
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
USBMSD_RAMFS.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef USBMSDRAMFS_H 00018 #define USBMSDRAMFS_H 00019 00020 #include "mbed.h" 00021 #include "USBMSD.h" 00022 #include "RAMFileSystem.h" 00023 #include <stdint.h> 00024 00025 /** 00026 * USBMSD_RAMFS class: Allows the mbed board to expose a FAT file system in SDRAM as a USB memory stick 00027 */ 00028 class USBMSD_RAMFS : public USBMSD { 00029 public: 00030 00031 /** 00032 * Constructor 00033 * 00034 * @param ramfs The RAM file system 00035 * @param vendor_id Your vendor_id 00036 * @param product_id Your product_id 00037 * @param product_release Your preoduct_release 00038 */ 00039 USBMSD_RAMFS(/*RAMFileSystem* ramfs*/HeapBlockDevice* ramfs, uint16_t vendor_id = 0x0703, uint16_t product_id = 0x0104, uint16_t product_release = 0x0001); 00040 00041 protected: 00042 #if 0 00043 /* 00044 * read one or more blocks on a storage chip 00045 * 00046 * @param data pointer where will be stored read data 00047 * @param block starting block number 00048 * @param count number of blocks to read 00049 * @returns 0 if successful 00050 */ 00051 virtual int disk_read(uint8_t* data, uint64_t block, uint8_t count); 00052 00053 /* 00054 * write one or more blocks on a storage chip 00055 * 00056 * @param data data to write 00057 * @param block starting block number 00058 * @param count number of blocks to write 00059 * @returns 0 if successful 00060 */ 00061 virtual int disk_write(const uint8_t* data, uint64_t block, uint8_t count); 00062 00063 /* 00064 * Disk initilization 00065 */ 00066 virtual int disk_initialize(); 00067 00068 /* 00069 * Return the number of blocks 00070 * 00071 * @returns number of blocks 00072 */ 00073 virtual uint64_t disk_sectors(); 00074 00075 /* 00076 * Return memory size 00077 * 00078 * @returns memory size 00079 */ 00080 virtual uint64_t disk_size(); 00081 00082 00083 /* 00084 * To check the status of the storage chip 00085 * 00086 * @returns status: 0: OK, 1: disk not initialized, 2: no medium in the drive, 4: write protected 00087 */ 00088 virtual int disk_status(); 00089 #endif 00090 00091 protected: 00092 00093 //RAMFileSystem* ramfs; 00094 }; 00095 00096 #endif
Generated on Tue Jul 12 2022 14:18:31 by
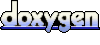