoint(); 00501 } 00502 00503 // modify tail 00504 switch (current->getType()) { 00505 case BULK_ENDPOINT: 00506 tailBulkEndpoint = prec; 00507 break; 00508 case INTERRUPT_ENDPOINT: 00509 tailInterruptEndpoint = prec; 00510 break; 00511 default: 00512 break; 00513 } 00514 } 00515 current->setState(USB_TYPE_FREE); 00516 return; 00517 } 00518 prec = current; 00519 current = current->nextEndpoint(); 00520 } 00521 } 00522 } 00523 00524 00525 USBDeviceConnected * USBHost::getDevice(uint8_t index) 00526 { 00527 if ((index >= MAX_DEVICE_CONNECTED) || (!deviceInUse[index])) { 00528 return NULL; 00529 } 00530 return (USBDeviceConnected*)&devices[index]; 00531 } 00532 00533 // create an USBEndpoint descriptor. the USBEndpoint is not linked 00534 USBEndpoint * USBHost::newEndpoint(ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir, uint32_t size, uint8_t addr) 00535 { 00536 int i = 0; 00537 HCED * ed = (HCED *)getED(); 00538 HCTD* td_list[2] = { (HCTD*)getTD(), (HCTD*)getTD() }; 00539 00540 memset((void *)td_list[0], 0x00, sizeof(HCTD)); 00541 memset((void *)td_list[1], 0x00, sizeof(HCTD)); 00542 00543 // search a free USBEndpoint 00544 for (i = 0; i < MAX_ENDPOINT; i++) { 00545 if (endpoints[i].getState() == USB_TYPE_FREE) { 00546 endpoints[i].init(ed, type, dir, size, addr, td_list); 00547 USB_DBG("USBEndpoint created (%p): type: %d, dir: %d, size: %d, addr: %d, state: %s", &endpoints[i], type, dir, size, addr, endpoints[i].getStateString()); 00548 return &endpoints[i]; 00549 } 00550 } 00551 USB_ERR("could not allocate more endpoints!!!!"); 00552 return NULL; 00553 } 00554 00555 00556 USB_TYPE USBHost::resetDevice(USBDeviceConnected * dev) 00557 { 00558 int index = findDevice(dev); 00559 if (index != -1) { 00560 USB_DBG("Resetting hub %d, port %d\n", dev->getHub(), dev->getPort()); 00561 ThisThread::sleep_for(100); 00562 if (dev->getHub() == 0) { 00563 resetRootHub(); 00564 } 00565 #if MAX_HUB_NB 00566 else { 00567 dev->getHubParent()->portReset(dev->getPort()); 00568 } 00569 #endif 00570 ThisThread::sleep_for(100); 00571 deviceReset[index] = true; 00572 return USB_TYPE_OK; 00573 } 00574 00575 return USB_TYPE_ERROR; 00576 } 00577 00578 // link the USBEndpoint to the linked list and attach an USBEndpoint to a device 00579 bool USBHost::addEndpoint(USBDeviceConnected * dev, uint8_t intf_nb, USBEndpoint * ep) 00580 { 00581 00582 if (ep == NULL) { 00583 return false; 00584 } 00585 00586 HCED * prevEd; 00587 00588 // set device address in the USBEndpoint descriptor 00589 if (dev == NULL) { 00590 ep->setDeviceAddress(0); 00591 } else { 00592 ep->setDeviceAddress(dev->getAddress()); 00593 } 00594 00595 if ((dev != NULL) && dev->getSpeed()) { 00596 ep->setSpeed(dev->getSpeed()); 00597 } 00598 00599 ep->setIntfNb(intf_nb); 00600 00601 // queue the new USBEndpoint on the ED list 00602 switch (ep->getType()) { 00603 00604 case CONTROL_ENDPOINT: 00605 prevEd = ( HCED*) controlHeadED(); 00606 if (!prevEd) { 00607 updateControlHeadED((uint32_t) ep->getHCED()); 00608 USB_DBG_TRANSFER("First control USBEndpoint: %08X", (uint32_t) ep->getHCED()); 00609 headControlEndpoint = ep; 00610 tailControlEndpoint = ep; 