Adaptation of the official mbed USBHost repository to work with the LPC4088 Display Module
Dependents: DMSupport DMSupport DMSupport DMSupport
Fork of DM_USBHost by
USBEndpoint.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef USBENDPOINT_H 00018 #define USBENDPOINT_H 00019 00020 #include "FunctionPointer.h" 00021 #include "USBHostTypes.h" 00022 #include "rtos.h" 00023 00024 class USBDeviceConnected; 00025 00026 /** 00027 * USBEndpoint class 00028 */ 00029 class USBEndpoint 00030 { 00031 public: 00032 /** 00033 * Constructor 00034 */ 00035 USBEndpoint() { 00036 state = USB_TYPE_FREE; 00037 nextEp = NULL; 00038 }; 00039 00040 /** 00041 * Initialize an endpoint 00042 * 00043 * @param hced hced associated to the endpoint 00044 * @param type endpoint type 00045 * @param dir endpoint direction 00046 * @param size endpoint size 00047 * @param ep_number endpoint number 00048 * @param td_list array of two allocated transfer descriptors 00049 */ 00050 void init(HCED * hced, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir, uint32_t size, uint8_t ep_number, HCTD* td_list[2]); 00051 00052 /** 00053 * Set next token. Warning: only useful for the control endpoint 00054 * 00055 * @param token IN, OUT or SETUP token 00056 */ 00057 void setNextToken(uint32_t token); 00058 00059 /** 00060 * Queue an endpoint 00061 * 00062 * @param endpoint endpoint which will be queued in the linked list 00063 */ 00064 void queueEndpoint(USBEndpoint * endpoint); 00065 00066 00067 /** 00068 * Queue a transfer on the endpoint 00069 */ 00070 void queueTransfer(); 00071 00072 /** 00073 * Unqueue a transfer from the endpoint 00074 * 00075 * @param td hctd which will be unqueued 00076 */ 00077 void unqueueTransfer(volatile HCTD * td); 00078 00079 /** 00080 * Attach a member function to call when a transfer is finished 00081 * 00082 * @param tptr pointer to the object to call the member function on 00083 * @param mptr pointer to the member function to be called 00084 */ 00085 template<typename T> 00086 inline void attach(T* tptr, void (T::*mptr)(void)) { 00087 if((mptr != NULL) && (tptr != NULL)) { 00088 rx.attach(tptr, mptr); 00089 } 00090 } 00091 00092 /** 00093 * Attach a callback called when a transfer is finished 00094 * 00095 * @param fptr function pointer 00096 */ 00097 inline void attach(void (*fptr)(void)) { 00098 if(fptr != NULL) { 00099 rx.attach(fptr); 00100 } 00101 } 00102 00103 /** 00104 * Call the handler associted to the end of a transfer 00105 */ 00106 inline void call() { 00107 rx.call(); 00108 }; 00109 00110 00111 // setters 00112 inline void setState(USB_TYPE st) { state = st; } 00113 void setState(uint8_t st); 00114 void setDeviceAddress(uint8_t addr); 00115 inline void setLengthTransferred(int len) { transferred = len; }; 00116 void setSpeed(uint8_t speed); 00117 void setSize(uint32_t size); 00118 inline void setDir(ENDPOINT_DIRECTION d) { dir = d; } 00119 inline void setIntfNb(uint8_t intf_nb_) { intf_nb = intf_nb_; }; 00120 00121 // getters 00122 const char * getStateString(); 00123 inline USB_TYPE getState() { return state; } 00124 inline ENDPOINT_TYPE getType() { return type; }; 00125 inline uint8_t getDeviceAddress() { return hced->control & 0x7f; }; 00126 inline int getLengthTransferred() { return transferred; } 00127 inline uint8_t * getBufStart() { return buf_start; } 00128 inline uint8_t getAddress(){ return address; }; 00129 inline uint32_t getSize() { return (hced->control >> 16) & 0x3ff; }; 00130 inline volatile HCTD * getHeadTD() { return (volatile HCTD*) ((uint32_t)hced->headTD & ~0xF); }; 00131 inline volatile HCTD** getTDList() { return td_list; }; 00132 inline volatile HCED * getHCED() { return hced; }; 00133 inline ENDPOINT_DIRECTION getDir() { return dir; } 00134 inline volatile HCTD * getProcessedTD() { return td_current; }; 00135 inline volatile HCTD* getNextTD() { return td_current; }; 00136 inline bool isSetup() { return setup; } 00137 inline USBEndpoint * nextEndpoint() { return (USBEndpoint*)nextEp; }; 00138 inline uint8_t getIntfNb() { return intf_nb; }; 00139 00140 USBDeviceConnected * dev; 00141 00142 Queue<uint8_t, 1> ep_queue; 00143 00144 private: 00145 ENDPOINT_TYPE type; 00146 volatile USB_TYPE state; 00147 ENDPOINT_DIRECTION dir; 00148 bool setup; 00149 00150 uint8_t address; 00151 00152 int transfer_len; 00153 int transferred; 00154 uint8_t * buf_start; 00155 00156 FunctionPointer rx; 00157 00158 USBEndpoint* nextEp; 00159 00160 // USBEndpoint descriptor 00161 volatile HCED * hced; 00162 00163 volatile HCTD * td_list[2]; 00164 volatile HCTD * td_current; 00165 volatile HCTD * td_next; 00166 00167 uint8_t intf_nb; 00168 00169 }; 00170 00171 #endif
Generated on Tue Jul 12 2022 21:45:29 by
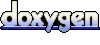