Adaptation of the official mbed USBHost repository to work with the LPC4088 Display Module
Dependents: DMSupport DMSupport DMSupport DMSupport
Fork of DM_USBHost by
MtxCircBuffer.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MTXCIRCBUFFER_H 00018 #define MTXCIRCBUFFER_H 00019 00020 #include "stdint.h" 00021 #include "rtos.h" 00022 00023 //Mutex protected circular buffer 00024 template<typename T, int size> 00025 class MtxCircBuffer { 00026 public: 00027 00028 MtxCircBuffer() { 00029 write = 0; 00030 read = 0; 00031 } 00032 00033 bool isFull() { 00034 mtx.lock(); 00035 bool r = (((write + 1) % size) == read); 00036 mtx.unlock(); 00037 return r; 00038 } 00039 00040 bool isEmpty() { 00041 mtx.lock(); 00042 bool r = (read == write); 00043 mtx.unlock(); 00044 return r; 00045 } 00046 00047 void flush() { 00048 write = 0; 00049 read = 0; 00050 } 00051 00052 void queue(T k) { 00053 mtx.lock(); 00054 while (((write + 1) % size) == read) { 00055 mtx.unlock(); 00056 Thread::wait(10); 00057 mtx.lock(); 00058 } 00059 buf[write++] = k; 00060 write %= size; 00061 mtx.unlock(); 00062 } 00063 00064 uint16_t available() { 00065 mtx.lock(); 00066 uint16_t a = (write >= read) ? (write - read) : (size - read + write); 00067 mtx.unlock(); 00068 return a; 00069 } 00070 00071 bool dequeue(T * c) { 00072 mtx.lock(); 00073 bool empty = (read == write); 00074 if (!empty) { 00075 *c = buf[read++]; 00076 read %= size; 00077 } 00078 mtx.unlock(); 00079 return (!empty); 00080 } 00081 00082 private: 00083 volatile uint16_t write; 00084 volatile uint16_t read; 00085 volatile T buf[size]; 00086 Mutex mtx; 00087 }; 00088 00089 #endif
Generated on Tue Jul 12 2022 21:45:29 by
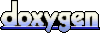