00611 return true; 00612 } 00613 tailControlEndpoint->queueEndpoint(ep); 00614 tailControlEndpoint = ep; 00615 return true; 00616 00617 case BULK_ENDPOINT: 00618 prevEd = ( HCED*) bulkHeadED(); 00619 if (!prevEd) { 00620 updateBulkHeadED((uint32_t) ep->getHCED()); 00621 USB_DBG_TRANSFER("First bulk USBEndpoint: %08X\r\n", (uint32_t) ep->getHCED()); 00622 headBulkEndpoint = ep; 00623 tailBulkEndpoint = ep; 00624 break; 00625 } 00626 USB_DBG_TRANSFER("Queue BULK Ed %p after %p\r\n",ep->getHCED(), prevEd); 00627 tailBulkEndpoint->queueEndpoint(ep); 00628 tailBulkEndpoint = ep; 00629 break; 00630 00631 case INTERRUPT_ENDPOINT: 00632 prevEd = ( HCED*) interruptHeadED(); 00633 if (!prevEd) { 00634 updateInterruptHeadED((uint32_t) ep->getHCED()); 00635 USB_DBG_TRANSFER("First interrupt USBEndpoint: %08X\r\n", (uint32_t) ep->getHCED()); 00636 headInterruptEndpoint = ep; 00637 tailInterruptEndpoint = ep; 00638 break; 00639 } 00640 USB_DBG_TRANSFER("Queue INTERRUPT Ed %p after %p\r\n",ep->getHCED(), prevEd); 00641 tailInterruptEndpoint->queueEndpoint(ep); 00642 tailInterruptEndpoint = ep; 00643 break; 00644 default: 00645 return false; 00646 } 00647 00648 ep->dev = dev; 00649 dev->addEndpoint(intf_nb, ep); 00650 00651 return true; 00652 } 00653 00654 00655 int USBHost::findDevice(USBDeviceConnected * dev) 00656 { 00657 for (int i = 0; i < MAX_DEVICE_CONNECTED; i++) { 00658 if (dev == &devices[i]) { 00659 return i; 00660 } 00661 } 00662 return -1; 00663 } 00664 00665 int USBHost::findDevice(uint8_t hub, uint8_t port, USBHostHub * hub_parent) 00666 { 00667 for (int i = 0; i < MAX_DEVICE_CONNECTED; i++) { 00668 if (devices[i].getHub() == hub && devices[i].getPort() == port) { 00669 if (hub_parent != NULL) { 00670 if (hub_parent == devices[i].getHubParent()) 00671 return i; 00672 } else { 00673 return i; 00674 } 00675 } 00676 } 00677 return -1; 00678 } 00679 00680 void USBHost::printList(ENDPOINT_TYPE type) 00681 { 00682 #if DEBUG_EP_STATE 00683 volatile HCED * hced; 00684 switch(type) { 00685 case CONTROL_ENDPOINT: 00686 hced = (HCED *)controlHeadED(); 00687 break; 00688 case BULK_ENDPOINT: 00689 hced = (HCED *)bulkHeadED(); 00690 break; 00691 case INTERRUPT_ENDPOINT: 00692 hced = (HCED *)interruptHeadED(); 00693 break; 00694 } 00695 volatile HCTD * hctd = NULL; 00696 const char * type_str = (type == BULK_ENDPOINT) ? "BULK" : 00697 ((type == INTERRUPT_ENDPOINT) ? "INTERRUPT" : 00698 ((type == CONTROL_ENDPOINT) ? "CONTROL" : "ISOCHRONOUS")); 00699 USB_DBG_X("State of %s:\r\n", type_str); 00700 while (hced != NULL) { 00701 uint8_t dir = ((hced->control & (3 << 11)) >> 11); 00702 USB_DBG_X("hced: %p [ADDR: %d, DIR: %s, EP_NB: 0x%X]\r\n", hced, 00703 hced->control & 0x7f, 00704 (dir == 1) ? "OUT" : ((dir == 0) ? "FROM_TD":"IN"), 00705 (hced->control & (0xf << 7)) >> 7); 00706 hctd = (HCTD *)((uint32_t)(hced->headTD) & ~(0xf)); 00707 while (hctd != hced->tailTD) { 00708 USB_DBG_X("\thctd: %p [DIR: %s]\r\n", hctd, ((hctd->control & (3 << 19)) >> 19) == 1 ? "OUT" : "IN"); 00709 hctd = hctd->nextTD; 00710 } 00711 USB_DBG_X("\thctd: %p\r\n", hctd); 00712 hced = hced->nextED; 00713 } 00714 USB_DBG_X("\r\n\r\n"); 00715 #endif 00716 } 00717 00718 00719 // add a transfer on the TD linked list 00720 USB_TYPE USBHost::addTransfer(USBEndpoint * ed, uint8_t * buf, uint32_t len) 00721 { 00722 ASSERT_MEM_REGION(buf); 00723 00724 00725 td_mutex.lock(); 00726 00727 // allocate a TD which will be freed in TDcompletion 00728 volatile HCTD * td = ed->getNextTD(); 00729 if (td == NULL) { 00730 return USB_TYPE_ERROR; 00731 } 00732 00733 uint32_t token = (ed->isSetup() ? TD_SETUP : ( (ed->getDir() == IN) ? TD_IN : TD_OUT )); 00734 00735 uint32_t td_toggle; 00736 00737 if (ed->getType() == CONTROL_ENDPOINT) { 00738 if (ed->isSetup()) { 00739 td_toggle = TD_TOGGLE_0; 00740 } else { 00741 td_toggle = TD_TOGGLE_1; 00742 } 00743 } else { 00744 td_toggle = 0; 00745 } 00746 00747 td->control = (TD_ROUNDING | token | TD_DELAY_INT(0) | td_toggle | TD_CC); 00748 td->currBufPtr = buf; 00749 td->bufEnd = (buf + (len - 1)); 00750 00751 ENDPOINT_TYPE type = ed->getType(); 00752 00753 disableList(type); 00754 ed->queueTransfer(); 00755 printList(type); 00756 enableList(type); 00757 00758 td_mutex.unlock(); 00759 00760 return USB_TYPE_PROCESSING; 00761 } 00762 00763 00764 00765 USB_TYPE USBHost::getDeviceDescriptor(USBDeviceConnected * dev, uint8_t * buf, uint16_t max_len_buf, uint16_t * len_dev_descr) 00766 { 00767 USB_TYPE t = controlRead( dev, 00768 USB_DEVICE_TO_HOST | USB_RECIPIENT_DEVICE, 00769 GET_DESCRIPTOR, 00770 (DEVICE_DESCRIPTOR << 8) | (0), 00771 0, buf, MIN(DEVICE_DESCRIPTOR_LENGTH, max_len_buf)); 00772 if (len_dev_descr) 00773 *len_dev_descr = MIN(DEVICE_DESCRIPTOR_LENGTH, max_len_buf); 00774 00775 return t; 00776 } 00777 00778 USB_TYPE USBHost::getConfigurationDescriptor(USBDeviceConnected * dev, uint8_t * buf, uint16_t max_len_buf, uint16_t * len_conf_descr) 00779 { 00780 USB_TYPE res; 00781 uint16_t total_conf_descr_length = 0; 00782 00783 // fourth step: get the beginning of the configuration descriptor to have the total length of the conf descr 00784 res = controlRead( dev, 00785 USB_DEVICE_TO_HOST | USB_RECIPIENT_DEVICE, 00786 GET_DESCRIPTOR, 00787 (CONFIGURATION_DESCRIPTOR << 8) | (0), 00788 0, buf, CONFIGURATION_DESCRIPTOR_LENGTH); 00789 00790 if (res != USB_TYPE_OK) { 00791 USB_ERR("GET CONF 1 DESCR FAILED"); 00792 return res; 00793 } 00794 total_conf_descr_length = buf[2] | (buf[3] << 8); 00795 total_conf_descr_length = MIN(max_len_buf, total_conf_descr_length); 00796 00797 if (len_conf_descr) 00798 *len_conf_descr = total_conf_descr_length; 00799 00800 USB_DBG("TOTAL_LENGTH: %d \t NUM_INTERF: %d", total_conf_descr_length, buf[4]); 00801 00802 return controlRead( dev, 00803 USB_DEVICE_TO_HOST | USB_RECIPIENT_DEVICE, 00804 GET_DESCRIPTOR, 00805 (CONFIGURATION_DESCRIPTOR << 8) | (0), 00806 0, buf, total_conf_descr_length); 00807 } 00808 00809 00810 USB_TYPE USBHost::setAddress(USBDeviceConnected * dev, uint8_t address) { 00811 return controlWrite( dev, 00812 USB_HOST_TO_DEVICE | USB_RECIPIENT_DEVICE, 00813 SET_ADDRESS, 00814 address, 00815 0, NULL, 0); 00816 00817 } 00818 00819 USB_TYPE USBHost::setConfiguration(USBDeviceConnected * dev, uint8_t conf) 00820 { 00821 return controlWrite( dev, 00822 USB_HOST_TO_DEVICE | USB_RECIPIENT_DEVICE, 00823 SET_CONFIGURATION, 00824 conf, 00825 0, NULL, 0); 00826 } 00827 00828 uint8_t USBHost::numberDriverAttached(USBDeviceConnected * dev) { 00829 int index = findDevice(dev); 00830 uint8_t cnt = 0; 00831 if (index == -1) 00832 return 0; 00833 for (uint8_t i = 0; i < MAX_INTF; i++) { 00834 if (deviceAttachedDriver[index][i]) 00835 cnt++; 00836 } 00837 return cnt; 00838 } 00839 00840 // enumerate a device with the control USBEndpoint 00841 USB_TYPE USBHost::enumerate(USBDeviceConnected * dev, IUSBEnumerator* pEnumerator) 00842 { 00843 uint16_t total_conf_descr_length = 0; 00844 USB_TYPE res; 00845 00846 do 00847 { 00848 Lock lock(this); 00849 00850 // don't enumerate a device which all interfaces are registered to a specific driver 00851 int index = findDevice(dev); 00852 00853 if (index == -1) { 00854 return USB_TYPE_ERROR; 00855 } 00856 00857 uint8_t nb_intf_attached = numberDriverAttached(dev); 00858 USB_DBG("dev: %p nb_intf: %d", dev, dev->getNbIntf()); 00859 USB_DBG("dev: %p nb_intf_attached: %d", dev, nb_intf_attached); 00860 if ((nb_intf_attached != 0) && (dev->getNbIntf() == nb_intf_attached)) { 00861 USB_DBG("Don't enumerate dev: %p because all intf are registered with a driver", dev); 00862 return USB_TYPE_OK; 00863 } 00864 00865 USB_DBG("Enumerate dev: %p", dev); 00866 00867 // third step: get the whole device descriptor to see vid, pid 00868 res = getDeviceDescriptor(dev, data, DEVICE_DESCRIPTOR_LENGTH); 00869 00870 if (res != USB_TYPE_OK) { 00871 USB_DBG("GET DEV DESCR FAILED"); 00872 return res; 00873 } 00874 00875 dev->setClass(data[4]); 00876 dev->setSubClass(data[5]); 00877 dev->setProtocol(data[6]); 00878 dev->setVid(data[8] | (data[9] << 8)); 00879 dev->setPid(data[10] | (data[11] << 8)); 00880 USB_DBG("CLASS: %02X \t VID: %04X \t PID: %04X", data[4], data[8] | (data[9] << 8), data[10] | (data[11] << 8)); 00881 00882 pEnumerator->setVidPid( data[8] | (data[9] << 8), data[10] | (data[11] << 8) ); 00883 00884 res = getConfigurationDescriptor(dev, data, SAFE_USB_HOST_DATA_BUFF_SIZE/*sizeof(data)*/, &total_conf_descr_length); 00885 if (res != USB_TYPE_OK) { 00886 return res; 00887 } 00888 00889 #if (DEBUG > 3) 00890 USB_DBG("CONFIGURATION DESCRIPTOR:\r\n"); 00891 for (int i = 0; i < total_conf_descr_length; i++) 00892 USB_DBG_X("%02X ", data[i]); 00893 USB_DBG_X("\r\n\r\n"); 00894 #endif 00895 00896 // Parse the configuration descriptor 00897 parseConfDescr(dev, data, total_conf_descr_length, pEnumerator); 00898 00899 // only set configuration if not enumerated before 00900 if (!dev->isEnumerated()) { 00901 00902 USB_DBG("Set configuration 1 on dev: %p", dev); 00903 // sixth step: set configuration (only 1 supported) 00904 res = setConfiguration(dev, 1); 00905 00906 if (res != USB_TYPE_OK) { 00907 USB_DBG("SET CONF FAILED"); 00908 return res; 00909 } 00910 } 00911 00912 dev->setEnumerated(); 00913 00914 // Now the device is enumerated! 00915 USB_DBG("dev %p is enumerated\r\n", dev); 00916 00917 } while(0); 00918 00919 // Some devices may require this delay 00920 ThisThread::sleep_for(100); 00921 00922 return USB_TYPE_OK; 00923 } 00924 // this method fills the USBDeviceConnected object: class,.... . It also add endpoints found in the descriptor. 00925 void USBHost::parseConfDescr(USBDeviceConnected * dev, uint8_t * conf_descr, uint32_t len, IUSBEnumerator* pEnumerator) 00926 { 00927 uint32_t index = 0; 00928 uint32_t len_desc = 0; 00929 uint8_t id = 0; 00930 int nb_endpoints_used = 0; 00931 USBEndpoint * ep = NULL; 00932 uint8_t intf_nb = 0; 00933 bool parsing_intf = false; 00934 uint8_t current_intf = 0; 00935 00936 while (index < len) { 00937 len_desc = conf_descr[index]; 00938 id = conf_descr[index+1]; 00939 switch (id) { 00940 case CONFIGURATION_DESCRIPTOR: 00941 USB_DBG("dev: %p has %d intf", dev, conf_descr[4]); 00942 dev->setNbIntf(conf_descr[4]); 00943 break; 00944 case INTERFACE_DESCRIPTOR: 00945 if(pEnumerator->parseInterface(conf_descr[index + 2], conf_descr[index + 5], conf_descr[index + 6], conf_descr[index + 7])) { 00946 if (intf_nb++ <= MAX_INTF) { 00947 current_intf = conf_descr[index + 2]; 00948 dev->addInterface(current_intf, conf_descr[index + 5], conf_descr[index + 6], conf_descr[index + 7]); 00949 nb_endpoints_used = 0; 00950 USB_DBG("ADD INTF %d on device %p: class: %d, subclass: %d, proto: %d", current_intf, dev, conf_descr[index + 5],conf_descr[index + 6],conf_descr[index + 7]); 00951 } else { 00952 USB_DBG("Drop intf..."); 00953 } 00954 parsing_intf = true; 00955 } else { 00956 parsing_intf = false; 00957 } 00958 break; 00959 case ENDPOINT_DESCRIPTOR: 00960 if (parsing_intf && (intf_nb <= MAX_INTF) ) { 00961 if (nb_endpoints_used < MAX_ENDPOINT_PER_INTERFACE) { 00962 if( pEnumerator->useEndpoint(current_intf, (ENDPOINT_TYPE)(conf_descr[index + 3] & 0x03), (ENDPOINT_DIRECTION)((conf_descr[index + 2] >> 7) + 1)) ) { 00963 // if the USBEndpoint is isochronous -> skip it (TODO: fix this) 00964 if ((conf_descr[index + 3] & 0x03) != ISOCHRONOUS_ENDPOINT) { 00965 ep = newEndpoint((ENDPOINT_TYPE)(conf_descr[index+3] & 0x03), 00966 (ENDPOINT_DIRECTION)((conf_descr[index + 2] >> 7) + 1), 00967 conf_descr[index + 4] | (conf_descr[index + 5] << 8), 00968 conf_descr[index + 2] & 0x0f); 00969 USB_DBG("ADD USBEndpoint %p, on interf %d on device %p", ep, current_intf, dev); 00970 if (ep != NULL && dev != NULL) { 00971 addEndpoint(dev, current_intf, ep); 00972 } else { 00973 USB_DBG("EP NULL"); 00974 } 00975 nb_endpoints_used++; 00976 } else { 00977 USB_DBG("ISO USBEndpoint NOT SUPPORTED"); 00978 } 00979 } 00980 } 00981 } 00982 break; 00983 case HID_DESCRIPTOR: 00984 lenReportDescr = conf_descr[index + 7] | (conf_descr[index + 8] << 8); 00985 break; 00986 default: 00987 break; 00988 } 00989 index += len_desc; 00990 } 00991 } 00992 00993 00994 USB_TYPE USBHost::bulkWrite(USBDeviceConnected * dev, USBEndpoint * ep, uint8_t * buf, uint32_t len, bool blocking) 00995 { 00996 return generalTransfer(dev, ep, buf, len, blocking, BULK_ENDPOINT, true); 00997 } 00998 00999 USB_TYPE USBHost::bulkRead(USBDeviceConnected * dev, USBEndpoint * ep, uint8_t * buf, uint32_t len, bool blocking) 01000 { 01001 return generalTransfer(dev, ep, buf, len, blocking, BULK_ENDPOINT, false); 01002 } 01003 01004 USB_TYPE USBHost::interruptWrite(USBDeviceConnected * dev, USBEndpoint * ep, uint8_t * buf, uint32_t len, bool blocking) 01005 { 01006 return generalTransfer(dev, ep, buf, len, blocking, INTERRUPT_ENDPOINT, true); 01007 } 01008 01009 USB_TYPE USBHost::interruptRead(USBDeviceConnected * dev, USBEndpoint * ep, uint8_t * buf, uint32_t len, bool blocking) 01010 { 01011 return generalTransfer(dev, ep, buf, len, blocking, INTERRUPT_ENDPOINT, false); 01012 } 01013 01014 USB_TYPE USBHost::generalTransfer(USBDeviceConnected * dev, USBEndpoint * ep, uint8_t * buf, uint32_t len, bool blocking, ENDPOINT_TYPE type, bool write) 01015 { 01016 01017 #if DEBUG_TRANSFER 01018 const char * type_str = (type == BULK_ENDPOINT) ? "BULK" : ((type == INTERRUPT_ENDPOINT) ? "INTERRUPT" : "ISOCHRONOUS"); 01019 USB_DBG_TRANSFER("----- %s %s [dev: %p - %s - hub: %d - port: %d - addr: %d - ep: %02X]------", type_str, (write) ? "WRITE" : "READ", dev, dev->getName(ep->getIntfNb()), dev->getHub(), dev->getPort(), dev->getAddress(), ep->getAddress()); 01020 #endif 01021 01022 Lock lock(this); 01023 01024 USB_TYPE res; 01025 ENDPOINT_DIRECTION dir = (write) ? OUT : IN; 01026 01027 if (dev == NULL) { 01028 USB_ERR("dev NULL"); 01029 return USB_TYPE_ERROR; 01030 } 01031 01032 if (ep == NULL) { 01033 USB_ERR("ep NULL"); 01034 return USB_TYPE_ERROR; 01035 } 01036 01037 if (ep->getState() != USB_TYPE_IDLE) { 01038 USB_WARN("[ep: %p - dev: %p - %s] NOT IDLE: %s", ep, ep->dev, ep->dev->getName(ep->getIntfNb()), ep->getStateString()); 01039 return ep->getState(); 01040 } 01041 01042 if ((ep->getDir() != dir) || (ep->getType() != type)) { 01043 USB_ERR("[ep: %p - dev: %p] wrong dir or bad USBEndpoint type", ep, ep->dev); 01044 return USB_TYPE_ERROR; 01045 } 01046 01047 if (dev->getAddress() != ep->getDeviceAddress()) { 01048 USB_ERR("[ep: %p - dev: %p] USBEndpoint addr and device addr don't match", ep, ep->dev); 01049 return USB_TYPE_ERROR; 01050 } 01051 01052 #if DEBUG_TRANSFER 01053 if (write) { 01054 USB_DBG_TRANSFER("%s WRITE buffer", type_str); 01055 for (int i = 0; i < ep->getLengthTransferred(); i++) 01056 USB_DBG_X("%02X ", buf[i]); 01057 USB_DBG_X("\r\n\r\n"); 01058 } 01059 #endif 01060 addTransfer(ep, buf, len); 01061 01062 if (blocking) { 01063 01064 ep->ep_queue.get(); 01065 res = ep->getState(); 01066 01067 USB_DBG_TRANSFER("%s TRANSFER res: %s on ep: %p\r\n", type_str, ep->getStateString(), ep); 01068 01069 if (res != USB_TYPE_IDLE) { 01070 return res; 01071 } 01072 01073 return USB_TYPE_OK; 01074 } 01075 01076 return USB_TYPE_PROCESSING; 01077 01078 } 01079 01080 01081 USB_TYPE USBHost::controlRead(USBDeviceConnected * dev, uint8_t requestType, uint8_t request, uint32_t value, uint32_t index, uint8_t * buf, uint32_t len) { 01082 return controlTransfer(dev, requestType, request, value, index, buf, len, false); 01083 } 01084 01085 USB_TYPE USBHost::controlWrite(USBDeviceConnected * dev, uint8_t requestType, uint8_t request, uint32_t value, uint32_t index, uint8_t * buf, uint32_t len) { 01086 return controlTransfer(dev, requestType, request, value, index, buf, len, true); 01087 } 01088 01089 USB_TYPE USBHost::controlTransfer(USBDeviceConnected * dev, uint8_t requestType, uint8_t request, uint32_t value, uint32_t index, uint8_t * buf, uint32_t len, bool write) 01090 { 01091 Lock lock(this); 01092 USB_DBG_TRANSFER("----- CONTROL %s [dev: %p - hub: %d - port: %d] ------", (write) ? "WRITE" : "READ", dev, dev->getHub(), dev->getPort()); 01093 01094 int length_transfer = len; 01095 USB_TYPE res; 01096 uint32_t token; 01097 01098 control->setSpeed(dev->getSpeed()); 01099 control->setSize(dev->getSizeControlEndpoint()); 01100 if (dev->isActiveAddress()) { 01101 control->setDeviceAddress(dev->getAddress()); 01102 } else { 01103 control->setDeviceAddress(0); 01104 } 01105 01106 USB_DBG_TRANSFER("Control transfer on device: %d\r\n", control->getDeviceAddress()); 01107 fillControlBuf(requestType, request, value, index, len); 01108 01109 #if DEBUG_TRANSFER 01110 USB_DBG_TRANSFER("SETUP PACKET: "); 01111 for (int i = 0; i < 8; i++) 01112 USB_DBG_X("%01X ", setupPacket[i]); 01113 USB_DBG_X("\r\n"); 01114 #endif 01115 01116 control->setNextToken(TD_SETUP); 01117 addTransfer(control, (uint8_t*)setupPacket, SAFE_USB_HOST_SETUP_PACKET_BUFF_SIZE); 01118 01119 control->ep_queue.get(); 01120 res = control->getState(); 01121 01122 USB_DBG_TRANSFER("CONTROL setup stage %s", control->getStateString()); 01123 01124 if (res != USB_TYPE_IDLE) { 01125 return res; 01126 } 01127 01128 if (length_transfer) { 01129 token = (write) ? TD_OUT : TD_IN; 01130 control->setNextToken(token); 01131 01132 addTransfer(control, (uint8_t *)buf, length_transfer); 01133 01134 control->ep_queue.get(); 01135 res = control->getState(); 01136 01137 #if DEBUG_TRANSFER 01138 USB_DBG_TRANSFER("CONTROL %s stage %s", (write) ? "WRITE" : "READ", control->getStateString()); 01139 if (write) { 01140 USB_DBG_TRANSFER("CONTROL WRITE buffer"); 01141 for (int i = 0; i < control->getLengthTransferred(); i++) 01142 USB_DBG_X("%02X ", buf[i]); 01143 USB_DBG_X("\r\n\r\n"); 01144 } else { 01145 USB_DBG_TRANSFER("CONTROL READ SUCCESS [%d bytes transferred]", control->getLengthTransferred()); 01146 for (int i = 0; i < control->getLengthTransferred(); i++) 01147 USB_DBG_X("%02X ", buf[i]); 01148 USB_DBG_X("\r\n\r\n"); 01149 } 01150 #endif 01151 01152 if (res != USB_TYPE_IDLE) { 01153 return res; 01154 } 01155 } 01156 01157 token = (write) ? TD_IN : TD_OUT; 01158 control->setNextToken(token); 01159 01160 addTransfer(control, NULL, 0); 01161 01162 control->ep_queue.get(); 01163 res = control->getState(); 01164 01165 USB_DBG_TRANSFER("CONTROL ack stage %s", control->getStateString()); 01166 01167 if (res != USB_TYPE_IDLE) 01168 return res; 01169 01170 return USB_TYPE_OK; 01171 } 01172 01173 01174 void USBHost::fillControlBuf(uint8_t requestType, uint8_t request, uint16_t value, uint16_t index, int len) 01175 { 01176 setupPacket[0] = requestType; 01177 setupPacket[1] = request; 01178 setupPacket[2] = (uint8_t) value; 01179 setupPacket[3] = (uint8_t) (value >> 8); 01180 setupPacket[4] = (uint8_t) index; 01181 setupPacket[5] = (uint8_t) (index >> 8); 01182 setupPacket[6] = (uint8_t) len; 01183 setupPacket[7] = (uint8_t) (len >> 8); 01184 }
Generated on Tue Jul 12 2022 21:45:29 by
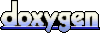